The prelaunch task in C/C++ allows developers to execute specific commands or scripts before building or running their code, ensuring the environment is properly configured.
Here’s a simple example of a prelaunch task that compiles a C++ file using `g++`:
g++ -o my_program my_program.cpp
Understanding Prelaunch Tasks
What is a Prelaunch Task?
A prelaunch task is an automated command or series of commands that runs before launching or debugging an application in development environments like those used for C/C++ development. These tasks are crucial for preparing the environment, building the application, and ensuring everything is set up correctly and runs smoothly when execution begins. Prelaunch tasks help streamline the development process, allowing developers to focus on coding rather than environment configuration each time.
The Role of Prelaunch Tasks in Development
The role of prelaunch tasks is to enhance the efficiency and reliability of the development workflow. Here’s how they contribute:
- Error Detection: They help catch syntax or compilation errors early in the process. This saves time by preventing runtime issues that could arise later when the application is executed.
- Code Consistency: By automating the build process, prelaunch tasks ensure that all developers are working with the same version of the application, maintaining consistency across different environments.
- Development Speed: Automating routine tasks speeds up the overall development process. Developers can set up and forget these tasks, leading to increased productivity.
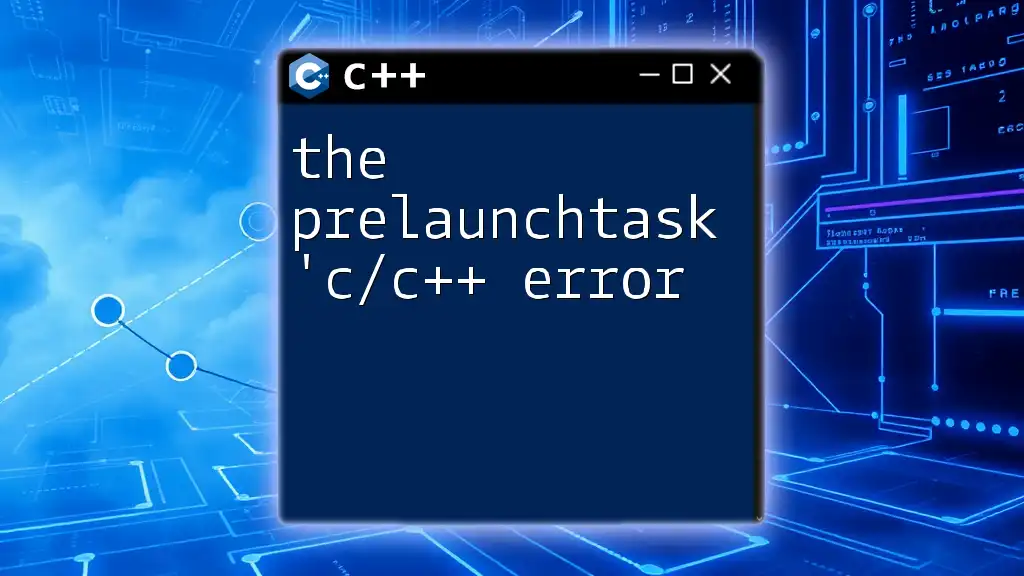
Setting Up Prelaunch Tasks in C/C++
Configuring Your Development Environment
To leverage prelaunch tasks in C/C++, start by configuring your development environment. Popular IDEs such as Visual Studio and Code::Blocks offer built-in capabilities for prelaunch tasks.
- Visual Studio: Navigate to the project properties to configure prelaunch tasks under the tasks tab and define the build process.
- Code::Blocks: Use the project settings to define scripts or commands that run prior to launching.
Sample Prelaunch Command for C/C++
To illustrate, a common command for compiling and executing a C++ program using the GNU Compiler Collection (g++) might look like this:
g++ -o myProgram myProgram.cpp && ./myProgram
This command accomplishes two key tasks:
- It compiles the `myProgram.cpp` source file into an executable named `myProgram`.
- It executes the compiled program immediately after successful compilation.
Understanding this command's components is essential, including options like `-o`, which specifies the output file, and `&&`, which runs the next command only if the preceding command is successful.
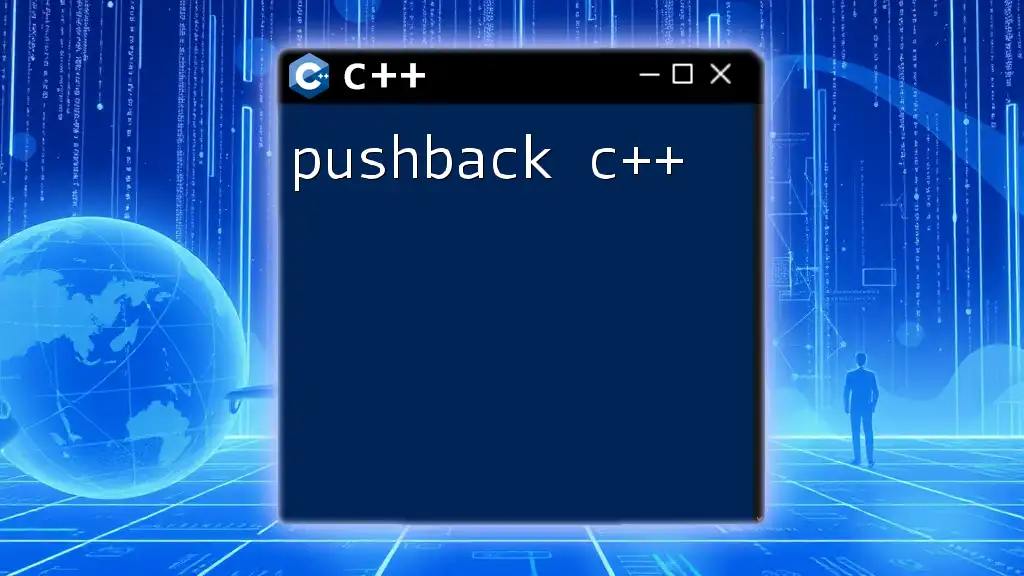
Creating and Managing Prelaunch Tasks
Creating a Simple Prelaunch Task
To create a prelaunch task, you might define it in your project's configuration file. A simple JSON configuration might look like this:
{
"version": "2.0.0",
"preLaunchTask": "build"
}
In this snippet:
- `version` indicates the configuration schema version.
- `preLaunchTask` specifies which task will be executed before the program runs.
Managing Multiple Prelaunch Tasks
For more complex projects, managing multiple prelaunch tasks becomes necessary. A sample setup could include commands for compiling, static analysis, and even running tests:
{
"tasks": [
{
"label": "build",
"type": "shell",
"command": "g++",
"args": ["-o", "myProgram", "myProgram.cpp"]
},
{
"label": "run",
"type": "shell",
"command": "./myProgram"
}
]
}
In this configuration:
- The `build` task compiles the application.
- The `run` task executes the compiled application.
This organization allows developers to streamline their workflow by switching between tasks as needed.
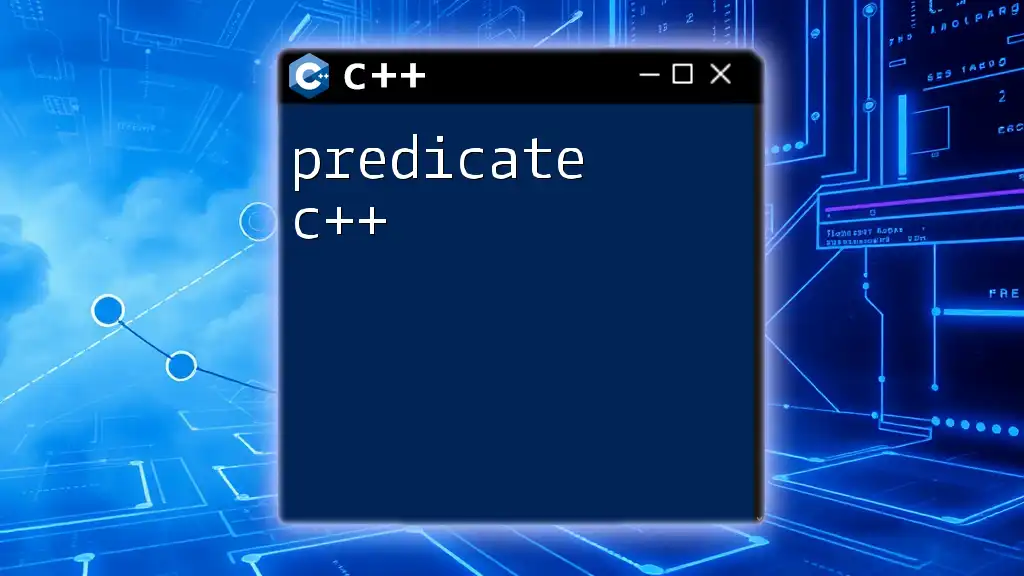
Understanding the Build Process
Common Build Commands in C++
In C/C++ development, a few commands are essential for the build process. The `g++` command is frequently used for compiling C++ files, while `make` is invaluable for managing larger projects through Makefiles. Best practices in using these commands include:
- Keeping build commands simple and understandable.
- Using comments within Makefiles to document commands for future reference.
Error Handling in Prelaunch Tasks
While working with prelaunch tasks, it's important to anticipate and handle errors. Common pitfalls include missing dependencies or incorrect compiler flags. Using a C++ `try-catch` block is a good example of how to manage runtime exceptions:
try {
// Your code goes here
} catch (const std::exception &e) {
std::cerr << "Error: " << e.what() << std::endl;
}
This approach allows your program to react gracefully to exceptions, enhancing both user experience and debugging efficiency.
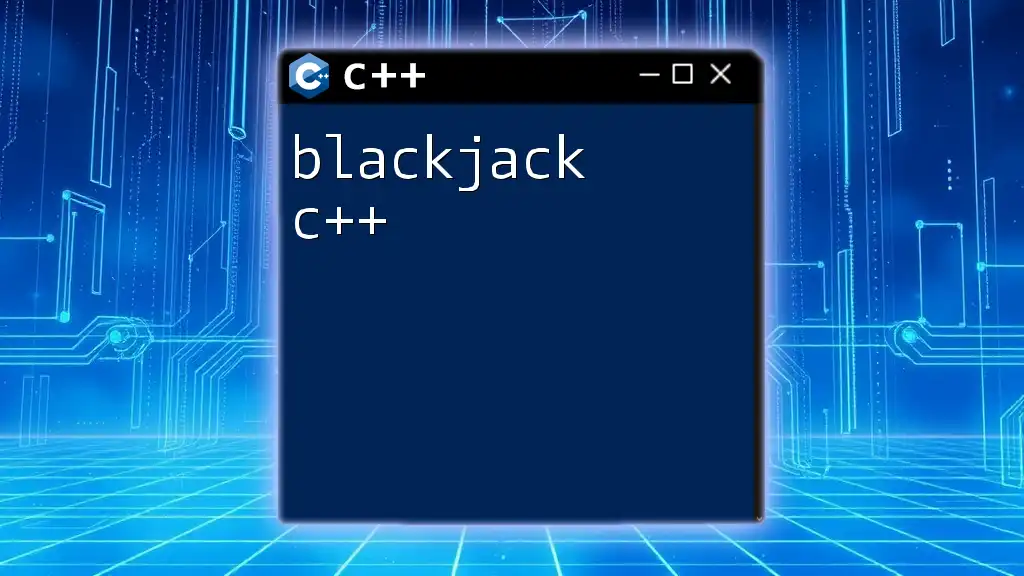
Advanced Prelaunch Task Techniques
Customizing Prelaunch Command Parameters
For more nuanced control, customizing command parameters can significantly enhance the functionality of your prelaunch tasks. For instance, specifying different compiler flags for debugging versus release builds may be necessary:
{
"args": ["-DDEBUG=1"]
}
This command ensures your program is compiled with debug information when needed, aiding in the troubleshooting process.
Integrating with Version Control Systems
Incorporating prelaunch tasks into a version control workflow can optimize your development process. For instance, setting up a pre-launch task to run tests each time you pull changes from a repository can help catch integration issues early. This can be configured as part of your Continuous Integration (CI) setup, which assists in maintaining software quality over time.
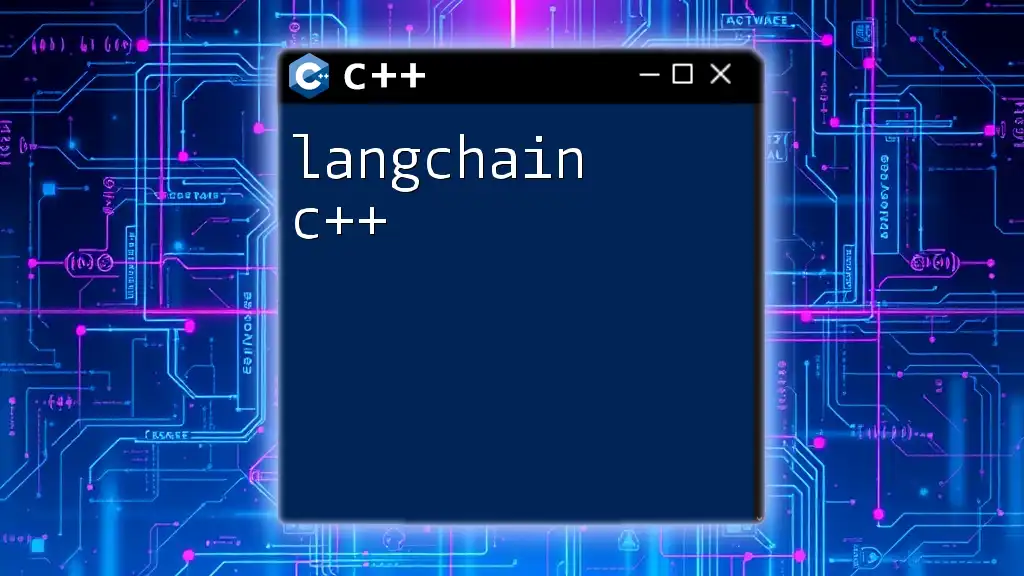
Testing and Debugging Prelaunch Tasks
Importance of Testing Prelaunch Tasks
Regular testing of your prelaunch tasks is imperative. Ensuring they function as expected helps avoid problems before execution. Utilizing tools such as CMake can facilitate the build testing process, ensuring that any configuration changes are promptly validated.
Debugging Prelaunch Tasks
Should a prelaunch task fail, understanding debugging techniques will be essential to resolve issues. Check task outputs for error messages, refine commands, and use logging to pinpoint failures. Logging can provide crucial insight into what went wrong during execution, allowing developers to rectify issues efficiently.
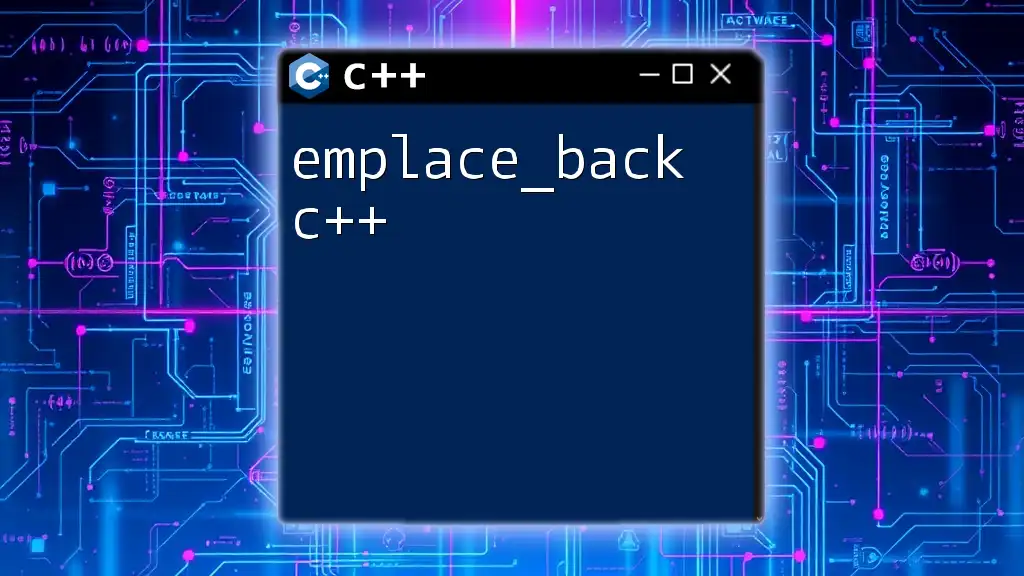
Conclusion
Prelaunch tasks are an essential component of the C/C++ development workflow that greatly enhances efficiency, reliability, and productivity. By adopting and optimizing these tasks within your development process, you can ensure a smoother, more consistent coding environment. We encourage you to explore prelaunch task configurations in your projects. This proactive approach to development will not only streamline your coding practice but also foster better quality software.
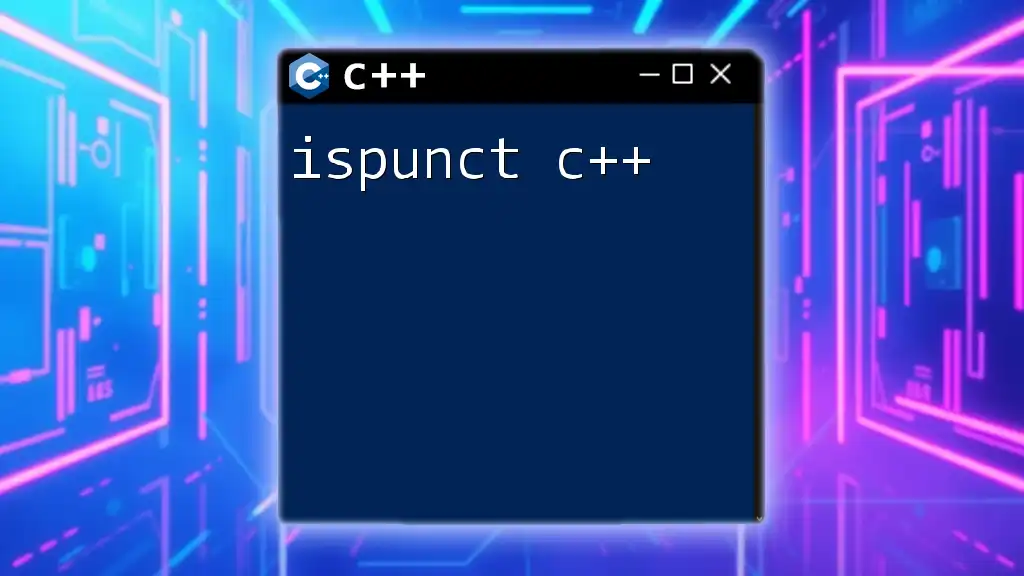
Call to Action
Stay updated with upcoming classes and workshops that focus on mastering C/C++ commands and enhancing your development skills. Subscribe for more insightful articles and tips on optimizing your coding experience.