SHA-256 is a cryptographic hash function that generates a fixed-size (256-bit) hash value from input data, ensuring data integrity and security, which can be easily implemented in C++ using libraries like OpenSSL.
Here's a simple example of how to compute a SHA-256 hash in C++ using OpenSSL:
#include <iostream>
#include <openssl/sha.h>
std::string sha256(const std::string &input) {
unsigned char hash[SHA256_DIGEST_LENGTH];
SHA256(reinterpret_cast<const unsigned char*>(input.c_str()), input.size(), hash);
std::string output;
for (const auto &byte : hash) {
output += sprintf("%02x", byte);
}
return output;
}
int main() {
std::string data = "Hello, World!";
std::cout << "SHA-256 Hash: " << sha256(data) << std::endl;
return 0;
}
Understanding Hash Functions
What is a Hash Function?
A hash function is a mathematical algorithm that transforms an input (or 'message') into a fixed-length string of characters, which is typically a sequence of numbers and letters. The output is known as a hash value, hash code, or digest. In the realm of cryptography and data integrity, hash functions serve crucial roles, ensuring data consistency, verifying integrity, and securing sensitive information.
Properties of a Secure Hash Function
A secure hash function must meet several essential properties:
- Deterministic: It should produce the same output whenever the same input is applied.
- Fast Computation: The hash value must be quick to compute for both large and small inputs.
- Pre-image Resistance: Given a hash value, it should be computationally infeasible to derive the original input.
- Small Changes Result in Large Differences: Even a tiny change to the input should create a completely different output, a property known as the avalanche effect.
- Collision Resistance: It must be extremely difficult to find two distinct inputs that yield the same hash output.
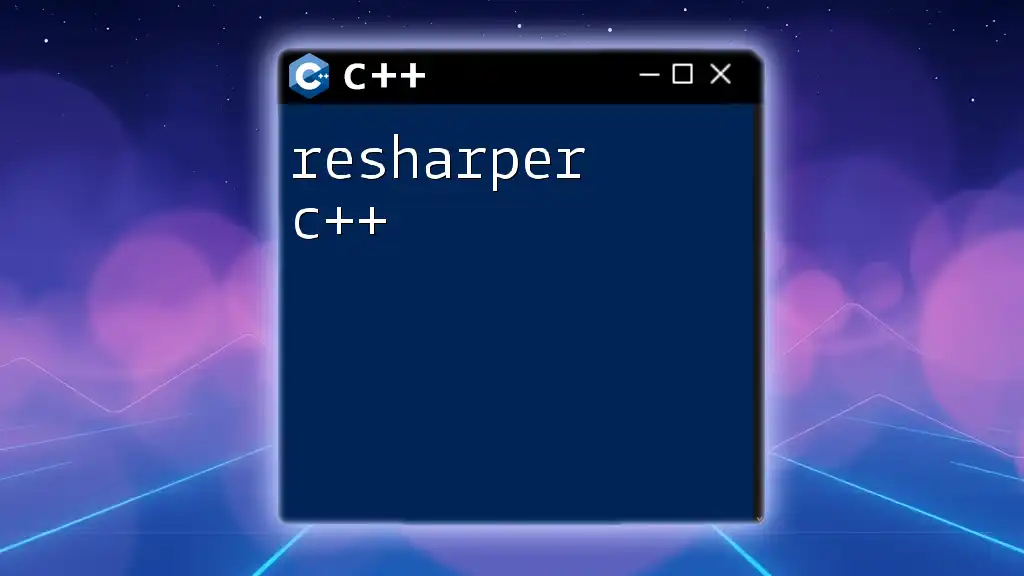
Overview of SHA-256
How SHA-256 Works
SHA-256, part of the SHA-2 family, processes input data in blocks. It accepts input with a flexible length and outputs a 256-bit (32-byte) hash. The algorithm works through various rounds of processing the input message and applying transformations through bitwise operations, modular arithmetic, and logical functions.
- Initial Hash Values: SHA-256 uses eight initial hash values, which are constants derived from the square roots of the first 64 prime numbers.
- Pre-processing: The input gets padded to ensure its length is a multiple of 512 bits. This includes appending a single '1', followed by enough '0's, and finally, the original message length.
- Main Loop: The core of SHA-256 processing involves 64 rounds of computations where message schedules are generated and mixed with the hash values.
- Finalization: Once processed, the output hash is generated and consists of the final hash values.
Use Cases of SHA-256
SHA-256 is used widely in various domains:
- Data Integrity: Ensuring data has not been altered during transmission.
- Cryptocurrencies: It is foundational in the blockchain technology of cryptocurrencies like Bitcoin, securing transactions.
- Digital Signatures and Certificates: By hashing messages, SHA-256 helps to produce unique signatures that verify authenticity.
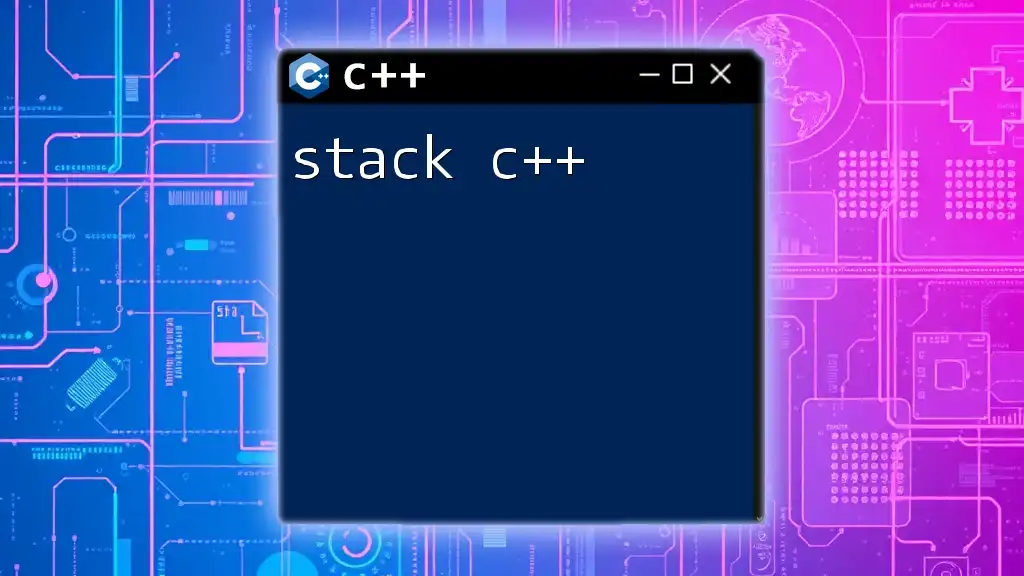
Implementing SHA-256 in C++
Required Libraries and Dependencies
To work with SHA-256 in C++, libraries such as OpenSSL and Crypto++ can significantly simplify the implementation. OpenSSL is particularly popular due to its robust functionality and extensive documentation. Installing these libraries typically involves using a package manager or downloading them directly from their official repositories.
Setting Up Your C++ Development Environment
Prepare your C++ development environment according to the library you choose. For instance, if you opt for OpenSSL, ensure that your IDE can locate the header files and link against the corresponding object files. This setup usually includes:
- Installing required libraries.
- Configuring include paths in your IDE.
- Setting linker settings for linking against the SHA library.
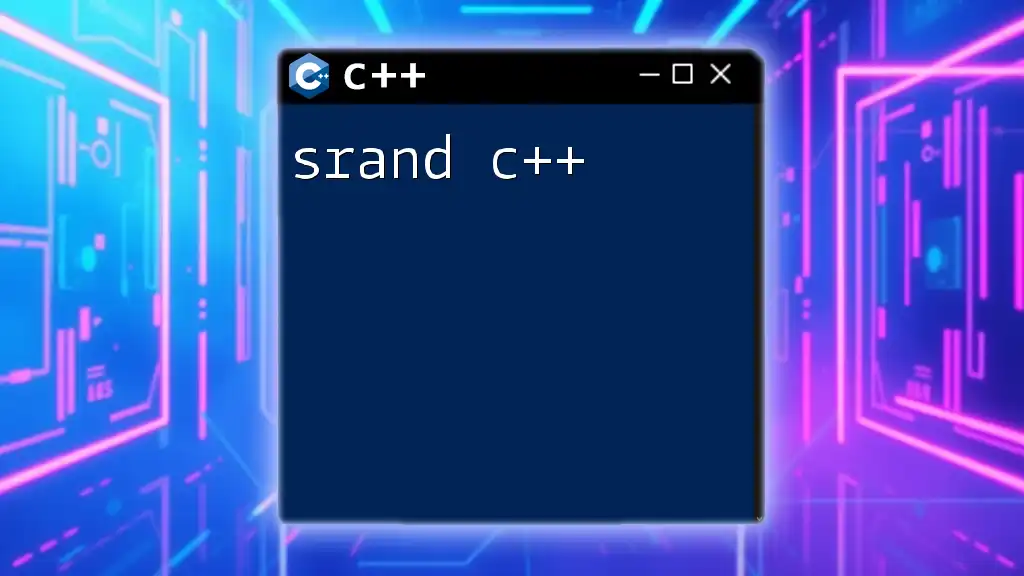
Writing Your First SHA-256 Implementation in C++
Simple SHA-256 Example using OpenSSL
Here's a quick example illustrating how to use OpenSSL to compute SHA-256 hashes.
#include <iostream>
#include <openssl/sha.h>
void SHA256_example(const std::string& input) {
unsigned char hash[SHA256_DIGEST_LENGTH];
SHA256_CTX sha256;
SHA256_Init(&sha256);
SHA256_Update(&sha256, input.c_str(), input.size());
SHA256_Final(hash, &sha256);
std::cout << "SHA-256 Hash: ";
for(int i = 0; i < SHA256_DIGEST_LENGTH; i++) {
printf("%02x", hash[i]);
}
std::cout << std::endl;
}
In this code:
- We initialize the SHA-256 context using `SHA256_Init()`.
- We feed the input string into the hashing process with `SHA256_Update()`.
- Finally, `SHA256_Final()` finalizes the hash and stores it in the `hash` array.
Custom SHA-256 Algorithm Implementation
For educational purposes, you might want to implement SHA-256 from scratch. Here’s a code snippet to get you started:
#include <iostream>
#include <vector>
#include <string>
#include <iomanip>
class SHA256 {
// SHA256 class declaration and private methods
void transform(const uint8_t* message, size_t block_count);
static void initialize();
void update(const uint8_t* message, size_t length);
void finalize();
public:
SHA256();
void update(const std::string& input);
std::string digest();
static std::string hash(const std::string& input);
};
// Add your transform, initialize, update, finalize, and other methods here
In this custom implementation:
- initialize() sets up initial hash values and other configurations.
- transform() performs multiple rounds of hashing using bitwise operations, creating the final digest based on the pre-processed input.
- update() allows data to be fed incrementally.
- digest() retrieves the final hash as a string.
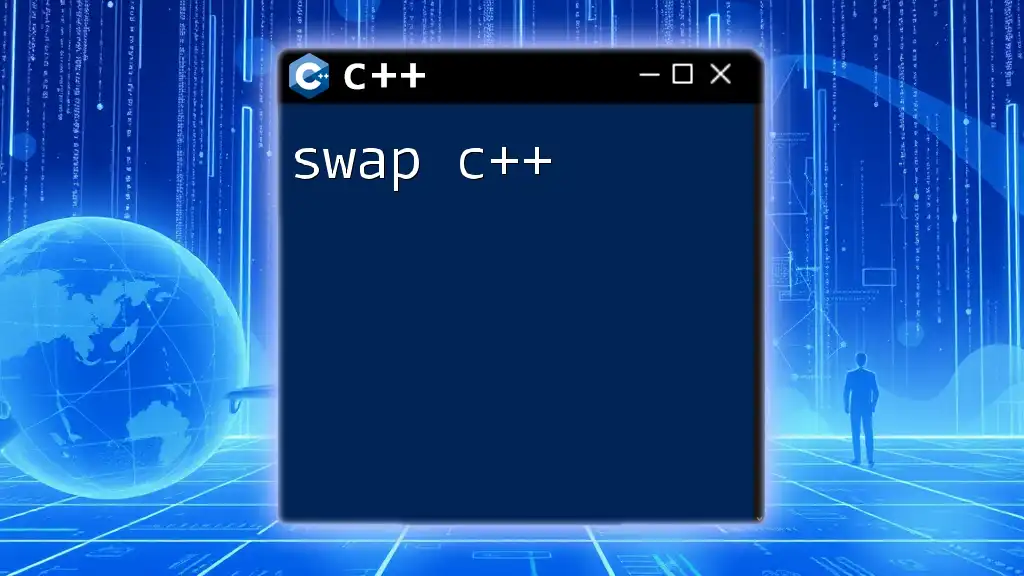
Testing Your SHA-256 Implementation
Unit Testing
Unit testing is crucial for verifying the correctness of cryptographic implementations. It helps ensure that your SHA-256 implementation works as intended and produces accurate results. You can create a set of test vectors, which are predetermined input values and their expected hash outputs, to validate your implementation against known standards.
Validating SHA-256 Output
When testing your implementation, consider known SHA-256 test vectors. Websites like the NIST official website provide these vectors. Comparing your output against these vectors helps confirm the accuracy of your algorithm.
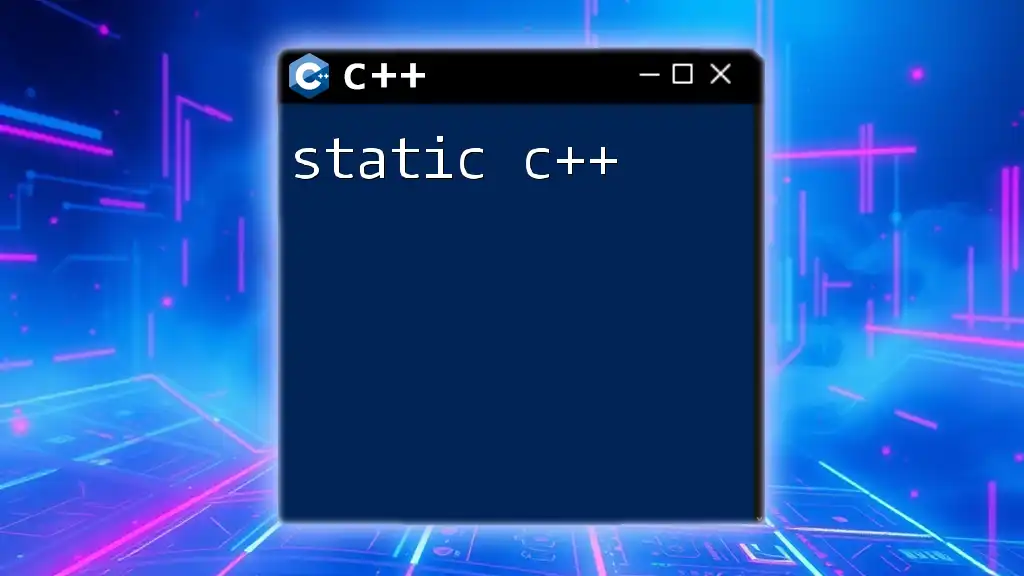
Common Errors and Debugging
Typical Issues When Implementing SHA-256
While implementing SHA-256, developers frequently encounter common pitfalls. Issues often arise from incorrect data padding, failures in managing residual bytes, or miscalculating hash values due to incorrect transformations. It's crucial to understand the algorithm's flow to identify these issues early in the implementation phase.
Best Practices for Error Handling
Implement robust error handling within your implementation. Use proper assertions and checks to monitor the validity of input data and ensure consistent hashing behavior. This aids significantly in debugging and maintaining the integrity of your hash function.
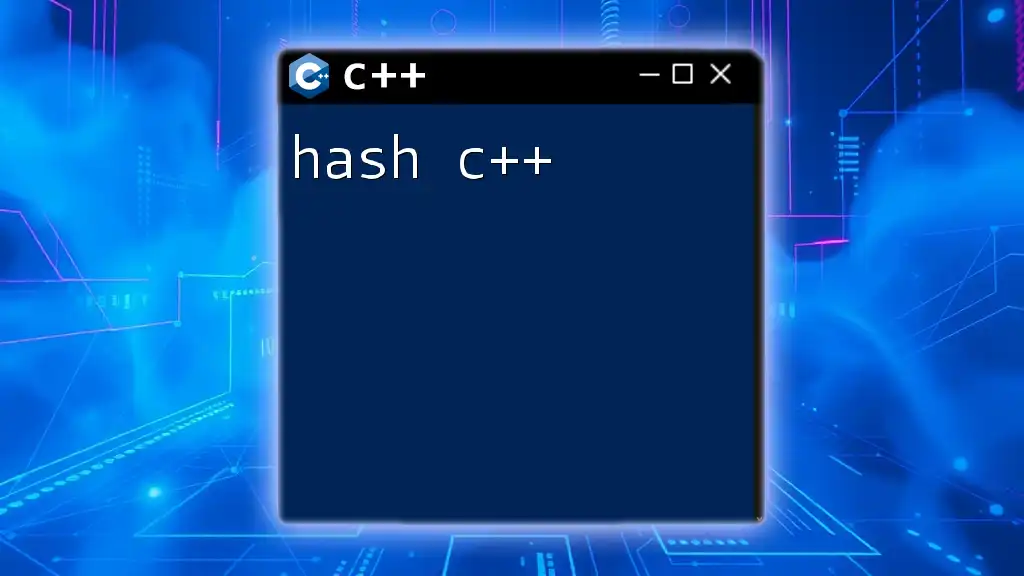
Conclusion
The significance of SHA-256 in the modern digital landscape cannot be overstated. Its applications in data integrity verification and secure transactions make it invaluable. As you venture further into cryptography, understanding and mastering SHA-256 in C++ will provide a strong foundation. Explore additional cryptographic techniques and libraries to enhance your knowledge and implementation skills.
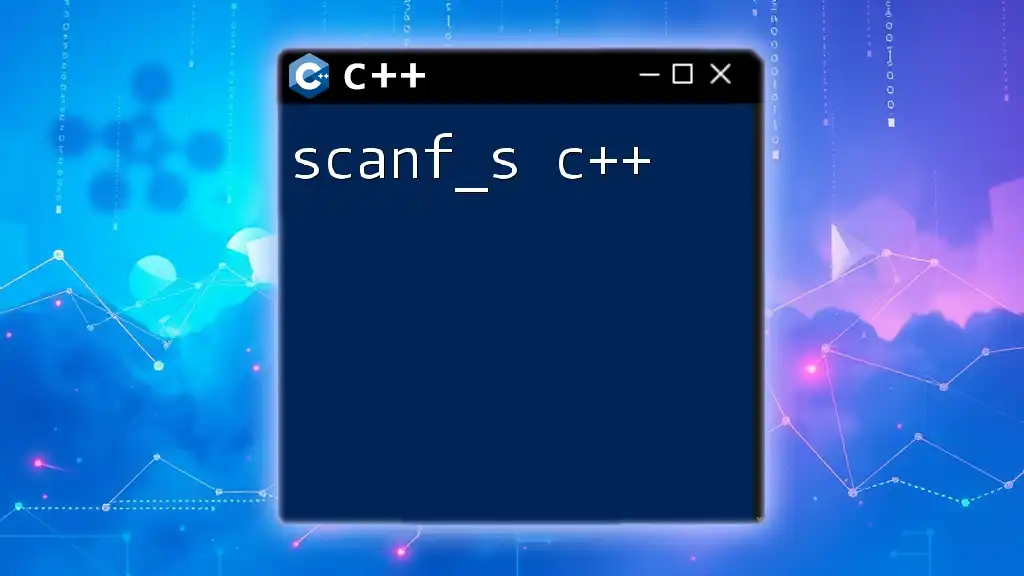
Additional Resources
For a deeper dive into SHA-256:
- Check the OpenSSL documentation for detailed library usage.
- Explore resources on cryptography principles to understand the underlying math better.
- Participate in forums such as Stack Overflow to connect with other developers learning SHA-256 and C++.