"SFML (Simple and Fast Multimedia Library) is a powerful C++ library that provides a simple interface to various multimedia components, allowing developers to create interactive applications easily."
Here’s a basic code snippet demonstrating how to create a window using SFML in C++:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "SFML Dev CPP");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.display();
}
return 0;
}
What is SFML?
SFML (Simple and Fast Multimedia Library) is an open-source multimedia library that simplifies the process of developing multimedia applications, including games. It provides a simple API that offers access to various features like graphics, audio, and networking. This abstraction allows developers to focus more on building their application instead of tackling low-level programming intricacies.
SFML is an ideal choice for those looking to dive into game development or other multimedia projects using C++, thanks to its ease of use, flexibility, and high performance.
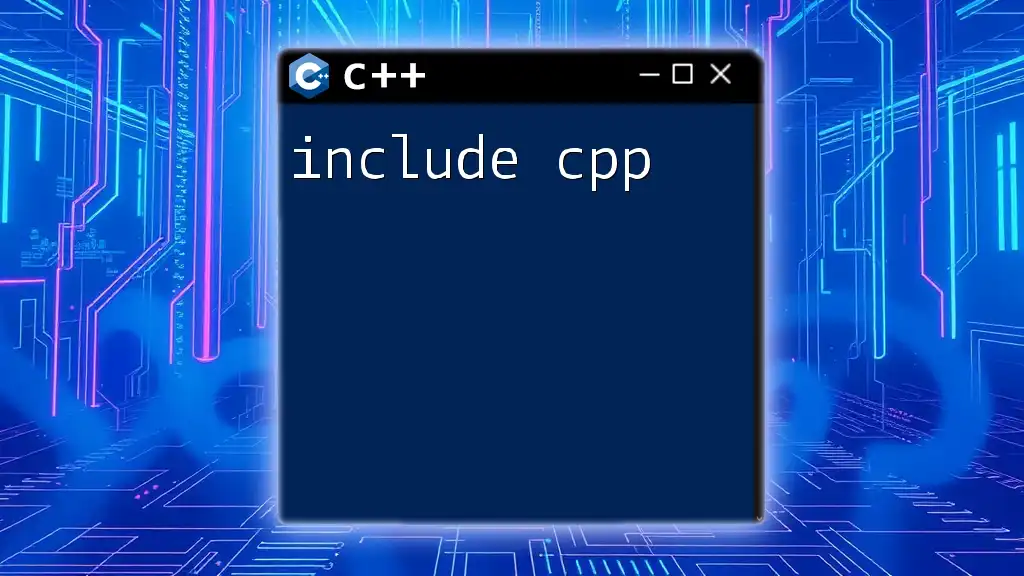
Why Use SFML with C++?
Using SFML in combination with C++ brings numerous benefits to developers:
- High-level Abstraction: Unlike other libraries, SFML provides a minimalistic, yet efficient way to handle various multimedia tasks without delving too deep into complex programming models.
- Cross-platform Support: SFML operates on multiple operating systems, including Windows, macOS, and Linux. This means that you can write your application once and run it everywhere.
- Active Community and Documentation: With an enthusiastic user base and extensive documentation, developers can easily find solutions to common issues and stay updated on best practices.
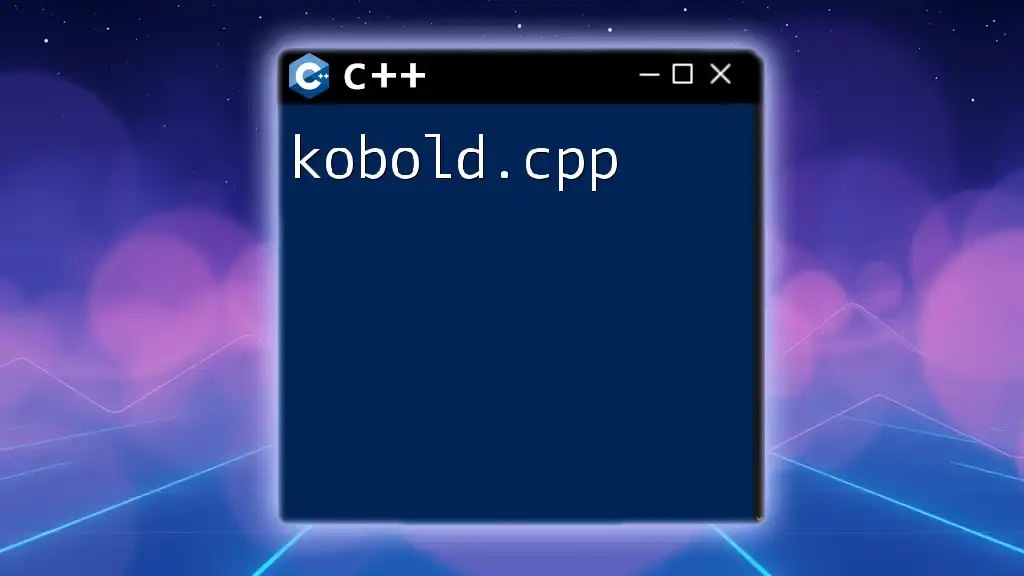
Getting Started with SFML
Installing SFML
To get started with SFML, you first need to install it on your system. Here’s a brief guide on how to do this for various platforms:
-
Windows: You can use vcpkg to install SFML easily:
vcpkg install sfml
Alternatively, download binaries from the SFML website and set them up manually.
-
macOS: If you have Homebrew installed, you can run:
brew install sfml
-
Linux: Use your package manager. For example, on Ubuntu, you can run:
sudo apt-get install libsfml-dev
Setting Up Your Development Environment
For a successful SFML setup, you'll need a suitable IDE. Popular choices include:
- Visual Studio
- CLion
- Code::Blocks
Once you've chosen your IDE, create a new project and configure it to link against the SFML libraries. This usually involves setting include directories and linking against SFML modules such as `sfml-graphics`, `sfml-window`, and `sfml-system`.
Creating Your First SFML Project
To create a simple SFML project, follow this step-by-step outline:
- Open your chosen IDE and create a new C++ project.
- Configure your project settings to include the SFML include directory and link the necessary SFML libraries.
- Write the following code to create and display a simple window:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "SFML Window");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.display();
}
return 0;
}
This code snippet initializes a window with a resolution of 800x600 pixels and includes a basic event loop to handle window closure.
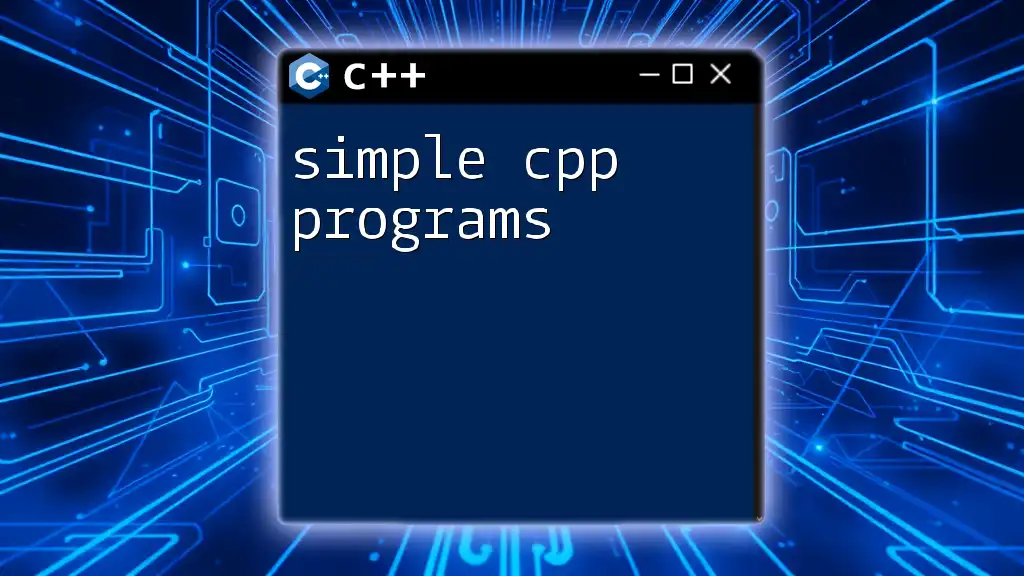
Core Concepts of SFML
SFML Modules Overview
SFML is modular, dividing functionality into several modules:
- Graphics Module: Provides access to 2D graphics technologies.
- Window Module: Responsible for creating windows and managing input from mouse and keyboard.
- Audio Module: Facilitates audio playback, allowing you to add sound effects and music to your application.
Creating a Simple Window
The example in the previous section showed how to create a window. This is often the first step in any sfml dev cpp project.
Understanding SFML Events
Event handling is crucial in an interactive application. SFML simplifies responding to user inputs. For example, consider code that reacts to keyboard and mouse events:
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
if (event.type == sf::Event::KeyPressed) {
if (event.key.code == sf::Keyboard::Escape) {
window.close();
}
}
}
}
This loop listens for the window's close event and the Escape key press. Understanding events allows you to make your applications interactive.
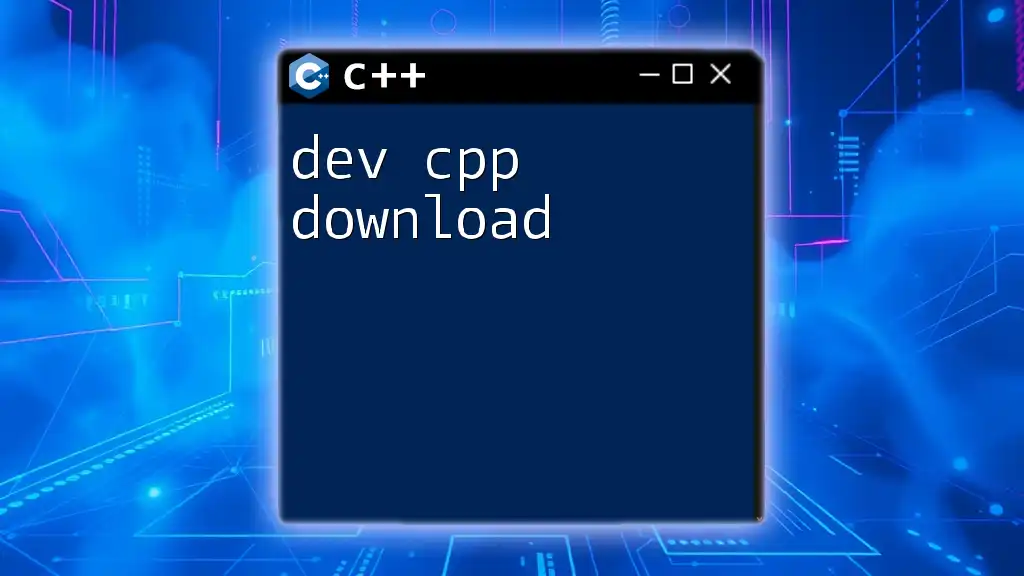
Drawing with SFML
Basic Shapes and Colors
SFML makes it easy to draw shapes using its graphics module. You can create various 2D shapes and set their colors, making visual elements stand out.
For example, to draw a green circle:
sf::CircleShape circle(50); // Radius of 50
circle.setFillColor(sf::Color::Green);
window.draw(circle);
Text and Fonts
Displaying text is another essential feature of SFML. To display text, first, you need to load a font:
sf::Font font;
font.loadFromFile("arial.ttf");
sf::Text text("Hello SFML", font, 30);
text.setFillColor(sf::Color::White);
window.draw(text);
Make sure you have the font file available in your project directory!
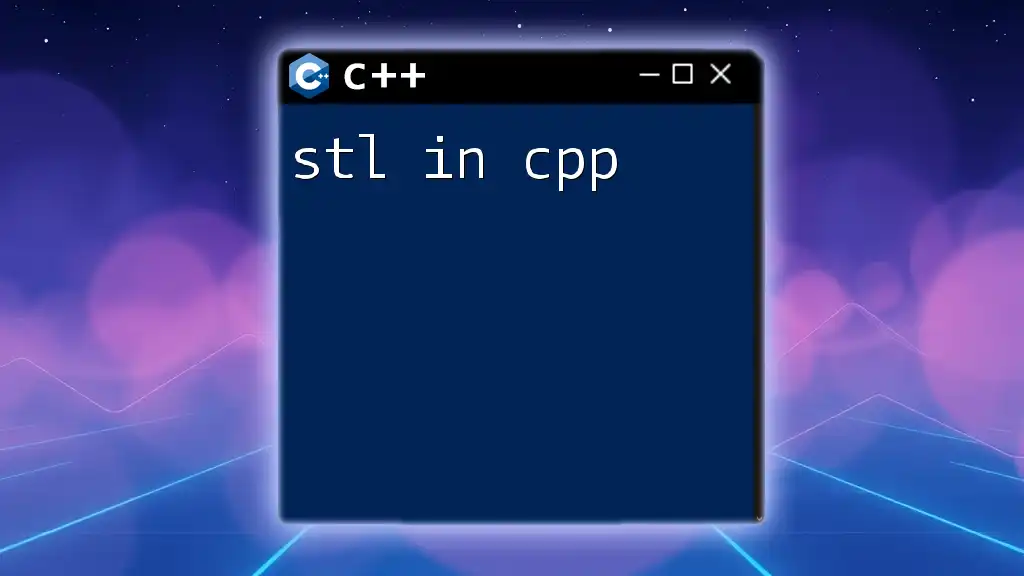
Advanced Graphics Techniques
Transformations and Animations
Using transformations like scaling, rotation, and translation enriches your graphics. For example, to rotate a shape:
circle.setRotation(45); // Rotate 45 degrees
Animating objects, like moving a circle across the screen, can be achieved by changing its position frame by frame in the game loop.
Using Textures and Sprites
Textures allow you to apply images to your shapes, turning them into sprites.
Here’s how you can load a texture and create a sprite:
sf::Texture texture;
texture.loadFromFile("image.png");
sf::Sprite sprite(texture);
window.draw(sprite);
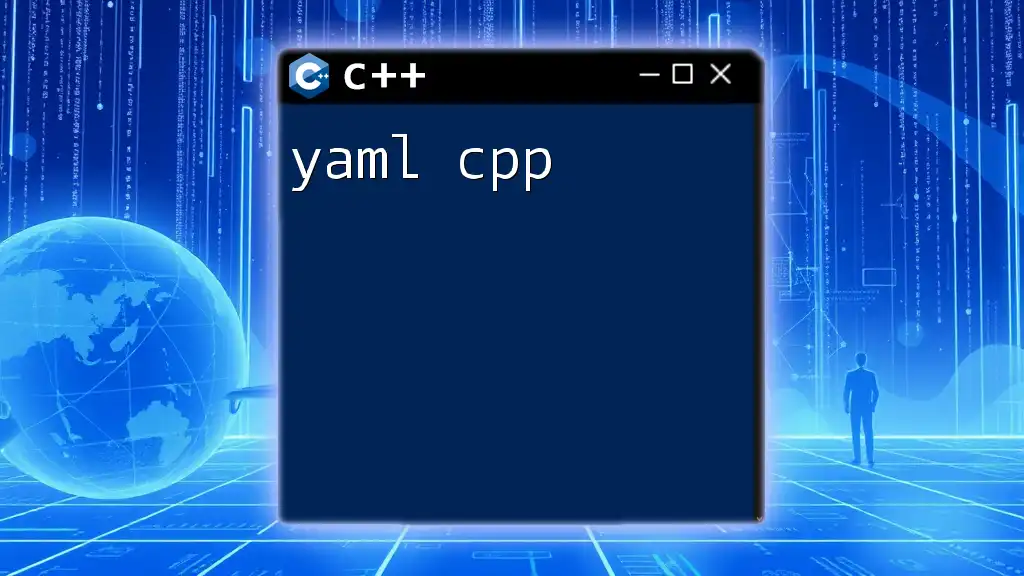
Audio in SFML
Playing Sounds and Music
Adding sound effects and background music enhances user experience. SFML supports multiple audio formats, allowing you to play sounds and music effortlessly.
Here’s how to handle sound:
sf::SoundBuffer buffer;
buffer.loadFromFile("sound.wav");
sf::Sound sound(buffer);
sound.play();
This code snippet loads a sound file and plays it.
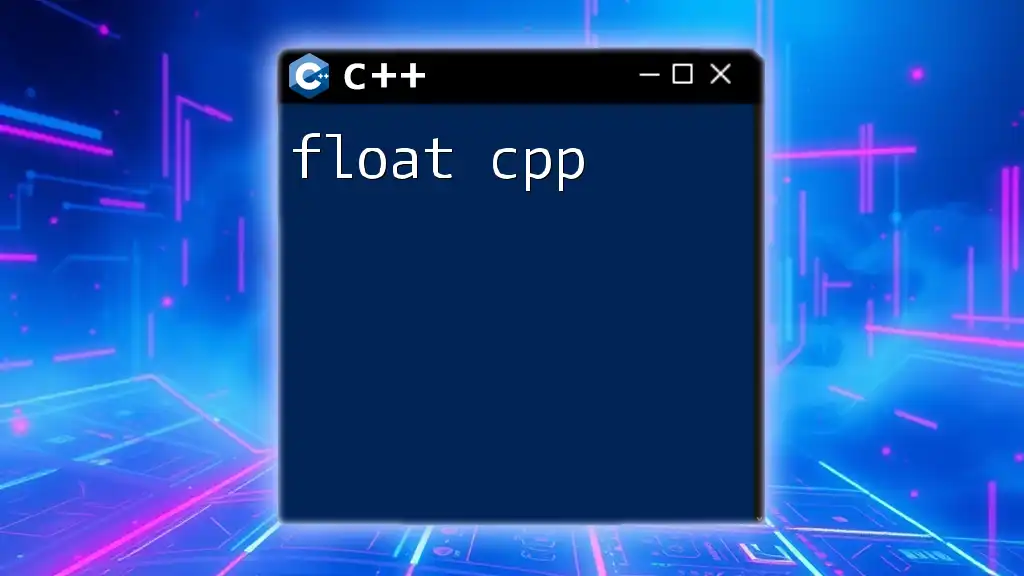
Building Complete Projects with SFML
Project Structure and Best Practices
Maintaining an organized project structure is critical, especially when your application grows. Consider separating your source files, header files, and resources into distinct directories. This practice enhances readability and maintainability.
Moreover, using Git for version control allows you to track changes effectively and revert to previous states if necessary.
Example Project: A Simple Game
Imagine creating a simple shooting game. You would want to implement mechanics like player movement, shooting bullets, and handling enemies. For instance:
- Game Loop: Create a loop to handle updates and renders.
- Collision Detection: Use bounding boxes or circles to manage interactions between game entities.
if (player.getGlobalBounds().intersects(enemy.getGlobalBounds())) {
// Handle collision
}
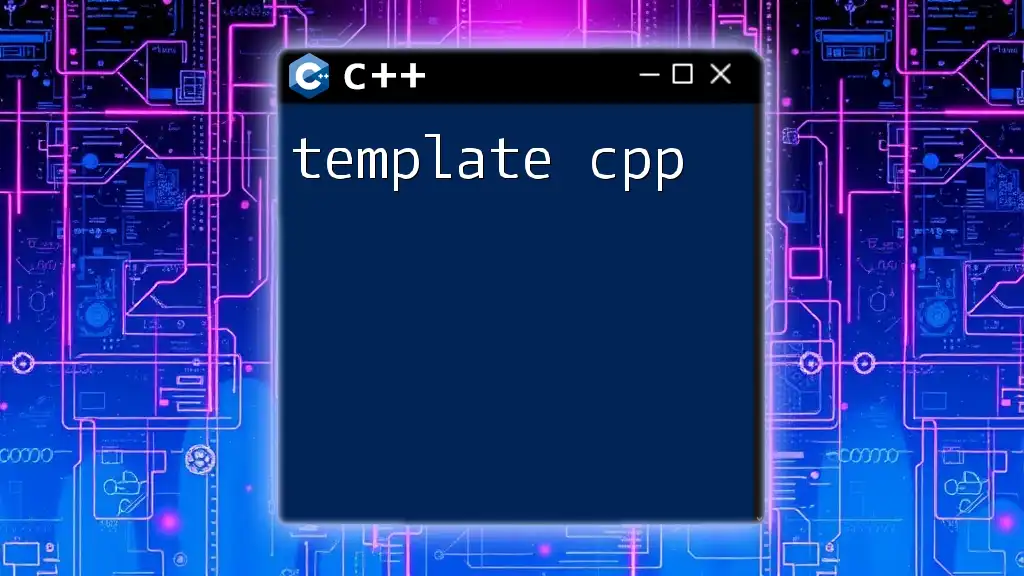
Troubleshooting Common SFML Issues
Common Errors and Fixes
While working with SFML, you may encounter linking errors. These often arise from not linking the required libraries. Make sure that the library paths and linker settings in your IDE are correctly set.
Runtime errors usually point to issues in your code logic or resource loading failures. Utilize debugging tools within your IDE or add logging to help trace these issues.
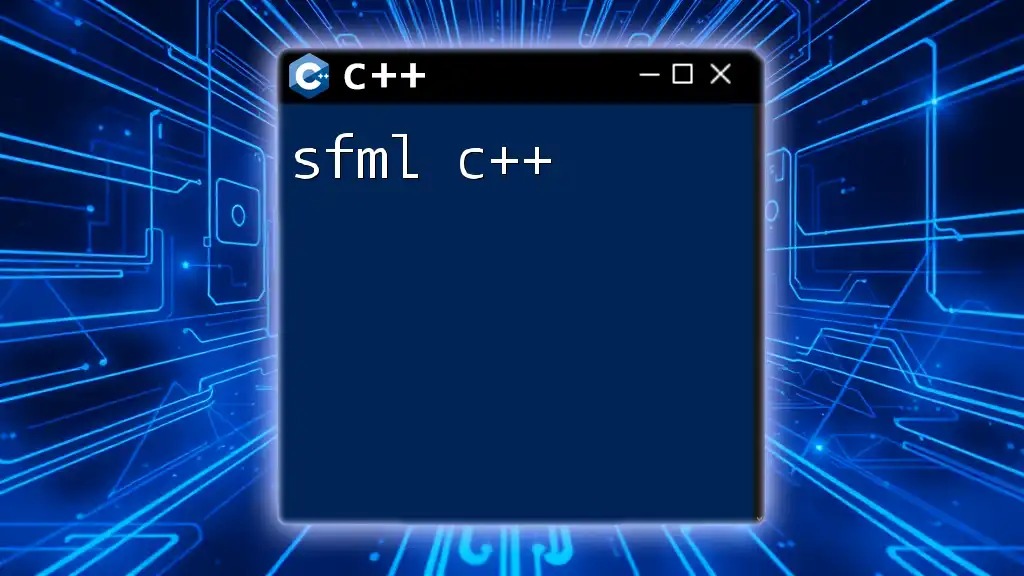
Final Thoughts on SFML and C++
SFML is a powerful yet user-friendly library that can significantly reduce the complexity of multimedia application development. By mastering the topics outlined in this guide, you will be well-equipped to create your own engaging applications using sfml dev cpp.
Moreover, don’t hesitate to explore further resources and engage with the SFML community. This will allow you to enhance your skills and stay up to date with the latest advancements. Happy coding!