C++ UTF-16 refers to the encoding of Unicode characters using two bytes per character, commonly utilized in applications that require support for a wide range of international text.
Here’s a simple code snippet for handling UTF-16 strings in C++:
#include <iostream>
#include <string>
#include <codecvt>
#include <locale>
int main() {
// UTF-16 string
std::wstring utf16Str = L"Hello, World! 🌍";
// Output UTF-16 string
std::wcout << utf16Str << std::endl;
return 0;
}
What is UTF-16?
UTF-16 is an encoding system that represents Unicode characters using one or two 16-bit code units. It's a popular choice in many software applications due to its ability to efficiently encode all characters in the Unicode standard, which includes a wide variety of symbols, scripts, and glyphs. UTF-16 is particularly useful for handling texts in languages that are widely used across the globe, such as Chinese, Japanese, and Korean.
Why Use UTF-16 in C++?
Using UTF-16 in C++ opens up numerous benefits, especially when it comes to internationalization (i18n). The encoding allows for seamless representation and manipulation of diverse character sets, making it ideal for applications targeting a global audience. UTF-16 is also widely adopted in popular platforms and libraries, which means developers can leverage existing resources and frameworks without worry.
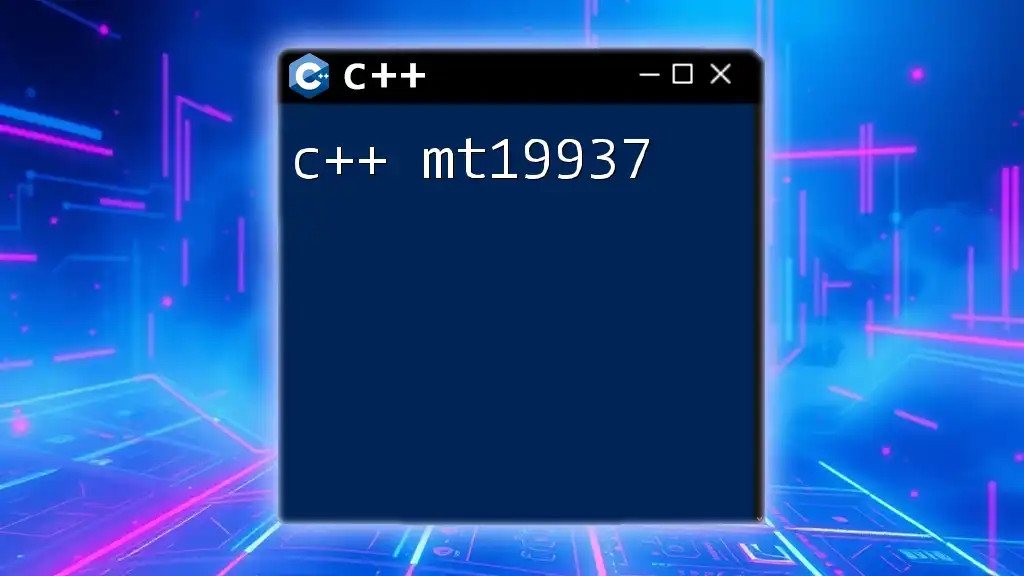
Understanding Unicode
What is Unicode?
Unicode is a comprehensive system for character encoding that assigns a unique code point to every character across different languages and scripts. By standardizing character representation, Unicode enables data interchange between systems.
UTF-16 fits into the Unicode scheme as one of its encoding forms, providing efficient representation of characters while maintaining compatibility with older systems using ASCII.
Code Points and Characters
In the context of Unicode, code points are numerical values assigned to each character. For example, the code point for the character 'A' is U+0041. It's important to note that not all code points correspond to a single character, as many characters might require multiple code units for their representation in UTF-16. Understanding the difference between characters and code points is crucial when working with UTF-16 in C++.
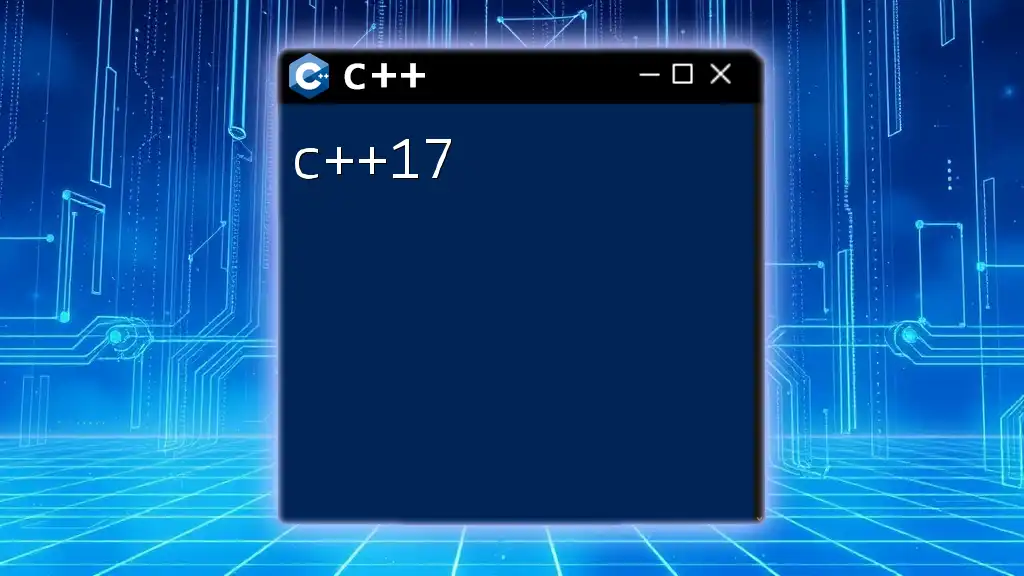
C++ and UTF-16
Native Support for UTF-16 in C++
C++ natively supports UTF-16 through the `char16_t` data type. This type is specifically designed to hold UTF-16 encoded characters, allowing developers to work directly with Unicode text. UTF-16 is represented in C++ as a sequence of 16-bit units.
Including UTF-16 Libraries
To work effectively with UTF-16 in C++, developers can utilize standard libraries tailored for Unicode handling. Notably, the `<codecvt>` library in C++11 and later versions enables encoding and decoding of UTF-16. Additionally, third-party libraries like ICU (International Components for Unicode) offer advanced features for managing UTF-16 characters, including support for various transformations and text manipulation.
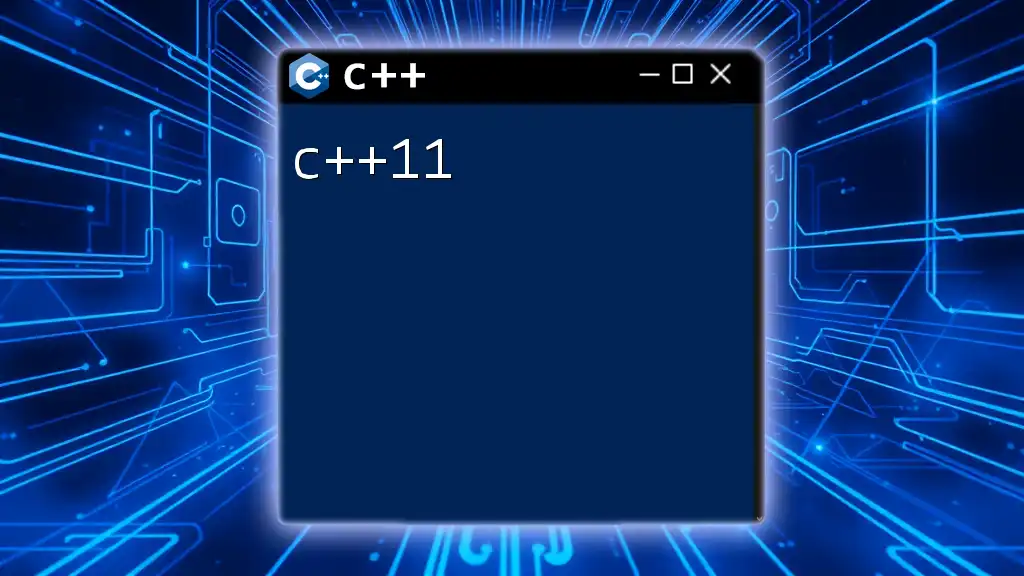
Handling UTF-16 Strings in C++
Creating UTF-16 Strings
In C++, defining a UTF-16 string is straightforward. You can use the `u` prefix before string literals to indicate that they are in UTF-16 format:
std::u16string utf16_str = u"Hello, 世界!";
This code snippet initializes a UTF-16 string containing both ASCII and non-ASCII characters.
Length and Size of UTF-16 Strings
When working with UTF-16 strings, it’s vital to understand how length is determined. The `length()` method returns the number of code units in the string, which may differ from the number of characters due to the presence of surrogate pairs, particularly for characters outside the Basic Multilingual Plane (BMP).
std::cout << "Length: " << utf16_str.length() << std::endl; // Outputs the number of code units
Converting Between UTF-8 and UTF-16
Conversions between UTF-8 and UTF-16 are often necessary when dealing with external data sources. The following function illustrates how to convert a UTF-8 encoded string to UTF-16:
std::u16string convert_utf8_to_utf16(const std::string& utf8)
{
using convert_type = std::codecvt_utf8<char16_t>;
std::wstring_convert<convert_type, char16_t> converter;
return converter.from_bytes(utf8);
}
This function utilizes the `std::wstring_convert` along with the appropriate codecvt facet to handle the conversion.
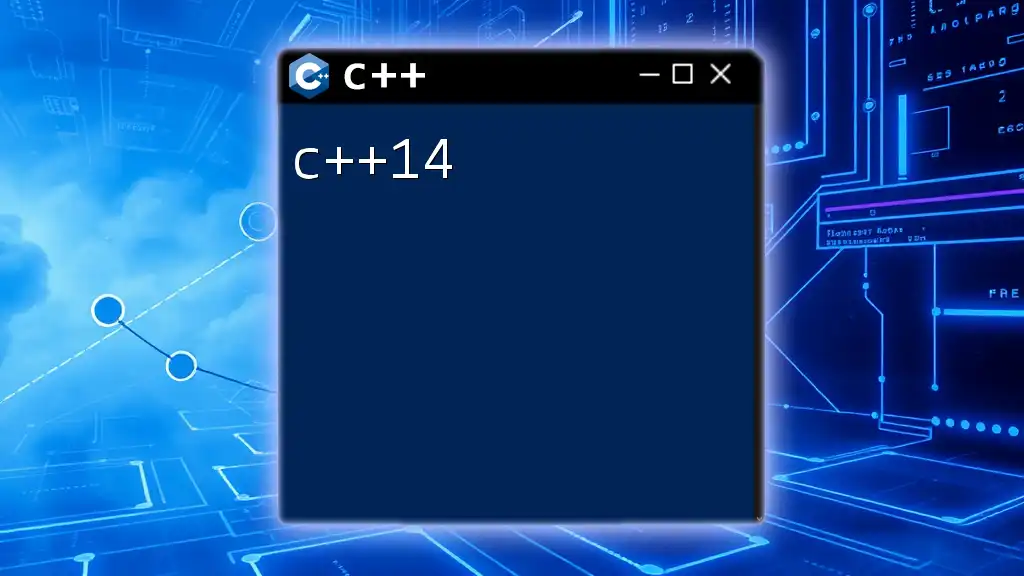
Manipulating UTF-16 Strings
Accessing Characters in UTF-16 Strings
Accessing individual characters in a UTF-16 string requires care, especially when dealing with surrogate pairs. While you can index into the string using standard array syntax, you must ensure that you don’t mistakenly treat surrogate pairs as individual characters.
Modifying UTF-16 Strings
Modifying a UTF-16 string typically involves appending, erasing, or replacing characters. For instance, the following line demonstrates how to append additional UTF-16 text:
utf16_str += u" Add more text!";
Searching and Finding in UTF-16 Strings
Searching within a UTF-16 string can be accomplished using standard string functions. For example, you can locate the position of a specific UTF-16 substring:
auto pos = utf16_str.find(u"世界"); // Returns the position of the substring if found
This capability is essential for text processing, especially in applications requiring user interaction with multilingual input.
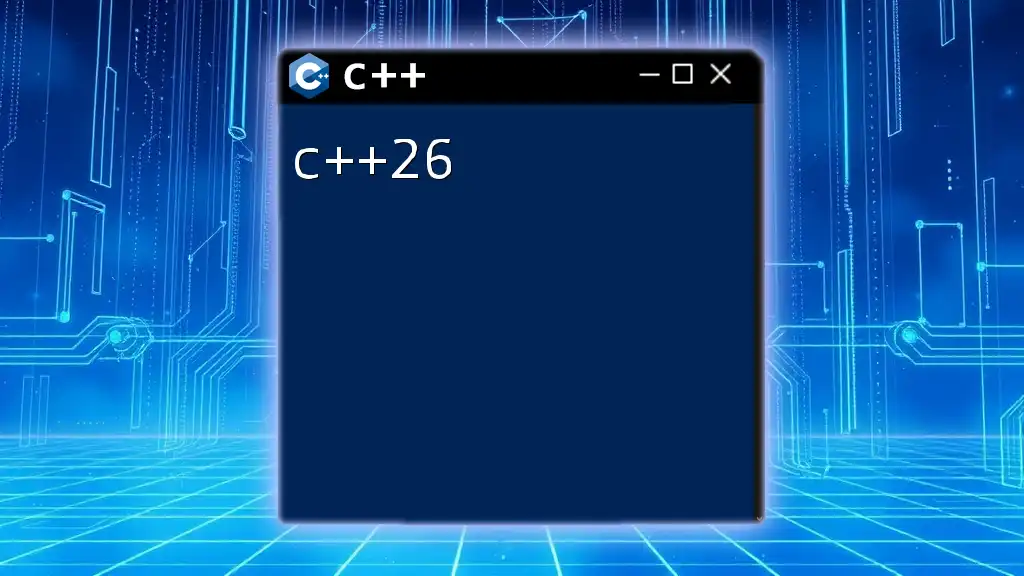
Practical Applications of UTF-16
Use Cases in C++
UTF-16 finds its applications in systems that require robust international character support. It is particularly beneficial in user interfaces, where displaying a variety of languages is crucial. Additionally, file handling using UTF-16 can ensure correct encoding while writing or reading text from files.
For instance, to write a UTF-16 string to a file, you could use the following code snippet:
std::wofstream wof("example.txt", std::ios::out | std::ios::binary);
wof.imbue(std::locale(wof.getloc(), new std::codecvt_utf16<wchar_t>));
wof << utf16_str;
Internationalization Support
Drawing from its versatility, UTF-16 serves as a powerful tool for enabling internationalization in applications. By supporting diverse languages and character sets, developers can create software that caters to users across different regions, fostering a global reach.
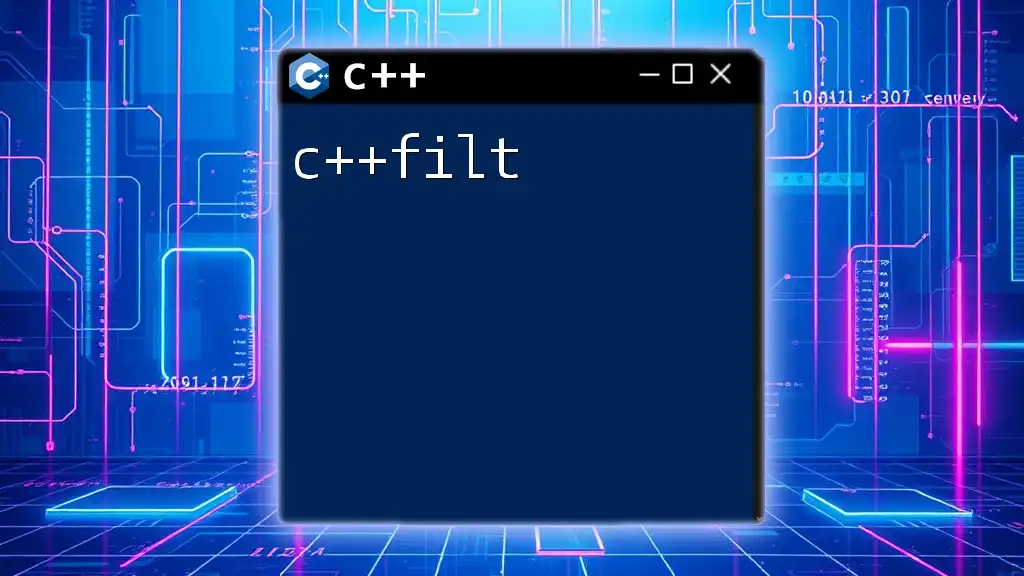
Performance Considerations
Memory Usage
One of the critical aspects of using UTF-16 is its memory footprint compared to other encodings like UTF-8 or UTF-32. While UTF-8 is generally more compact for texts primarily composed of ASCII characters, UTF-16 may become more efficient when encoding languages with large character sets.
Speed of Operations
The choice of encoding can affect performance depending on the operations performed. For instance, operations requiring frequent access to characters outside the ASCII range might be faster with UTF-16 due to the fixed size of code units. In contrast, the overhead of converting from one encoding to another can introduce latency.
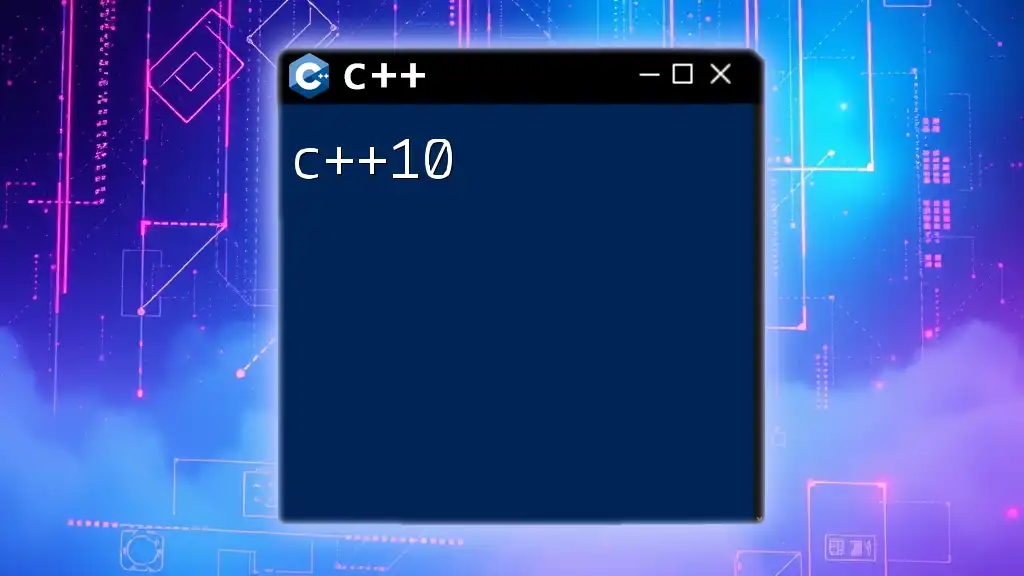
Troubleshooting Common Issues
Encoding Errors
When working with UTF-16, handling invalid sequences is crucial. These errors may arise when incorrectly interpreting byte sequences. Implementing a validation function can help maintain data integrity:
bool isValidUTF16(const std::u16string& str) {
// Logic to check the validity of UTF-16 sequences
}
Performance Bottlenecks
As with any data format, improper use of UTF-16 can lead to performance bottlenecks. Be sure to analyze your code for areas where excessive conversions or inefficient string operations occur. Performance profiling tools can assist in pinpointing where optimizations can be made.
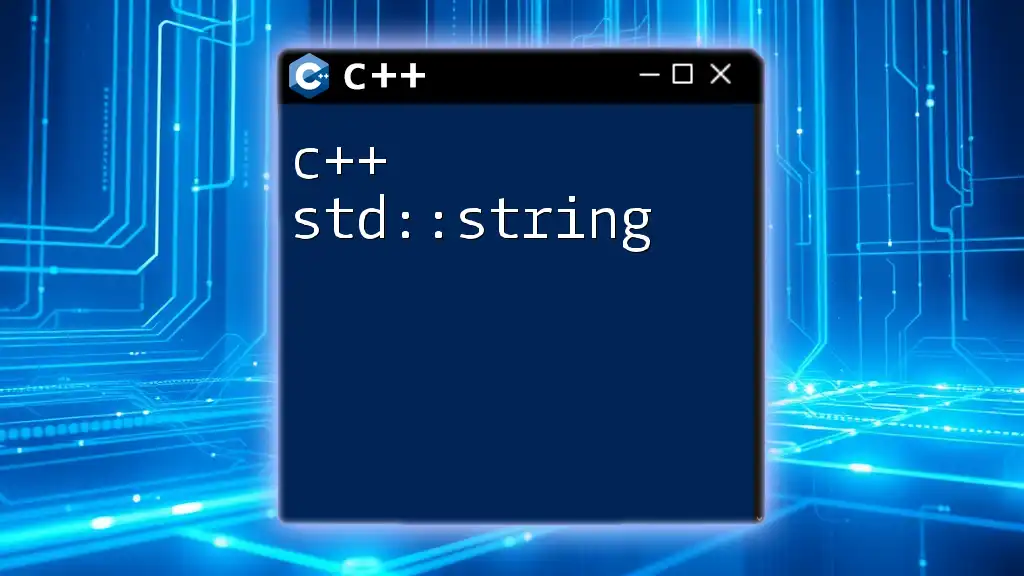
Conclusion
In summary, UTF-16 is a powerful tool for handling Unicode data within C++. Understanding its capabilities, proper use, and integration into applications is essential for creating software that supports a broad range of languages and scripts. By adopting UTF-16, developers can ensure their applications are well-equipped for globalization.
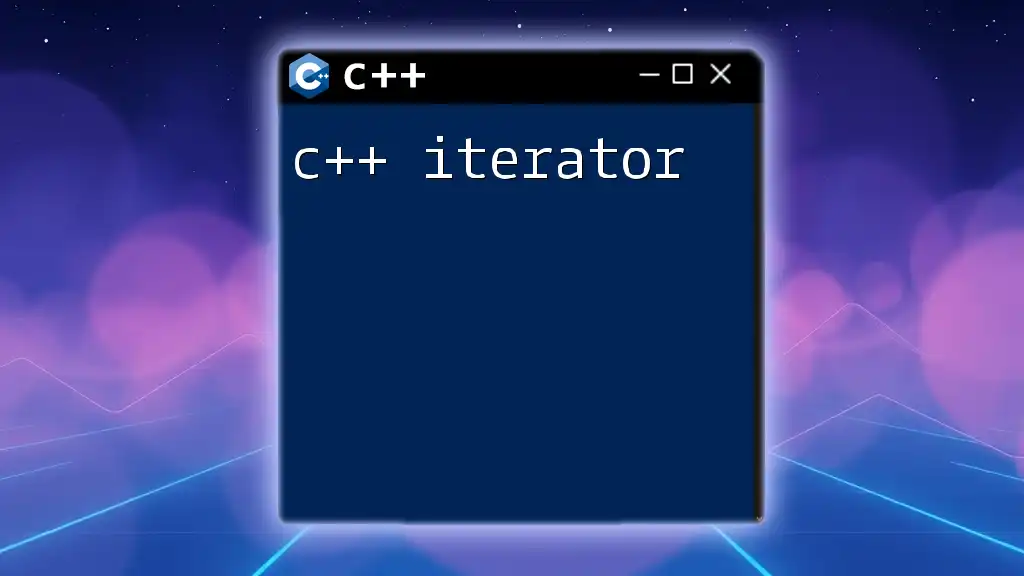
Additional Resources
For further learning, consider exploring recommended books on Unicode, official C++ documentation for string handling, and relevant libraries such as ICU. Engaging with these tools will enhance your understanding and proficiency in using UTF-16 with C++.
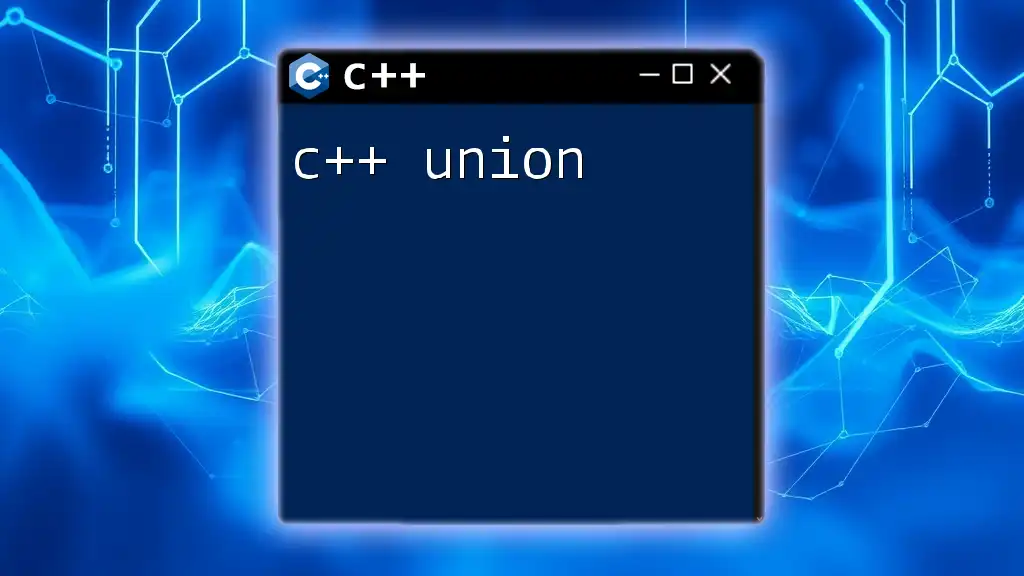
Call to Action
Practice implementing the examples provided, and consider subscribing for more insights into using C++ commands and features effectively. Embrace the diversity of UTF-16 to build robust applications that resonate with users around the world.