In C++, the `std::string` class is mutable, allowing you to modify its contents after creation.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello";
str[0] = 'h'; // Modifying the first character
std::cout << str; // Output: hello
return 0;
}
Understanding Strings in C++
What is a String?
In C++, a string is a sequence of characters used to represent text. Strings are one of the most commonly used data types in programming, allowing developers to manage and manipulate textual data easily.
Types of Strings in C++
C-style Strings
C-style strings, or character arrays, are arrays of characters terminated by a null character (`'\0'`). They require manual memory management, leading to increased complexity and potential errors.
Here’s an example of a C-style string:
char myString[] = "Hello, world!";
C++ Standard Library Strings
The C++ Standard Library provides the `std::string` class, which is more versatile and user-friendly. `std::string` handles memory automatically, provides various methods for manipulation, and can grow dynamically.
#include <string>
std::string myString = "Hello, world!";
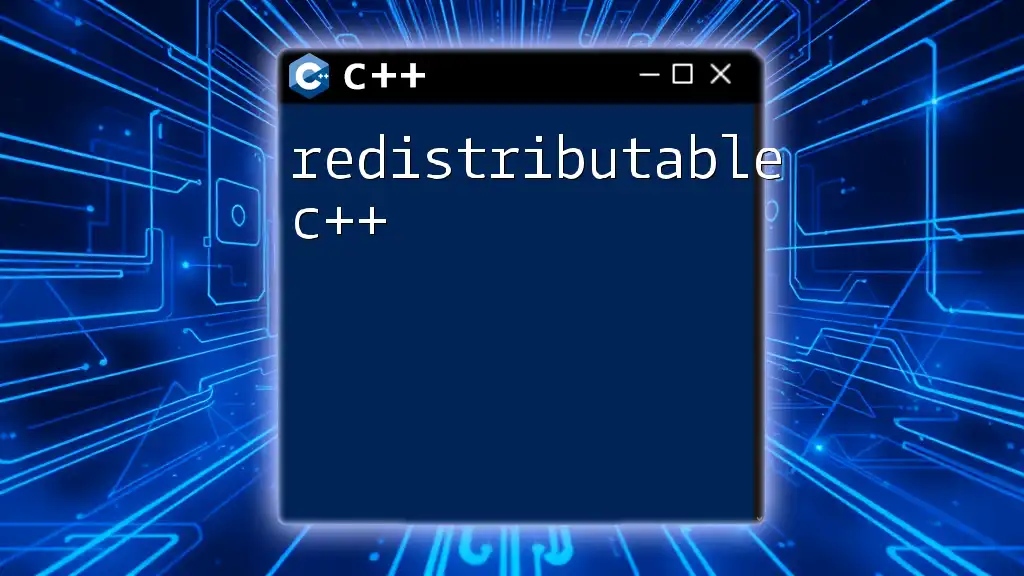
Mutability Explained
Defining Mutability
Mutability refers to the ability of an object to change its state or value after it has been created. An object is said to be immutable if it cannot be altered once it has been instantiated. Understanding this concept is crucial for managing data and its integrity effectively in programming.
Strings in C++: Mutable or Immutable?
When it comes to C++ strings, the core question emerges: Are strings mutable in C++?
- `std::string` is indeed mutable. You can modify the contents of a `std::string` after it has been created. For example:
std::string str = "Hello";
str += ", World!"; // Modifying the string
In the above snippet, the `+=` operator appends `", World!"` to the original string.
On the other hand, C-style strings operate differently. They are also mutable, but you must be careful with memory management:
char myString[] = "Hello";
myString[5] = ',';
myString[6] = ' ';
myString[7] = 'W';
myString[8] = 'o';
myString[9] = 'r';
myString[10] = 'l';
myString[11] = 'd';
myString[12] = '!';
myString[13] = '\0'; // Null-terminating the string
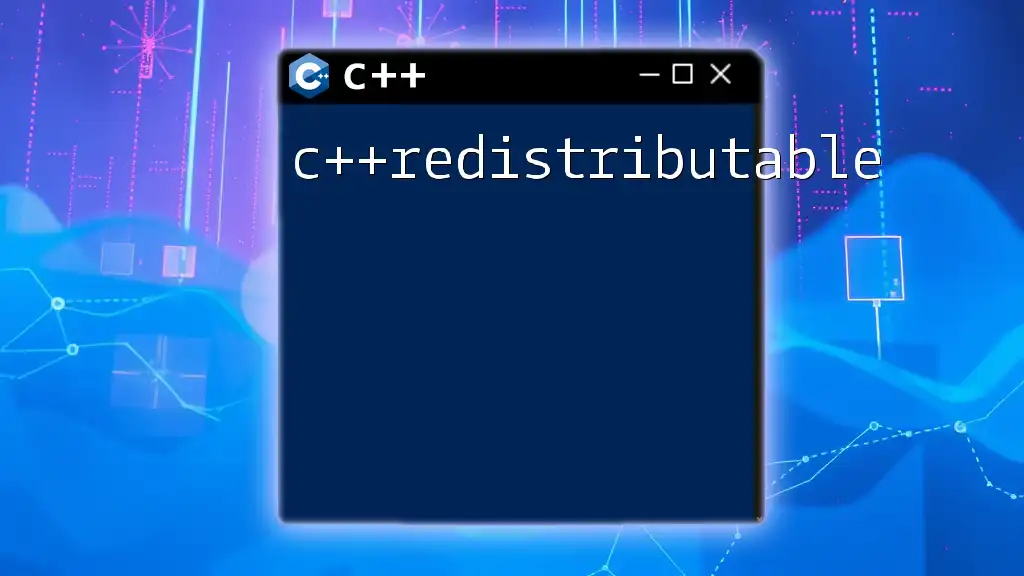
Practical Implications of String Mutability
When and Why to Use Mutable Strings
Using mutable strings can significantly simplify operations like concatenation, modification, or insertion of characters. In scenarios where dynamic text manipulation is required, mutable strings are invaluable. For instance, when building a text editor application, being able to modify strings easily is crucial.
Examples of String Mutations in C++
Example 1: Using `std::string` methods
The `std::string` class provides several methods to manipulate strings easily:
std::string str = "C++ is fun!";
str.append(" Let's learn more.");
str.replace(0, 3, "C#"); // Replaces "C++" with "C#"
str.insert(8, "programming "); // Inserts text at index 8
Each method demonstrates how `std::string` allows for seamless modifications.
Example 2: Modifying C-style Strings
Modifying C-style strings must be done with caution. Here’s an example showing how to change a character:
char message[] = "Hello, C!";
message[7] = 'C'; // Changes the 'C' to 'C'
However, keep in mind the risks of buffer overflows if you're not careful with memory allocation.
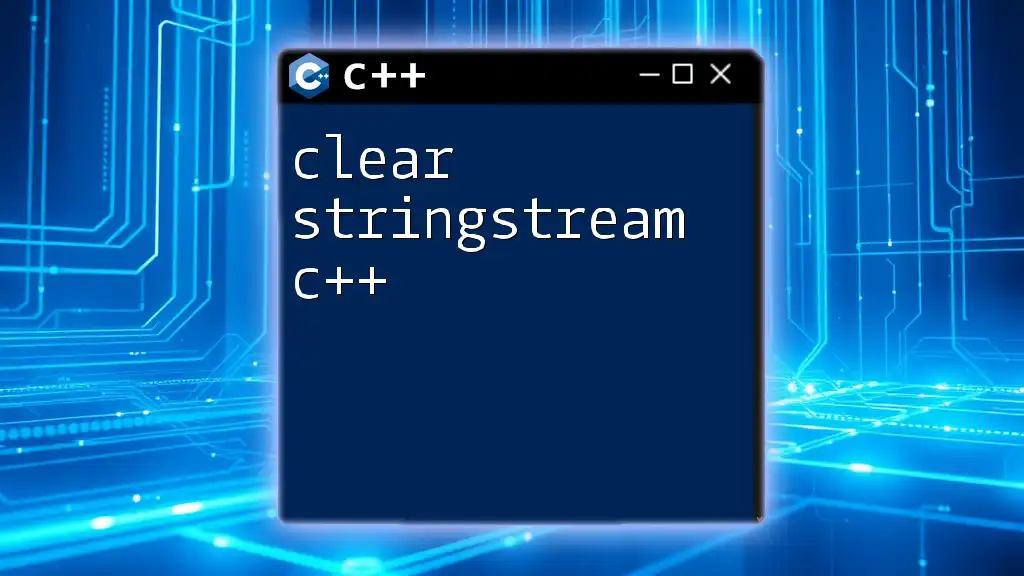
Performance Considerations
Memory Management with Mutable Strings
`std::string` handles memory allocation automatically, which means it can allocate and deallocate memory as needed. This makes `std::string` more memory efficient compared to manual character arrays, reducing the risk of memory leaks or improper memory access.
Comparing Mutable and Immutable Strings in C++
The choice between mutable and immutable strings boils down to specific use cases. On one hand, mutable strings (`std::string`) simplify text manipulation and reduce the overhead of managing memory manually. On the other hand, immutable strings can provide benefits in terms of safety, as they prevent unintended alterations to data. For example, if you need to ensure that data remains constant throughout the program, immutability holds advantages.
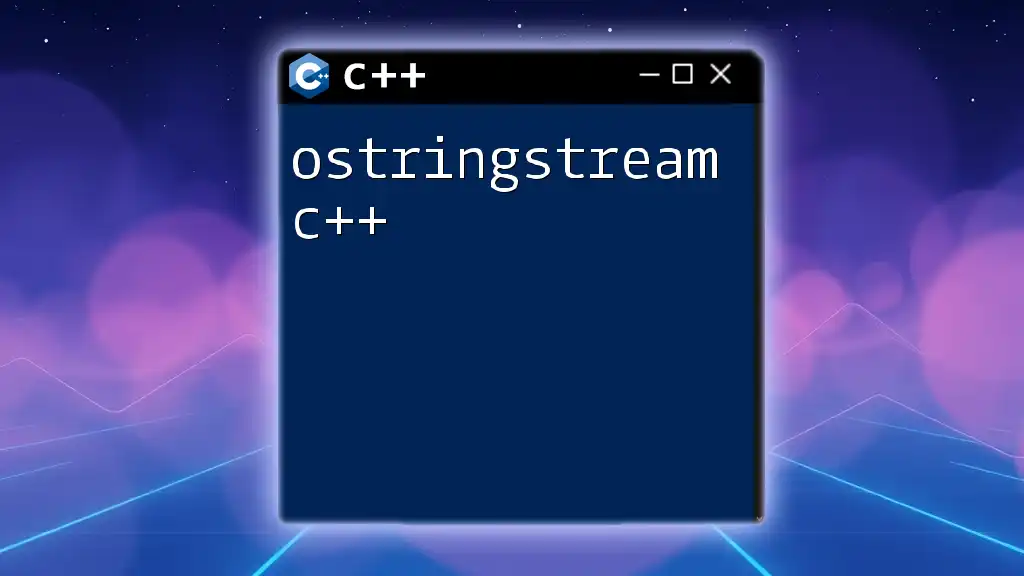
Common Misconceptions about String Mutability
Clarifying Myths
A frequent myth is that strings in C++ are immutable like in some other languages (e.g., Java). In reality, C++ strings are mutable. The misunderstanding can lead to incorrect assumptions about string manipulation and memory management.
Summary of Key Points
In summary, are strings mutable in C++? The answer is yes. Both `std::string` and C-style strings allow modifications, but they differ significantly in ease of use and safety. Understanding the mutability of strings is vital for effective programming in C++.
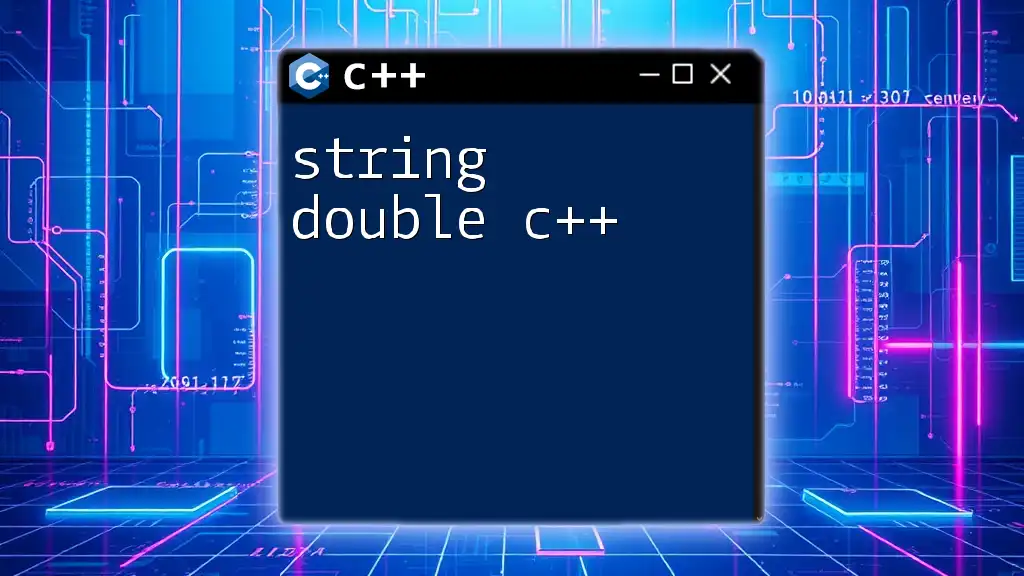
Conclusion
In conclusion, grasping the concept of string mutability is essential in C++. Mutable strings simplify data manipulation and are integral to many programming tasks. As you dive deeper into C++, understanding when and how to use mutable strings will be a valuable asset.
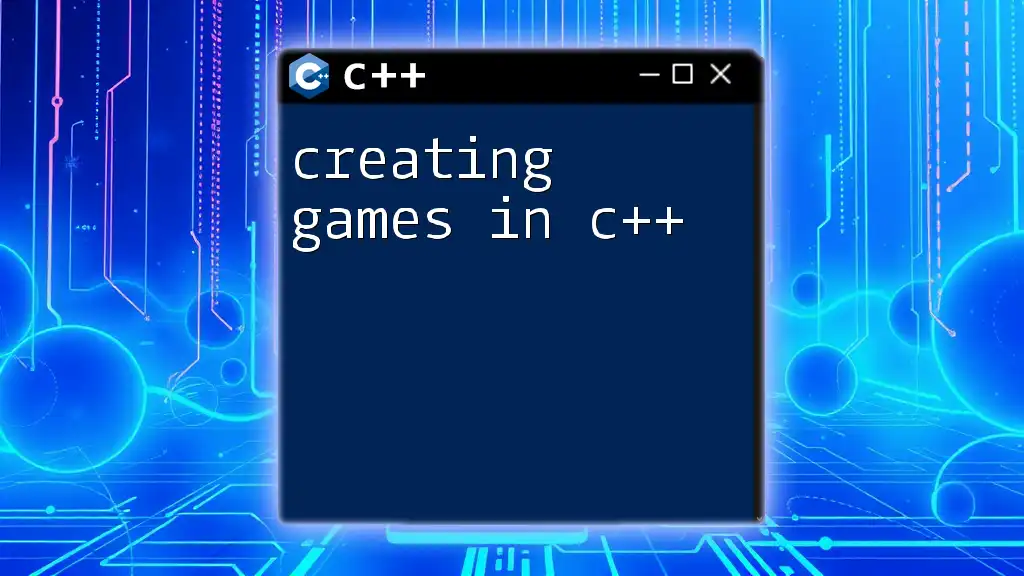
Additional Resources
To enhance your knowledge further, consider exploring books and online courses on C++ string manipulation, memory management, and best practices for efficient programming. Engaging with practical exercises and scenarios will solidify your understanding and preparation for real-world applications.
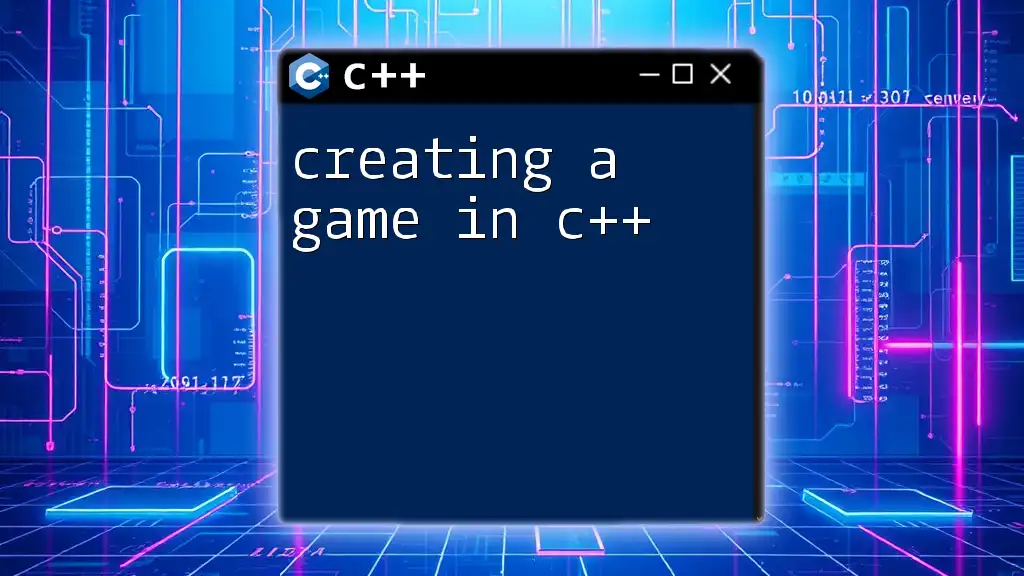
Call to Action
If you're eager to learn more about C++ and string manipulation, join our classes today! Share your experiences and insights on working with mutable and immutable strings in the comments below. Your journey into mastering C++ strings starts here!