C++ gRPC is a high-performance, open-source framework that enables efficient remote procedure calls across different platforms and languages, facilitating communication between clients and servers.
Here's a simple example of a gRPC server implementation in C++:
#include <grpcpp/grpcpp.h>
#include "your_service.grpc.pb.h"
class YourServiceImpl final : public YourService::Service {
// Implement your service methods here
};
int main(int argc, char** argv) {
std::string server_address("0.0.0.0:50051");
YourServiceImpl service;
grpc::ServerBuilder builder;
builder.AddListeningPort(server_address, grpc::InsecureServerCredentials());
builder.RegisterService(&service);
std::unique_ptr<grpc::Server> server(builder.BuildAndStart());
std::cout << "Server listening on " << server_address << std::endl;
server->Wait();
return 0;
}
What is gRPC?
gRPC (gRPC Remote Procedure Calls) is a modern remote procedure call (RPC) framework that enables efficient communication between client and server applications. It uses HTTP/2 for transport and Protocol Buffers as its interface description language. In the context of C++ gRPC, it facilitates seamless interaction between systems developed in C++ and other programming languages.
Why Use gRPC with C++?
Using gRPC with C++ offers multiple advantages:
- Performance: gRPC is designed for high performance with features like multiplexing and streaming.
- Cross-Platform Communication: It supports multiple programming languages, enabling C++ applications to easily communicate with services written in languages like Python, Java, or Go.
- Strong Asynchronous Support: gRPC provides built-in asynchronous features, giving developers the ability to write non-blocking code, which is essential for developing responsive and scalable applications.
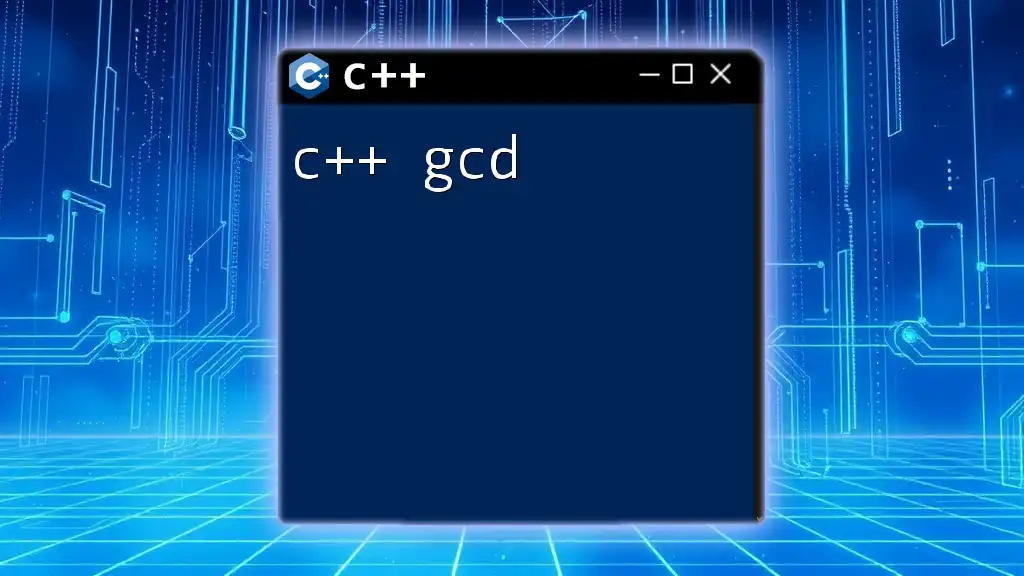
Setting Up Your C++ gRPC Environment
Prerequisites
To start building C++ gRPC applications, ensure you have the following installed:
- C++ Compiler: Make sure you have a modern C++ compiler installed (like g++ or Clang).
- CMake: This is essential for building C++ applications.
- Protocol Buffers: gRPC uses Protocol Buffers for serializing structured data.
Installing gRPC and Protocol Buffers
Here’s how to install gRPC and Protocol Buffers on Linux (similar steps apply for macOS and Windows):
-
Clone the gRPC repository:
git clone https://github.com/grpc/grpc.git cd grpc git submodule update --init
-
Build and install Protocol Buffers:
mkdir -p cmake/build cd cmake/build cmake ../.. make sudo make install
-
Build and install gRPC:
cd ../.. mkdir -p cmake/build cd cmake/build cmake ../.. make sudo make install
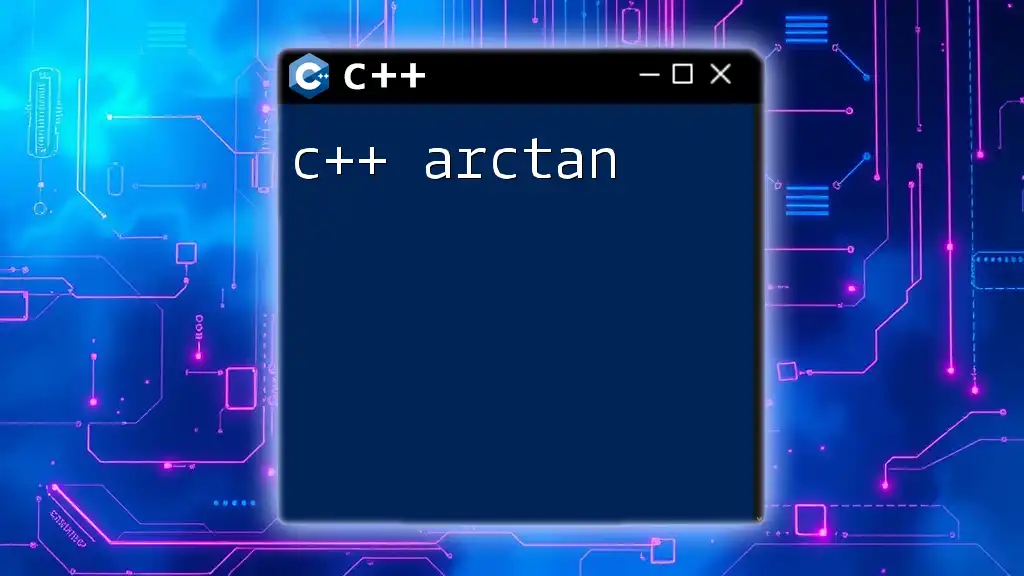
Understanding the gRPC Architecture
Core Concepts of gRPC
gRPC operates on a client-server architecture and revolves around a few core concepts:
- Protobuf: Protocol Buffers define the structure of the data being exchanged. This is done through a `.proto` file which specifies the service interface and message types.
- Service Definitions: Within the `.proto` file, you specify your service and the methods it exposes.
- RPC Calls: gRPC supports various types of RPC calls, which include unary, server streaming, client streaming, and bidirectional streaming.
Unary vs. Server Streaming vs. Client Streaming vs. Bidirectional Streaming
-
Unary RPC: This is the simplest type, where the client sends a single request and gets a single response.
// Example of Unary RPC in a .proto file service Greeter { rpc SayHello (HelloRequest) returns (HelloReply); }
-
Server Streaming RPC: The client sends a single request and gets a stream of responses.
-
Client Streaming RPC: The client sends a stream of requests and gets a single response.
-
Bidirectional Streaming RPC: Both client and server send messages in both directions.
Each RPC type caters to different application requirements, allowing flexible communication patterns.
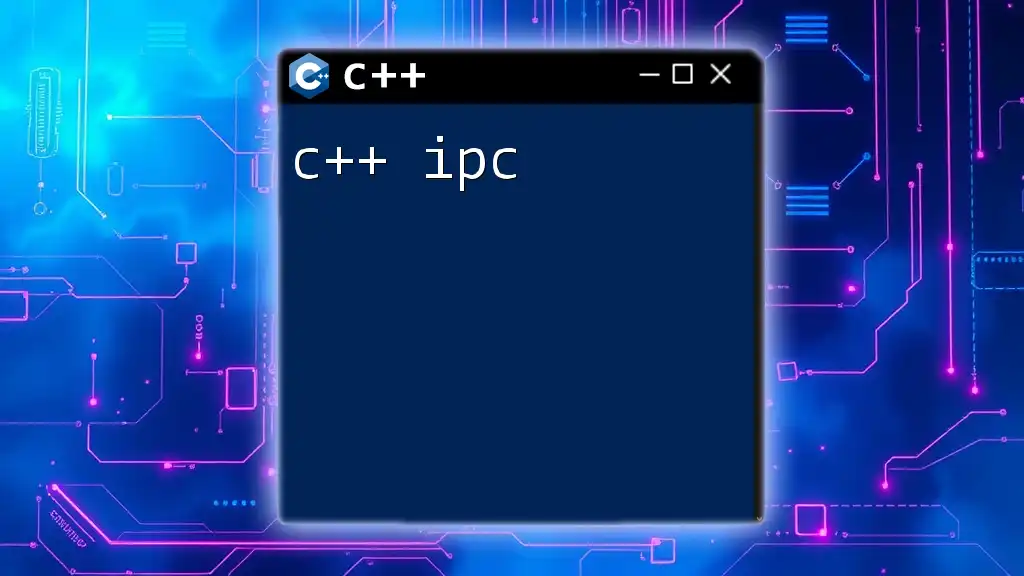
Creating Your First gRPC Service with C++
Defining Your Service
To create a gRPC service, you start by defining it in a `.proto` file. Here's an example:
syntax = "proto3";
package helloworld;
// The greeting service definition.
service Greeter {
// Sends a greeting
rpc SayHello (HelloRequest) returns (HelloReply);
}
// The request message containing the user's name.
message HelloRequest {
string name = 1;
}
// The response message containing the greetings.
message HelloReply {
string message = 1;
}
Generating C++ Code from Proto File
After defining your service, you need to generate C++ classes from your `.proto` file using the `protoc` compiler:
protoc -I=. --cpp_out=. --grpc_out=. --plugin=protoc-gen-grpc=$(which grpc_cpp_plugin) helloworld.proto
This command generates the necessary header and source files for both Protocol Buffers and gRPC.
Implementing the Service in C++
Now, you can implement the service defined in the `.proto` file in a C++ file. Here’s how you might implement the `SayHello` method:
#include "helloworld.grpc.pb.h"
class GreeterServiceImpl final : public helloworld::Greeter::Service {
grpc::Status SayHello(grpc::ServerContext* context, const helloworld::HelloRequest* request,
helloworld::HelloReply* reply) override {
std::string prefix("Hello ");
reply->set_message(prefix + request->name());
return grpc::Status::OK;
}
};
In this implementation, when a client sends a request to the `SayHello` method, it returns a greeting message.
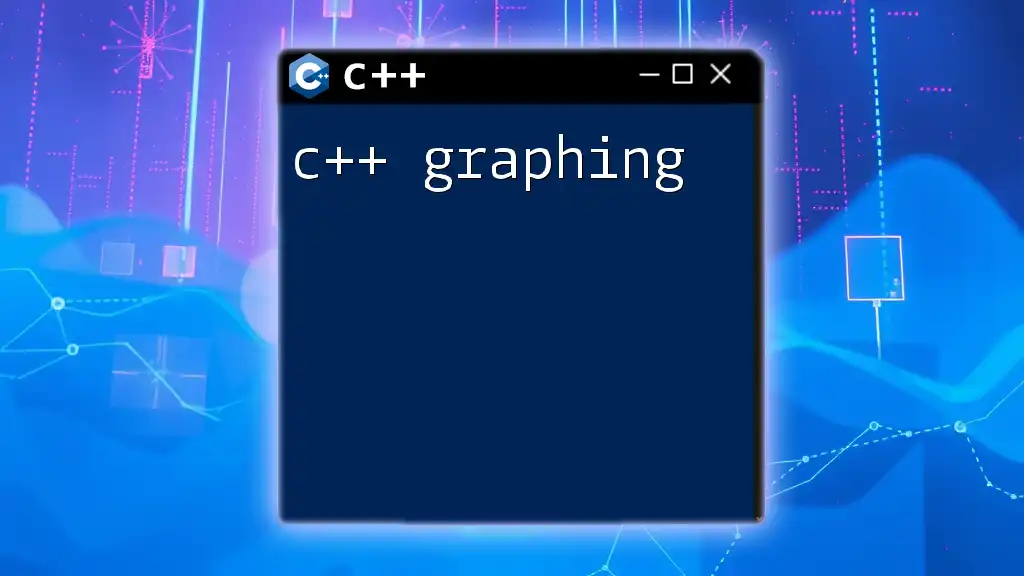
Building and Running Your gRPC Server
Building Your Application
To build your C++ gRPC application, you can use CMake. Here's a sample `CMakeLists.txt` file:
cmake_minimum_required(VERSION 3.5)
project(helloworld)
find_package(Protobuf REQUIRED)
find_package(gRPC REQUIRED)
include_directories(${Protobuf_INCLUDE_DIRS} ${gRPC_INCLUDE_DIRS})
add_executable(helloworld_server helloworld_server.cc helloworld.pb.cc helloworld.grpc.pb.cc)
target_link_libraries(helloworld_server ${Protobuf_LIBRARIES} ${gRPC_LIBRARIES})
Running the gRPC Server
After building, you can run the server:
./helloworld_server
The server starts listening for incoming requests.
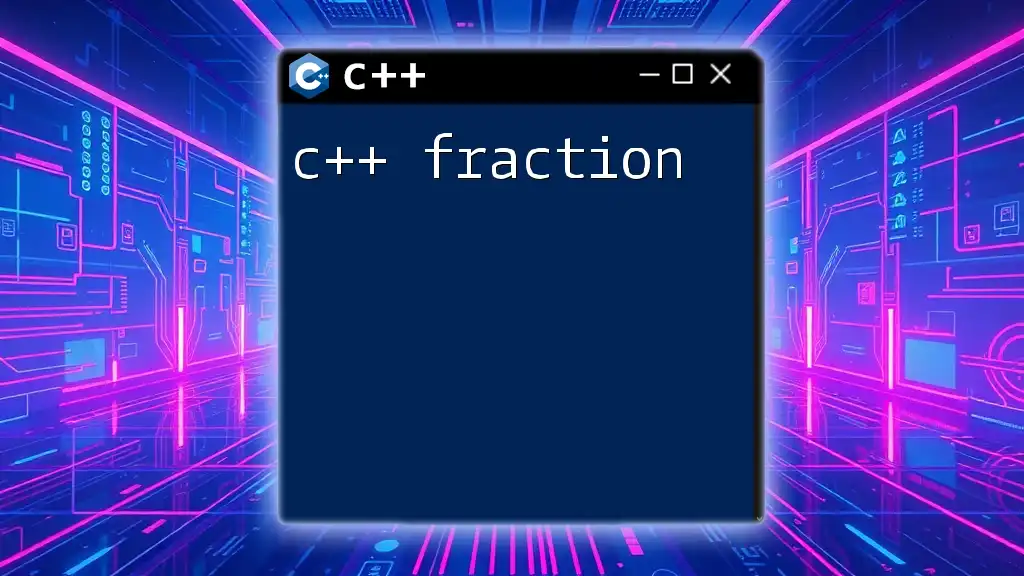
Building and Running the gRPC Client
Creating a gRPC Client in C++
You will also need to create a client that can communicate with your server. Below is a simple client implementation:
#include "helloworld.grpc.pb.h"
int main(int argc, char** argv) {
grpc::ChannelArguments ch_args;
auto channel = grpc::CreateCustomChannel("localhost:50051", grpc::InsecureChannelCredentials(), ch_args);
std::unique_ptr<helloworld::Greeter::Stub> stub = helloworld::Greeter::NewStub(channel);
helloworld::HelloRequest request;
request.set_name("World");
helloworld::HelloReply reply;
grpc::ClientContext context;
grpc::Status status = stub->SayHello(&context, request, &reply);
if (status.ok()) {
std::cout << "Greeting: " << reply.message() << std::endl;
} else {
std::cerr << "RPC failed" << std::endl;
}
return 0;
}
This client connects to the server and sends a request, then prints the response.
Handling Responses and Errors
When handling responses from the server, it is essential to check the `grpc::Status`. Proper error handling ensures that your application can gracefully manage issues like timeouts or connection problems.
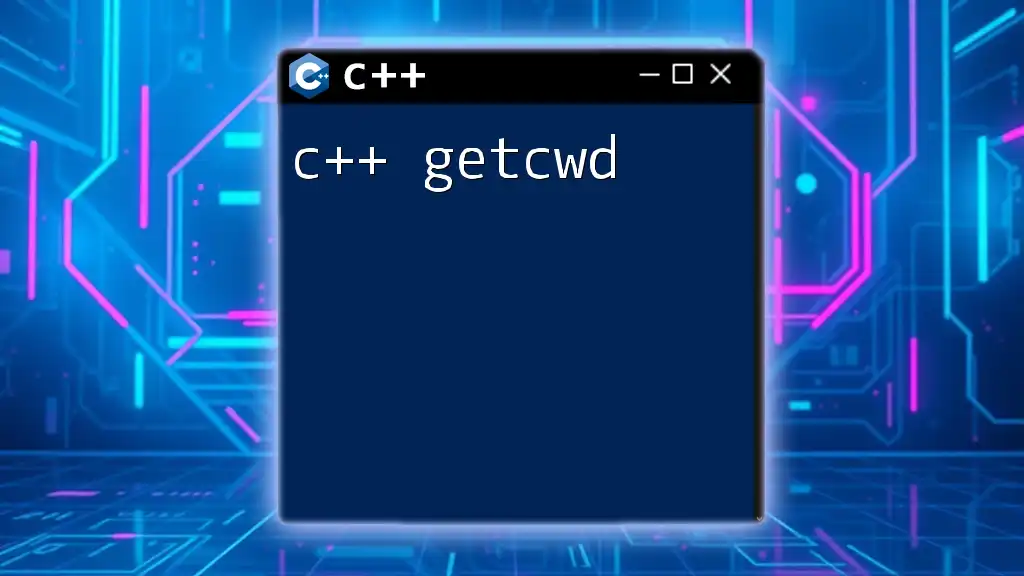
Advanced gRPC Features
Interceptors
Interceptors in gRPC allow you to add custom behavior to a call process. They can be used for logging, authentication, and other cross-cutting concerns.
class MyInterceptor : public grpc::experimental::Interceptor {
public:
void Intercept(grpc::experimental::InterceptorBatchMethods* methods) override {
// Implement interceptor logic here
}
};
Authentication and Security
Security is crucial in any application. gRPC allows you to secure your services using transport security via TLS. Here’s how you can enable it:
- Create and use SSL certificates for your server.
- Use `grpc::SslServerCredentials` to create secure server credentials.
Load Balancing and Service Discovery
gRPC supports various load balancing strategies. Implement your service discovery mechanism, which can route requests based on client conditions.
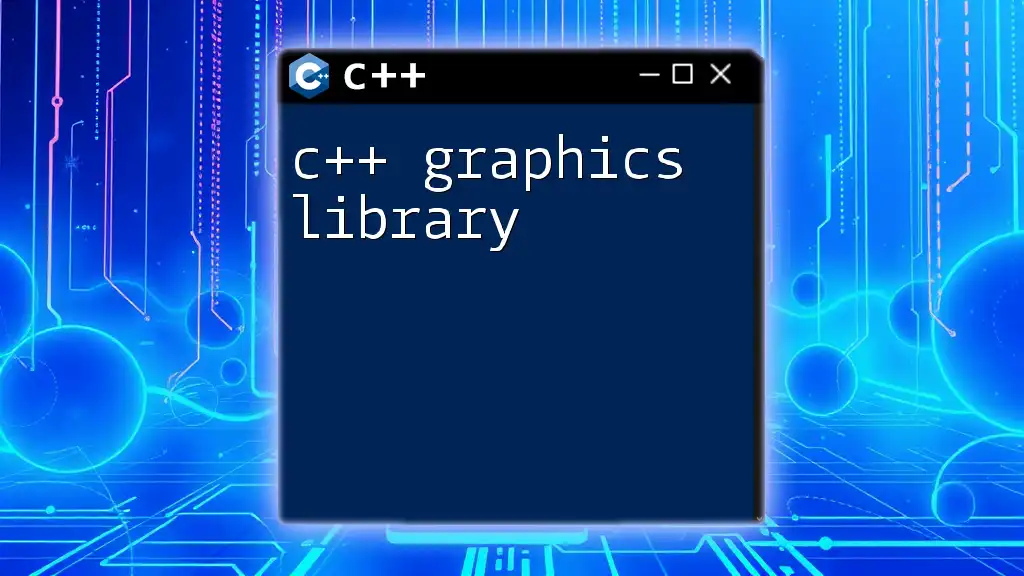
Best Practices for gRPC with C++
Performance Optimization
To achieve high performance in your gRPC applications, consider:
- Efficient serialization: Use Protocol Buffers instead of JSON for faster serialization.
- Connection pooling: Minimize connection overhead by reusing channels.
Maintaining Code Quality
Writing clean code is essential. Follow these practices:
- Modularization: Break down services into manageable components.
- Testing: Regularly test your services using gRPC testing tools and frameworks.
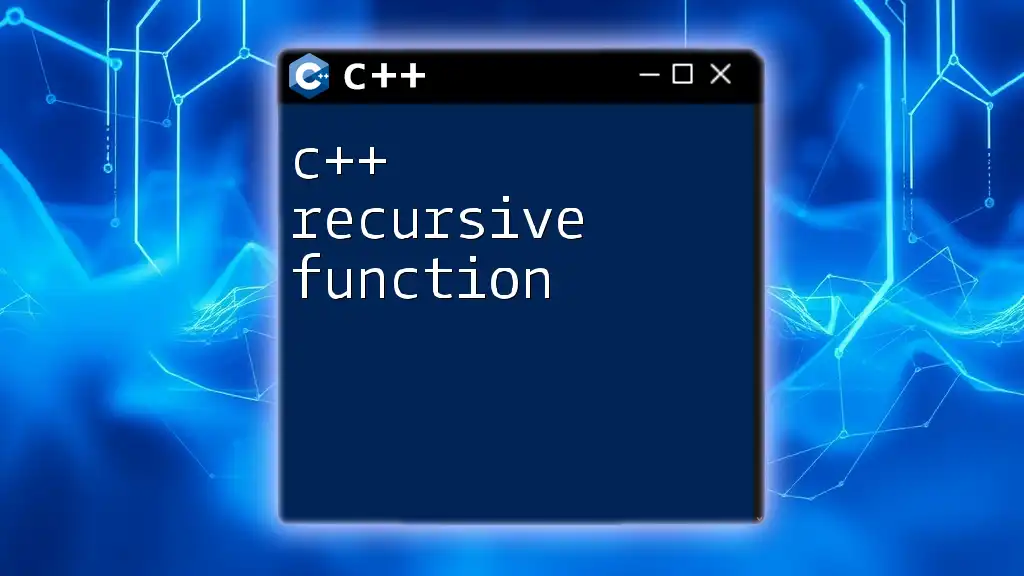
Conclusion
In this guide, you've learned about C++ gRPC fundamentals, from setup to the implementation of both servers and clients. By understanding gRPC architecture, building services, and exploring advanced features, you're well-equipped to integrate gRPC into your C++ applications. Keep experimenting and continue to enhance your skills!
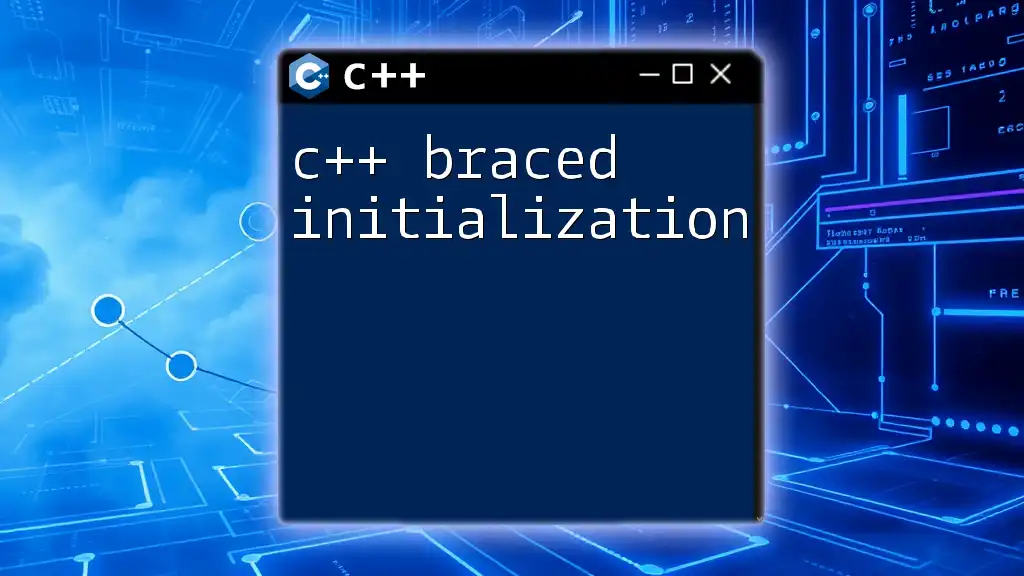
Additional Resources
- Official Documentation: Check out the [gRPC Documentation](https://grpc.io/docs/) and [Protocol Buffers Documentation](https://developers.google.com/protocol-buffers/).
- Community and Support: Participate in forums like Stack Overflow or the gRPC GitHub page for community-driven support and insights.