In C++, you can remove an element from a `std::vector` at a specific index using the `erase()` method, which shifts the subsequent elements to the left.
Here's an example code snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {10, 20, 30, 40, 50};
int indexToRemove = 2; // Index of the element to remove (30)
vec.erase(vec.begin() + indexToRemove);
for (int num : vec) {
std::cout << num << " "; // Output: 10 20 40 50
}
return 0;
}
Understanding Vectors in C++
What is a Vector?
A vector in C++ is a dynamic array that can grow or shrink in size. Unlike traditional arrays, which have a fixed size, vectors allow for flexible management of elements. This adaptability makes them a popular choice for data structures where the number of elements can vary.
Key Characteristics of Vectors
Vectors have several key characteristics that enhance their usability:
- Dynamic Size: Vectors automatically resize to accommodate new elements, eliminating the need for manual memory management.
- Memory Management: The C++ Standard Library handles memory allocation and deallocation, ensuring efficient use of resources.
- Performance Considerations: Vectors are generally more efficient than arrays for many operations, but they can incur overhead during resizing, particularly when many elements are added or removed sequentially.
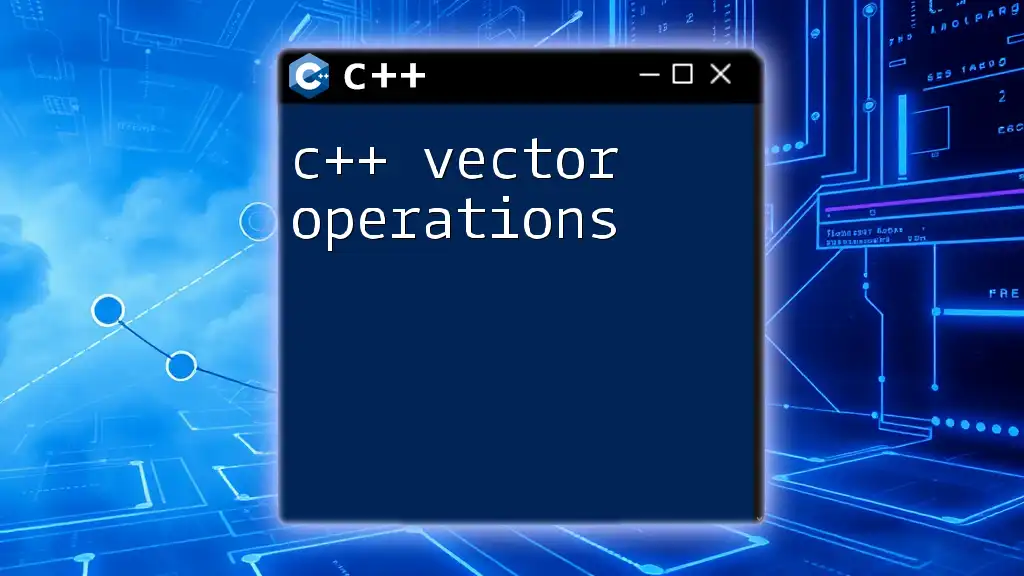
The Syntax of C++ Vectors
Basic Syntax
To use vectors, you must include the `<vector>` header and declare a vector as follows:
#include <vector>
std::vector<int> myVector = {1, 2, 3, 4, 5};
Commonly Used Methods
Vectors come with several built-in methods that facilitate various operations:
- `push_back()`: Adds a new element to the end of the vector.
- `pop_back()`: Removes the last element from the vector.
- `size()`: Returns the number of elements contained in the vector.
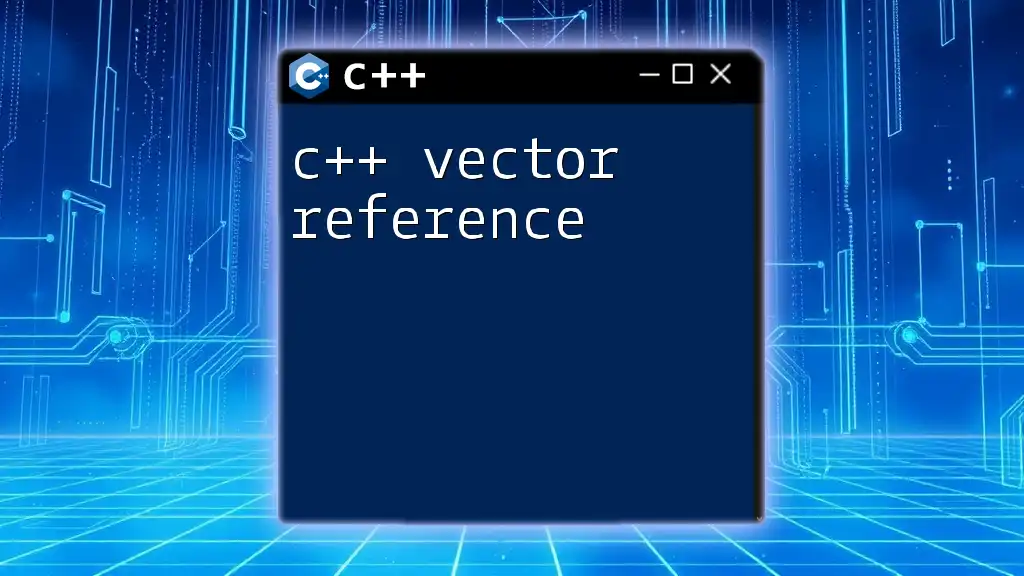
Removing Elements from a Vector
Why Remove Elements?
Removing elements from a vector can be essential for managing data effectively. Whether you're filtering out unwanted data points or simply updating your data structure, knowing how to remove elements at specific indices is crucial.
The `erase()` Method
The primary method for removing an element at a specific index is `erase()`. This method requires an iterator pointing to the element you want to remove. Here’s how it works:
myVector.erase(myVector.begin() + index);
Detailed Example of Removing at an Index
Step-by-Step Guide
Let’s walk through a complete example of removing an element from a vector at a specified index.
-
Creating and Populating a Vector: You can create and populate a vector like this:
std::vector<int> numbers = {10, 20, 30, 40, 50};
-
Displaying the Vector Before Removal: Before removing an element, you might want to display the contents of the vector:
for(int n : numbers) { std::cout << n << " "; } // Output: 10 20 30 40 50
-
Removing an Element at a Specific Index: Suppose you want to remove the element at index 2:
numbers.erase(numbers.begin() + 2);
-
Displaying the Vector After Removal: You can check the vector's content again:
for(int n : numbers) { std::cout << n << " "; } // Output: 10 20 40 50
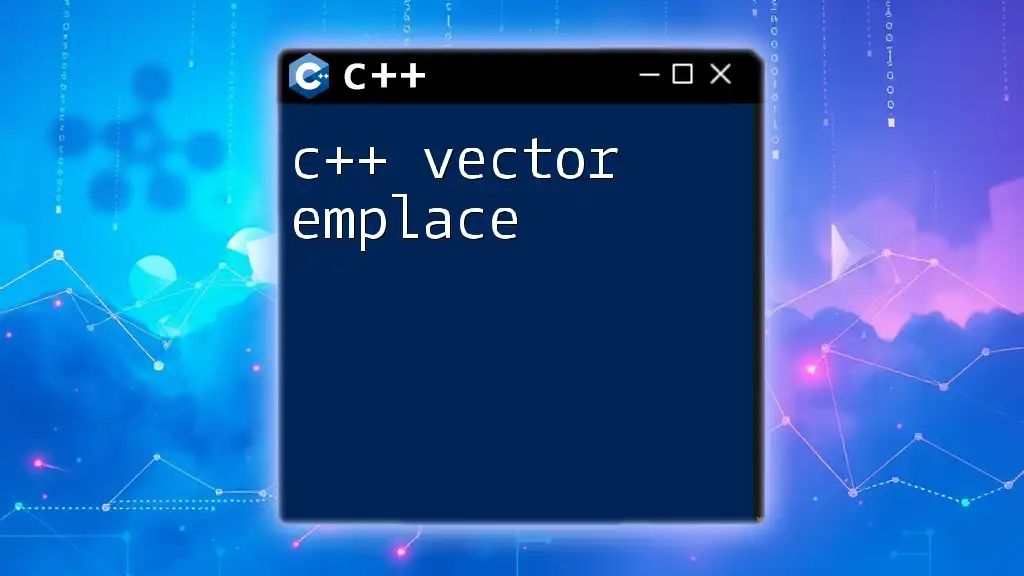
Considerations When Removing Elements
Out-of-Bounds Index
Attempting to remove an element using an invalid index (one that is outside the bounds of the vector) can lead to undefined behavior. Therefore, it is crucial to check that the index is valid before calling `erase()`. One way to do this is by ensuring that the index falls within the range of `0` to `size() - 1` of the vector.
Performance Implications
Remember that when you remove an element from a vector, the remaining elements are shifted to fill the gap, which can affect performance. This shifting operation can be expensive for large vectors, especially if the removed element is located near the front.
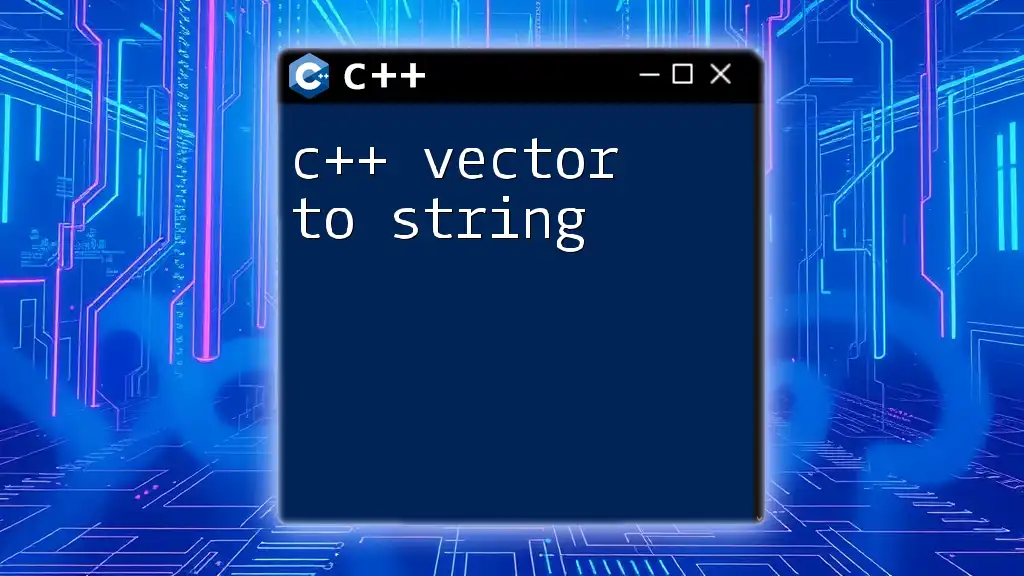
Alternative Approaches to Removing Elements
Using `std::remove_if` and `Erase` Idiom
If your goal is to remove multiple elements based on a condition, you can use the combination of `std::remove_if` and `erase()`. This approach is particularly useful when you need to filter out unwanted elements.
For example, to remove all even numbers from a vector:
#include <algorithm>
numbers.erase(std::remove_if(numbers.begin(), numbers.end(), [](int x) { return x % 2 == 0; }), numbers.end());
This translates to removing all elements that satisfy the condition defined in the lambda function (in this case, all even numbers).
Utilizing `std::remove` for Sequential Removal
Another method for managing elements is utilizing `std::remove`, which can help with removing non-contiguous elements. This method rearranges the vector to bring desired elements to the front before you can erase them based on a specified condition.
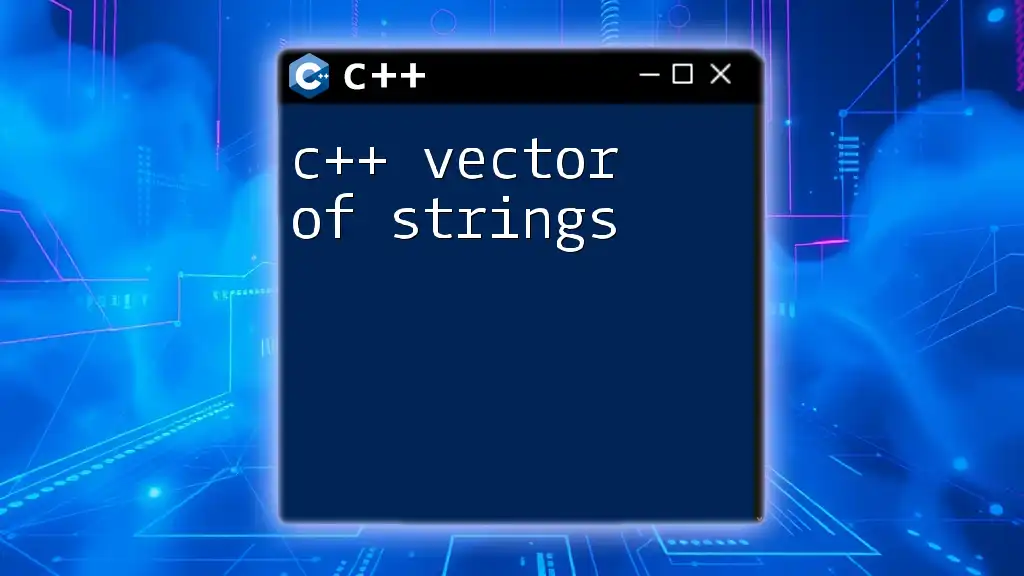
Conclusion
Mastering how to C++ vector remove at index is a vital skill for any programmer working with data structures in C++. With the `erase()` method and alternative techniques at your disposal, you have the flexibility to manage and manipulate your data effectively.
Explore various practical applications of these concepts in your coding projects, and remember that understanding the nuances of vector management will enhance your programming capabilities.
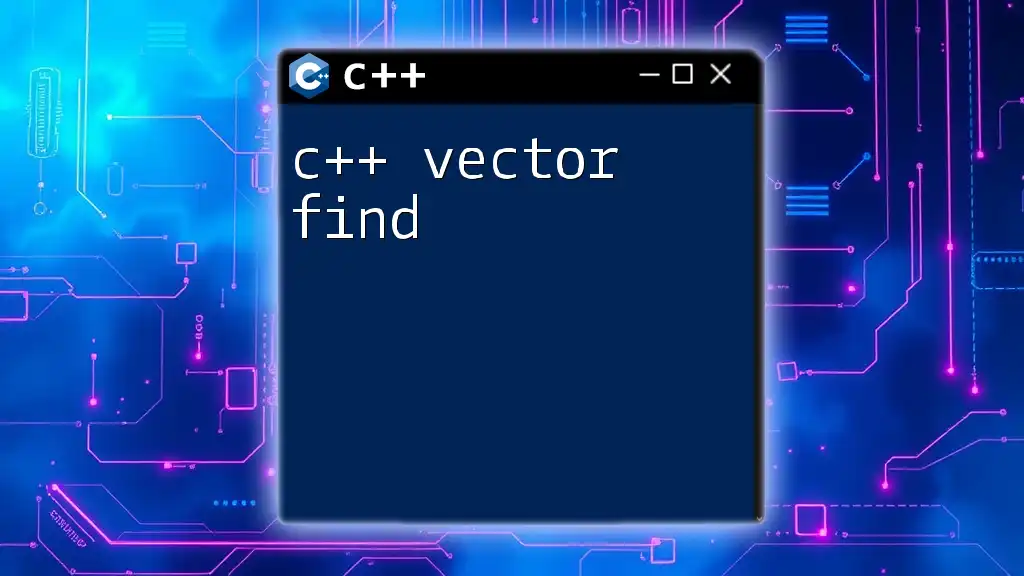
Additional Resources
Recommended Reading
For more in-depth information about vectors and their methods, you can explore the [C++ Standard Library documentation](https://en.cppreference.com/w/cpp/container/vector).
Practice Problems
Consider creating a few practice problems related to vector manipulation to hone your skills further. Challenge yourself to remove elements under different scenarios, such as maintaining vector order or removing duplicates.
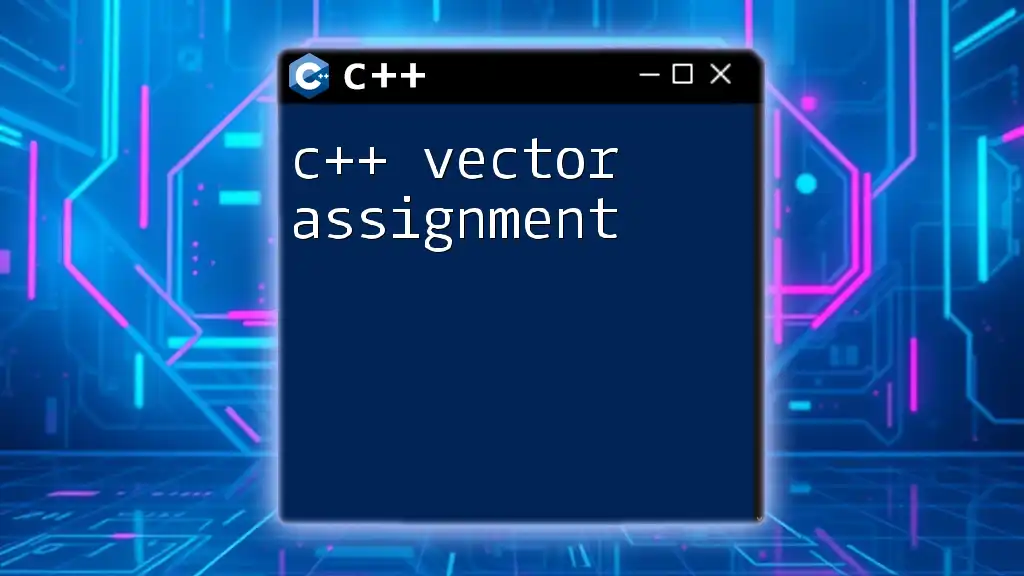
Call to Action
We invite you to share your thoughts, experiences, or any questions regarding C++ vectors in the comments section. Don't forget to subscribe for more concise C++ learning materials and articles!