The preLaunchTask error in C/C++ usually indicates that your build process is not correctly defined in your project settings, often due to a missing or misconfigured task in the tasks.json file.
{
"version": "2.0.0",
"tasks": [
{
"label": "build",
"type": "shell",
"command": "g++",
"args": [
"-g",
"main.cpp",
"-o",
"main"
],
"group": {
"kind": "build",
"isDefault": true
}
}
]
}
Understanding PreLaunchTasks in C/C++
What are PreLaunchTasks?
PreLaunchTasks are scripts or commands defined in the task runner of your development environment, typically employed to establish an environment or perform preparatory operations prior to launching your application. They play a crucial role in streamlining the software build process, helping developers save time, ensure code quality, and maintain efficiency.
Importance of PreLaunchTasks
PreLaunchTasks are indispensable for enhancing productivity in C/C++ development. By automating routine tasks, they allow developers to focus on writing code rather than managing setup issues. PreLaunchTasks also ensure that essential scripts such as compiler commands, preprocessors, and valgrind running requirements are handled automatically, leading to smoother workflows and ultimately better code.
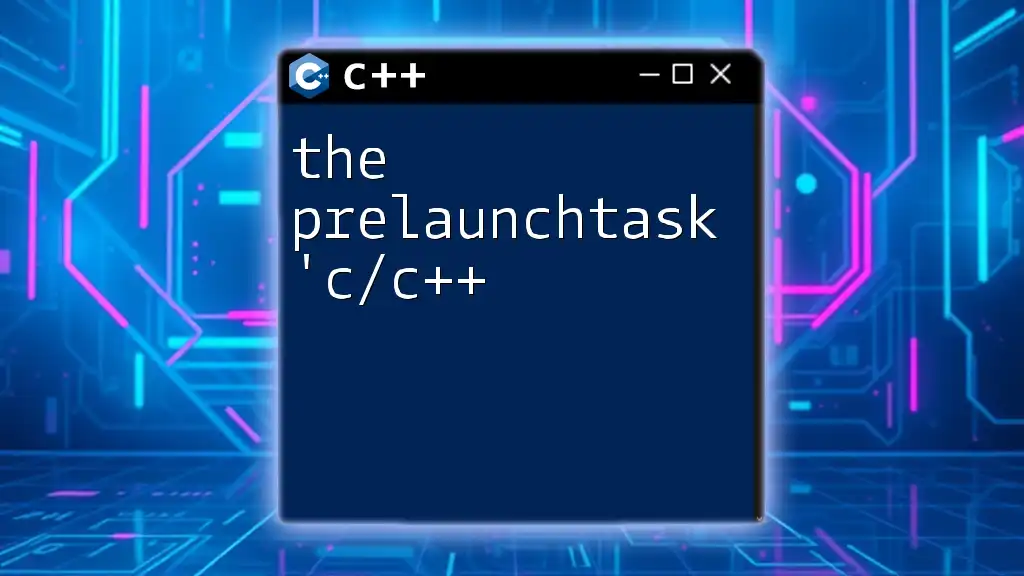
Common C/C++ PreLaunchTask Errors
What is the 'C/C++ Error'?
The preLaunchTask 'C/C++ error' refers to various error states that can occur when attempting to execute tasks associated with C/C++ programs, particularly during the build phase in your integrated development environment (IDE). This could stem from a variety of issues, including incorrect configurations or missing dependencies.
Why Does the 'C/C++ Error' Occur?
This error typically arises due to:
- Misconfiguration in the task settings: If your `tasks.json` is incorrectly set up, the IDE may struggle to find or execute the necessary commands.
- Compiler issues: An incorrect or outdated compiler can trigger errors when it is unable to compile your code correctly.
- Missing dependencies: Your project might rely on libraries or files that are not available in the specified locations, leading to compile-time failures.
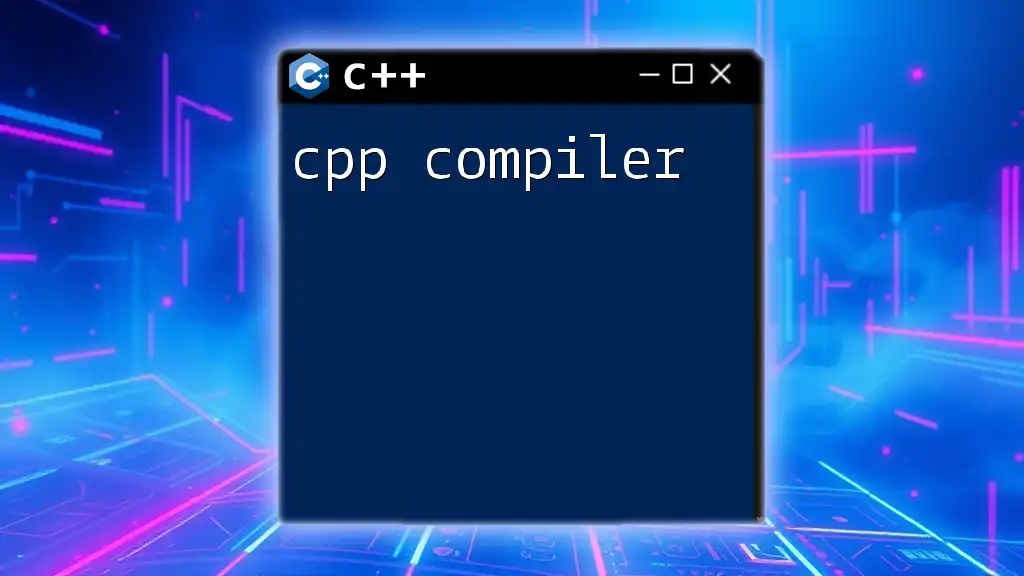
Identifying the 'C/C++ Error'
Recognizing Error Messages
When facing the preLaunchTask 'C/C++ error,' various error messages may appear that can help you diagnose the problem. Some common examples include:
- "task 'build' failed with exit code 1": This signifies that a command in your task did not execute successfully.
- "Cannot find file": This indicates that the compiler or IDE is unable to locate the source file you are trying to compile.
Understanding and accurately interpreting these error messages is vital for troubleshooting effectively.
Troubleshooting Steps
When you encounter the 'C/C++ error', follow these steps to identify the underlying issue:
- Check console output: Review the terminal or output console for specific error messages related to your preLaunchTask.
- Verify file paths and names: Ensure that all paths defined in your configuration files are correct and that no typos exist in your file names or extensions.
- Review your configuration settings: Ensure your `tasks.json` and `settings.json` files are set up correctly.
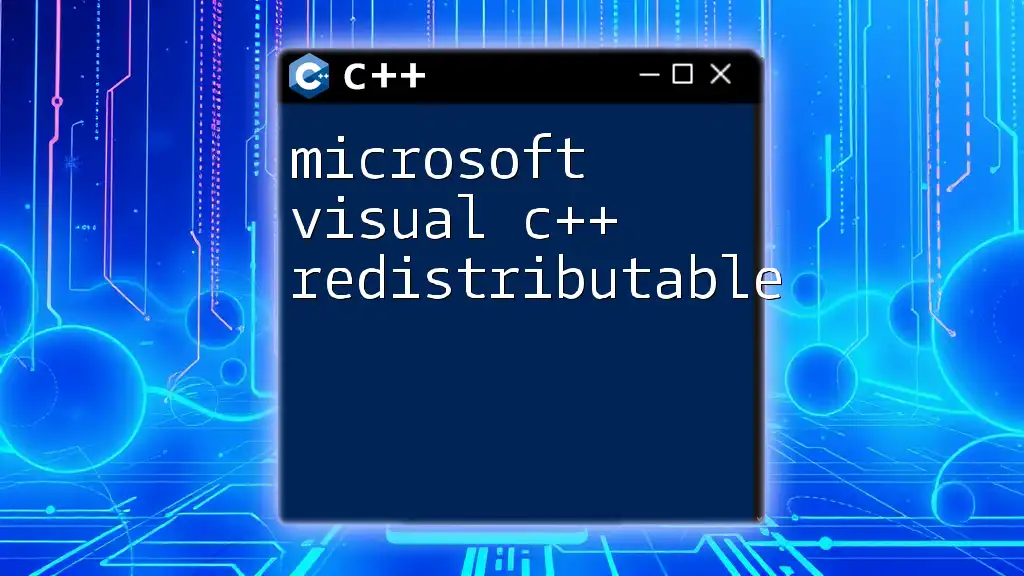
Configuring PreLaunchTasks to Prevent Errors
Setting Up Your Build Environment
To prevent the preLaunchTask 'C/C++ error', it is essential to establish a correct build environment right from the start. A basic configuration in the `tasks.json` file may look like this:
{
"version": "2.0.0",
"tasks": [
{
"label": "build",
"type": "shell",
"command": "g++",
"args": [
"-Wall",
"your_file.cpp",
"-o",
"your_file"
],
"group": {
"kind": "build",
"isDefault": true
},
"problemMatcher": ["$gcc"]
}
]
}
In the example above, the build command uses `g++`, along with flags such as `-Wall` to display all warnings, and specifies the output file name. Understanding how each parameter works is crucial for a successful configuration.
Defining Error Handling in PreLaunchTasks
Implementing error handling mechanisms within your PreLaunchTasks can help mitigate errors. You can incorporate scripts that check for the success of each command and provide fallback options or error messages accordingly. For example:
#!/bin/bash
g++ your_file.cpp -o your_file
if [ $? -ne 0 ]; then
echo "Compilation failed. Please check your code."
else
echo "Compilation successful!"
fi
In this bash script, the `$?` variable checks the exit status of the last executed command (`g++`). If the compilation fails, a user-friendly message is displayed.
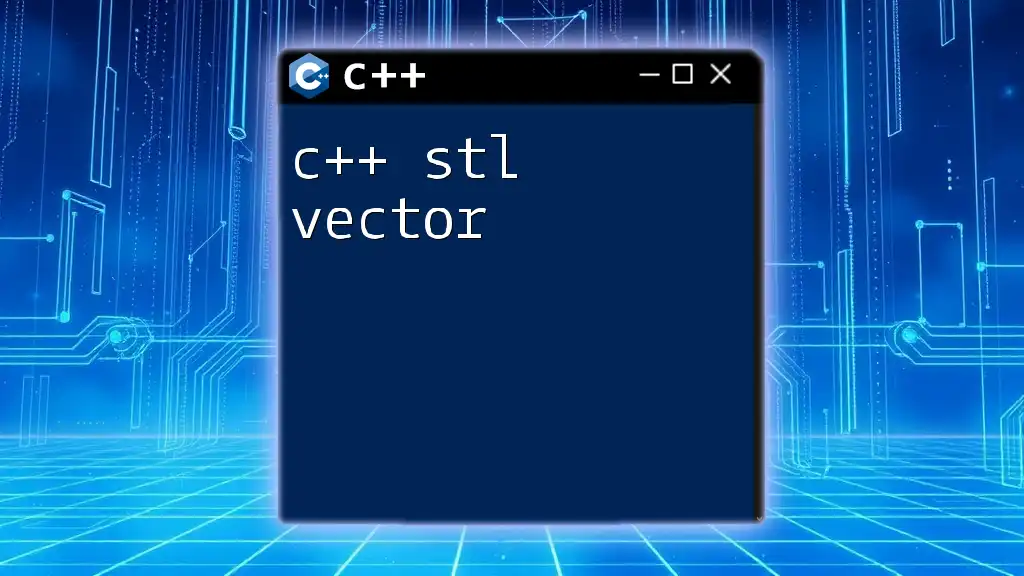
Fixing the 'C/C++ Error'
Practical Solutions to Resolve the Error
Fixes for the preLaunchTask 'C/C++ error' typically involve adjusting your configurations:
- Correct configuration issues: Double-check the syntax and structure of your `tasks.json` and `settings.json` files to ensure all paths and commands are correctly specified.
- Adjusting environment paths: Sometimes, the IDE may not find the compiler or necessary libraries due to incorrect paths. Make sure that your environment variables include the necessary routes that lead to compilers and include directories.
For example, you may need to configure `settings.json` to include paths like this:
{
"C_CPP.default.includePath": [
"${workspaceFolder}/**",
"/usr/include/c++/9",
"/usr/local/include"
],
"C_CPP.default.defines": [
"_DEBUG",
"UNICODE"
]
}
The above snippet defines include paths for standard libraries which might resolve common compilation errors.
Verifying Your Changes
After implementing changes, always verify that your modifications resolve the error:
- Build your project again: Re-run the task to confirm that the error no longer appears.
- Check the console output: Look for any new error messages or warnings that need to be addressed.
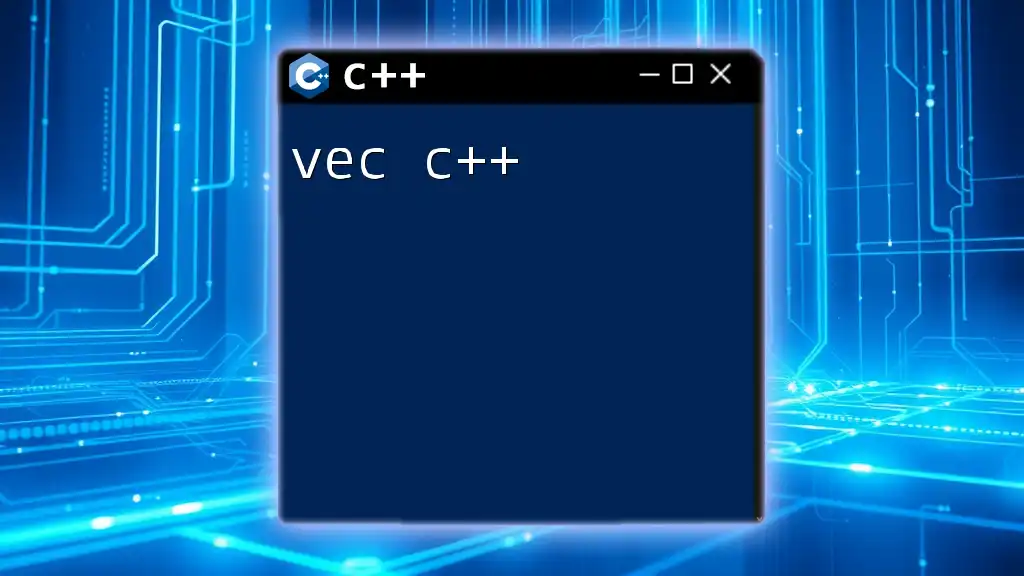
Advanced Troubleshooting Techniques
Utilizing Debugging Tools
Debugging tools like `gdb` or built-in IDE debuggers are essential for identifying deeper issues. For instance, you can run:
gdb ./your_file
This command allows you to step through the running process, inspect variable values, and identify problematic code sections.
Seeking Help and Documentation
When persistent error messages surface, don't hesitate to seek help. Utilize online forums, documentation, and communities like Stack Overflow or GitHub discussions to find solutions. Share your exact error messages and steps already taken to receive more targeted assistance.
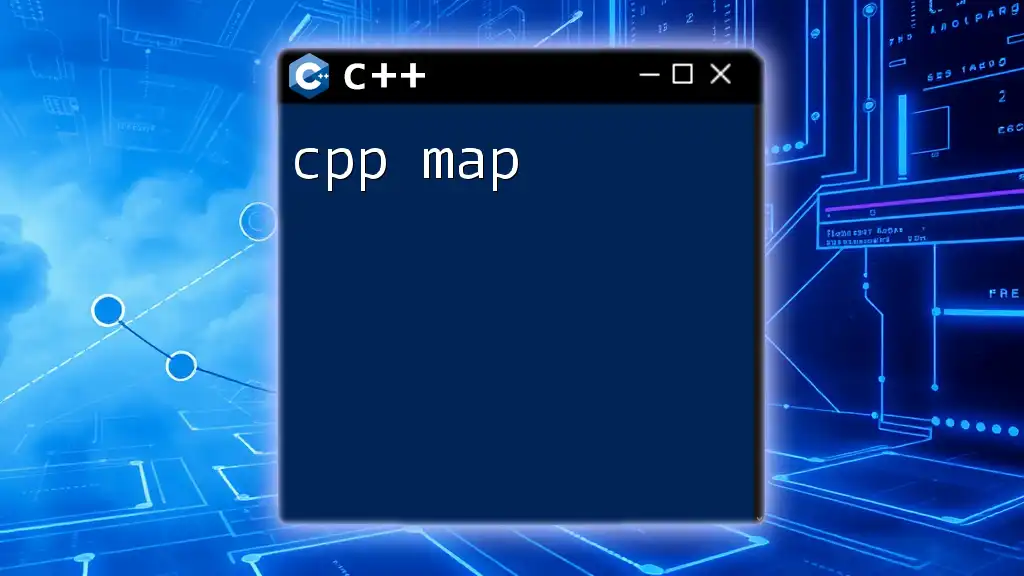
Best Practices for Avoiding PreLaunchTask Errors
Consistent Development Environment
Maintaining a uniform development environment across team members is critical. Utilize containerization tools like Docker to ensure that everyone works within the same setup, avoiding discrepancies in configurations and dependencies.
Regular Updates and Maintenance
Keep your compilers, IDEs, and libraries updated to the latest versions. Doing so ensures that you benefit from bug fixes and improvements, reducing the chances of encountering errors related to outdated tools.
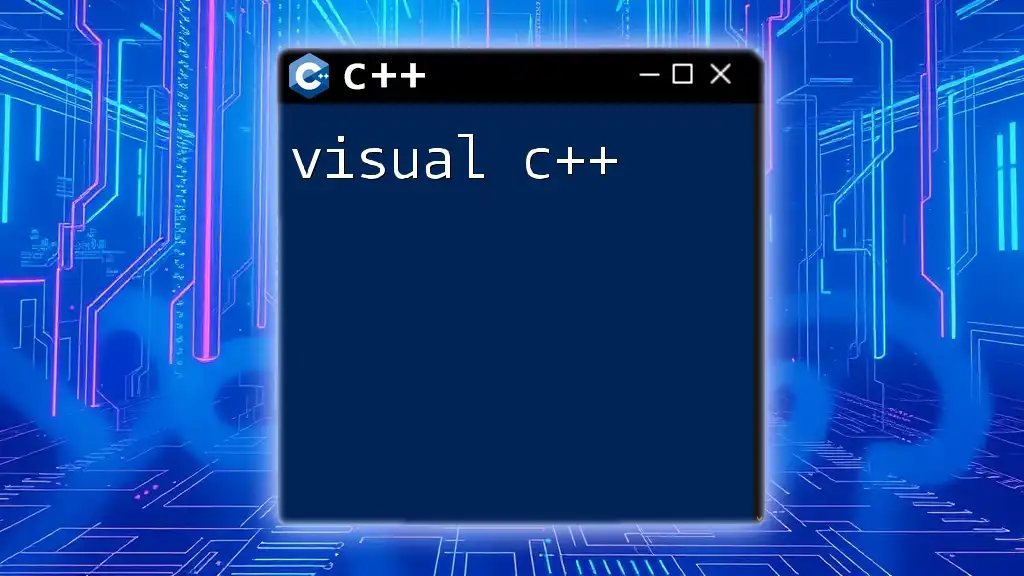
Conclusion
Addressing the preLaunchTask 'C/C++ error' can seem daunting, but equipping yourself with the right knowledge and strategies can ease the process. By understanding potential causes, implementing effective configurations, and following best practices, you can effectively minimize the occurrence of such errors. Build a proactive approach to development, and remember that challenges are merely opportunities for learning and improvement in the realm of C/C++.
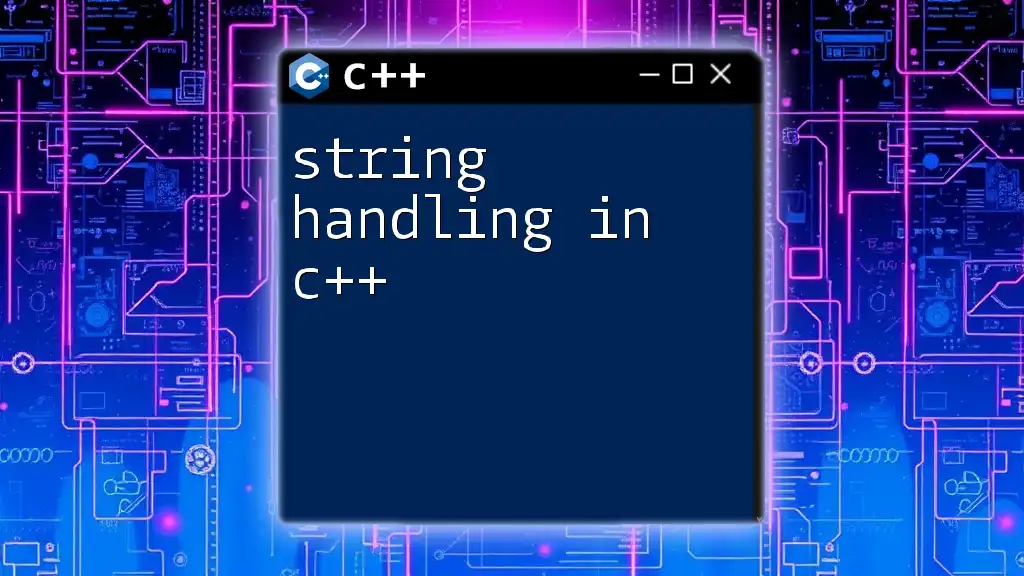
Additional Resources
References
Look into comprehensive resources such as official documentation or community forums for further reading on tackling the preLaunchTask 'C/C++ error'.
Call to Action
We encourage you to share your experiences with the preLaunchTask 'C/C++ error' in the comments section below. Your insights may help fellow developers navigate their own challenges! Subscribe for more tips and advice on mastering C/C++ commands and development best practices.