"Programming Principles and Practice Using C++ (3rd Edition) serves as a comprehensive introduction to programming using C++, emphasizing fundamental concepts and practical applications for beginners."
Here's a simple code snippet demonstrating a basic C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Overview of the Book
Programming Principles and Practice Using C++ 3rd Edition is a fundamental resource for those interested in learning C++. It serves as both an introductory text for novices and a useful reference for more experienced developers aiming to refine their understanding of programming principles.
Importance of Learning C++
Choosing to learn C++ is not just about mastering a language; it's about grasping essential programming concepts that apply to a wide range of programming environments. C++'s enduring popularity in system/software, game development, and performance-critical applications underlines its significance.
Target Audience
This book is particularly well-suited for beginners who may have little to no programming experience. However, it also caters to intermediate programmers eager to deepen their understanding of the principles of software development.
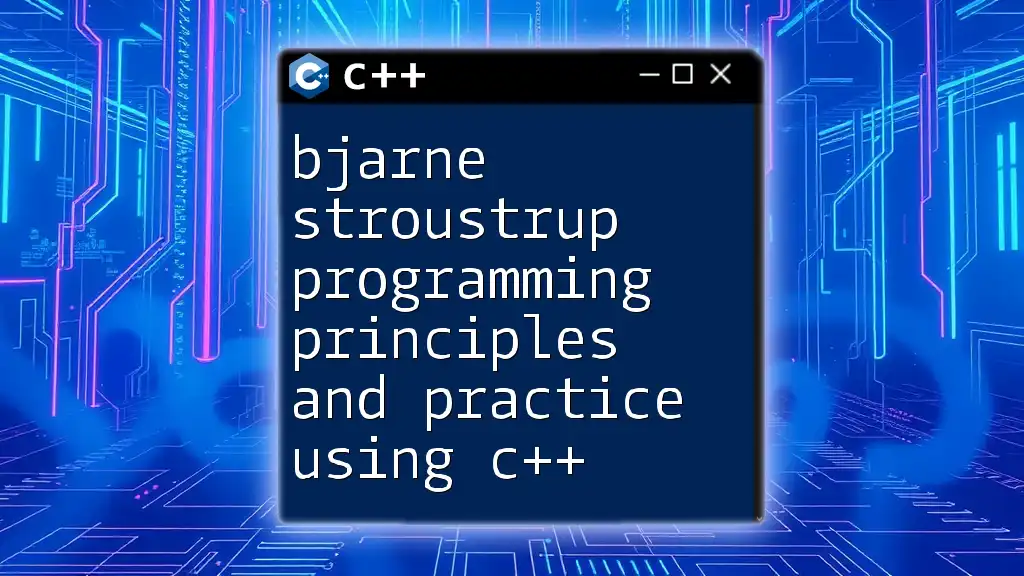
Getting Started with C++
Installing a C++ Compiler
Before diving into coding, setting up a C++ compiler is essential. Popular compilers include GCC, Clang, and MSVC.
-
GCC (GNU Compiler Collection):
- Available on most platforms including Linux, Windows, and macOS.
- Installation can typically be done via package managers. For example, on Ubuntu:
sudo apt-get install g++
-
Clang:
- Known for its excellent diagnostics, it can be installed on macOS or Linux using Homebrew or package managers.
brew install llvm
-
MSVC (Microsoft Visual C++):
- Included with Visual Studio, which can be downloaded from the Microsoft website.
To test your installation, execute a simple program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up an Integrated Development Environment (IDE)
A good IDE can enhance your programming experience. Popular choices include Code::Blocks, Visual Studio, and CLion. Each IDE offers unique features to simplify the coding process, such as syntax highlighting and debugging tools.
For example, in Visual Studio:
- Open Visual Studio Installer.
- Choose the Desktop development with C++ workload.
- Create a new project and write your first C++ program.
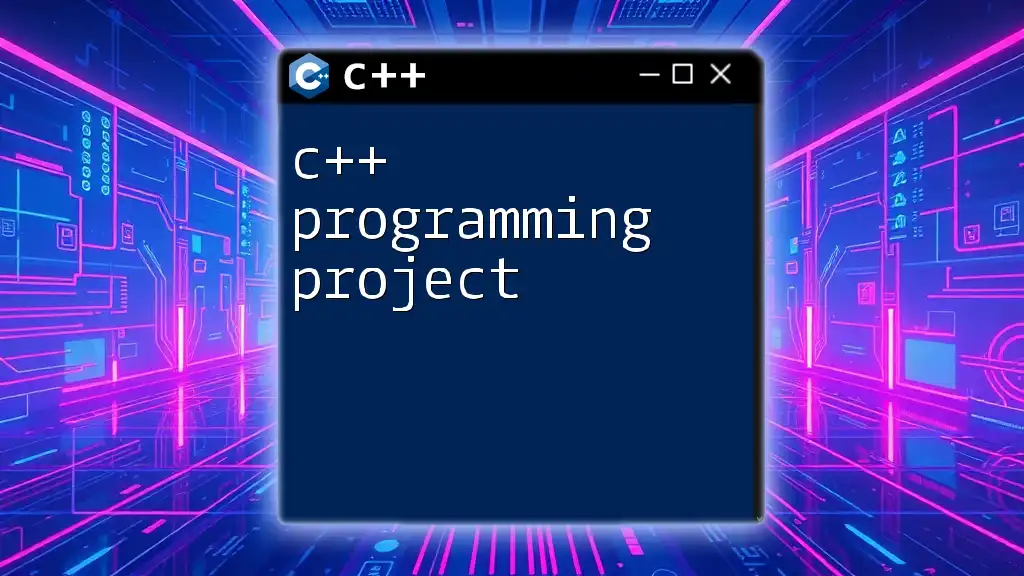
Core Programming Principles
Understanding Basic Programming Constructs
Variables and Data Types
Variables serve as storage locations for data. C++ offers several built-in data types, including:
- `int`: For integers
- `float`: For decimal numbers
- `char`: For single characters
For example:
int age = 21;
float salary = 45000.50;
char initial = 'A';
Control Flow Statements
Control flow allows the program to make decisions and execute different code paths.
- If-Else Statements: These enable conditional execution based on boolean expressions.
if (age >= 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
- Switch Statements: These provide a cleaner syntax for multiple conditions.
switch (grade) {
case 'A':
std::cout << "Excellent!" << std::endl;
break;
case 'B':
std::cout << "Good Job!" << std::endl;
break;
default:
std::cout << "Keep Trying!" << std::endl;
}
Functions: Building Blocks of C++
What are Functions?
Functions encapsulate specific tasks and can be reused throughout your code, enhancing modularity and readability.
Function Structure:
return_type function_name(parameters) {
// function body
}
Example of Function Creation
Here’s how to create a simple addition function:
int add(int a, int b) {
return a + b;
}
You can then call this function in your `main` function:
int result = add(5, 3);
std::cout << "Sum is: " << result << std::endl;
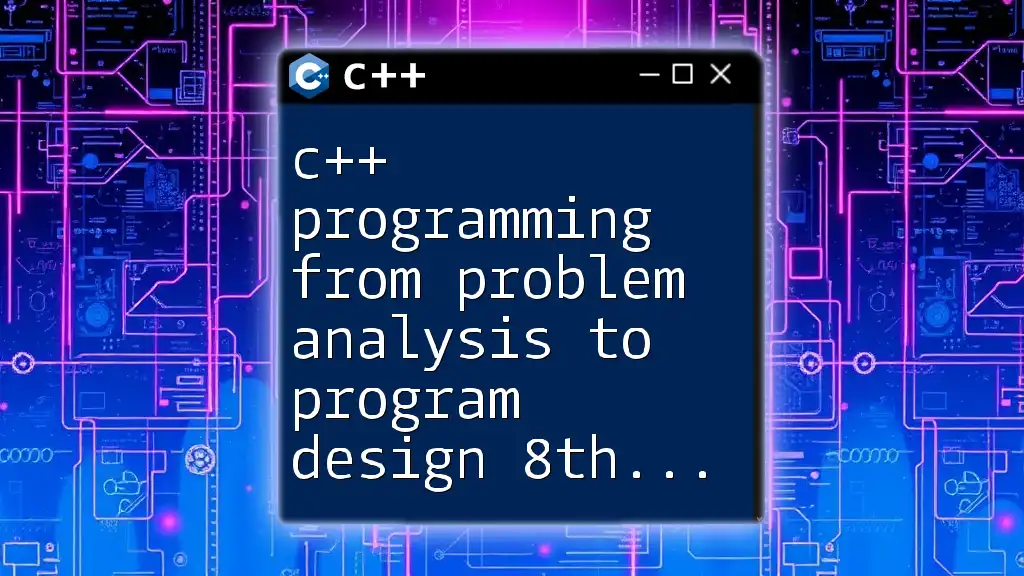
Object-Oriented Programming in C++
Introduction to OOP Concepts
Object-Oriented Programming revolves around the use of objects to encapsulate data and behavior, enhancing code organization through principles like Encapsulation, Inheritance, and Polymorphism.
Creating Classes and Objects
Classes act as blueprints for objects. You can create a class in C++ with:
class Dog {
public:
std::string name;
void bark() {
std::cout << "Woof!" << std::endl;
}
};
To create an object:
Dog myDog;
myDog.name = "Buddy";
myDog.bark(); // Outputs: Woof!
Constructors and Destructors
Constructors initialize an object’s state, while destructors perform cleanup. Here’s an example:
class Cat {
public:
Cat() { std::cout << "Cat created!" << std::endl; } // Constructor
~Cat() { std::cout << "Cat destroyed!" << std::endl; } // Destructor
};
Creating an instance of `Cat` automatically calls the constructor:
Cat myCat; // Outputs: Cat created!
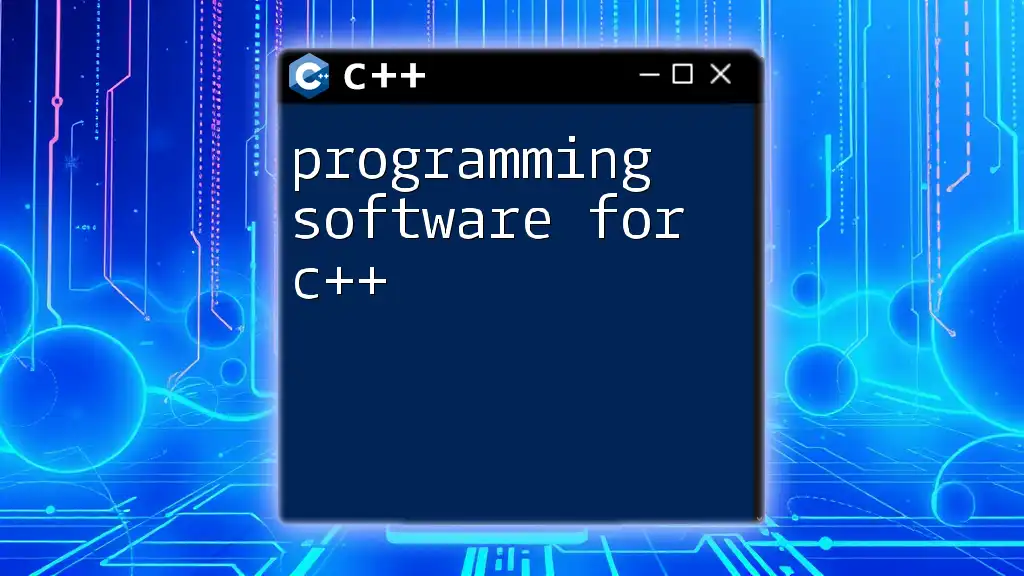
Error Handling Techniques
Understanding Exceptions
Error handling is a crucial element of robust programming. C++ employs a mechanism called exceptions, which can manage runtime issues elegantly.
Use try, catch, and throw statements to handle exceptions:
try {
throw std::runtime_error("An error occurred!");
} catch (const std::runtime_error& e) {
std::cout << e.what() << std::endl;
}
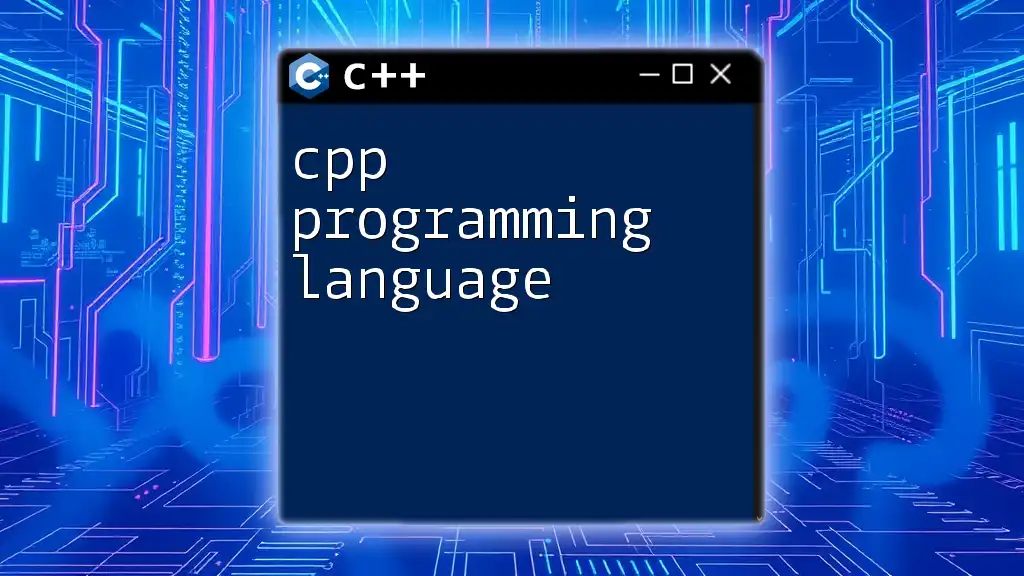
Advanced C++ Concepts
Templates: Writing Generic Code
Templates allow for the creation of functions and classes that work with any data type. Here is the syntax for a template function:
template<typename T>
T add(T a, T b) {
return a + b;
}
You can call the function with different types:
std::cout << add<int>(2, 3) << std::endl; // Outputs: 5
std::cout << add<float>(2.5, 3.1) << std::endl; // Outputs: 5.6
The Standard Template Library (STL)
Introduction to STL
The Standard Template Library provides a collection of templates for common data structures and algorithms, significantly boosting productivity.
Utilizing Common STL Data Structures
- Vectors for dynamic arrays:
#include <vector>
std::vector<int> numbers = {1, 2, 3};
numbers.push_back(4); // Adds 4 to the vector
- Maps for key-value pairs:
#include <map>
std::map<std::string, int> grades;
grades["Alice"] = 90;
grades["Bob"] = 85;
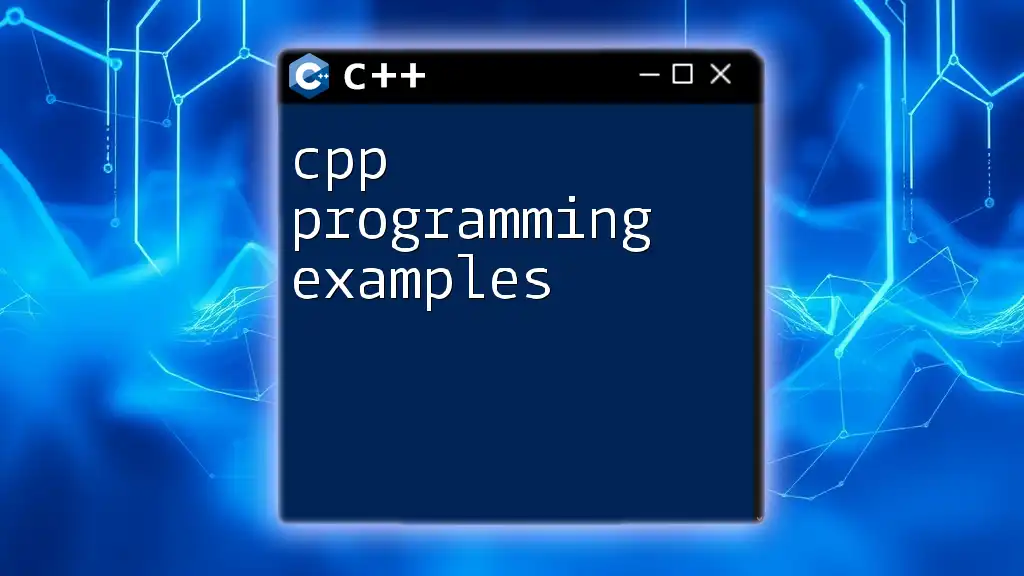
Best Practices in Programming
Writing Clean and Maintainable Code
Writing code that is not only functional but also easy to read and maintain is essential. Following established coding conventions and maintaining consistent naming patterns help ensure your code is accessible.
Comments and Documentation
Well-placed comments clarify the intention behind complex logic. Use documentation tools like Doxygen to automatically generate documentation from annotated code.
Debugging and Testing
Effective debugging techniques, such as step-through debugging and using asserts, play a vital role in finding and fixing bugs. Additionally, introductory unit testing in C++ can be done using frameworks like Google Test (gtest) to ensure your code behaves as expected.
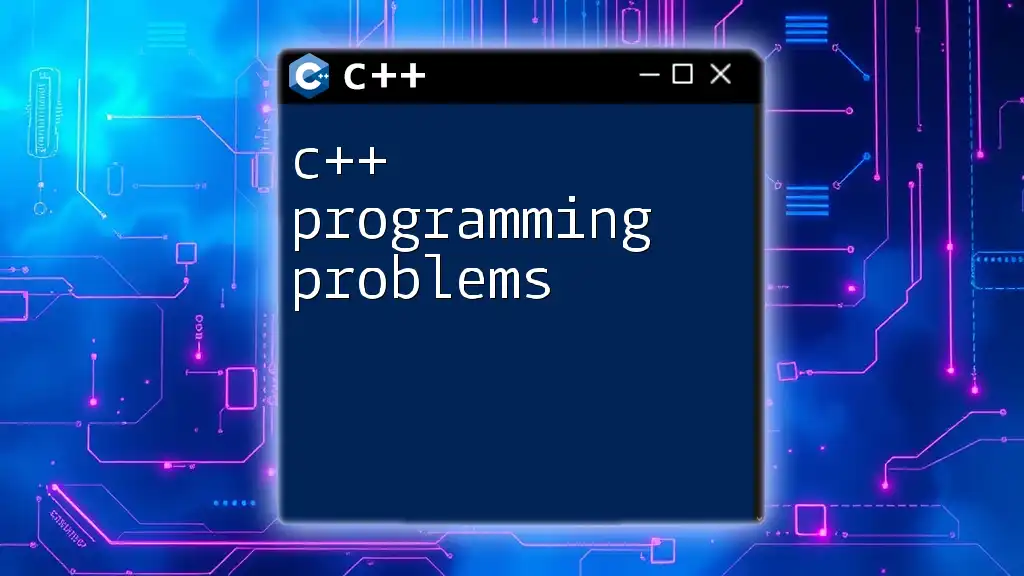
Conclusion
Recap of Key Learnings
In this article, we explored the programming principles and practice using C++ 3rd edition. From basic concepts up to advanced topics, you now have a foundational understanding of C++ that prepares you for further exploration.
Next Steps
Consider diving deep into more advanced C++ topics or collaborating with online communities for hands-on experience and support.
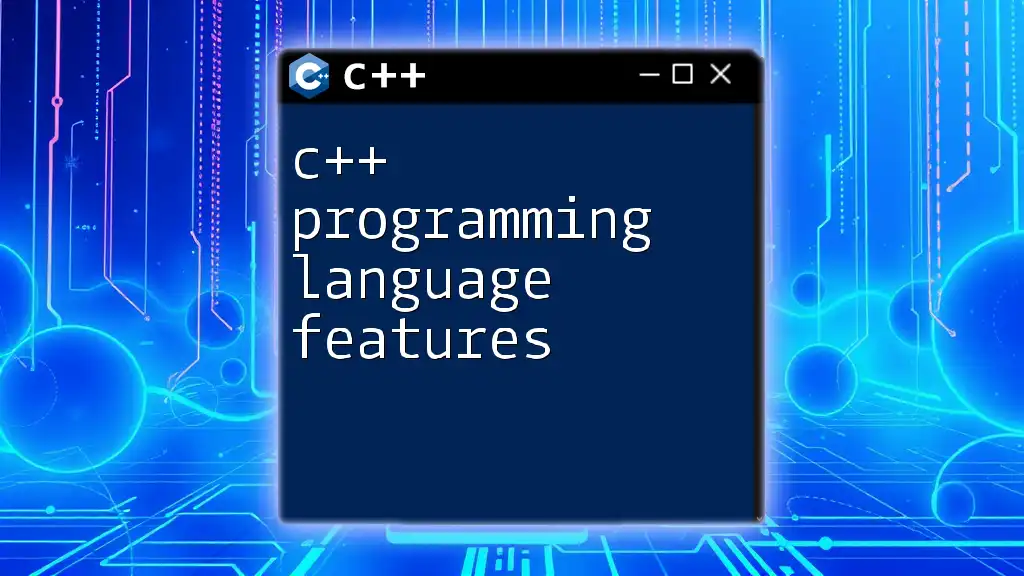
Additional Resources
Online Courses and Tutorials
Look for reputable online courses that delve deeper into both the theoretical and practical aspects of C++. Platforms like Coursera and Udacity offer excellent resources.
Books for Further Reading
Explore additional literature that further expands on specific C++ topics or advanced programming paradigms.
Community and Support Groups
Engage with groups on platforms like Stack Overflow or Reddit where programmers share their journey and support each other in learning C++.