In C++, the transpose of a matrix is obtained by swapping its rows with columns, allowing you to represent the same data in a different orientation. Here’s a simple code snippet to illustrate this:
#include <iostream>
using namespace std;
void transpose(int original[3][3], int transposed[3][3]) {
for (int i = 0; i < 3; i++)
for (int j = 0; j < 3; j++)
transposed[j][i] = original[i][j];
}
int main() {
int matrix[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
int transposed[3][3];
transpose(matrix, transposed);
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++)
cout << transposed[i][j] << " ";
cout << endl;
}
return 0;
}
What is a Matrix Transpose?
The transpose of a matrix in C++ is the operation of flipping the matrix over its diagonal. This means that the row and column indices of the matrix are swapped. For example, if you have a matrix A that is represented by:
A = | 1 2 3 |
| 4 5 6 |
The transpose A^T would look like this:
A^T = | 1 4 |
| 2 5 |
| 3 6 |
This simple yet powerful operation has far-reaching applications, including vector transformations, computer graphics, and even algorithms like the Singular Value Decomposition (SVD) used in machine learning.
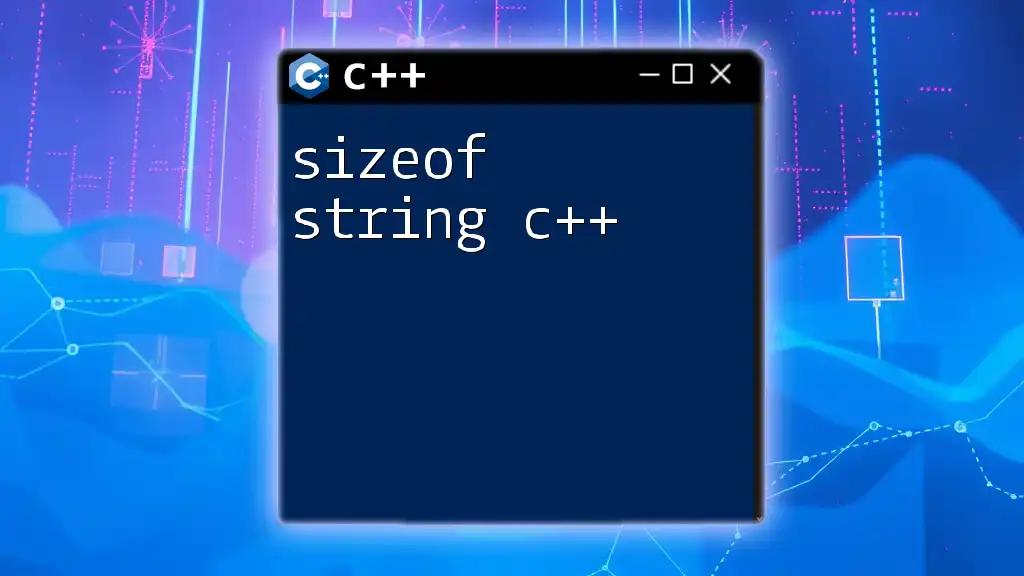
Understanding Matrix Representation in C++
Declaring a Matrix in C++
In C++, matrices can be represented using two-dimensional arrays. A matrix with `m` rows and `n` columns can be declared as:
int matrix[ROWS][COLS];
Where `ROWS` and `COLS` are the dimensions of your matrix. An example would look like this:
const int ROWS = 2;
const int COLS = 3;
int matrix[ROWS][COLS] = { {1, 2, 3}, {4, 5, 6} };
Dynamic Allocation of Matrices
For matrices of unknown size determined at runtime, a dynamic approach using pointers and arrays is often employed. Here's how you can create a 2D dynamic array:
int** createMatrix(int rows, int cols) {
int** matrix = new int*[rows];
for (int i = 0; i < rows; i++) {
matrix[i] = new int[cols];
}
return matrix;
}
Make sure to eventually `delete` the allocated memory to prevent memory leaks:
void deleteMatrix(int** matrix, int rows) {
for (int i = 0; i < rows; i++) {
delete[] matrix[i];
}
delete[] matrix;
}
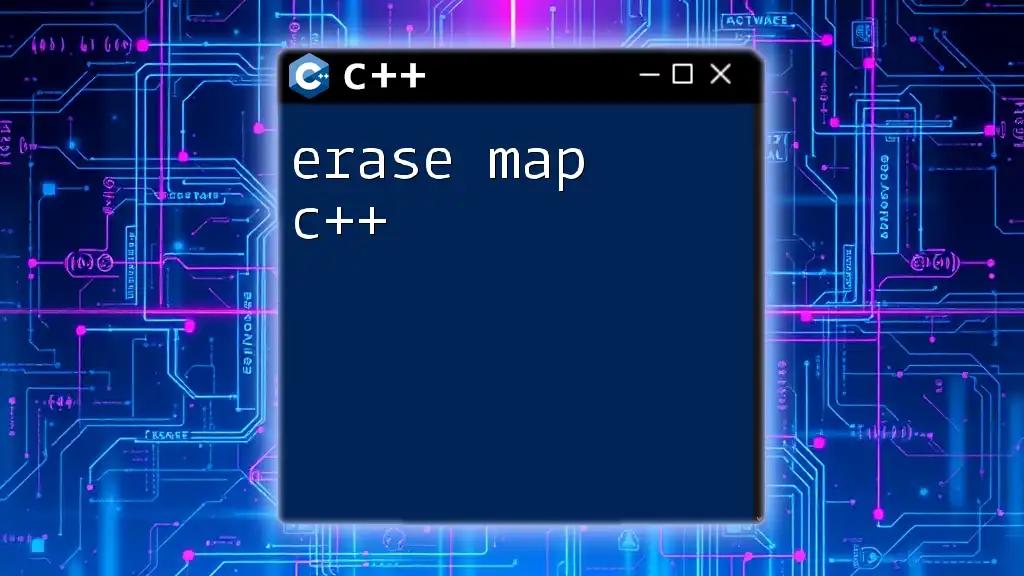
Techniques to Transpose a Matrix in C++
Method 1: Using a Nested Loop
The most straightforward way to transpose a matrix in C++ is through a nested loop approach. This method involves iterating over rows and columns of the original matrix and assigning values to the transposed matrix.
Here’s a simple implementation:
void transposeMatrix(int matrix[][COLS], int transposed[][ROWS]) {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
transposed[j][i] = matrix[i][j];
}
}
}
In the above example, the value at position `(i, j)` in the original matrix is assigned to position `(j, i)` in the transposed matrix. This code effectively flips the rows and columns, transforming the matrix.
Method 2: Using std::vector
Using `std::vector` is a more modern approach that provides better memory management. The syntax is cleaner and avoids manual memory management headaches:
void transposeMatrix(const std::vector<std::vector<int>>& matrix, std::vector<std::vector<int>>& transposed) {
int rows = matrix.size();
int cols = matrix[0].size();
transposed.resize(cols, std::vector<int>(rows));
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
transposed[j][i] = matrix[i][j];
}
}
}
This snippet uses the `resize` method from `std::vector` to create the transposed matrix with the appropriate dimensions. It provides dynamic sizing, allowing your code to adapt to different matrix sizes without extra management.
Method 3: Transposing in Place (for Square Matrices)
For square matrices (where the number of rows equals the number of columns), you can perform transposition in place. This approach minimizes additional memory usage, making it more efficient:
void transposeInPlace(std::vector<std::vector<int>>& matrix) {
int n = matrix.size();
for (int i = 0; i < n; i++) {
for (int j = i + 1; j < n; j++) {
std::swap(matrix[i][j], matrix[j][i]);
}
}
}
This code uses the `std::swap` function to interchange elements only when necessary, thus achieving a transposed matrix without additional space. Remember, this method can only be used for square matrices.
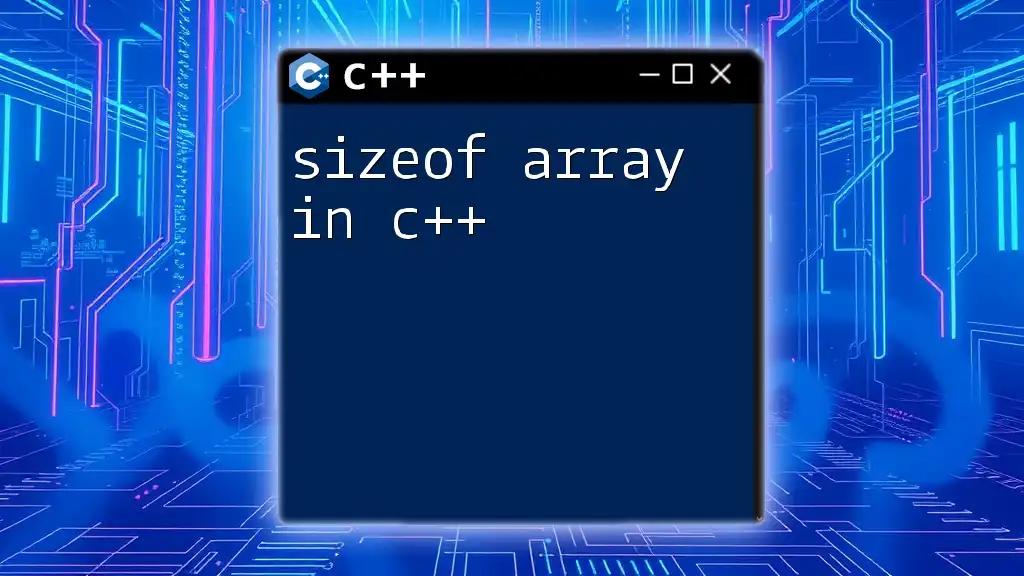
Visualizing Matrix Transpose
Visualizing matrix operations can significantly enhance understanding. When you transpose a matrix, envision flipping it over its diagonal. This mental image can help clarify how the original and transposed matrices relate to one another.
Consider the original and transposed matrices:
Original:
| 1 2 3 |
| 4 5 6 |
Transposed:
| 1 4 |
| 2 5 |
| 3 6 |
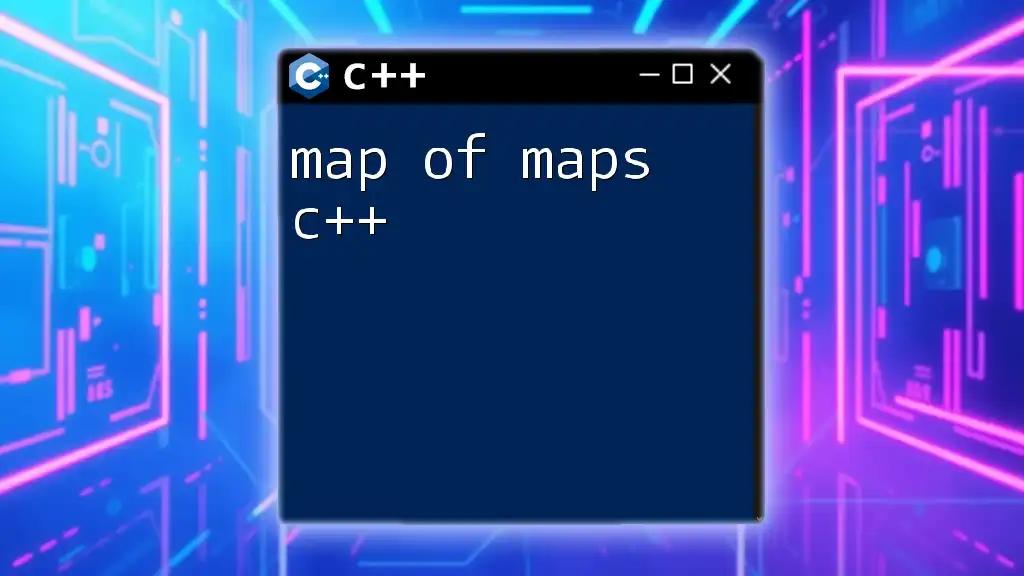
Common Mistakes and Troubleshooting
When performing the transpose of a matrix in C++, some common pitfalls include:
- Index Out of Bounds: Always check indexing to avoid accessing memory outside the declared array.
- Matrix Size Mismatch: Ensure that the dimensions of the original and transposed matrices align appropriately.
- Dangling Pointers: If you're using dynamic memory, always delete matrices when done to prevent memory leaks.
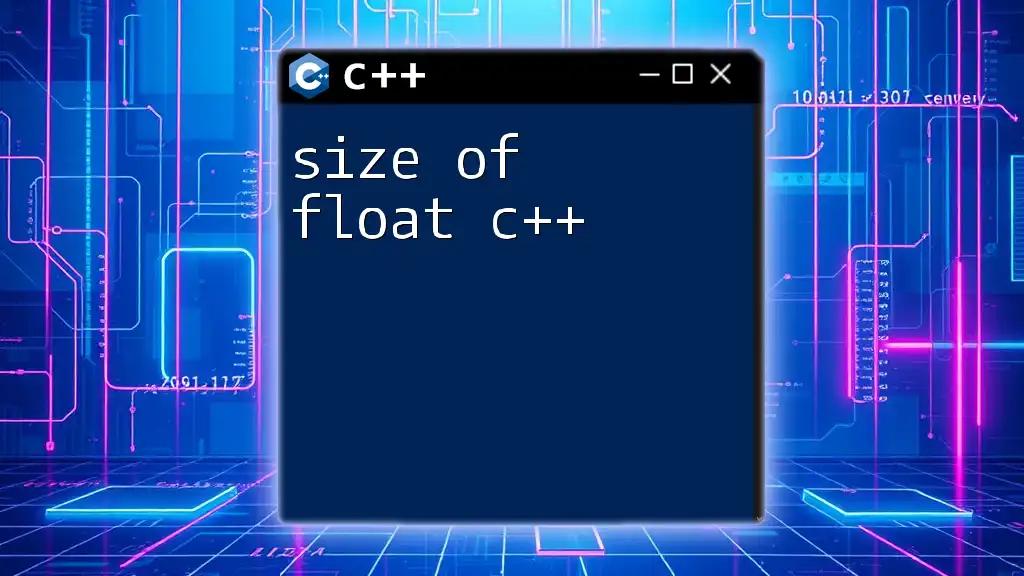
Performance Considerations
Keep in mind both time complexity and space complexity when choosing your transposition method:
- Nested Loop Method: O(n * m) time complexity and O(n * m) space complexity for non-square matrices.
- Using std::vector: Similar time complexity, but with better memory management.
- In-place Transposition: Only O(n²) time complexity but O(1) space for square matrices.
Generally, select the method that best aligns with your application’s specific requirements and constraints.
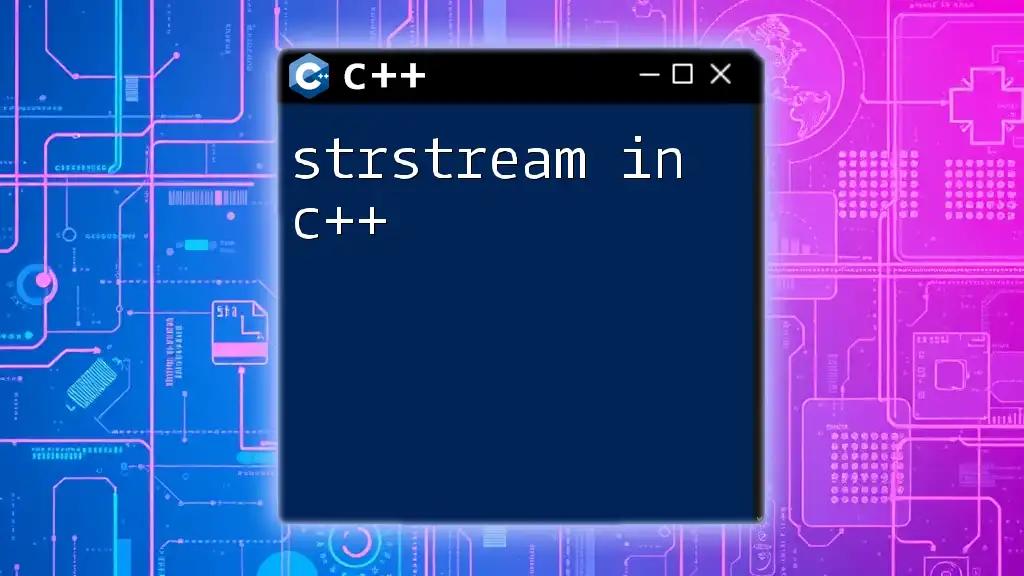
Conclusion
Understanding how to effectively transpose a matrix in C++ is essential for various programming applications. Whether maintaining tight control over memory with dynamic allocation or utilizing convenient data structures like `std::vector`, mastering these techniques will elevate your programming skills and enhance your capabilities in tasks involving matrices.
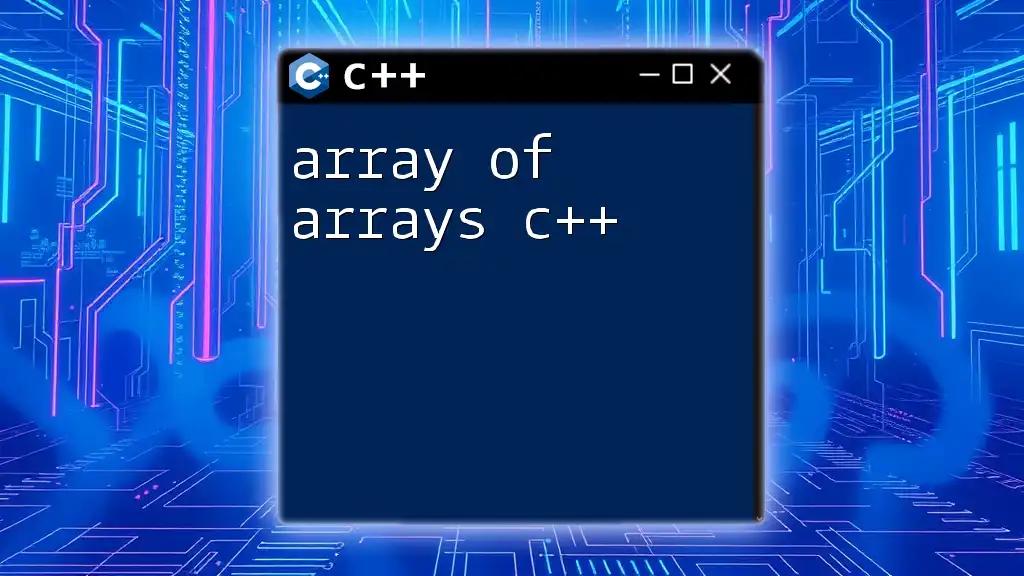
Additional Resources
For further learning on matrices and C++, consider online compilers for practicing your code snippets. Books and online tutorials on C++ programming will also provide more in-depth knowledge and help you solidify your understanding of this fundamental operation.