The "C++ no such file or directory" error typically occurs when the compiler cannot find a specified header file or source file due to an incorrect file path or missing file.
Here's a code snippet for illustration:
#include "myHeader.h" // Ensure 'myHeader.h' exists in the project directory or specify the correct path
What Does "No Such File or Directory" Mean?
The "no such file or directory" error is a common issue encountered in C++ programming, particularly when dealing with file management. This error signifies that the C++ program has attempted to access a file or a directory that it cannot find. Understanding the underlying causes of this error is crucial for both novice and experienced programmers.
Common Causes:
- Missing Files: The file explicitly referenced in the code does not exist in the specified location.
- Incorrect Paths: The file path provided may be incorrect or the file could be located in a different directory.
- Permission Issues: Sometimes, the file may exist, but the program lacks the necessary permissions to access it.
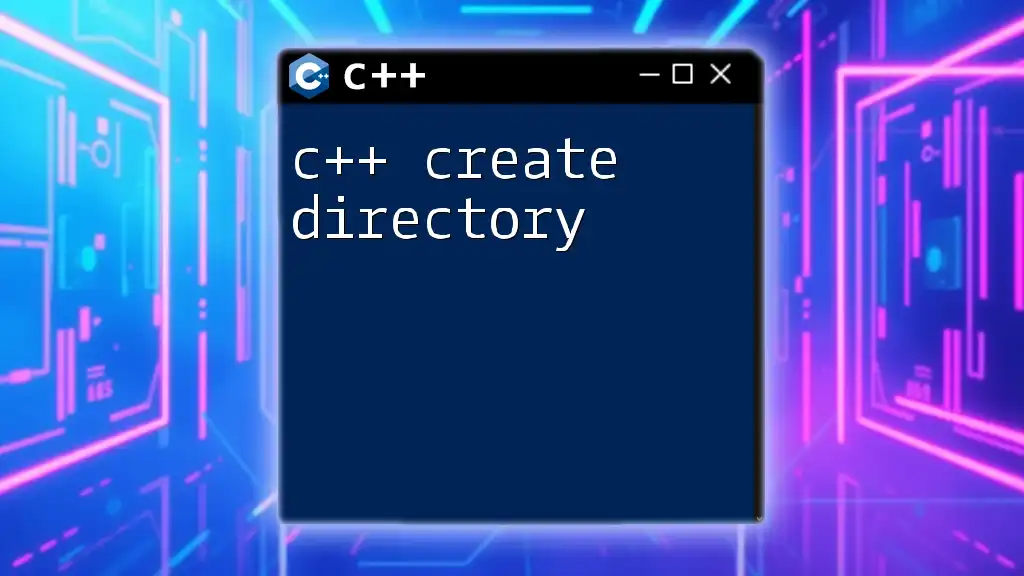
Common Scenarios Leading to the Error
Including Header Files
One of the first places you might run into the no such file or directory error is when you're including header files.
If the header file is missing or named incorrectly, the compiler will not be able to locate it.
Example:
#include "missingfile.h"
In the above code snippet, the file "missingfile.h" is likely to cause an error if it does not exist in the specified include directories.
Correcting Path Issues: Always make sure to double-check the name of the header files and their paths, especially in projects with multiple directories. If your header files are stored in a dedicated directory, ensure that your compiler knows where to locate them, often done using flags in your build commands (e.g., `-I` flag for GCC).
File Operations
Another frequent trigger of the no such file or directory error occurs when performing file input/output operations.
When you attempt to open a file that does not exist, the failure can lead to this error.
Example:
std::ifstream file("data.txt");
if (!file) {
std::cerr << "Could not open the file!" << std::endl;
}
In this code segment, if "data.txt" does not exist in the current directory (or the path is incorrect), it will output an error message to the console.
Check for Existence: Before performing file operations, consider validating the existence of the file using the filesystem library introduced in C++17:
#include <filesystem>
namespace fs = std::filesystem;
if (!fs::exists("data.txt")) {
std::cerr << "File does not exist!" << std::endl;
}
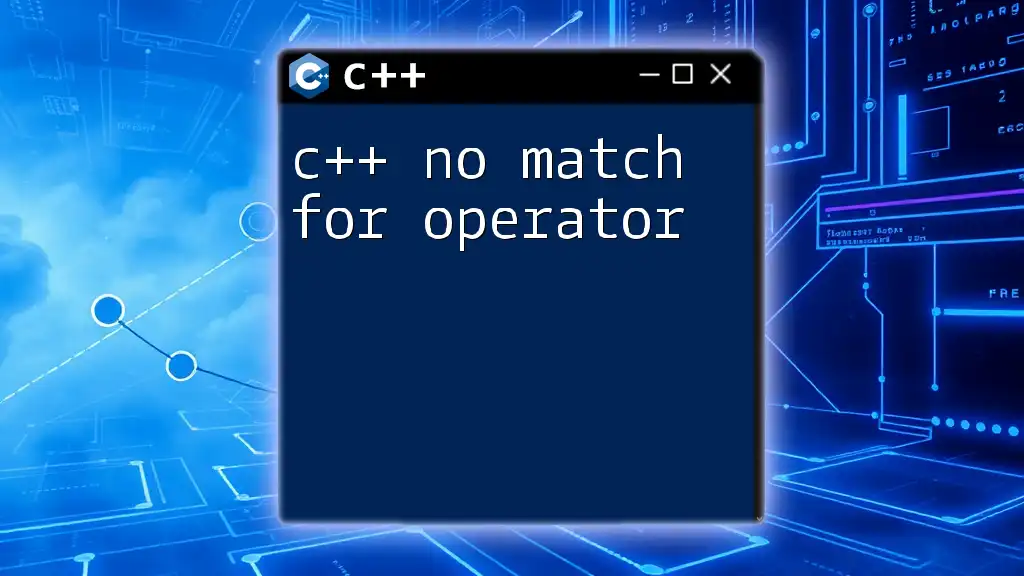
Fixing "No Such File or Directory" Errors
Check File Names and Paths
One of the first steps in resolving the no such file or directory error is to verify the file names and paths. Given that file paths can be case-sensitive, particularly in Linux environments, it is essential to ensure the correct casing is used.
Using Absolute vs. Relative Paths:
- Absolute Paths specify the complete path from the root directory to the file.
- Relative Paths depend on the current working directory of the program. Misunderstandings here can easily lead to this error.
For example, using:
std::ifstream file("/home/user/data.txt"); // Absolute path
versus
std::ifstream file("data.txt"); // Relative path
Program behavior can differ widely based on the current directory.
Ensure Correct Include Directives
Verifying your include directives is crucial. Incorrectly set include paths can lead to errors.
- Include Paths Configuration: Check the settings in your IDE or build system. For instance, in `g++,` the `-I` flag can be used to specify directories to search for header files:
g++ -I/path/to/headers main.cpp -o main
- Best Practices for Header Management: Organize header files into dedicated directories and keep naming conventions consistent. This minimizes confusion and helps avoid errors during compilation.
Verify File Permissions
In Unix/Linux systems, permission issues can also lead to the no such file or directory error. Even if a file exists, the accessing program might not have the required permissions.
Example Command:
You can use the command below to check the permissions of a file:
ls -l filename
Review the output to ensure the necessary read/write permissions are set for the user. If permissions need to be modified, you can adjust them:
chmod +r filename
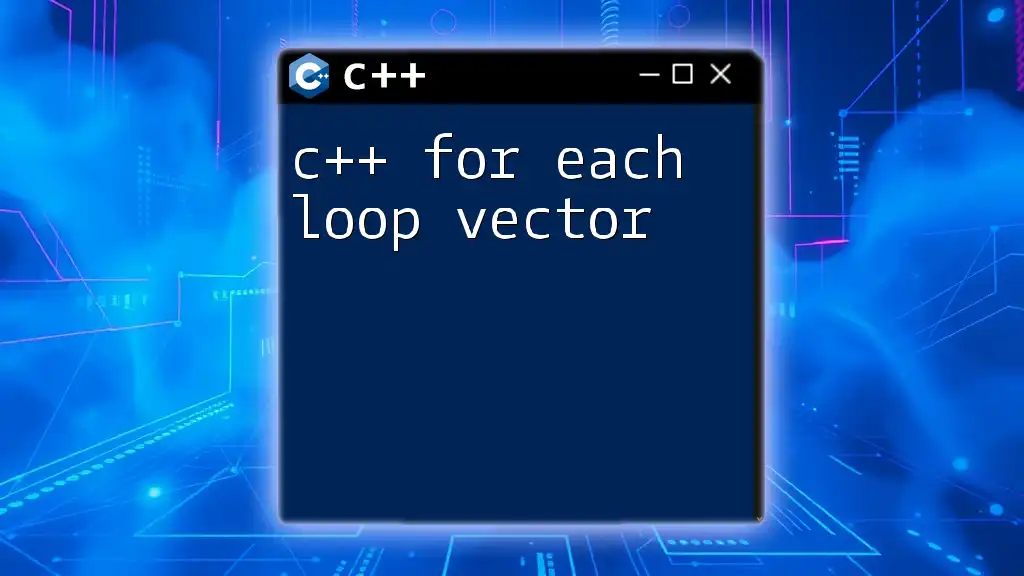
Troubleshooting Tips
Using Diagnostic Tools
Employ diagnostic tools like gdb for debugging your program or strace to monitor system calls including file accesses. These tools can provide insights into what the program is attempting to do and where it fails.
Logging Errors
Implementing error logging in your C++ program can help in capturing and diagnosing issues related to file access. For example:
std::ofstream log("error.log", std::ios_base::app);
if (!file) {
log << "Error: No such file or directory!" << std::endl;
}
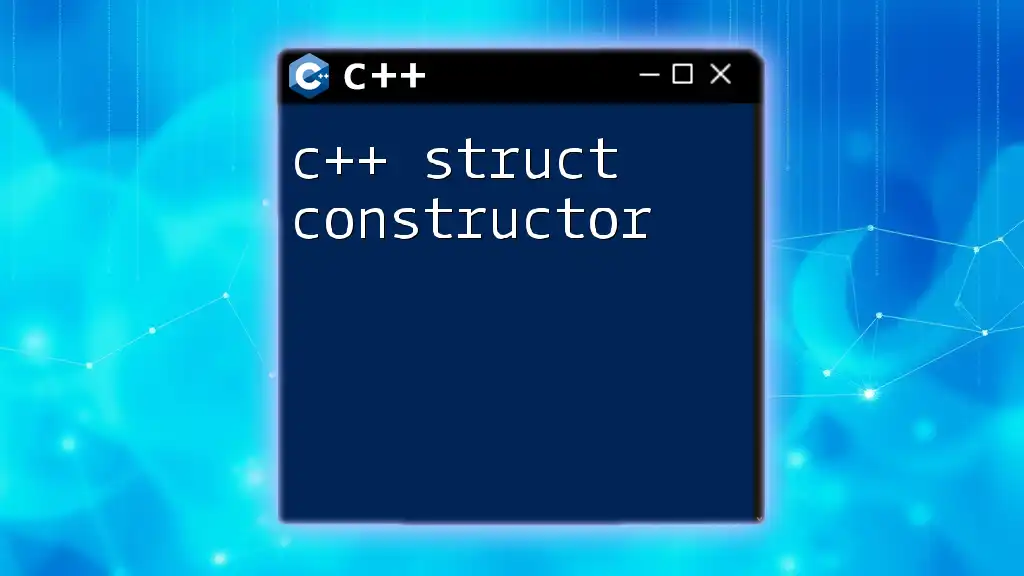
Example Code and Walkthrough
Here’s an example C++ program that demonstrates this error:
#include <iostream>
#include <fstream>
int main() {
std::ifstream file("nonexistent.txt");
if (!file) {
std::cerr << "Error: No such file or directory!" << std::endl;
}
return 0;
}
Walkthrough: In this code, the program attempts to open a file named "nonexistent.txt." When it fails, it outputs an error message. If you correct the filename or place the file in the directory where your program is running, this issue resolves.
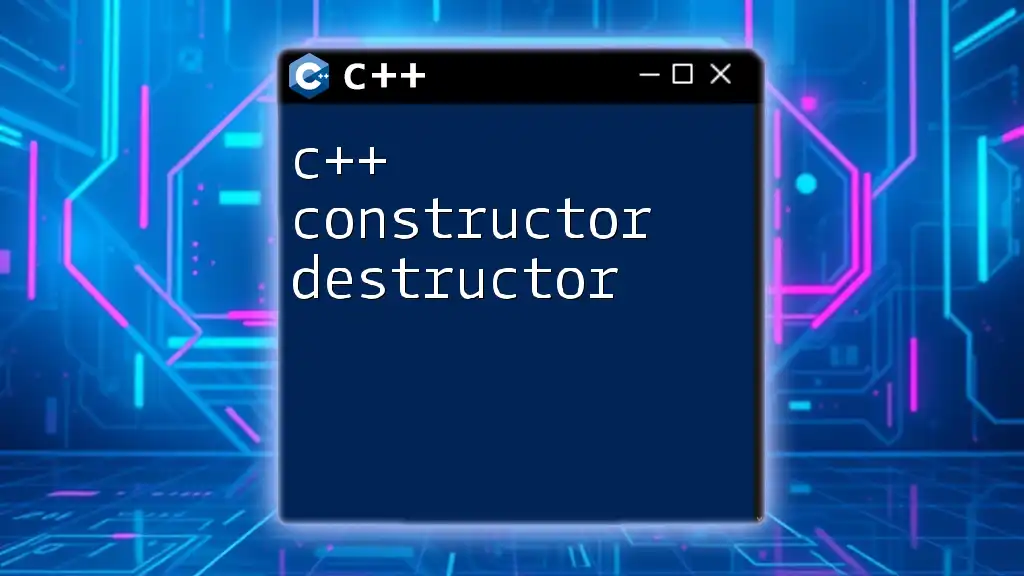
Preventing "No Such File or Directory" Errors
Development Best Practices
Developers can prevent the no such file or directory errors by adhering to best practices:
- Maintain consistent naming conventions for files and directories to reduce confusion.
- Use version control systems like Git which can track changes, making it easier to spot missing files.
Testing and Validation Before Execution
Incorporating unit tests that specifically check for file-related functionality can catch potential issues early in the development cycle, thus avoiding runtime errors.
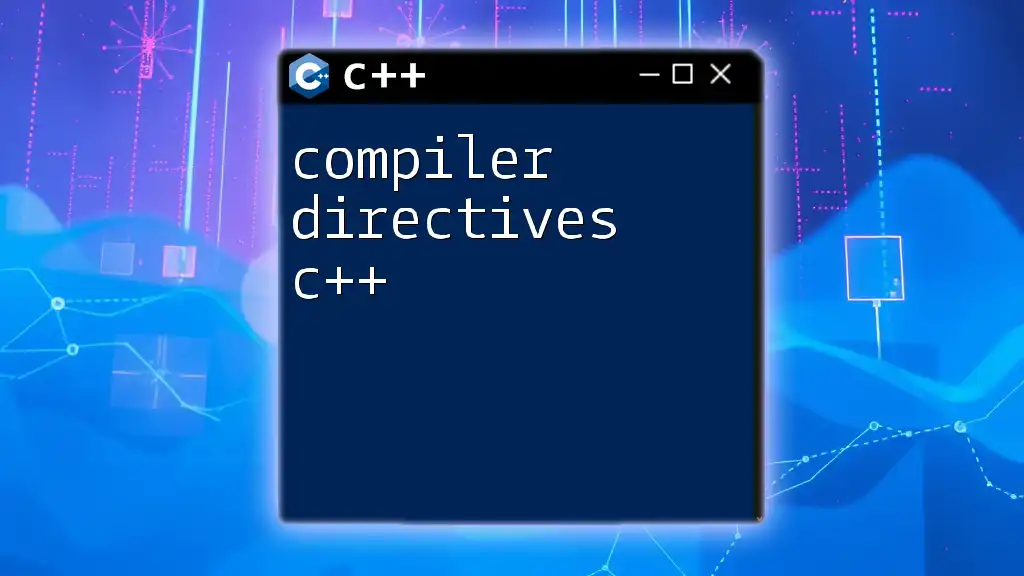
Conclusion
Understanding the "no such file or directory" error and its causes is vital for effective C++ programming. By paying attention to file management, paths, permissions, and systematic debugging, developers can greatly enhance their productivity and efficiency. Ensure that you not only react to errors as they occur but also implement practices that prevent them from happening in the first place.
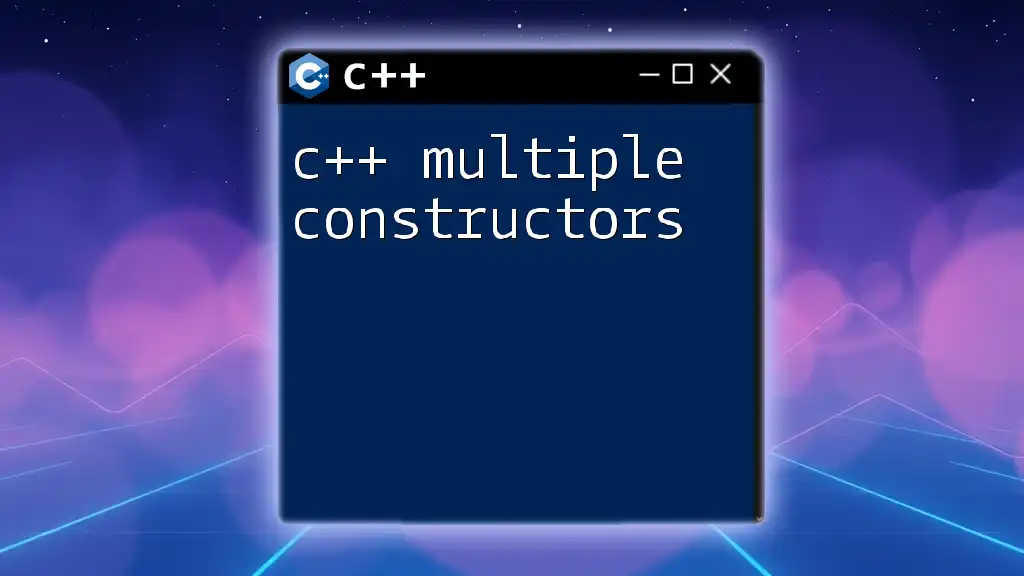
Additional Resources
For further reading on error handling and file input/output in C++, consider these resources:
- [C++ Reference Documentation](https://en.cppreference.com/)
- Books like "The C++ Programming Language" by Bjarne Stroustrup.
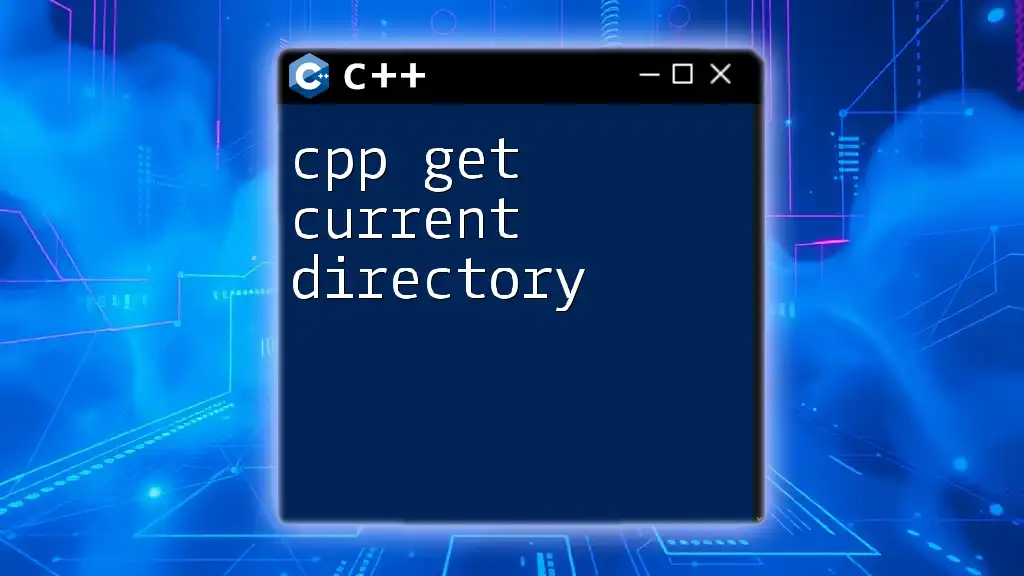
Call to Action
If you found this article helpful, consider subscribing to our blog for more insights on C++ programming. Join our community for discussions and tips that can help you overcome challenges and master C++.