Bjarne Stroustrup, the creator of C++, emphasized practical programming principles that prioritize simplicity, clarity, and efficiency, which can be illustrated through concise code examples such as creating a basic class.
Here's a simple C++ code snippet demonstrating class creation:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark();
return 0;
}
Bjarne Stroustrup: The Father of C++
Bjarne Stroustrup is recognized as the creator of C++, a language that has shaped the landscape of programming since its inception. His innovative approach combined the high-level features of programming with the power of low-level system access, making C++ a versatile tool for software developers across diverse domains. Stroustrup's philosophy underscores the significance of creating efficient software while remaining accessible to developers—an ideology that remains relevant today.
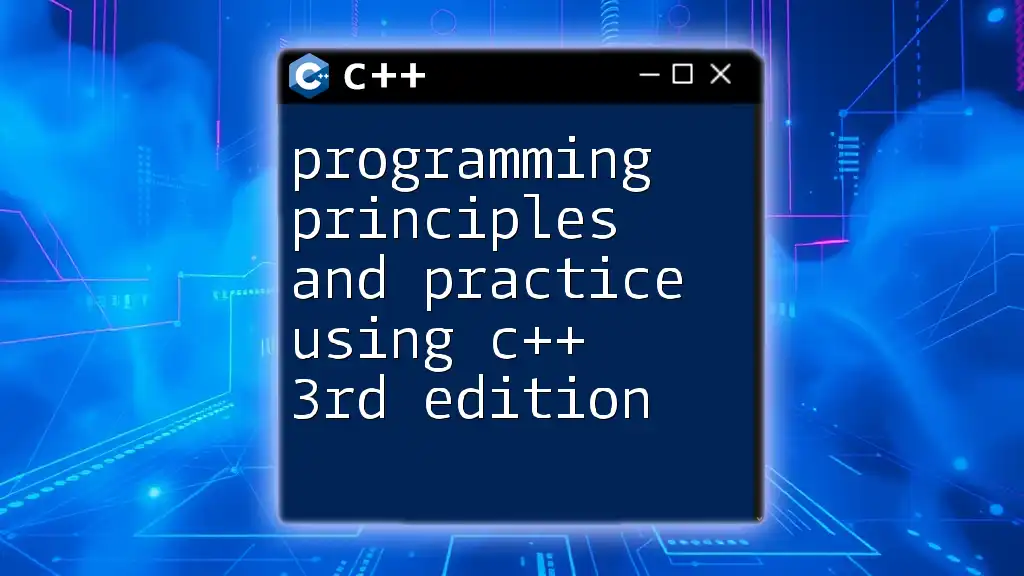
Understanding Programming Principles
Mastering programming principles is not merely an academic exercise; it is vital for anyone looking to excel in software development. Programming principles provide a foundation that leads to the creation of clean, efficient, and maintainable code. Effective programming involves more than just writing functional code; it encompasses best practices that elevate a programmer's ability to structure their solutions clearly and understandably.
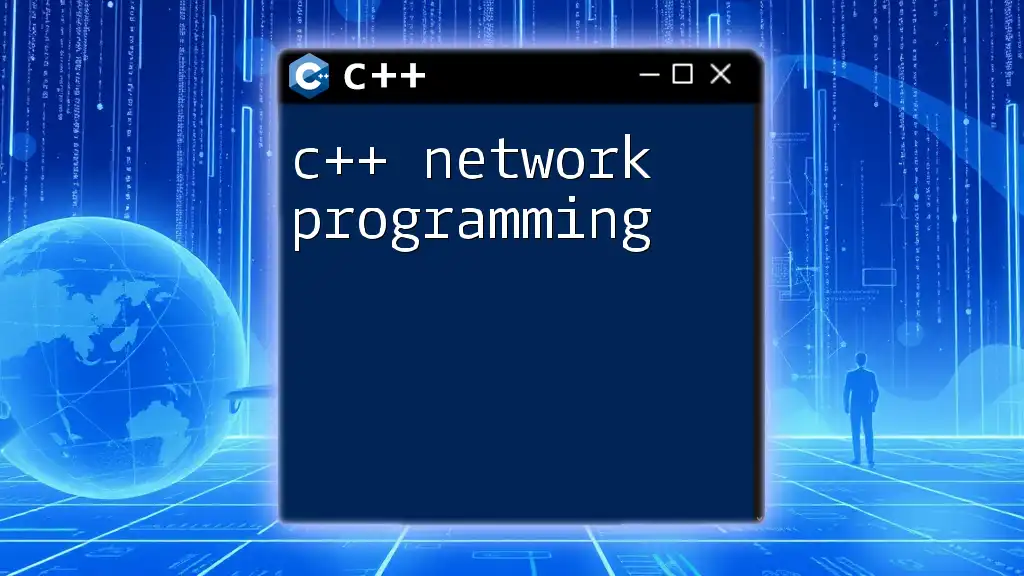
Core Principles from Stroustrup’s Book
Writing Clear Code
One of the cornerstones of Stroustrup’s teachings is the importance of writing clear, understandable code. Code readability plays a significant role in collaboration among teams and future code maintainability. Stroustrup advocates for avoiding unnecessary complexity and emphasizing clarity in every line of code:
// Poor readability
if(x>y){x=x+y;y=x-y;x=x-y;}
// Improved readability
int temp = x + y;
y = x;
x = temp;
By following consistent coding conventions—such as meaningful variable names and organized structure—programmers make their intentions clear, reducing misunderstandings during code reviews and increasing team efficiency.
Use of Abstraction
Abstraction is a powerful concept in programming that allows one to simplify problems by focusing on essential features while hiding unnecessary details. Stroustrup emphasizes leveraging abstraction to manage complexity effectively.
For example, consider a system where you need to manage complex geometrical shapes. By creating abstract classes and interfaces, you can define a base structure while allowing flexibility for future implementations:
class Shape {
public:
virtual double area() const = 0; // Pure virtual function
virtual void draw() const = 0; // Another pure virtual function
};
In this example, the `Shape` class abstracts the fundamentals of a shape without constraining the specific details of each concrete shape, allowing for scalable and organized code development.
Object-Oriented Programming (OOP) Principles
Stroustrup's principles closely align with the foundational concepts of Object-Oriented Programming (OOP). These principles include:
- Encapsulation: Hiding the internal state and requiring all interaction to occur through an object's methods.
- Inheritance: Allowing new classes to receive (or inherit) properties and methods from existing classes, promoting code reuse.
- Polymorphism: Providing a way to perform a single action in different forms, enhancing flexibility in programming.
To demonstrate these principles, consider the following example illustrating encapsulation and inheritance in C++:
class Animal {
protected:
std::string name;
public:
virtual void speak() const = 0; // Polymorphic behavior
};
class Dog : public Animal {
public:
Dog(std::string n) { name = n; }
void speak() const override { std::cout << name << " says Woof!" << std::endl; }
};
class Cat : public Animal {
public:
Cat(std::string n) { name = n; }
void speak() const override { std::cout << name << " says Meow!" << std::endl; }
};
In this code, `Animal` is an abstract class with two derived classes, `Dog` and `Cat`, each implementing the `speak` method differently yet sharing a common interface through polymorphism.
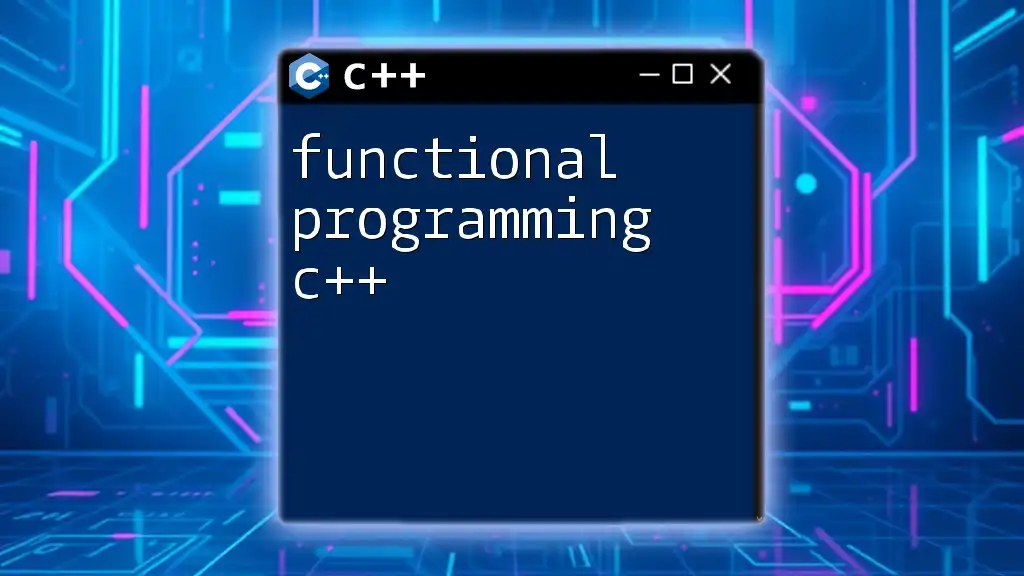
Practical Applications of C++
Understanding Stroustrup’s principles becomes invaluable when applying them to practical programming projects, whether they are small or large in scale.
Case Study: Small Projects
Small projects, such as developing a simple command-line tool, can strongly benefit from Stroustrup’s principles. By incorporating clear code structures and proper abstraction, developers can avoid pitfalls that often lead to technical debt.
For example, creating modular components encourages reusability and makes future enhancements more manageable.
Large Scale Applications
In larger applications, the implications of these principles amplify. Stroustrup's approach helps coders architect systems that are adaptable and maintainable. For instance, applying OOP principles allows for structured data management and encapsulation, which facilitates easier updates and collaborative development across teams.
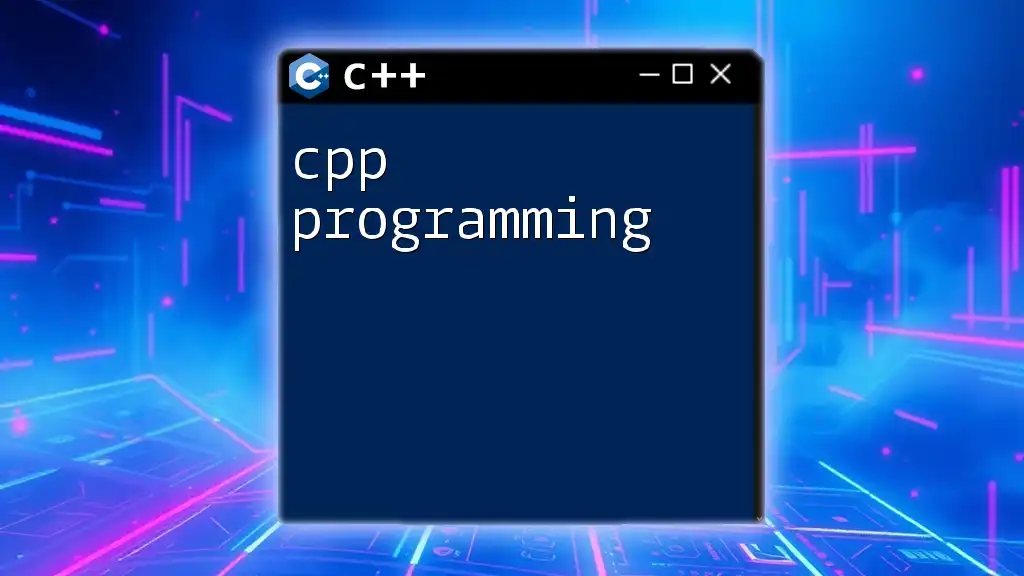
Challenges and Common Pitfalls in C++
Avoiding Common Mistakes
One of the significant challenges in C++ programming is memory management. Understanding pointers and references is essential, as improper handling can lead to memory leaks and software crashes. Stroustrup stresses the importance of managing memory wisely and using smart pointers when appropriate:
#include <memory>
std::unique_ptr<int> p1 = std::make_unique<int>(10);
Using smart pointers can help automate the memory management process, reducing the risk of common pitfalls associated with conventional pointer usage.
Best Practices for Code Maintenance
Regular refactoring is crucial for keeping code maintainable and robust. Stroustrup emphasizes the need for developers to revisit their code, enhancing clarity and simplifying logic over time. This ongoing process ensures that the codebase remains adaptable to changing requirements.
For instance, a simple refactor of a function can dramatically increase readability:
// Before refactoring
int calculate(int a, int b) {
return a * b + (a / b);
}
// After refactoring
int calculateProduct(int a, int b) {
return a * b;
}
int calculateSum(int a, int b) {
return a / b;
}
Refactoring the function into smaller, more manageable pieces enhances clarity and allows individuals to pinpoint specific areas of functionality easily.
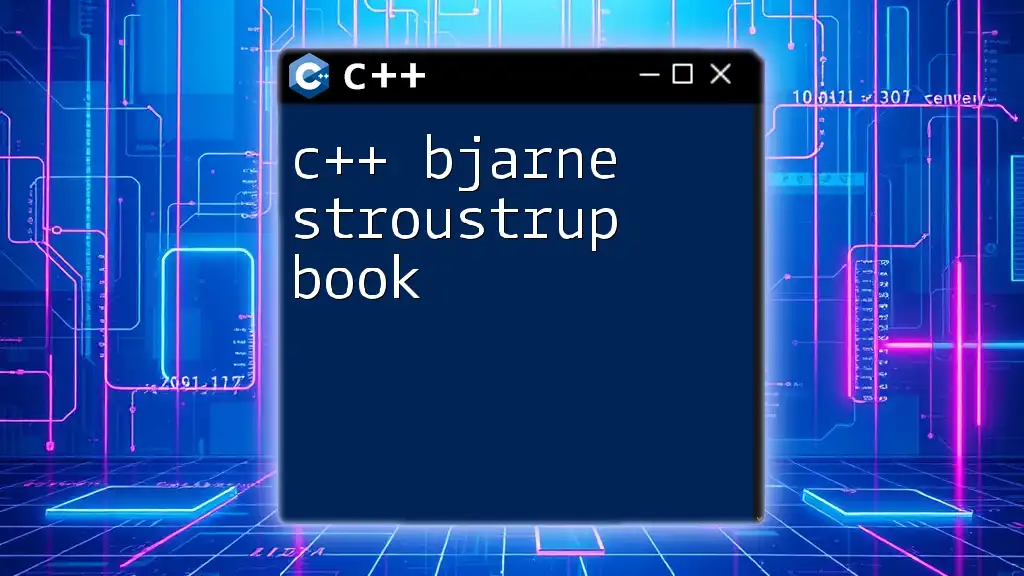
Conclusion
Embracing Bjarne Stroustrup's programming principles and practices using C++ can fundamentally transform a programmer’s approach to development. Through prioritizing readability, abstraction, and OOP principles, developers can craft code that is not only functional but also elegant and maintainable.
As you embark on your journey of learning and mastering C++, don't forget that continuous learning is key. Delving into the recommended resources, such as advanced textbooks and practice platforms, can further deepen your understanding and application of these principles.
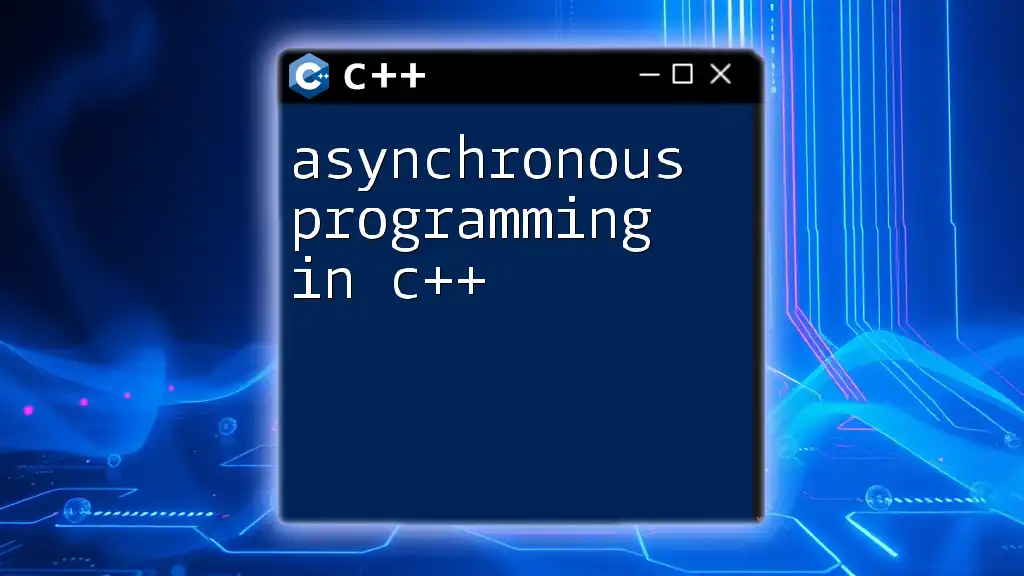
Call to Action
We invite you to share your own experiences with Bjarne Stroustrup’s principles in your programming journey. What challenges have you faced, and how have you applied these practices in your projects? Engage with us in the comments to foster a community of learning and growth in C++.