To access a member function of a class in C++, you create an object of that class and use the dot operator to call the function. Here's a code snippet that demonstrates this:
#include <iostream>
class MyClass {
public:
void display() {
std::cout << "Hello, World!" << std::endl;
}
};
int main() {
MyClass obj; // Create an object of MyClass
obj.display(); // Access the member function using the dot operator
return 0;
}
Understanding Classes and Member Functions
What is a Class in C++?
In C++, a class is a user-defined data type that represents a blueprint for creating objects. It encapsulates data and functions that operate on that data in a single entity. Classes are the foundation of object-oriented programming in C++.
A class typically consists of:
- Attributes (or data members): These are variables that hold the object's state.
- Member functions: These are functions defined within the class that operate on the class's data.
Here’s an example of a simple class declaration:
class Car {
public:
void startEngine();
void stopEngine();
};
What are Member Functions?
Member functions are functions that are defined inside a class and have access to the data members of the class. They can manipulate or retrieve the object's data.
Member functions can be categorized as:
- Public: Accessible from outside the class.
- Private: Accessible only from within the class.
- Protected: Accessible in derived classes.
Understanding these distinctions is vital for controlling access and maintaining encapsulation in your code.
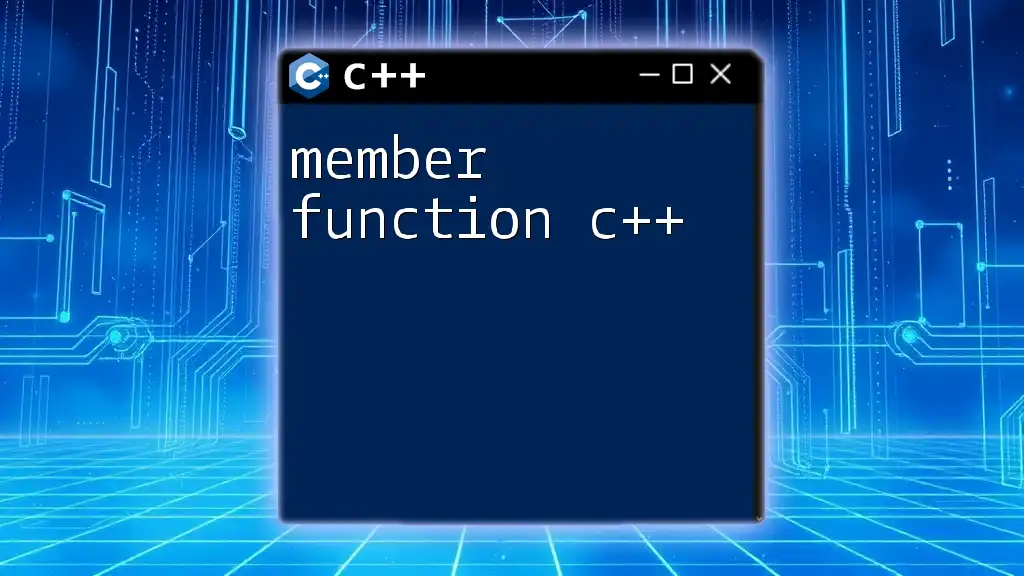
Accessing Member Functions
Creating an Object of a Class
To access member functions, you first need to create an object of the class. Object instantiation involves defining an object based on the class blueprint. The access specifiers determine the accessibility of the members.
For instance:
Car myCar; // Creating an object of the Car class
This line creates a new object named `myCar` which now has access to the public member functions defined in the `Car` class.
Using the Dot Operator
In C++, the dot operator (`.`) is the primary method used to access member functions. To call a member function using the dot operator, you simply use the object name, followed by the dot, and then the function name.
Here’s an example of how to call a member function using this operator:
myCar.startEngine(); // Calls the startEngine function
In this case, when `startEngine()` is invoked, it executes whatever functionality has been defined within that member function.
Using Pointers to Access Member Functions
Pointer Declaration
In addition to using objects directly, you can also access member functions using pointers. A pointer is a variable that stores the memory address of another variable, giving you the flexibility to dynamically manage memory.
You can declare a pointer to a class object like this:
Car* ptrCar = &myCar; // Pointer to myCar object
Calling Member Functions through Pointers
To call a member function using pointers, you’ll use the arrow operator (`->`). This operator dereferences the pointer to access the object's member functions.
Here’s how you can call a member function through a pointer:
ptrCar->stopEngine(); // Calls the stopEngine function via pointer
Using pointers can be especially useful in scenarios like dynamic memory allocation, where you might not know the number of objects at compile time.
Using References to Access Member Functions
Reference Declaration
Another effective way to access member functions is through references. A reference is an alias for another variable. Unlike pointers, they cannot be null and must be initialized when declared.
You can declare a reference to a class object as follows:
Car& refCar = myCar; // Reference to myCar object
Calling Member Functions through References
When using references, you can call member functions similarly as with objects because references behave like the original object.
Here’s an example illustrating how to call a member function using a reference:
refCar.startEngine(); // Calls the startEngine function
Using references can simplify code and improve readability, as they avoid the syntactical overhead associated with pointers.
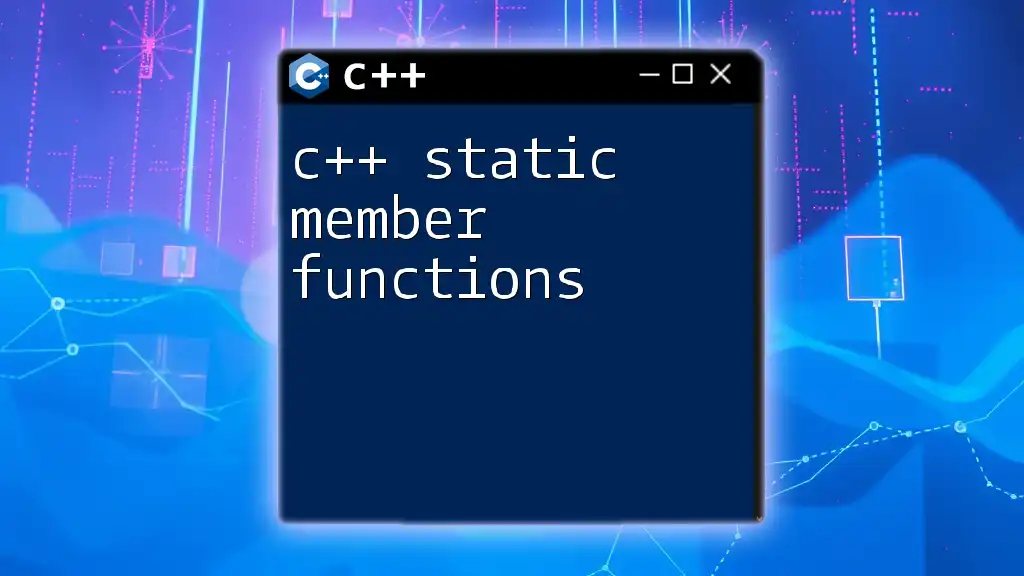
Accessing Member Functions in Inheritance
Understanding Inheritance
Inheritance is a powerful feature in C++ that allows you to create a new class based on an existing class. The new class (derived class) inherits attributes and member functions from the base class.
This mechanism enables code reuse and the creation of a hierarchical relationship between classes. Understanding how to access member functions in the context of inheritance is crucial for effective class design.
Accessing Member Functions of the Base Class
When you derive a class from a base class, you can also access the member functions of the base class. Here's an example:
class Truck : public Car {
public:
void loadCargo() {
startEngine(); // Accessing base class member function
}
};
In this example, the `Truck` class, which derives from the `Car` class, can call `startEngine()` directly because it inherits that member function.
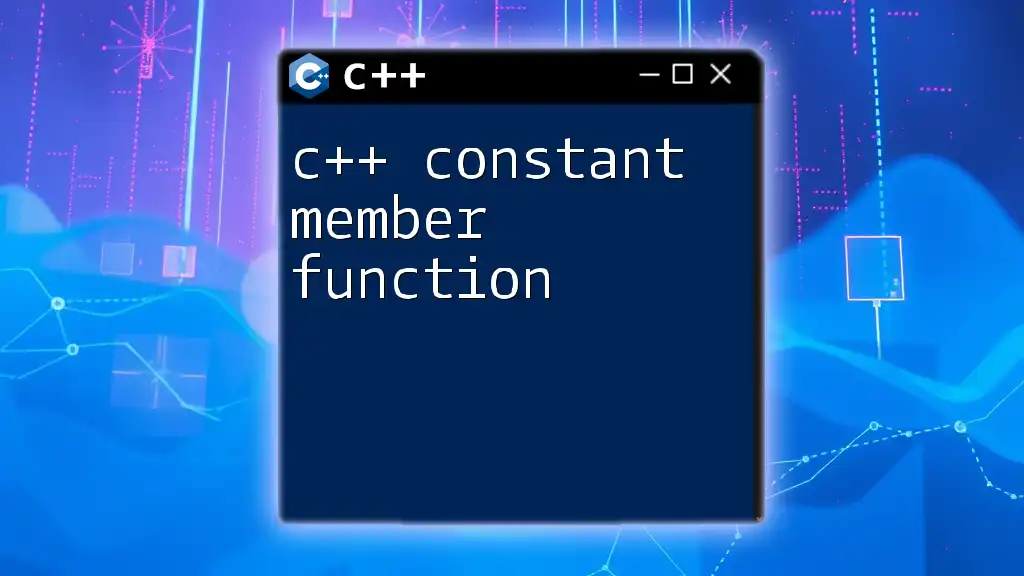
Conclusion
Accessing member functions of a class in C++ is a fundamental skill that enables you to manipulate and interact with object data. By understanding how to create objects, utilize the dot operator, employ pointers and references, and navigate class inheritance, you can build powerful and maintainable object-oriented applications.
Keep practicing these concepts, and soon you'll feel comfortable with more advanced aspects of C++ programming. Remember, the key to mastering C++ is consistent practice and exploration of its features!
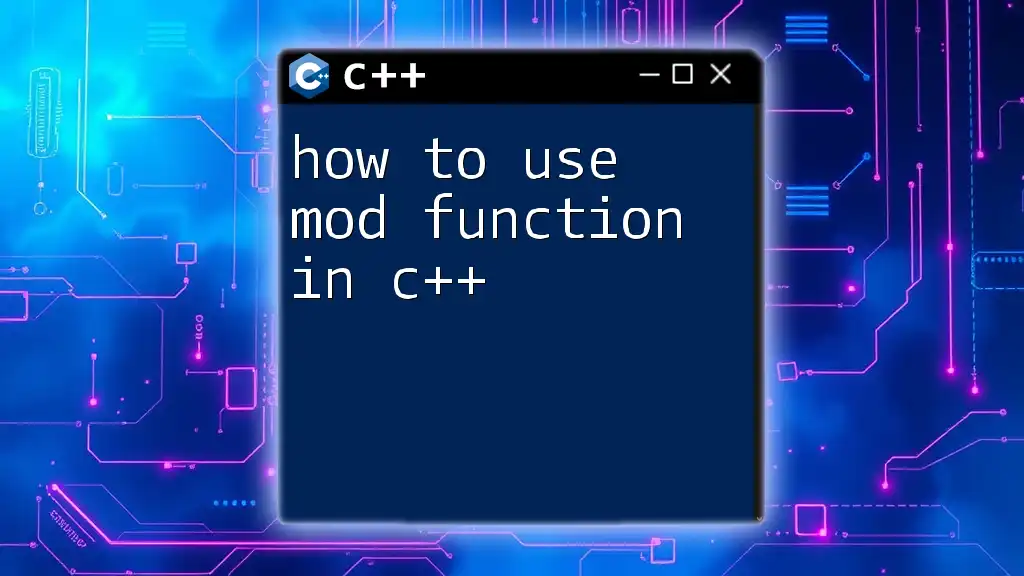
Additional Resources
To continue your learning journey, consider exploring:
- Books: "The C++ Programming Language" by Bjarne Stroustrup.
- Online tutorials: Websites like Codecademy and Coursera offer C++ courses.
- Community forums: Engage with other learners on platforms like Reddit or Stack Overflow for collaborative learning.