In C++, the `float` and `int` data types represent different kinds of numbers, with `float` being used for single-precision decimal values and `int` for whole numbers, and they can be returned from functions depending on the specified return type.
Here's a simple code snippet demonstrating the return of a `float` and an `int`:
#include <iostream>
int returnInt() {
return 42;
}
float returnFloat() {
return 3.14f;
}
int main() {
std::cout << "Integer: " << returnInt() << std::endl;
std::cout << "Float: " << returnFloat() << std::endl;
return 0;
}
Understanding Data Types in C++
What are Data Types?
In C++, data types define the type of data a variable can hold and lay the foundation for how data is manipulated. Understanding various data types is crucial, as it influences performance, memory usage, and the operations you can perform on them.
Common Data Types in C++
Integers (int)
The `int` data type is one of the fundamental types in C++. It represents whole numbers without a fractional component. Common use cases for integers include counting, indexing array elements, and performing arithmetic operations where fractions are not needed.
Floating Points (float)
The `float` data type represents numbers with fractional components—essentially real numbers. A typical scenario for using `float` arises in situations requiring precision, such as scientific calculations, graphics programming, or financial systems, where decimals matter.
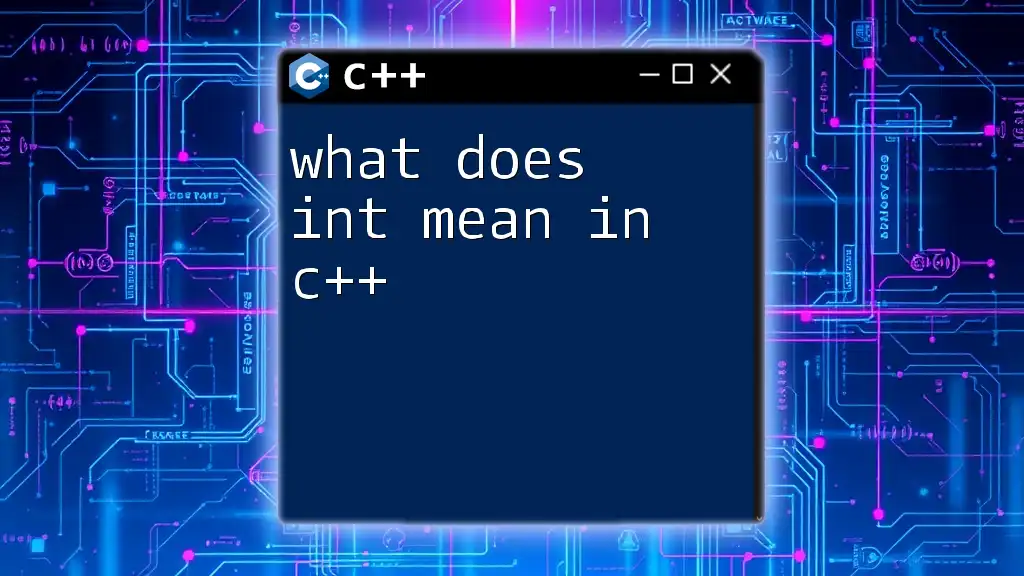
The Return Type Concept in C++
What Does Return Type Mean?
The return type in a function specifies what type of value the function will return to its caller. This is a critical aspect of function design as it informs the compiler and the programmer what kind of data is produced when calling the function.
How Return Types Affect Function Behavior
Return types directly influence how functions interact with other variables and functions. For instance, a function designed to return an integer can be used in arithmetic operations, while one returning a float can handle decimal computations.
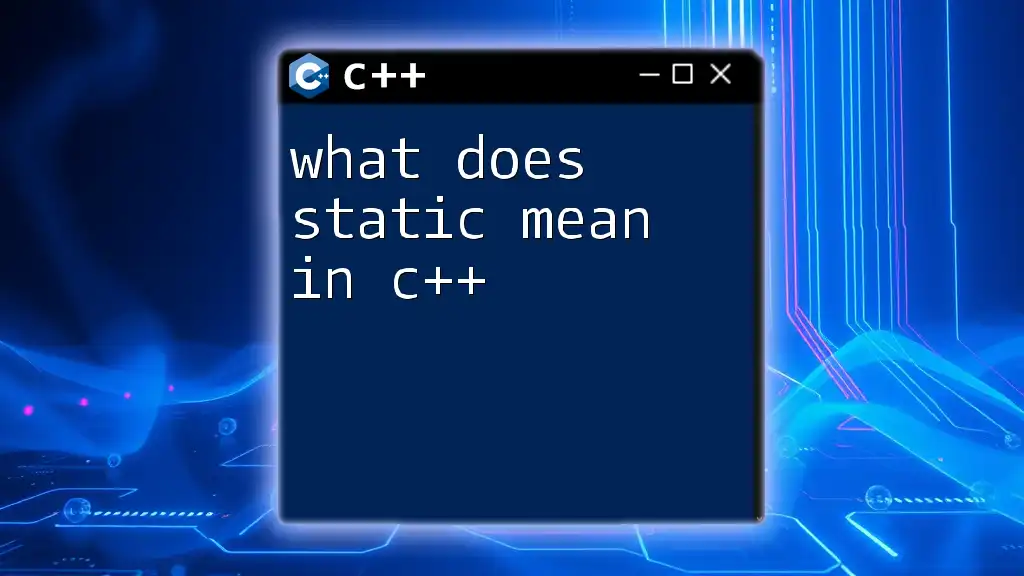
Returning Integers (int)
How to Return an Integer
Returning an integer from a function is straightforward. Below is an example function designed to return a fixed integer value:
int returnInteger() {
return 5;
}
In this example, the function `returnInteger` will always yield the integer value 5 when called. This type of function is beneficial when you want to retrieve a constant value.
Example of Calculating with Integers
Consider a function that adds two integers:
int add(int a, int b) {
return a + b;
}
Here, the function `add` takes two integer parameters, `a` and `b`, and returns their sum. This illustrates how integers are used in arithmetic operations effectively.
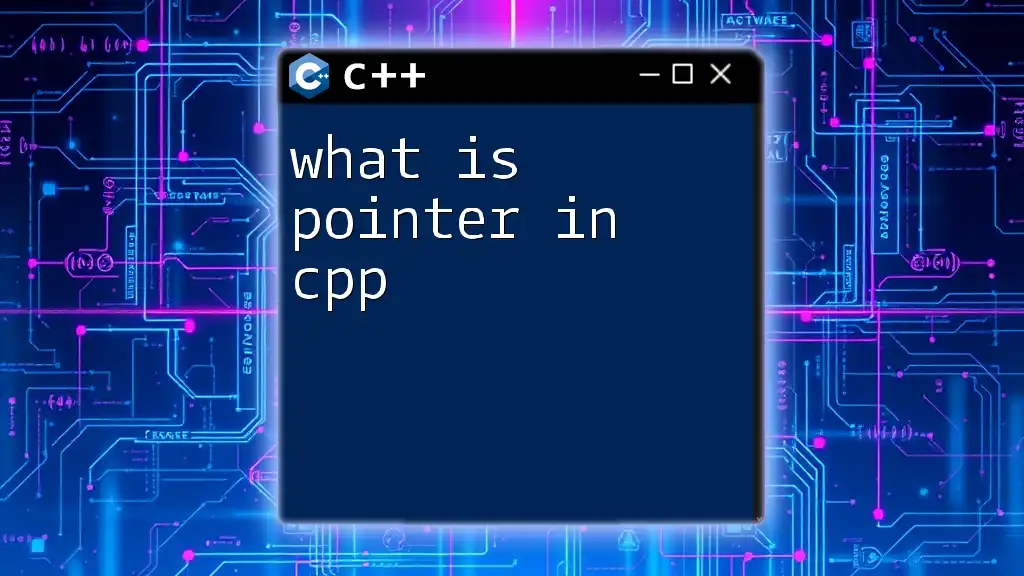
Returning Floating Points (float)
How to Return a Floating Point
To return a floating-point number, you can define a function as follows:
float returnFloat() {
return 3.14f;
}
The function `returnFloat` returns the float value 3.14. The f suffix indicates that the literal is a float type; this is essential for maintaining precision and ensuring that arithmetic operations treat this value correctly as a float.
Example of Operations with Floats
A common use case for floats is division. Consider the following function:
float divide(float a, float b) {
return a / b;
}
The `divide` function takes two float parameters and returns their division result. It demonstrates how floating-point arithmetic is performed. Be aware of precision issues that can arise with floating-point calculations, especially when dealing with very large or very small numbers.
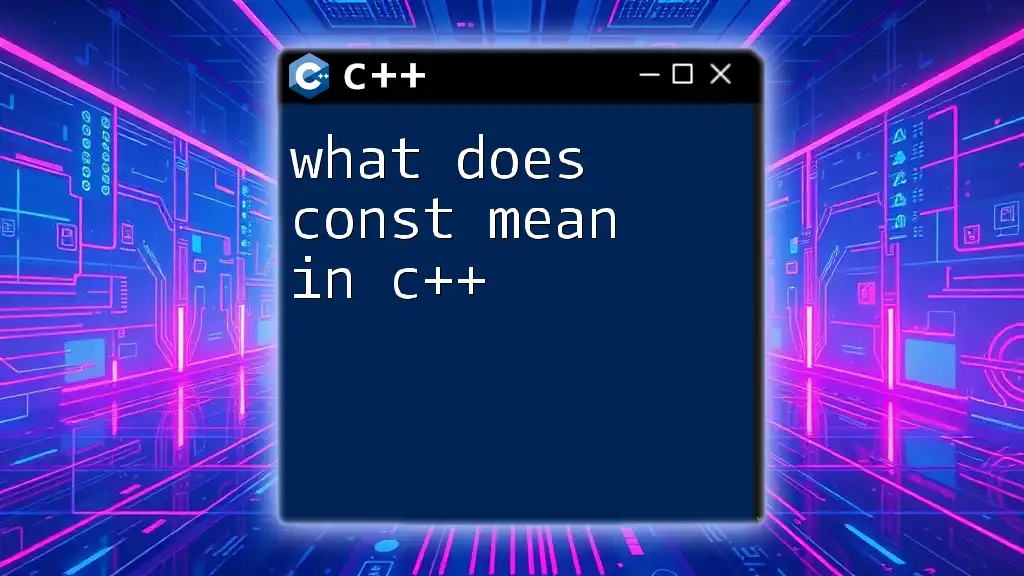
Mixed Return Types: Float and Int
What Happens When You Mix Return Types?
Mixing different return types can lead to implicit or explicit type conversions. These conversions can lead to unexpected behavior if not handled carefully. Understanding type safety is crucial in designing robust functions.
Example of a Mixed Type Function
Here’s an instance where you can return a float from an integer operation:
float mixedOperation(int a, int b) {
return (float)a / b; // Explicitly converting 'a' to float
}
In this example, `mixedOperation` takes two integer parameters, converts the first to a float, and then performs division. This illustrates the importance of casting to avoid losing precision in mathematical operations.
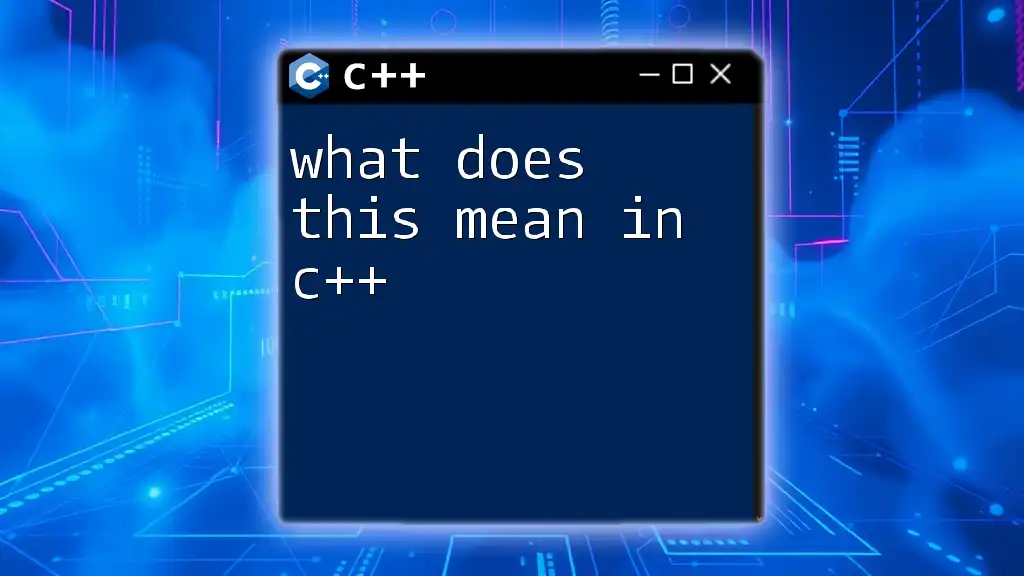
Common Use Cases for float and int Returns
Practical Applications of Returning Integers
Returning integers is common in counting, indexing arrays, and managing loop control. For example, functions that return the count of items, indices in arrays, or the number of iterations needed in a loop heavily rely on integer return types.
Practical Applications of Returning Floats
Returning float values is essential for mathematics, scientific computations, and scenarios where precision with decimals is needed, such as creating graphical applications that involve coordinates, physics engines, or financial applications where values can have cents.
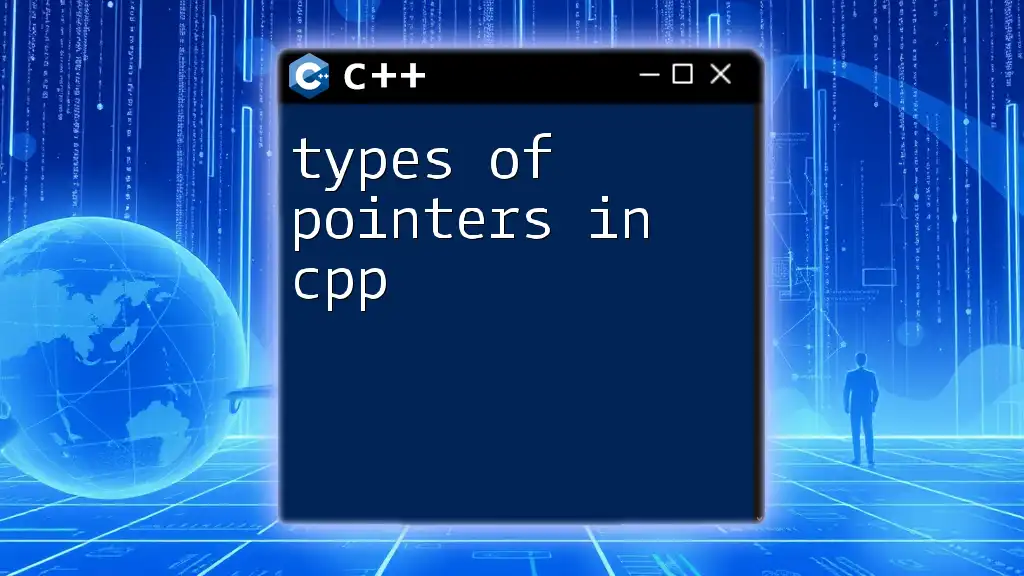
Debugging Float and Int Return Types
Common Errors When Working with Float and Int
Errors often occur when performing operations on mixed types. A common pitfall is integer division, which discards the fractional part if both operands are integers.
int divide(int a, int b) {
return a / b; // Potential for integer division error
}
If `a` is 5 and `b` is 2, the result will be 2 instead of 2.5 as one might initially expect. This behavior often leads to bugs and miscalculations.
How to Handle and Debug These Errors
To avoid these issues, you can ensure that at least one operand is of type float to get a correct result:
float divide(int a, int b) {
return (float)a / b; // Correctly returns a float
}
Always be cautious of data type conversions and ensure that you check the return types of functions carefully to prevent subtle bugs.
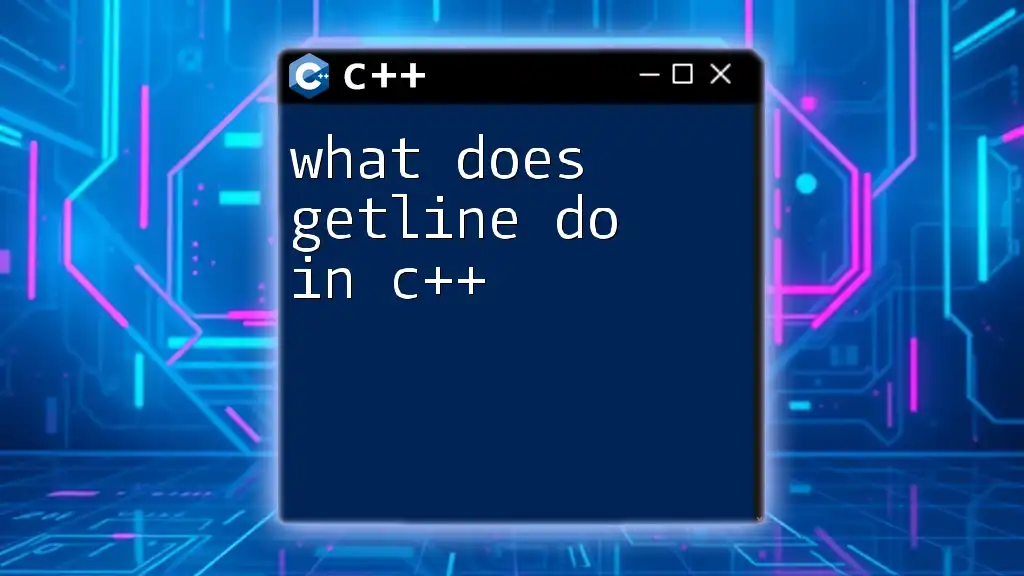
Best Practices for Using Return Types
Consistency in Function Design
Maintaining consistency in your function's return types is vital. A function that is expected to return an integer should always do so, as this makes the code predictable and easier to read.
Choosing the Right Type for Your Needs
Selecting between `float` and `int` depends on your specific requirements—integers for whole numbers and counts, and floats for precision-related tasks. Consider the precision required and the type of computations involved when designing your functions.
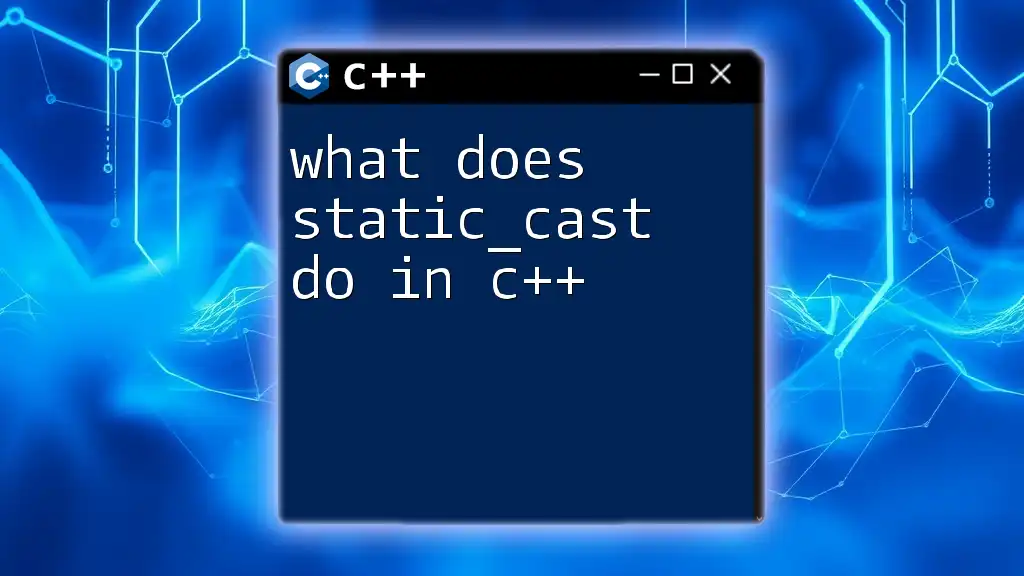
Conclusion
Understanding what float / int returns in C++ is foundational for effective programming. By grasping the nuances of data types and return types, developers can harness the power of C++ for complex applications. Armed with this knowledge, you’ll be better equipped to write accurate and efficient code.
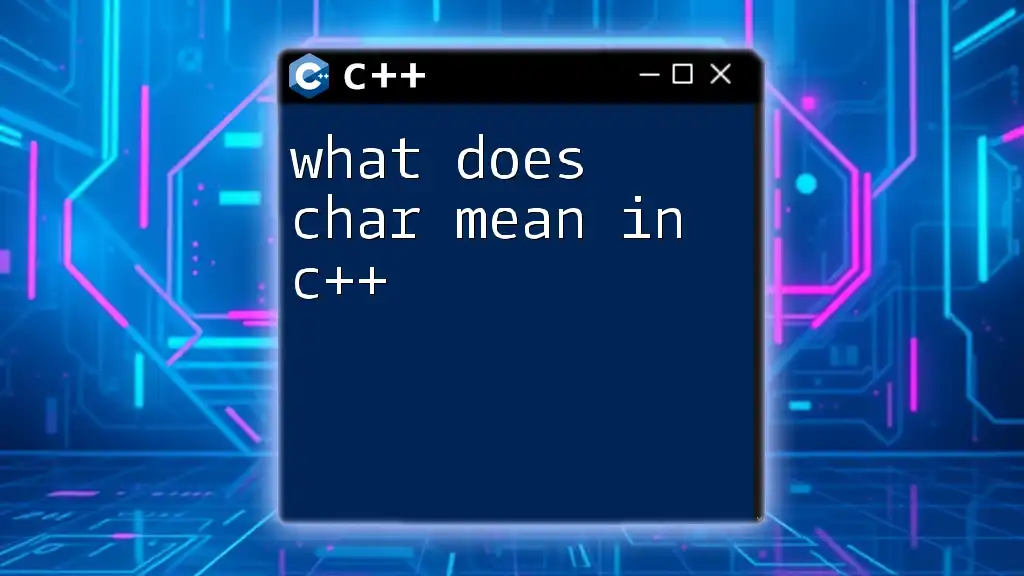
Additional Resources
For further reading, consider exploring the [C++ Documentation](https://en.cppreference.com/w/) for a deep dive into data types, function syntax, and community-driven forums for practical coding exercises. Embrace the learning journey and experiment with various data types to solidify your understanding!