C++ GUI cross-platform development allows you to create graphical user interfaces that can run on multiple operating systems using libraries like Qt or wxWidgets.
Here’s a simple example using the Qt framework to create a basic window:
#include <QApplication>
#include <QWidget>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QWidget window;
window.resize(320, 240);
window.setWindowTitle("Hello, Qt!");
window.show();
return app.exec();
}
What is a C++ GUI?
A Graphical User Interface (GUI) is a critical component of modern software applications that allows users to interact with the program through visual elements such as buttons, menus, and icons. Instead of typing commands, users can click and manipulate visual objects, making software more accessible to a broader audience.
In the context of C++, using a GUI framework enables developers to create applications that are not only functional but also user-friendly. Some popular GUI frameworks for C++ include Qt, wxWidgets, and GTKmm, each offering unique capabilities and features to enhance the user experience.
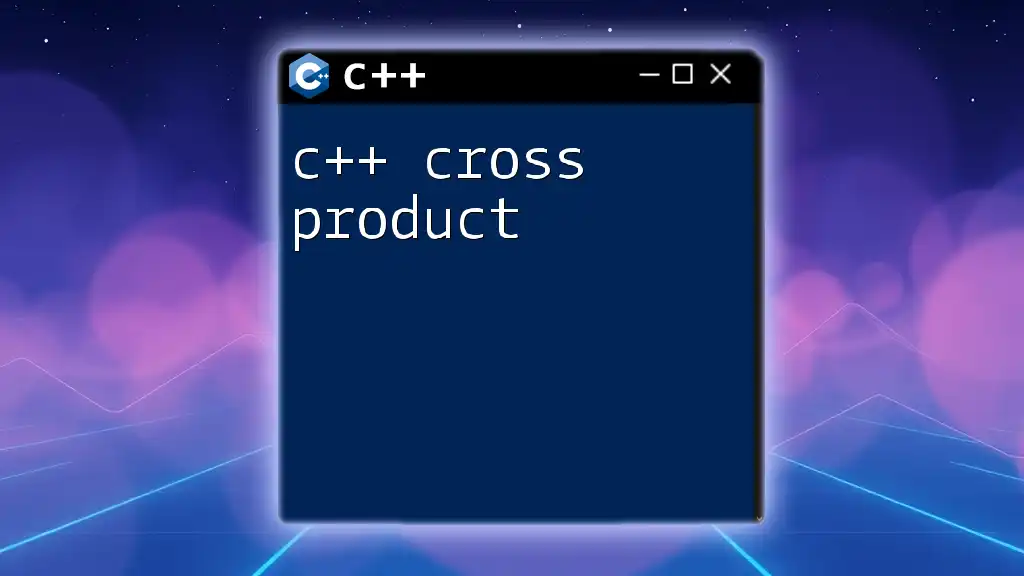
Why Choose Cross-Platform GUI Development?
Benefits of Cross-Platform Development
The primary advantage of cross-platform GUI development is the ability to write once and run everywhere. This efficiency leads to significantly reduced development time and costs since you don’t need to rewrite your code for every operating system. Additionally, your audience expands as your application can target various platforms, from Windows to macOS to Linux.
Another vital benefit is the faster development cycle. By using a single codebase, developers can implement features and fixes more quickly. Furthermore, this approach can lead to a more cohesive user experience across different platforms, enhancing brand consistency.
Challenges in Cross-Platform Development
Despite its benefits, cross-platform development poses certain challenges. Performance might differ significantly between platforms due to varying hardware configurations and operating system behavior. Furthermore, maintaining UI consistency could be tricky because different platforms have distinctive design guidelines and user expectations.
Managing dependencies can also complicate the process, particularly since not all libraries are available or function the same way across different systems. Developers must often deal with the nuances of each platform.
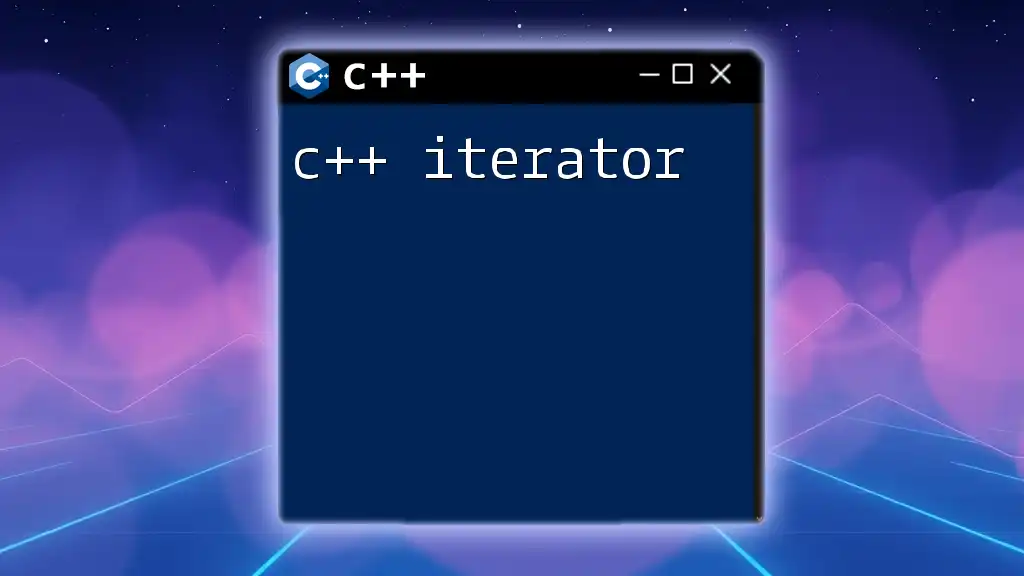
Popular Cross-Platform C++ GUI Frameworks
Qt
Qt is one of the most widely-used frameworks for cross-platform GUI development in C++. It boasts an extensive feature set that includes everything from widgets and layouts to internationalization and network capabilities.
One of the significant advantages of Qt is its support for signals and slots, a powerful way to connect different parts of a program and respond to events.
To create a simple GUI application using Qt, consider the following code snippet:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, world!");
button.resize(200, 100);
button.show();
return app.exec();
}
In this example, the application creates a basic window with a button labeled "Hello, world!" When the button is clicked, the application becomes responsive through the event loop managed by `app.exec()`.
wxWidgets
wxWidgets is another robust C++ framework that simplifies cross-platform development. Known for its native look and feel, wxWidgets allows developers to create applications that look like they belong on the operating systems they target.
Here’s how you can build a basic application with wxWidgets:
#include <wx/wx.h>
class MyApp : public wxApp {
public:
virtual bool OnInit();
};
class MyFrame : public wxFrame {
public:
MyFrame();
};
bool MyApp::OnInit() {
MyFrame *frame = new MyFrame();
frame->Show(true);
return true;
}
MyFrame::MyFrame() : wxFrame(nullptr, "Hello World", wxPoint(50, 50), wxSize(450, 340)) {}
wxIMPLEMENT_APP(MyApp);
In this code, we define an application with a frame that simply displays "Hello World." The application is initialized in the `OnInit` method, which sets up the main frame.
GTKmm
GTKmm is the official C++ interface for the popular GTK+ toolkit used mainly for the GNOME desktop environment. GTKmm provides a modern, object-oriented approach to GUI programming.
To create a simple GTKmm application, use the following code:
#include <gtkmm.h>
class MyWindow : public Gtk::Window {
public:
MyWindow();
};
MyWindow::MyWindow() {
set_title("Hello World");
set_default_size(400, 200);
}
int main(int argc, char *argv[]) {
auto app = Gtk::Application::create(argc, argv, "org.gtkmm.example");
MyWindow window;
return app->run(window);
}
This example creates a window titled "Hello World." The GTKmm framework takes care of the event loop with `app->run(window);`, allowing the application to remain responsive.
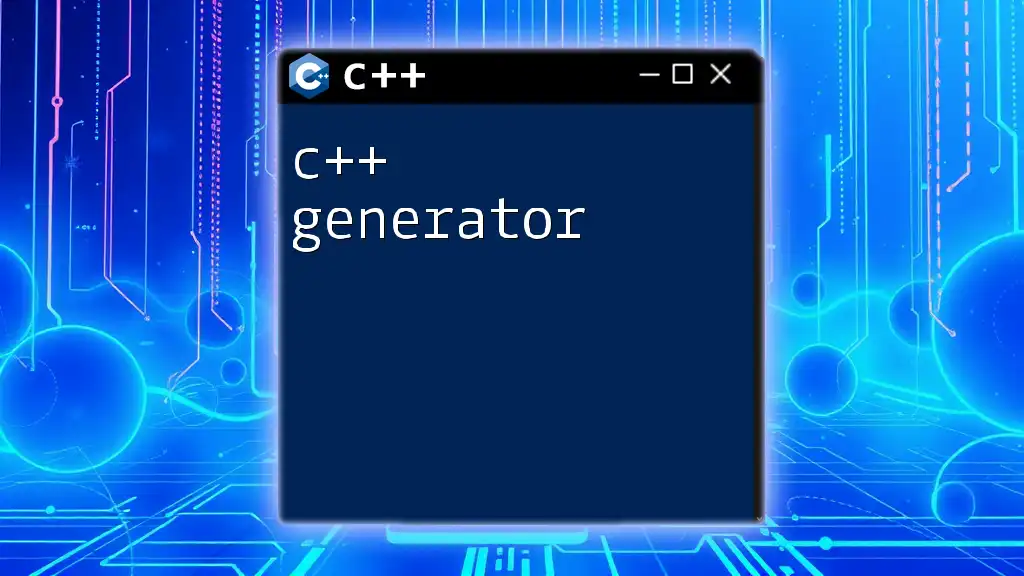
Choosing the Right Framework for Your Project
When selecting a framework for your C++ GUI cross-platform application, consider several factors:
- Target platforms: Different frameworks excel on different operating systems. Ensure the framework supports all the platforms you intend to target.
- Community support: A strong community can provide invaluable resources such as documentation, forums, and third-party libraries.
- Learning curve: Some frameworks are more beginner-friendly than others. Evaluate your team's experience level with GUI programming.
For instance, Qt is known for its extensive documentation and well-defined structure, making it a preferable choice for both beginners and experienced developers. Conversely, wxWidgets offers some unique features that may be appealing for specific projects.
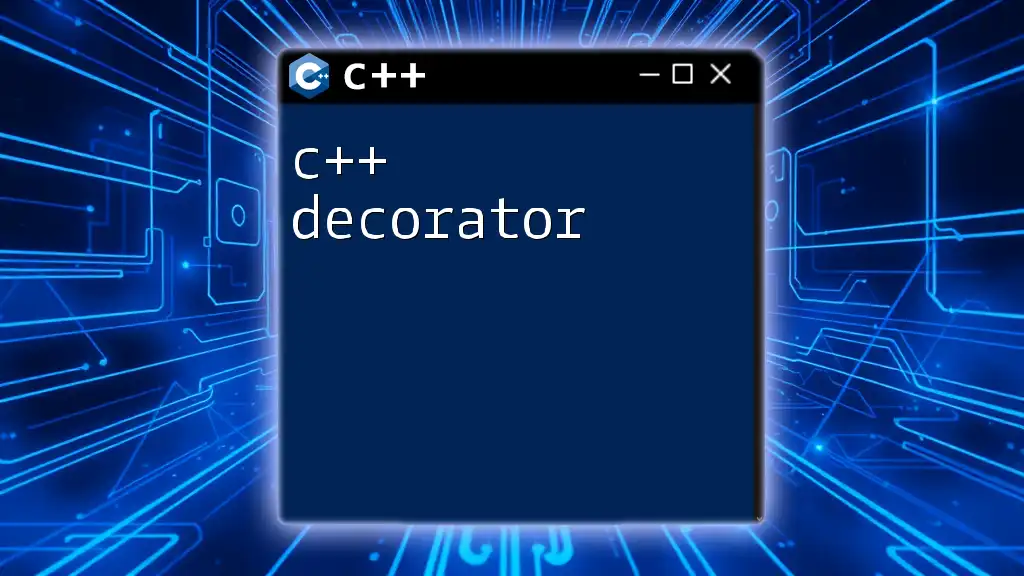
Best Practices for Cross-Platform GUI Development
Consistency Across Platforms
Maintaining a uniform look and feel across different platforms is essential for enhancing user experience. This can be achieved by adhering to common design principles and using shared libraries effectively. Opt for a consistent color scheme, typography, and layout to ensure that users feel comfortable regardless of their platform of choice.
Handling Platform-Specific Features
In cross-platform development, it’s not uncommon to encounter platform-specific functionalities. To manage these discrepancies, utilize preprocessor directives that enable you to conditionally compile code for different platforms. For instance:
#ifdef _WIN32
// Windows-specific code
#elif __APPLE__
// macOS-specific code
#else
// Linux, Unix-specific code
#endif
This approach allows you to isolate platform-specific code and keep your main codebase clean and maintainable.
Performance Optimization
To maintain optimal performance across different platforms, it’s crucial to profile and test your application regularly. Use profiling tools to identify bottlenecks and performance issues. Performance might vary based on the framework you choose; therefore, test your application on all targeted platforms to ensure it meets the necessary performance standards.
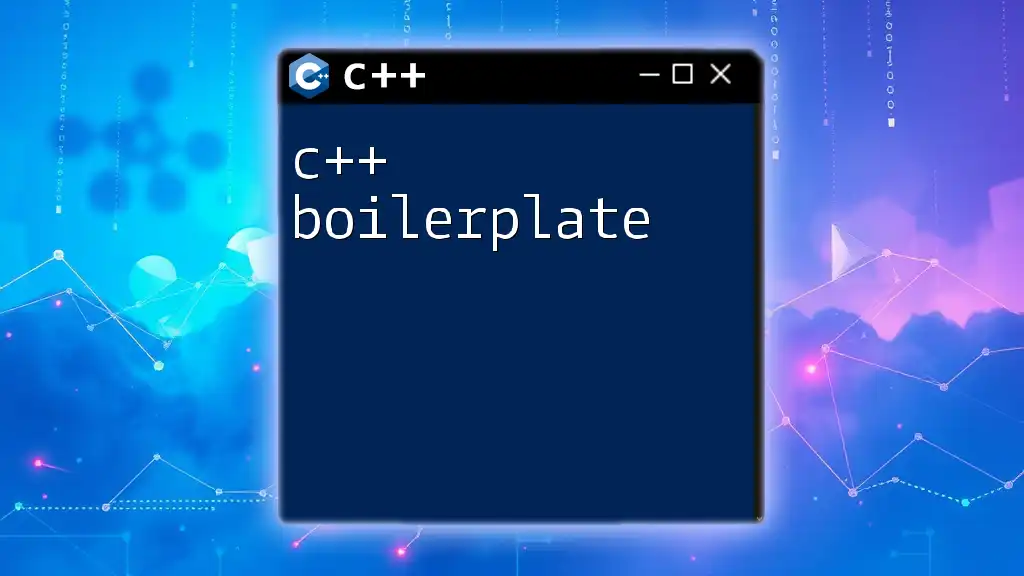
Conclusion
Developing a C++ GUI cross platform application opens many possibilities, allowing you to reach a wider audience while streamlining the development process. By selecting the right framework, adhering to best practices, and understanding the challenges involved, you can create a robust and user-friendly application that works seamlessly across various operating systems.
For those interested in diving deeper into the world of C++ development, exploring different frameworks, and optimizing for best practices, the journey can be both rewarding and enlightening. Embrace the challenges and let your creativity flourish in creating compelling cross-platform applications!
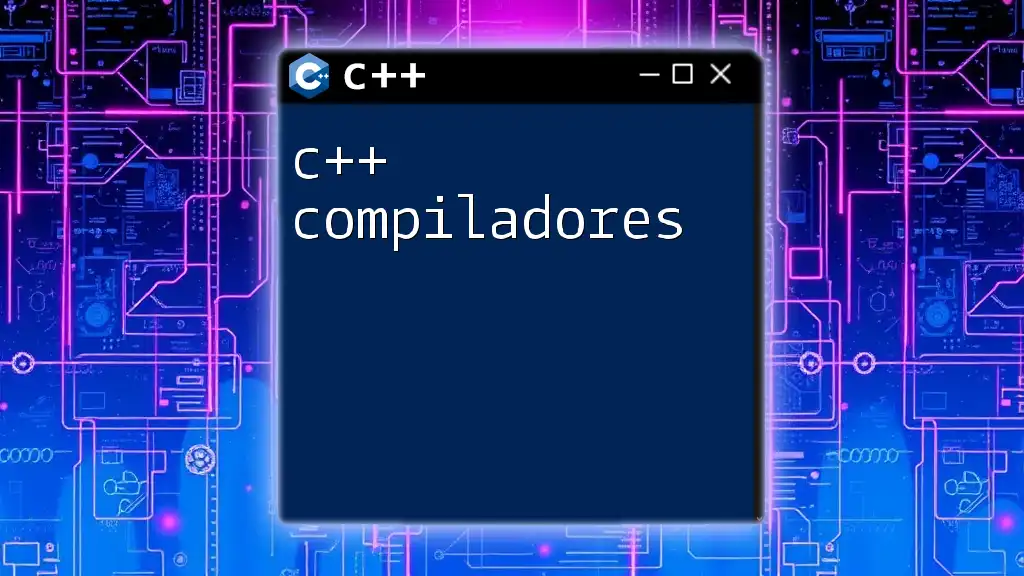
Additional Resources
For further learning, consider engaging with recommended books, websites, and community forums dedicated to C++ and GUI development. These resources can provide valuable insights and assistance as you navigate your coding projects.
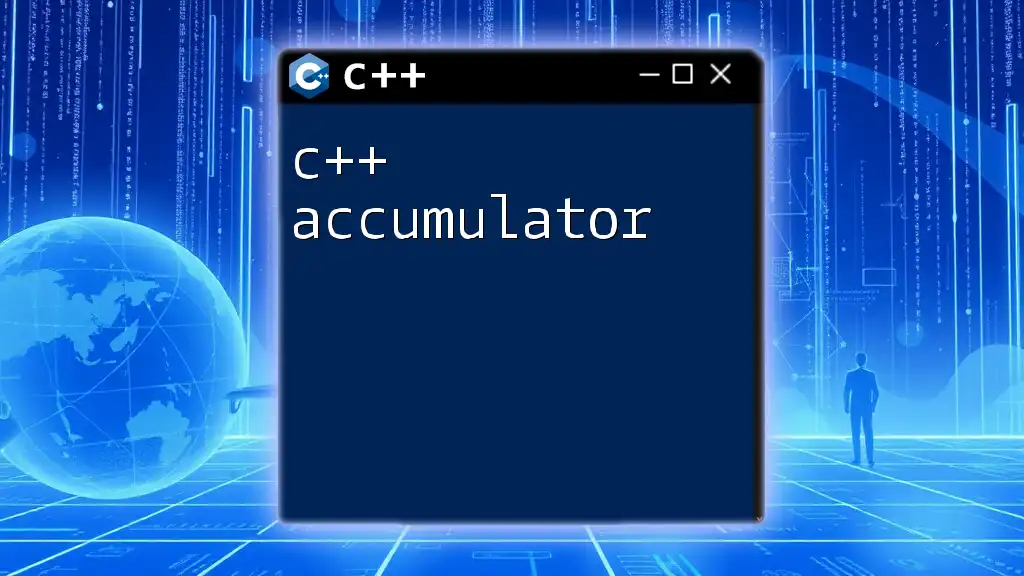
Call to Action
We’d love to hear your experiences or questions related to cross-platform GUI development in C++. Please share your thoughts in the comments below, and if you found this article helpful, consider sharing it with your network. Happy coding!