You can access elements of a string in C++ using the indexing operator `[]` or the `at()` method, which allows you to retrieve a character at a specific position within the string.
Here’s a code snippet illustrating both methods:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, C++!";
// Accessing using the indexing operator
char firstChar = str[0];
std::cout << "First character: " << firstChar << std::endl;
// Accessing using the at() method
char secondChar = str.at(1);
std::cout << "Second character: " << secondChar << std::endl;
return 0;
}
Understanding Strings in C++
What is a String?
In C++, a string is a sequence of characters that can represent text data. There are two primary types of strings you’ll encounter: C-style strings and C++ strings.
-
C-style strings are arrays of characters terminated by a null character (`'\0'`). They can be less convenient to work with because they require manual management of memory and size.
const char* c_string = "Hello, World!"; // C-style string
-
C++ strings, on the other hand, are objects of the `std::string` class. They offer a multitude of built-in functions, making string manipulation simpler and safer.
std::string cpp_string = "Hello, World!"; // C++ string
Why Use C++ Strings?
C++ strings provide enhanced safety and functionality. They:
- Self-manage memory and size.
- Support various built-in operations like concatenation, substring extraction, and comparison.
Using C++ strings reduces the risk of common programming errors such as buffer overflows, thus promoting safer coding practices.
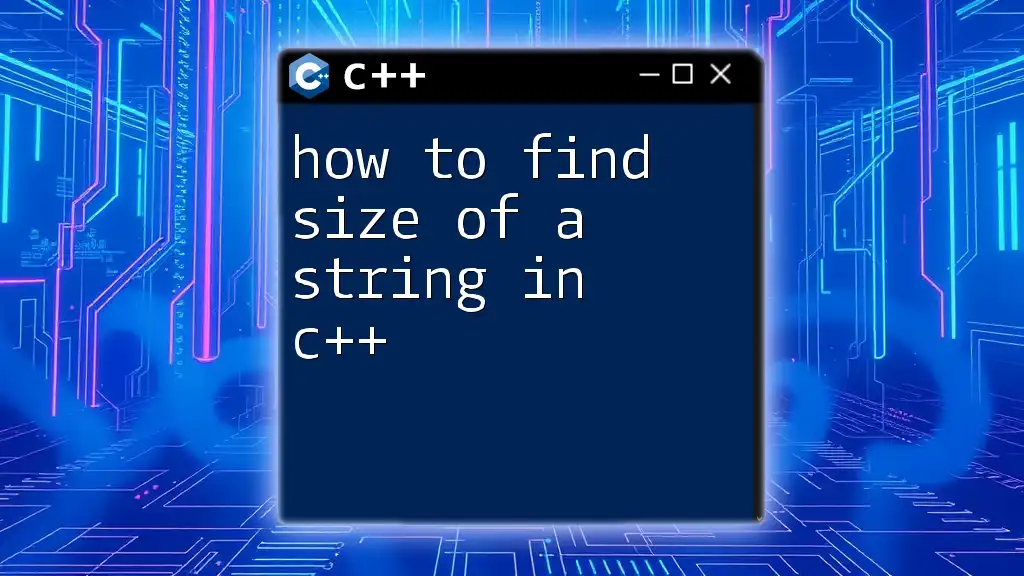
Accessing Elements of a C++ String
Using the Subscript Operator
One of the easiest ways to access the elements of a string is by using the subscript operator `[]`. This allows you to access characters by their index:
char character = cpp_string[index];
Example of accessing characters:
std::string cpp_string = "Hello";
char first_char = cpp_string[0]; // 'H'
char last_char = cpp_string[4]; // 'o'
Using the at() Method
An alternative to the subscript operator is the `at()` method. This method provides bounds-checking, throwing an exception if the index is out of range:
char character = cpp_string.at(index);
Example of using the `at()` method:
std::string cpp_string = "Hello";
char second_char = cpp_string.at(1); // 'e'
Difference Between `[]` and `at()`
While both methods allow you to access string elements, they handle errors differently. The subscript operator can lead to undefined behavior if you attempt to access an index that is out of bounds:
std::string cpp_string = "Hello";
// Out of bounds access using []
char error_char = cpp_string[10]; // Undefined Behavior (no exception)
Conversely, using `at()` will throw a `std::out_of_range` exception, allowing for safer code:
std::string cpp_string = "Hello";
// Out of bounds access using at()
char exception_char = cpp_string.at(10); // Throws std::out_of_range exception
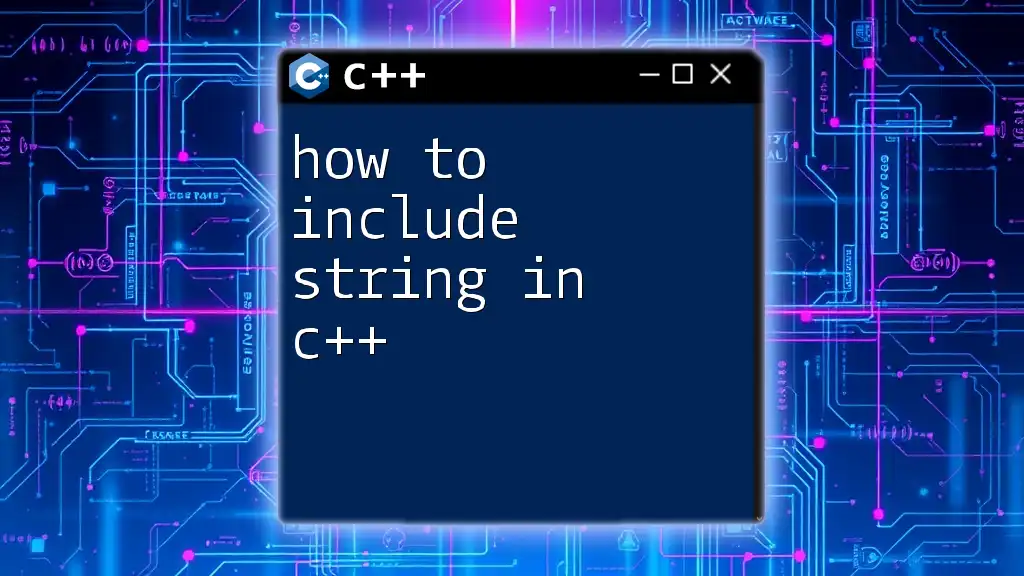
Iterating Through Strings
Using a Loop
To access each character in a string, you can use a traditional loop. Here’s an example illustrating how to iterate through a string:
std::string cpp_string = "Hello";
for (size_t i = 0; i < cpp_string.length(); ++i) {
std::cout << cpp_string[i] << " "; // Prints each character
}
Using Range-Based For Loop
C++11 introduced range-based for loops, simplifying the syntax for iterating through strings. Here’s how you can use it:
std::string cpp_string = "Hello";
for (char ch : cpp_string) {
std::cout << ch << " "; // Prints each character
}
Using Iterators
Another approach to iterate through a string is by using iterators. This method provides flexibility in handling string data:
std::string cpp_string = "Hello";
for (std::string::iterator it = cpp_string.begin(); it != cpp_string.end(); ++it) {
std::cout << *it << " "; // Prints each character
}
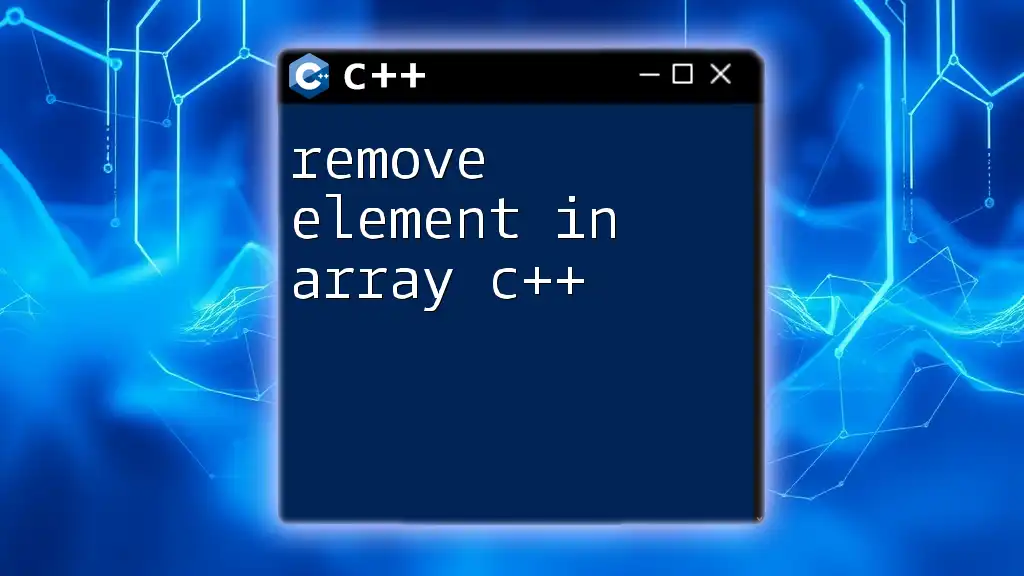
Modifying Strings
Changing Elements
C++ strings allow you to modify individual characters. You can easily change a character at a specific index:
std::string cpp_string = "Hello";
cpp_string[0] = 'h'; // Changes 'H' to 'h'
Inserting Characters
You can also insert characters into a string at a specific position using the `insert()` method:
std::string cpp_string = "Hello";
cpp_string.insert(5, " World"); // Inserts " World" at index 5
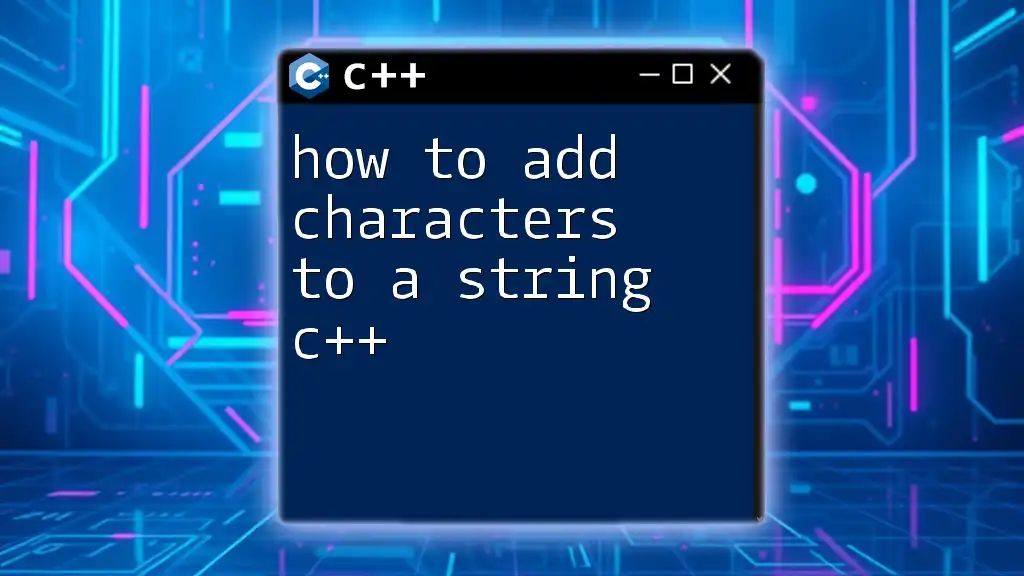
Best Practices
Choosing Between `[]` and `at()`
When working with strings, it’s essential to choose the right access method. Use the subscript operator when you are confident about index bounds and performance is critical. On the other hand, opt for `at()` if safety is a priority, as it provides built-in bounds checking.
Handling Out of Bounds
Regardless of the method you choose, always make sure to validate that your indexes are within the valid range of the string. Avoiding out-of-bounds access is crucial for writing robust code.
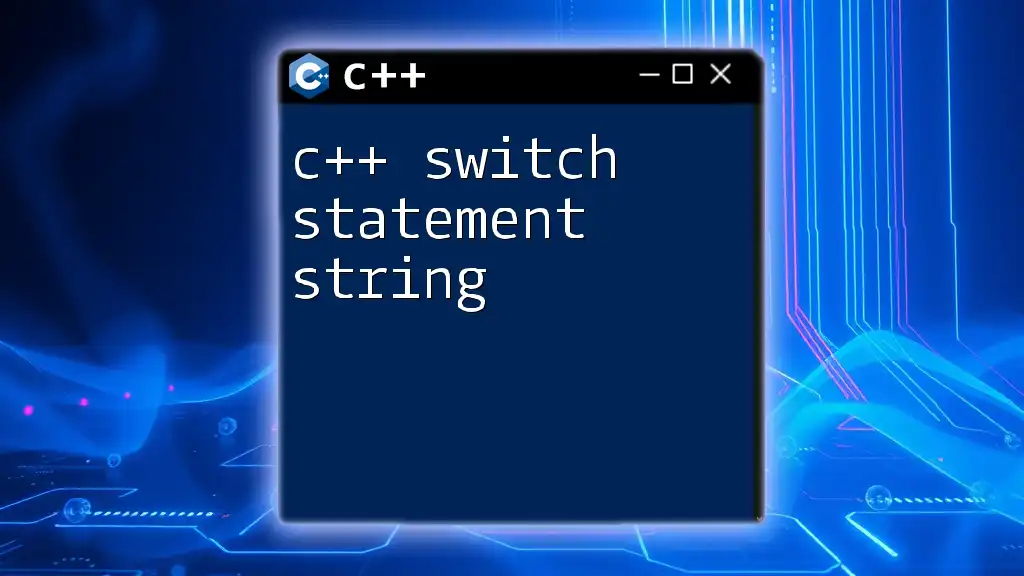
Conclusion
Knowing how to access elements of a string in C++ is fundamental for string manipulation. Understanding the differences between access methods and iterating effectively paves the way for better programming practices. As you continue to practice these techniques, you will gain confidence in managing string data efficiently.
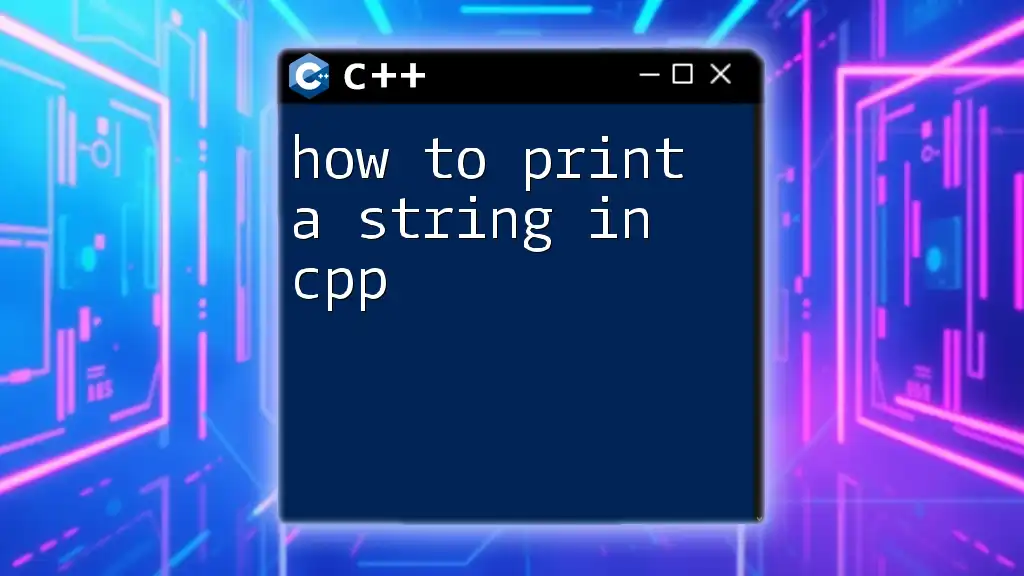
Call to Action
Feel free to share your experiences, ask questions, or leave comments below! If you found this guide helpful, consider subscribing for more concise C++ tutorials that simplify complex topics.
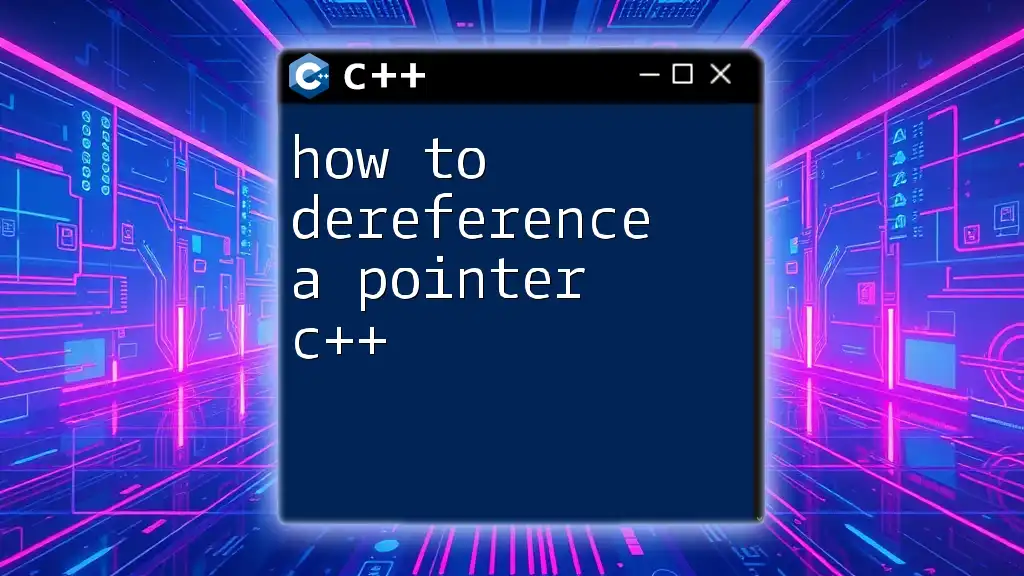
Additional Resources
For further learning, check out the official C++ documentation, or explore recommended books and online courses that delve deeper into string manipulation in C++.