In C++, a `long int` is a data type that is used to store long integer values, typically allowing for a larger range of numbers than a standard `int`.
Here's a simple code snippet demonstrating its use:
#include <iostream>
int main() {
long int largeNumber = 1234567890;
std::cout << "The long integer is: " << largeNumber << std::endl;
return 0;
}
Understanding Long Int in C++
What is Long Int?
A long int is a data type in C++ that can store larger integer values compared to the standard `int` type. Typically, long int is at least 32 bits in size but can be 64 bits on certain platforms. This makes it particularly useful for applications that need to handle large numbers, such as calculations involving population counts, large financial transactions, or computations requiring long-range data.
The primary advantage of using a long int is its larger range of values:
- int: Typically ranges from -2,147,483,648 to 2,147,483,647.
- long int: Can range from -2,147,483,648 to 2,147,483,647 (on a 32-bit system) or up to 9,223,372,036,854,775,807 on a 64-bit system.
How Long Int Fits in C++ Data Types
C++ offers several integer data types, and long int serves as a middle ground between regular integers and larger types like long long int. The choice of which data type to use depends on the nature of the problem you're trying to solve.
- int: Useful for smaller numbers.
- long int: Suitable for larger values that exceed the limits of an ordinary int.
- long long int: Used when exceptionally large integers are required, often being 64 bits in size regardless of platform.
Moreover, using the appropriate data type can also contribute to better memory utilization, potentially enhancing performance and reducing the risk of overflow errors.
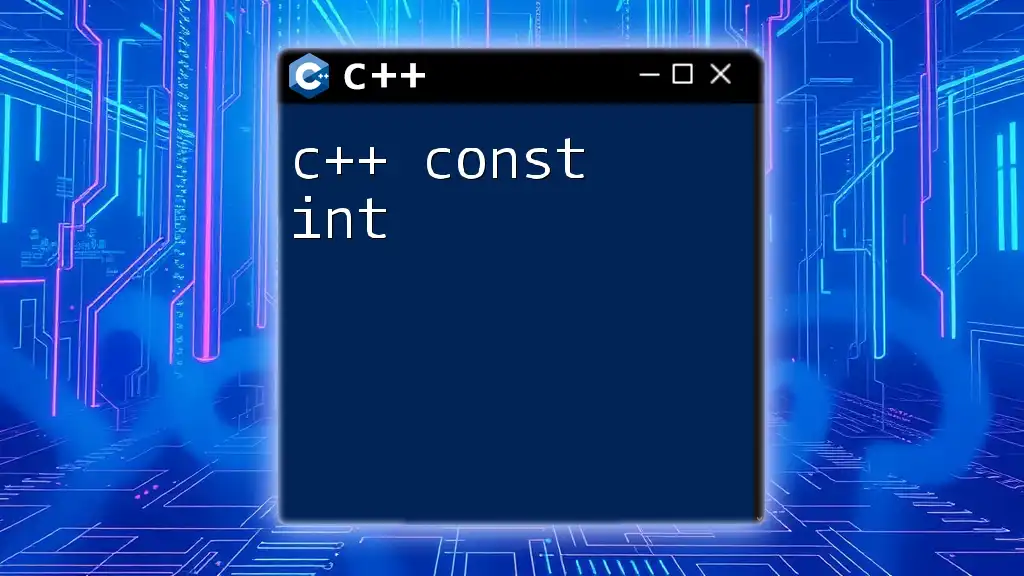
Declaring Long Int in C++
Syntax for Declaring Long Int
To declare a long int, the syntax is straightforward:
long int variableName;
You can initialize a long int upon declaration as well:
long int distance = 100000; // Example of initialization
Using Long Int with Other Data Types
Long Int with Const and Static
You may often need to define constant long integers, which can be done using the `const` keyword. This means that the value cannot be changed post-initialization:
const long int maxDistance = 1000000; // This value can't be changed
Static long ints are useful in scenarios where you want to retain the variable's state even when the function exits:
void countCalls() {
static long int callCount = 0;
callCount++;
cout << "Function has been called " << callCount << " times." << endl;
}
Combining Long Int with Other Types
You can perform arithmetic operations with long ints, and mixing them with other types is common:
long int result = myNumber + distance; // Simple addition
In this example, the compiler automatically promotes `myNumber` to ensure the addition is performed correctly without overflow.
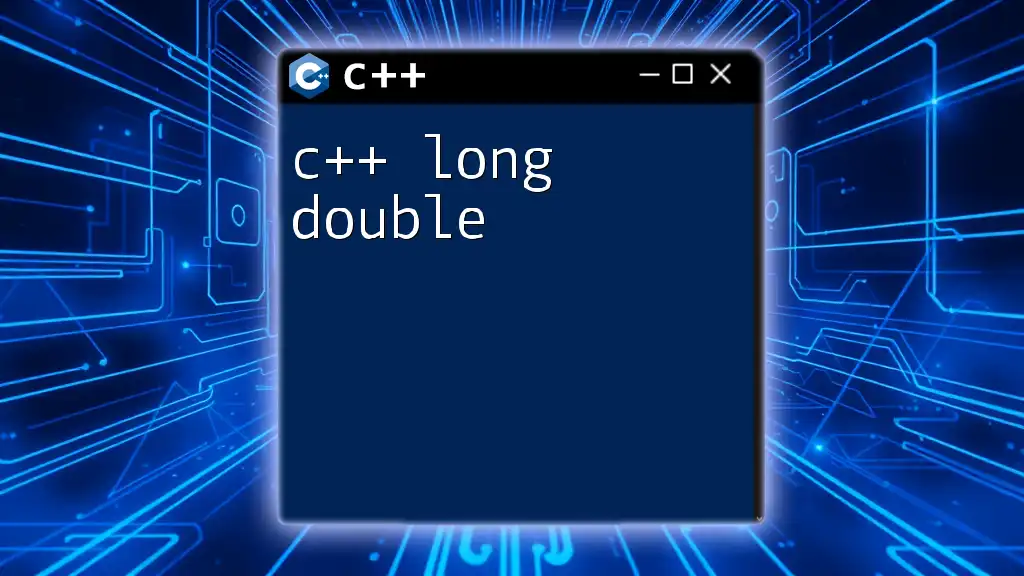
The Long Long Int in C++
What is Long Long Int?
A long long int is designed to hold even larger integers than long int, usually 64 bits across all platforms. This makes it extremely useful for situations where ordinary integers or even long ints are insufficient.
When to Use Long Long Int Over Long Int
You might prefer using long long int when you anticipate the need to store values exceeding the range of long int. For example, when calculating national demographics or handling data from extensive databases, utilizing long long int is beneficial:
long long int hugeNumber = 9223372036854775807; // Maximum for long long int
This ensures that your programs can manage larger datasets without the risk of overflow, especially in computational tasks.
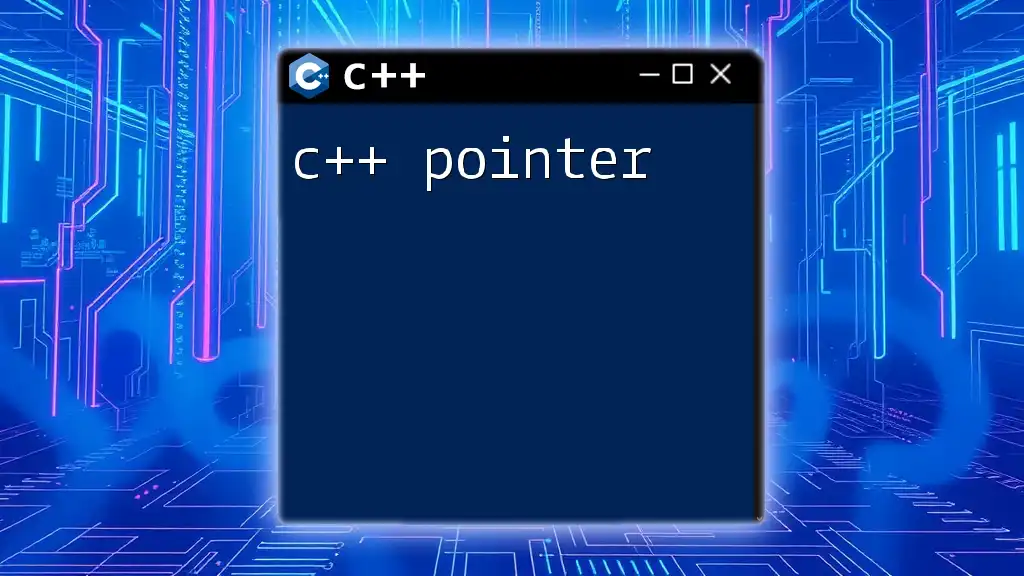
Practical Examples and Use Cases
Example 1: Calculating Large Numbers
Here’s a simple example of using long int for a real-world scenario:
#include <iostream>
using namespace std;
int main() {
long int population = 7837000000; // Approx. world population
cout << "The world population is: " << population << endl;
return 0;
}
In this example, the long int successfully stores a value that far exceeds what a standard integer could handle.
Example 2: Handling Overflow with Long Long Int
Understanding the potential for overflow is crucial. Here’s how to demonstrate that with long int and long long int:
#include <iostream>
using namespace std;
int main() {
long int a = 2147483647; // Maximum value of int (32-bit)
long int result = a + 1; // Potential overflow error
cout << "Result using long int: " << result << endl;
long long int b = 2147483647; // Using long long int for safety
long long int safeResult = b + 1; // No overflow possible here
cout << "Result using long long int: " << safeResult << endl;
return 0;
}
This example highlights the potential for overflow when using long int and showcases the safety provided by long long int.
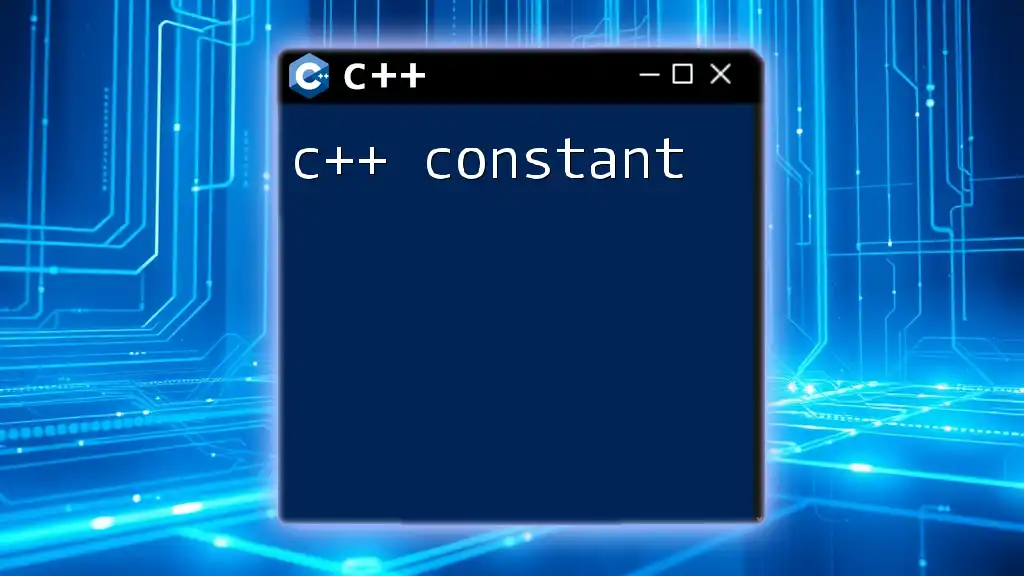
Best Practices for Using Long Int in C++
When to Prefer Long Int vs Long Long Int
While both long int and long long int are useful, knowing when to use each is vital. As a rule of thumb:
- If you expect values to remain within the range of a long integer and your calculations are modest, long int suffices.
- Opt for long long int if you're working with data that could exceed the limits of long int, thus ensuring greater safety against overflow.
Common Pitfalls
Developers often overlook the limits of data types, leading to problems such as overflow errors. Always ensure that:
- You understand the maximum and minimum values supported by your chosen data type.
- You consider using long long int in mathematically intensive applications or data-heavy contexts.
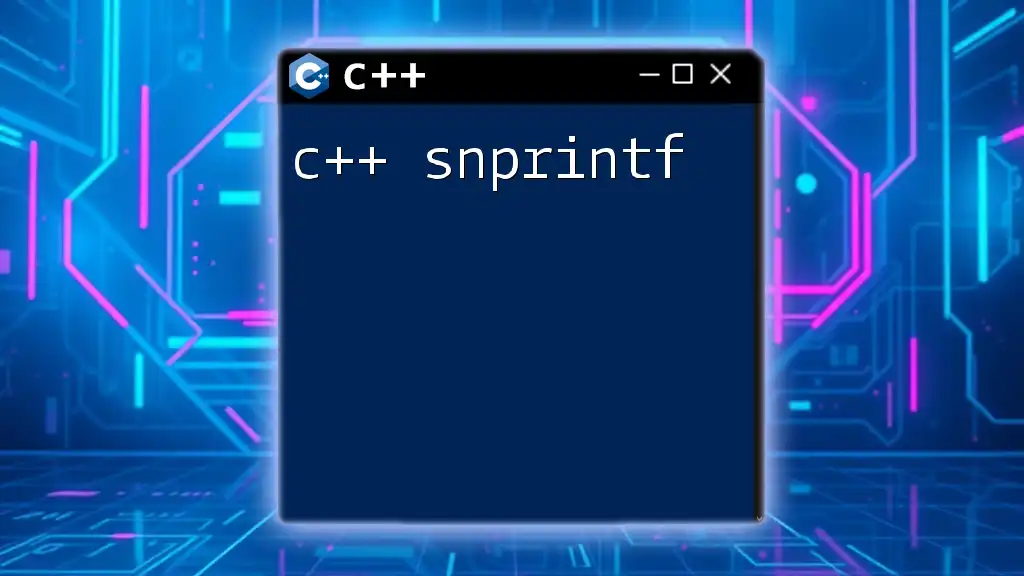
Conclusion
With the C++ programming language, understanding data types is essential for writing efficient and effective code. Using long int and long long int appropriately can help you handle expansive datasets while mitigating the risk of overflow. By practicing these principles and incorporating them into your projects, you can elevate your coding capabilities and improve performance within your applications.
As you continue your C++ programming journey, keep exploring these data types, and don’t hesitate to experiment with them in various coding scenarios!