Qt is a powerful framework for developing cross-platform graphical user interfaces (GUIs) in C++, allowing developers to create visually appealing and responsive applications efficiently.
Here's a simple example of a Qt C++ GUI application that creates a basic window:
#include <QApplication>
#include <QWidget>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QWidget window;
window.resize(400, 300);
window.setWindowTitle("My First Qt GUI");
window.show();
return app.exec();
}
Understanding the Core Components of Qt GUI
What is Qt?
Qt is a powerful and versatile framework for developing cross-platform applications with graphical user interfaces (GUIs). It provides the tools necessary to build highly interactive applications for both desktop and mobile platforms. With its extensive library of pre-built components, Qt enables developers to create visually appealing and user-friendly interfaces.
What is C++?
C++ is a high-performance programming language widely used in software development. It combines the features of low-level and high-level programming, offering both efficiency and ease of use. When integrated with Qt, C++ allows developers to create robust applications, leveraging the object-oriented features and abstraction capabilities of the language.
Qt Widgets Overview
At the heart of Qt's GUI framework are widgets, which are fundamental building blocks used for creating user interfaces. Widgets can represent various UI elements, from buttons to text boxes, and even complex containers like tables and trees. Examples of commonly used widgets include `QWidget`, `QPushButton`, and `QLabel`. Each widget can be customized and styled to fit the design requirements of your application.
Signals and Slots Mechanism
The signals and slots mechanism is a unique feature in Qt that facilitates communication between different components of an application. It is an event-driven programming approach, where a signal is emitted when a particular event occurs (like a button click), and a slot is a function designed to respond to that signal.
Example: Implementing a simple signal and slot connection can show how this mechanism works:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, World!");
QObject::connect(&button, &QPushButton::clicked, &app, &QApplication::quit);
button.show();
return app.exec();
}
In this example, when the button is clicked, the application will quit, demonstrating how signals can trigger actions. Understanding this mechanism is crucial for effective event handling in your Qt C++ GUI applications.
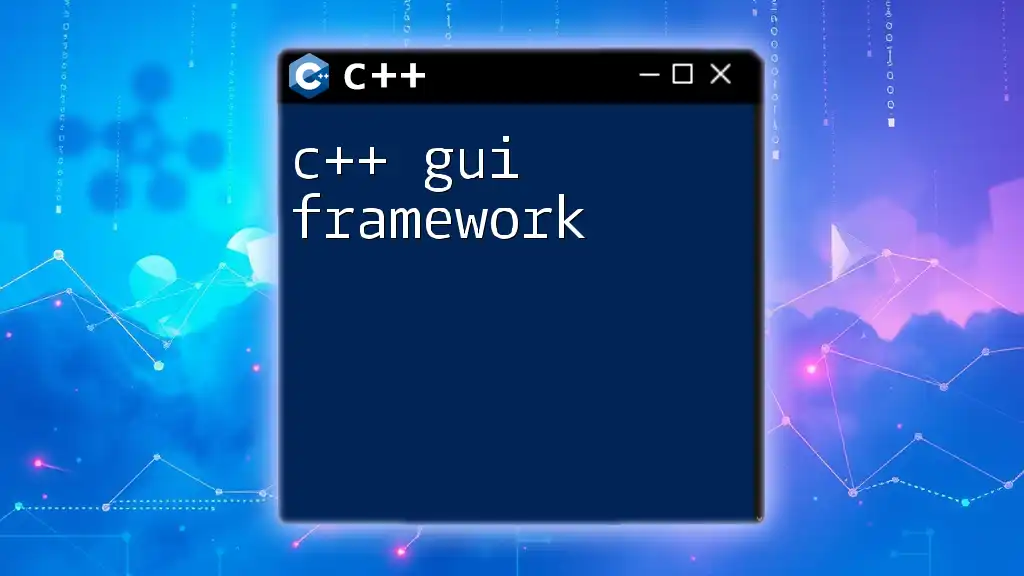
Setting Up the Qt Development Environment
Installing Qt and Qt Creator
To begin developing with Qt, you first need to install the framework along with the Qt Creator IDE. Visit the official Qt website and download the installer for your operating system. Once installed, you can select the components needed for your development, including Qt core modules, tools, and libraries.
Creating Your First Qt C++ GUI Application
Starting your first project in Qt is straightforward. Open Qt Creator and choose "New Project." Select "Qt Widgets Application" and follow the prompts to set your project name and location. After setting up your project, familiarize yourself with the project structure which typically includes source files, header files, and resource files.
Example: Below is a simplified process to create a “Hello World” GUI application.
-
Design the Interface: Use the drag-and-drop features in Qt Designer to add a `QLabel` for displaying text and a `QPushButton` for user interaction.
-
Implement Logic: Link the button's click signal to a slot that updates the label.
-
Run Your Application: Build and run the project to see your GUI in action.
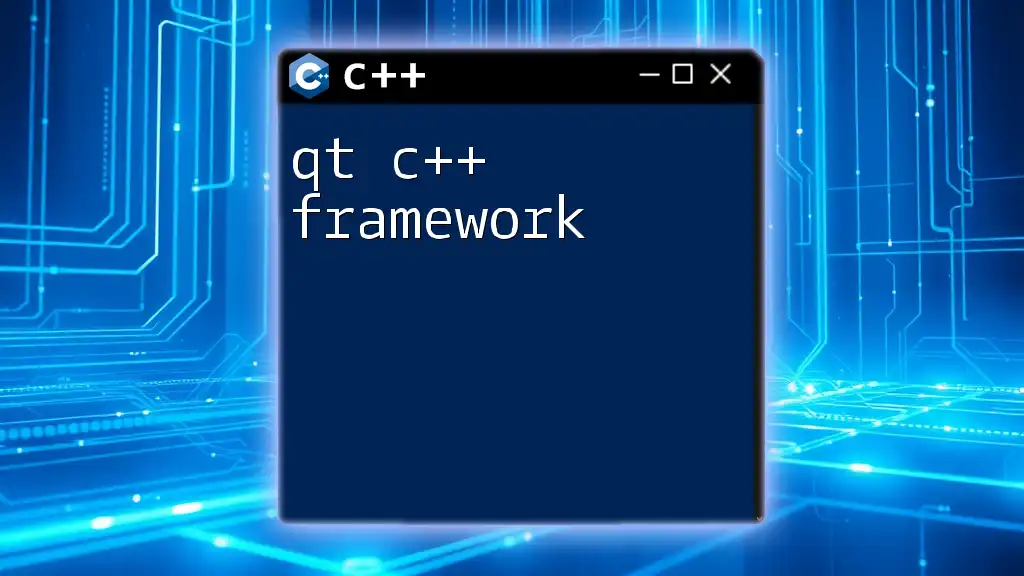
Designing User Interfaces with Qt Designer
What is Qt Designer?
Qt Designer is a standalone application that simplifies the process of designing GUIs. It provides a visual layout tool that allows developers to quickly create interfaces without coding every element by hand. This not only accelerates development time but also makes it easier for designers to contribute to the project.
Basic Layouts and Widgets
Qt Designer features various layout managers that help arrange widgets within the window. Common layout managers include `QVBoxLayout` for vertical arrangements and `QHBoxLayout` for horizontal arrangements. Using these layout managers ensures that your UI adapts to resizing and different screen resolutions efficiently.
Example: Designing a Simple Form Using Qt Designer
To create a simple form, you could drag and drop several widgets (like `QLineEdit` for user input and `QPushButton` for submission) onto the workspace. Set properties in the right panel—such as placeholder text for input fields or button labels—and connect signals to the backend logic using the signal-slot editor.
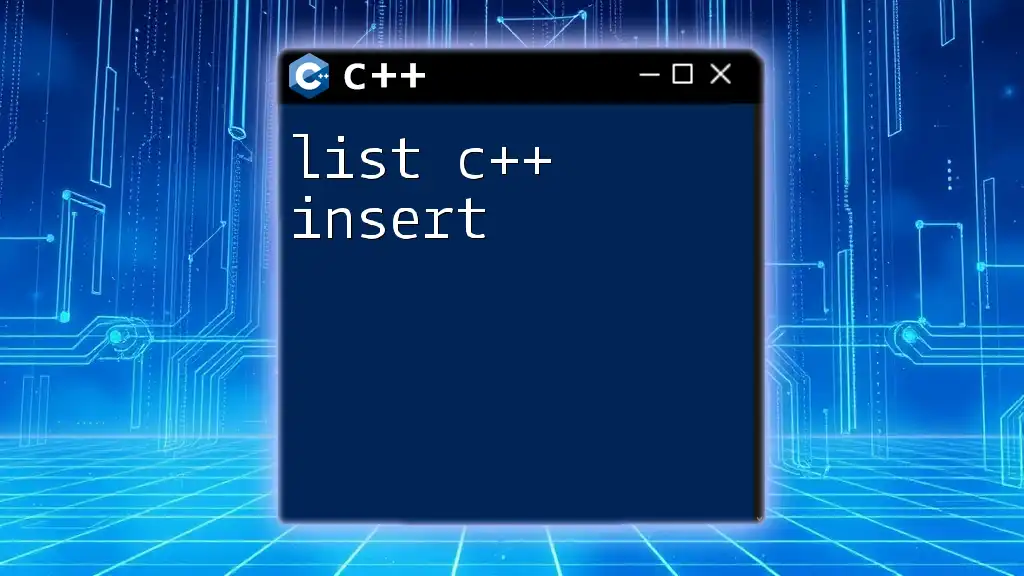
Integrating C++ Logic into the GUI
Connecting C++ Code to the GUI
Once your GUI is ready, integrating it with your backend logic is the next step. You can create slots in your C++ classes to handle user input and actions effectively.
Example: Below is a simple example of how to add functionality to a button click:
void MyWindow::on_button_clicked() {
QLabel *feedbackLabel = new QLabel("Button Clicked!", this);
feedbackLabel->show();
}
In this snippet, when the button is clicked, a label appears on the screen, giving immediate feedback to the user.
Managing Application State
Maintaining a robust application state is crucial for a great user experience. This can involve storing user preferences using `QSettings`, handling form states, or managing dynamic data models. By efficiently managing state, you ensure that the application behaves predictably and responsively to user interactions.
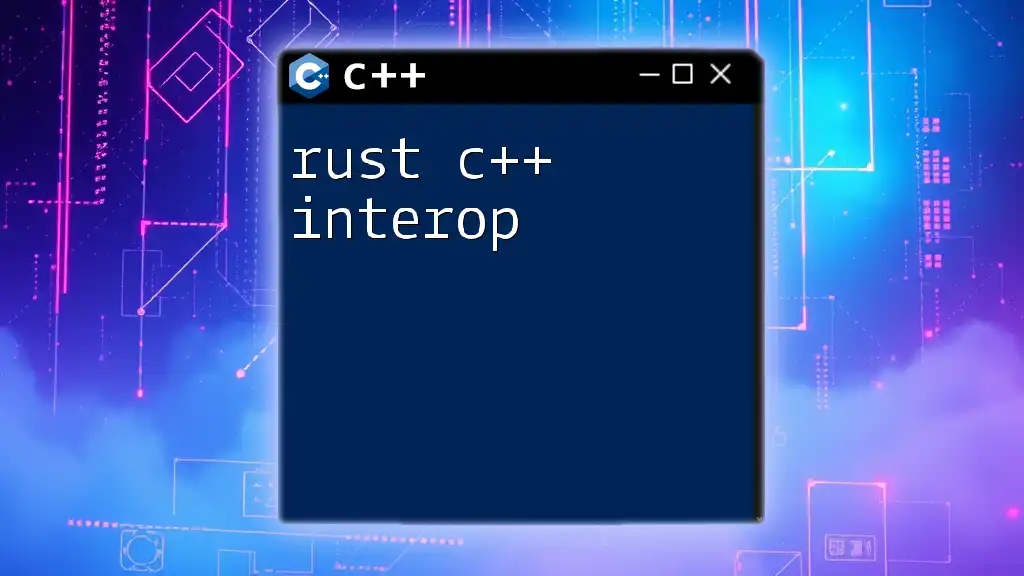
Advanced Qt C++ GUI Concepts
Custom Widgets
Sometimes, the standard widgets provided by Qt may not suit your needs. In such cases, creating custom widgets is a viable solution. Custom widgets allow you to encapsulate unique behavior or design patterns that standard widgets do not offer.
Example: Creating a custom widget could look like this:
class MyCustomWidget : public QWidget {
public:
MyCustomWidget(QWidget *parent = nullptr) : QWidget(parent) {
// Setup code for the custom widget
}
void paintEvent(QPaintEvent *event) override {
QPainter painter(this);
painter.drawRect(10, 10, 100, 100); // Custom drawing logic
}
};
In this example, we define a new widget that can perform its own custom drawing, allowing for greater flexibility in your application’s design.
Model-View-Controller (MVC) Architecture
Qt follows the Model-View-Controller (MVC) architecture, a design pattern that separates the application’s data (model), user interface (view), and logic (controller). This separation promotes organized code and allows changes in one part of the application without affecting others.
Implementing MVC in Qt involves using various model and view classes like `QAbstractItemModel` for creating data models and `QListView` or `QTableView` for views. This approach helps manage complex data efficiently while maintaining a clear and organized codebase.
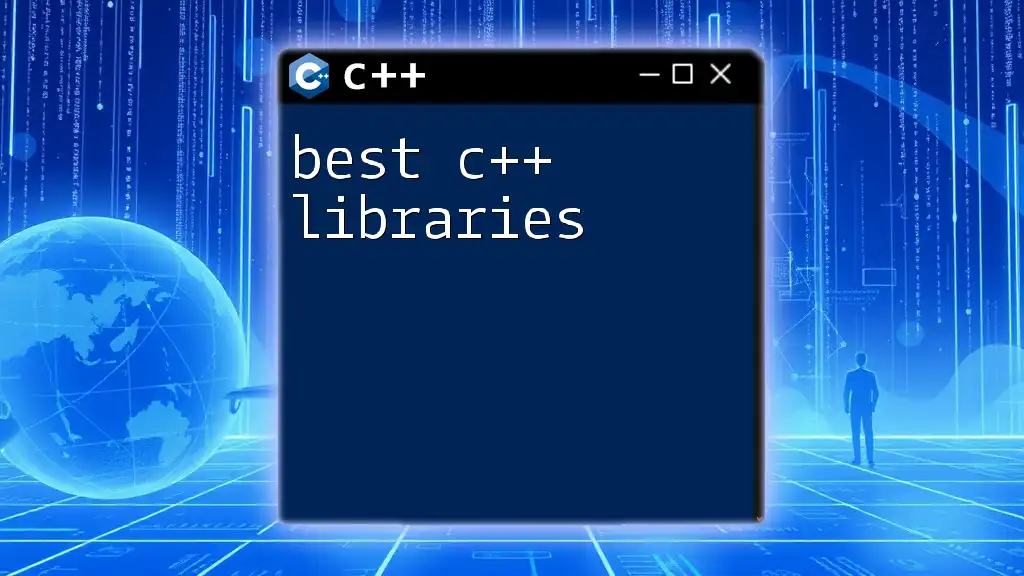
Best Practices in Qt C++ GUI Development
Code Organization
Keeping your code organized is vital, especially for larger projects. Adopt modular programming principles by separating functionalities into distinct classes and files. Follow naming conventions for clarity, and always comment your code for better readability.
Performance Considerations
Performance in a GUI application impacts user experience significantly. To enhance performance, ensure efficient memory management by avoiding frequent allocations and deallocations. Optimize your rendering code to handle complex visual elements smoothly and keep an eye out for unnecessary operations that could slow down the user interface.
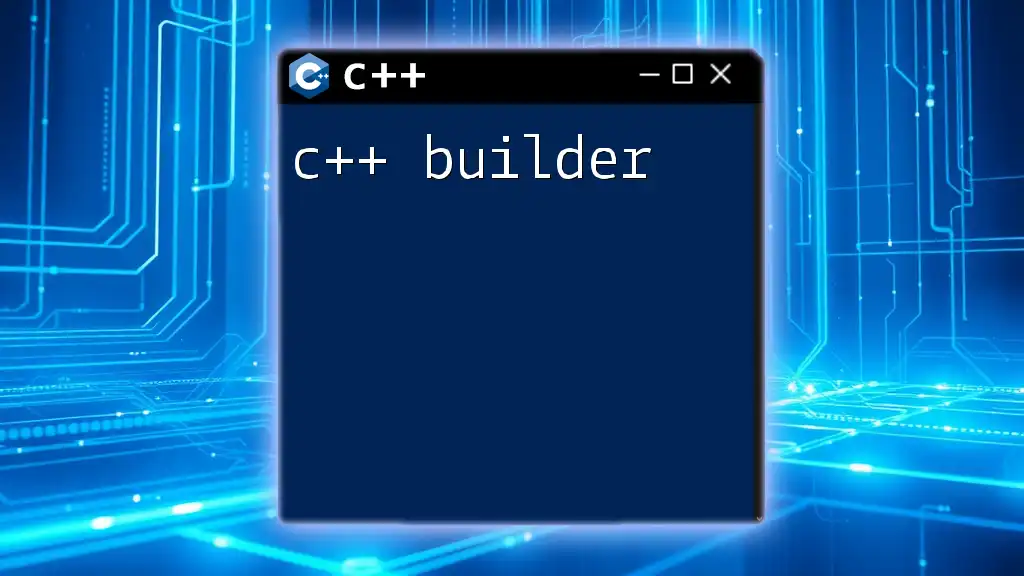
Conclusion
Recap of Key Points
Throughout this guide, we have delved into the essential aspects of developing a Qt C++ GUI application. From understanding the core components like widgets and signals to best practices in code organization and performance optimization, these foundational concepts will enable you to build interactive and user-friendly applications.
Next Steps and Resources
For further learning, consider exploring additional resources such as online tutorials, documentation, and community forums. Engage with other developers to share insights and experiences. Take advantage of the wealth of resources available to enhance your skills in Qt C++ GUI development.
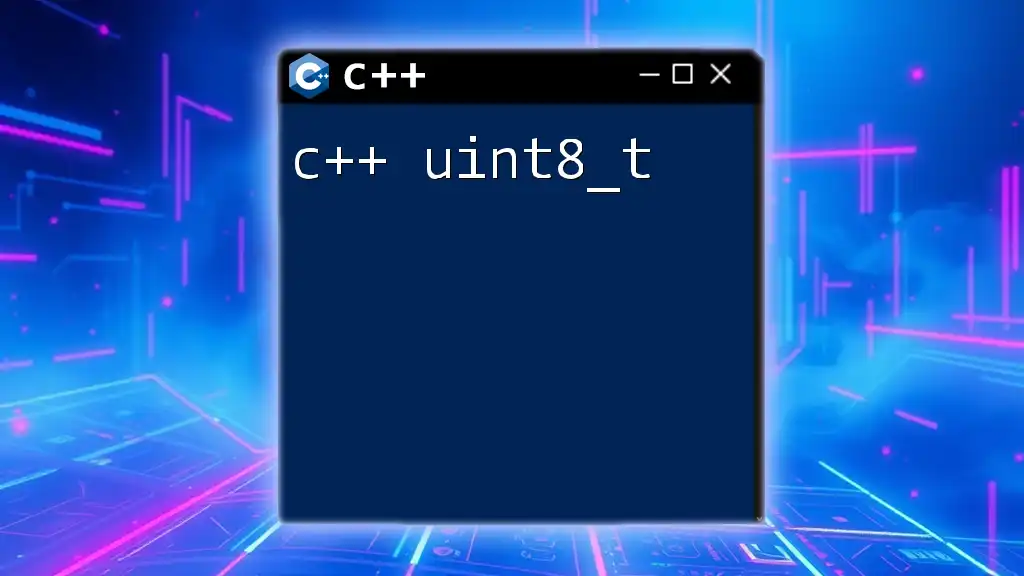
Call to Action
Now that you have a comprehensive overview of Qt C++ GUI development, it's time to get started! Create your own applications using the principles discussed and share your progress with the community. Engage in discussions, ask questions, and continue building your expertise in this exciting field!