Resource Acquisition Is Initialization (RAII) in C++ is a programming idiom that ensures resource management by tying resources' lifecycle to the lifespan of objects, automatically releasing resources when the object goes out of scope.
Here's a simple code snippet demonstrating RAII in action:
#include <iostream>
#include <memory>
class Resource {
public:
Resource() { std::cout << "Resource acquired.\n"; }
~Resource() { std::cout << "Resource released.\n"; }
};
void useResource() {
Resource res; // Resource is acquired here
// Use resource...
} // Resource is released automatically when going out of scope
int main() {
useResource();
return 0;
}
What is RAII?
Resource Acquisition Is Initialization (RAII) is a programming idiom used in C++ to manage resource lifetimes effectively. The fundamental principle of RAII is to bind the lifecycle of a resource — such as memory, file handles, or network connections — to the lifespan of an object. When the object is created, the resource is acquired; when the object is destroyed, the resource is released. This ensures that resources are properly cleaned up without requiring explicit deallocation by the programmer.
Why RAII Matters
In C++, managing resources manually can lead to several issues such as memory leaks, dangling pointers, and resource exhaustion, which can significantly affect an application’s performance and stability. RAII provides a systematic way to handle these issues with minimal overhead.
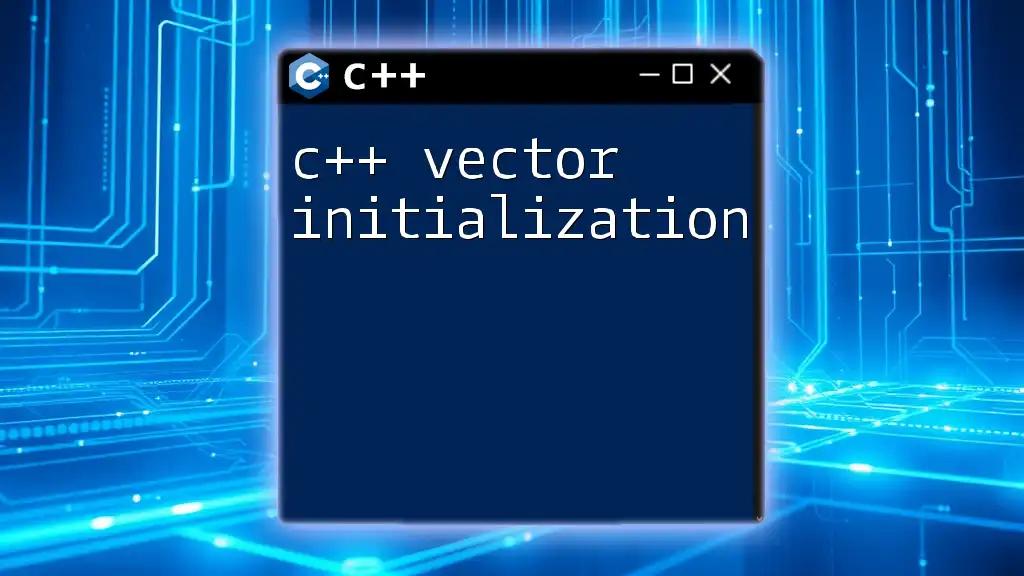
Historical Context
C++ has a rich history concerning resource management. Before RAII became a widely accepted practice, developers relied heavily on manual memory management and explicit calls to free resources. This method was error-prone and often led to resource leaks and undefined behavior. The introduction of RAII shifted the paradigm, emphasizing automatic resource management and exception safety within C++.

Understanding Resources in C++
Types of Resources
In C++, resources can be categorized into several types:
- Memory: Both stack and heap memory are used in variable allocation.
- File Handles: Opening, reading, and closing files, which must be managed carefully to avoid file descriptor leaks.
- Network Connections: Resources allocated for network communication, such as sockets, require proper handling to ensure connections are closed appropriately.
The Importance of Proper Resource Management
Improper resource management can lead to numerous problems:
- Memory Leaks: When memory is allocated but not deallocated, it continues to consume resources, eventually leading to application crashes.
- Dangling Pointers: Pointers that reference freed memory can cause undefined behavior or security vulnerabilities.
- Resource Exhaustion: Running out of resources like file handles or network connections due to poor management can halt application functionality.

Core Principles of RAII
How RAII Works
RAII operates on a straightforward principle: resources are acquired during an object's construction and released during its destruction. This guarantees that resources are properly managed regardless of how the objects are used in the code.
Automatic Resource Management Using Destructors
C++ utilizes destructors to manage resource deallocation automatically. When an object goes out of scope, its destructor is invoked, ensuring that any associated resources are released. Consider the following example:
class Resource {
public:
Resource() {
// Acquire resource, e.g., allocating memory
}
~Resource() {
// Release resource, e.g., deallocating memory
}
};
In this example, the `Resource` class acquires a resource upon construction and guarantees its release when the object is destroyed, whether that is through normal flow or via exceptions.

Implementing RAII in Your Code
Creating RAII Classes
Creating classes that utilize RAII principles allows for clear resource management. For instance, when managing file resources, you might design a class as follows:
class FileGuard {
private:
FILE* file;
public:
FileGuard(const char* filename) {
file = fopen(filename, "r");
if (!file) {
throw std::runtime_error("Failed to open file");
}
}
~FileGuard() {
if (file) {
fclose(file);
}
}
};
Here, `FileGuard` automatically handles resource acquisition and deallocation. If the file fails to open, an exception is thrown, preventing resource leaks.
Using std::unique_ptr and std::shared_ptr
C++11 introduced smart pointers, which are critical for RAII. Smart pointers manage the memory they point to automatically. Here’s an example using `std::unique_ptr`:
#include <memory>
void example() {
std::unique_ptr<int> ptr = std::make_unique<int>(10);
// Automatically frees memory when ptr goes out of scope.
}
Using smart pointers simplifies resource management and reinforces the RAII concept, as they ensure that memory is freed automatically.

Advantages of RAII
Safety and Clarity
RAII significantly enhances the safety and clarity of code. It reduces the risk of resources leaking, as the lifetime of resources is tightly bound to object lifetimes.
Exception Safety
One of the key advantages of RAII is its built-in mechanism for handling exceptions. If an exception occurs, destructors are still invoked, ensuring that resources are cleaned up properly. Consider this example:
void riskyFunction() {
FileGuard file("example.txt");
// Code that might throw an exception
}
In this function, even if an exception is thrown while processing, the destructor of `FileGuard` will ensure that the file is closed, maintaining resource integrity.
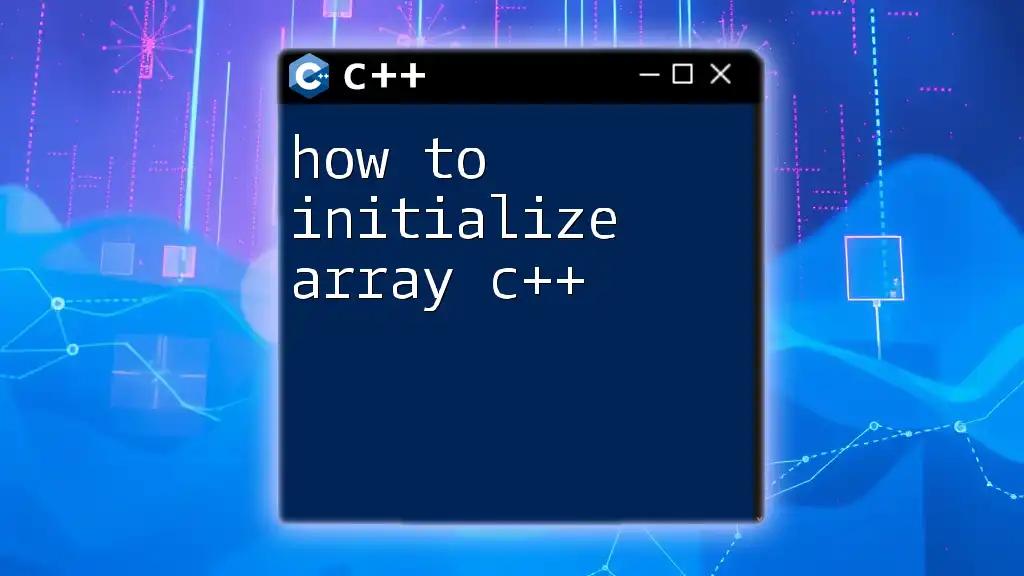
Common Pitfalls and How to Avoid Them
Misuse of RAII
While RAII is powerful, its misuse can lead to issues. Common mistakes include overusing RAII and not understanding object ownership, leading to unexpected behaviors and performance degradation.
Cyclic Dependencies in Smart Pointers
Using `std::shared_ptr` can introduce cyclic dependencies if two objects refer to each other. This prevents their destructors from being called, leading to memory leaks. Using `std::weak_ptr` can break this cycle by allowing one pointer to reference an object without extending its lifetime.
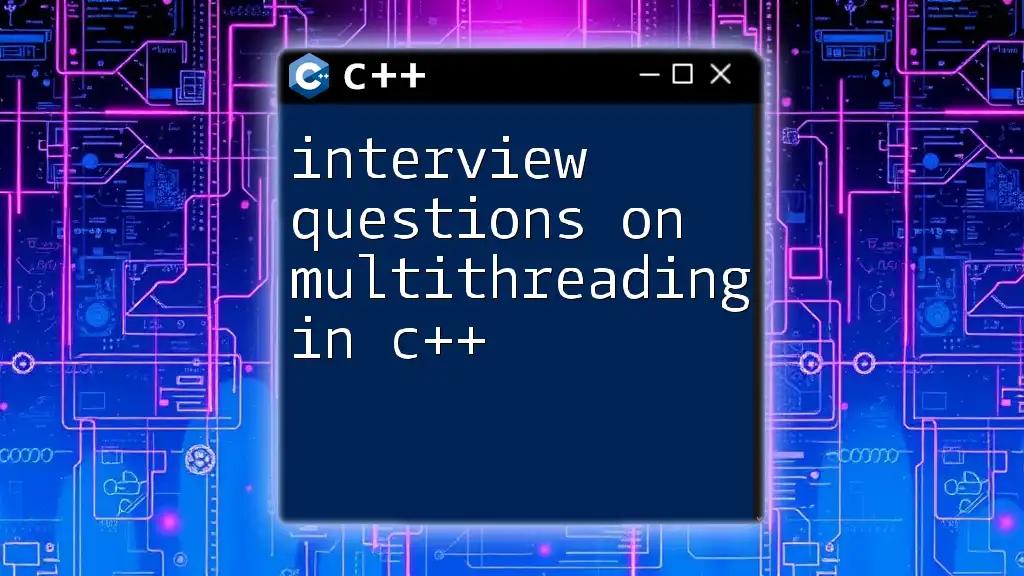
Best Practices for RAII in C++
Keeping RAII Classes Simple
Design RAII classes to be simple and focused. Each class should manage a single resource to avoid complexity and make management straightforward.
Use of Standard Library Features
Leverage the features provided by the C++ Standard Library, such as `std::unique_ptr` and `std::shared_ptr`, to maximize the benefits of RAII and minimize manual memory management.
Creating Custom Resource Managers
Design reusable RAII wrappers for various resources, making them applicable across different projects. This promotes code reusability and standardization in resource management.

Conclusion
RAII is a cornerstone of effective resource management in C++. By adhering to the principles of RAII, developers can ensure that resources are automatically acquired and released, mitigating the risks of leaks and undefined behaviors. Embracing this idiom not only enhances the reliability of applications but also leads to cleaner, more maintainable code.
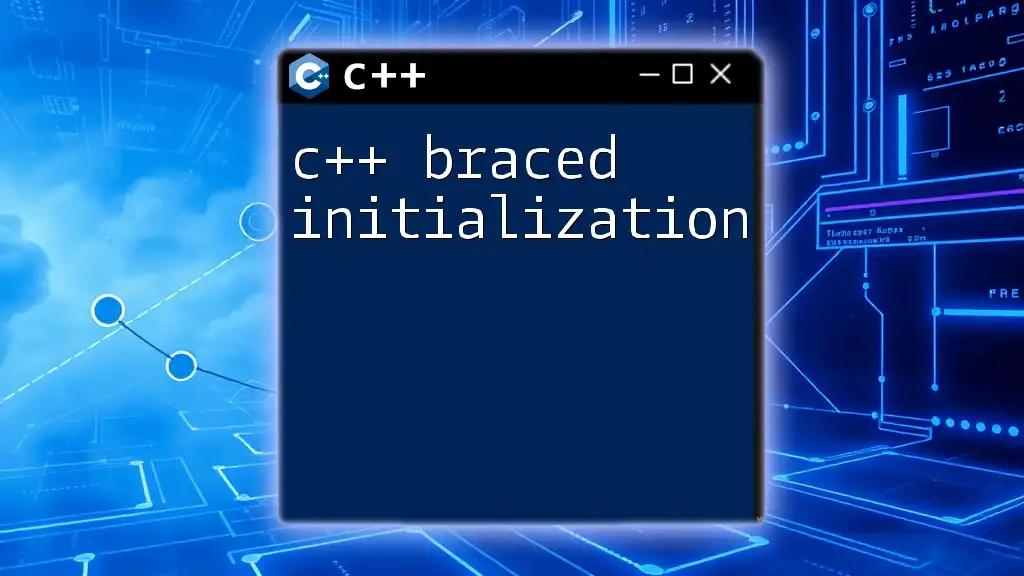
Additional Resources
For further exploration of RAII in C++, consider diving into comprehensive texts on C++, as well as participating in online forums and communities where you can share insights and seek guidance. Engaging with others who share an interest in C++ will deepen your understanding and broaden your knowledge of best practices in resource management.