The "power of 2" in C++ refers to calculating and verifying if a number is a power of 2 using bitwise operations, which can be both efficient and concise.
#include <iostream>
bool isPowerOfTwo(int n) {
return n > 0 && (n & (n - 1)) == 0;
}
int main() {
int num = 16;
std::cout << num << (isPowerOfTwo(num) ? " is a power of 2." : " is not a power of 2.") << std::endl;
return 0;
}
Understanding Powers of Two
Definition of Powers of Two
Powers of two are numerical values represented as \( 2^n \), where \( n \) is a non-negative integer. The sequence starts with \( 2^0 = 1 \), followed by \( 2^1 = 2 \), \( 2^2 = 4 \), \( 2^3 = 8 \), \( 2^4 = 16 \), and so forth. Each exponentiation signifies a doubling effect, making this concept pivotal in many areas of computer science and engineering. Understanding powers of two not only helps in mathematical computations but is also crucial for optimizing algorithms and data structures.
Importance of Powers of Two in Computing
In computing, binary systems form the foundation of how data is processed and stored. Each bit represents a power of two. Therefore, numbers and data types that are aligned to powers of two facilitate more efficient data handling. For instance, allocating memory in sizes like 256MB or 512MB ensures better performance, as most hardware operates optimally with data sizes that are powers of two.
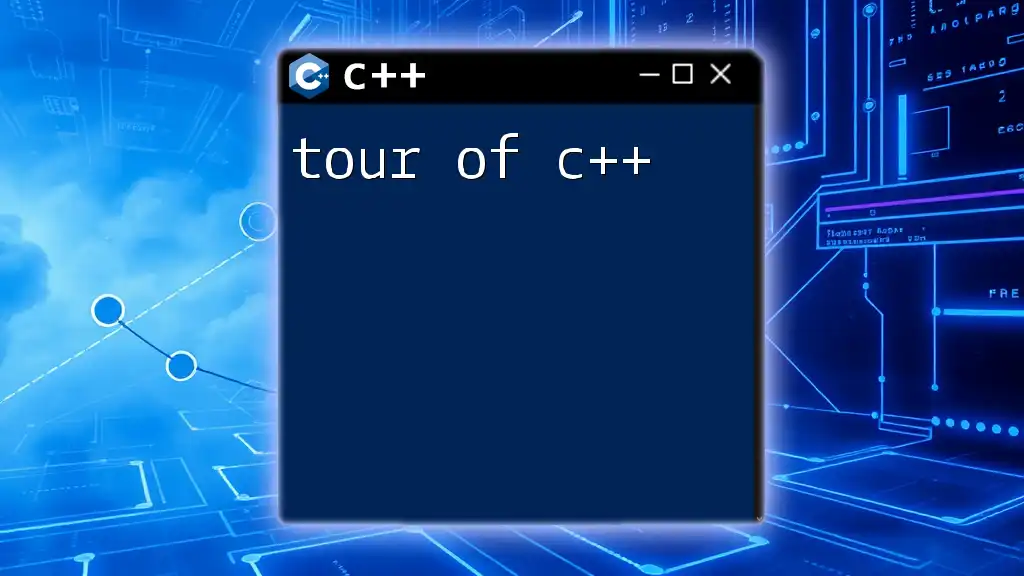
Calculating Power of 2 in C++
Using the `pow` Function
The simplest way to compute powers of two in C++ is by using the `pow` function from the `<cmath>` library. This function allows you to raise any base to a specified exponent. The syntax is straightforward:
#include <iostream>
#include <cmath>
int main() {
int exponent = 5;
double result = pow(2, exponent);
std::cout << "2^" << exponent << " = " << result << std::endl;
return 0;
}
In this example, we raise 2 to the power of 5, resulting in 32. Although this method is easy to understand, it is essential to recognize that using `pow` can sometimes lead to performance issues, particularly if the exponent is large or if you are performing the calculation repeatedly in a loop.
Using Bit Shifting for Power of 2
An efficient alternative to the `pow` function for calculating powers of two is bit manipulation. By utilizing the left shift operator (`<<`), we can efficiently compute \( 2^n \). This approach takes advantage of the binary representation of numbers. Here’s how it works:
#include <iostream>
int main() {
int exponent = 4;
int result = 1 << exponent; // Left shift operator
std::cout << "2^" << exponent << " = " << result << std::endl;
return 0;
}
In this code snippet, shifting the number 1 left by 4 bits gives us the same outcome as \( 2^4 \), which is 16. This method is computationally efficient and avoids the overhead involved with function calls.
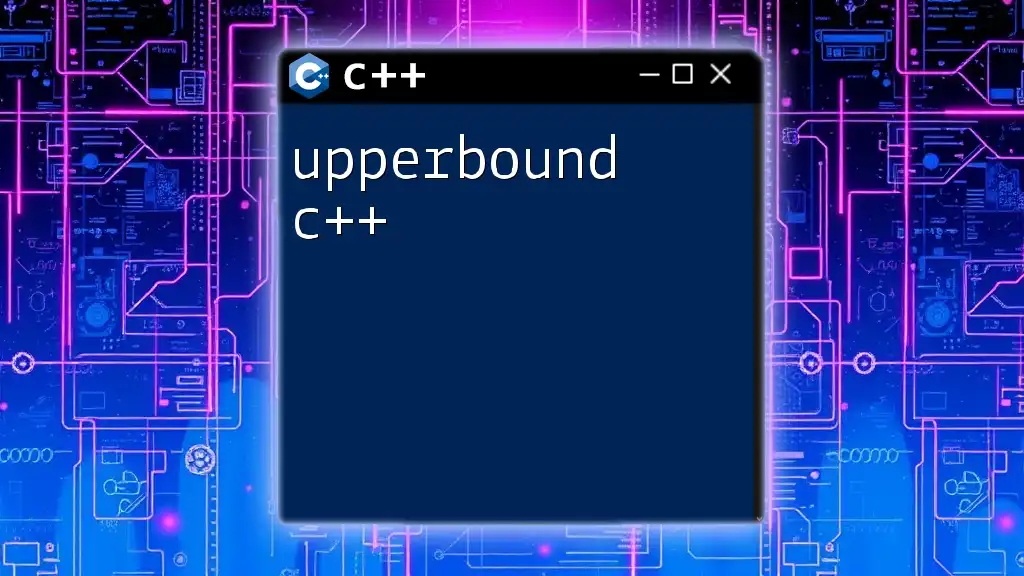
Checking if a Number is a Power of 2
Theoretical Background
Identifying whether a number is a power of two can have significant implications in various algorithms and data structures. A number \( n \) is a power of two if it has exactly one bit set in its binary representation. This property allows for quick checks without looping through all possible powers.
Implementing a Function to Check
To implement a function that checks if a number is a power of two, you can use the following approach, utilizing bit manipulation:
#include <iostream>
bool isPowerOfTwo(int n) {
return (n > 0) && (n & (n - 1)) == 0; // Using bit manipulation
}
int main() {
int number = 16;
if(isPowerOfTwo(number)) {
std::cout << number << " is a power of 2." << std::endl;
} else {
std::cout << number << " is not a power of 2." << std::endl;
}
return 0;
}
In this example, the function checks if \( n \) is greater than zero and determines if \( n \& (n - 1) \) equals zero. This clever technique efficiently verifies if a number is a power of two in constant time.
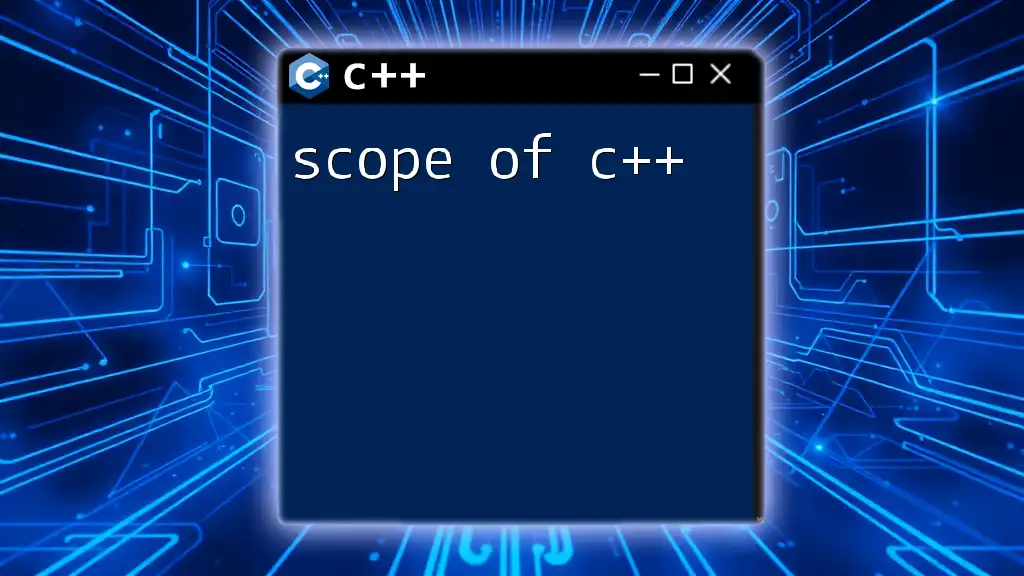
Practical Applications of Powers of Two
Memory Allocation
In practical scenarios, particularly in systems programming, memory allocation often hinges on powers of two. For example, hardware is frequently optimized for addressing memory in sizes like 256, 512, or 1024 bytes. Using memory allocations based on powers of two minimizes fragmentation and enhances cache performance.
Data Structures and Algorithms
Powers of two can lead to improved time complexity in algorithms. For instance, in implementing binary heaps, the array size often adheres to powers of two, allowing for efficient parent-child relationships. Furthermore, search algorithms that rely on binary trees benefit from the structural nature of powers of two.
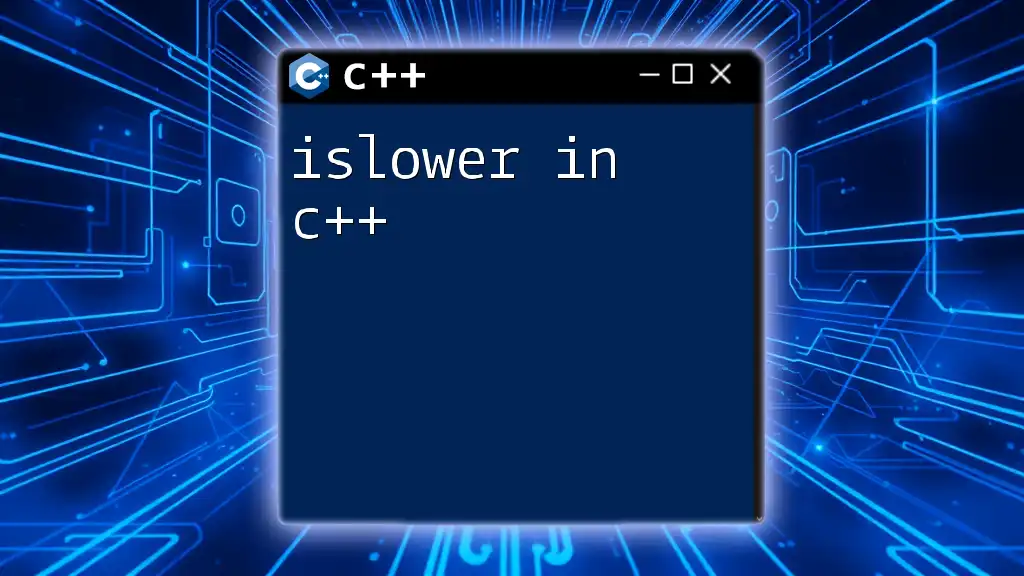
Common Pitfalls and Tips
Integer Overflow
While the `pow` function is convenient, it can lead to integer overflow when high exponent values are used. This overflow can cause unexpected behaviors in applications, particularly in critical systems. As a best practice, always consider the value range and data type usage to avoid overflow situations.
Performance Considerations
When comparing methods, bit manipulation generally outperforms the `pow` function in terms of speed and efficiency. When optimizing performance, understanding when to use one approach over another can significantly impact your program’s responsiveness and efficiency.
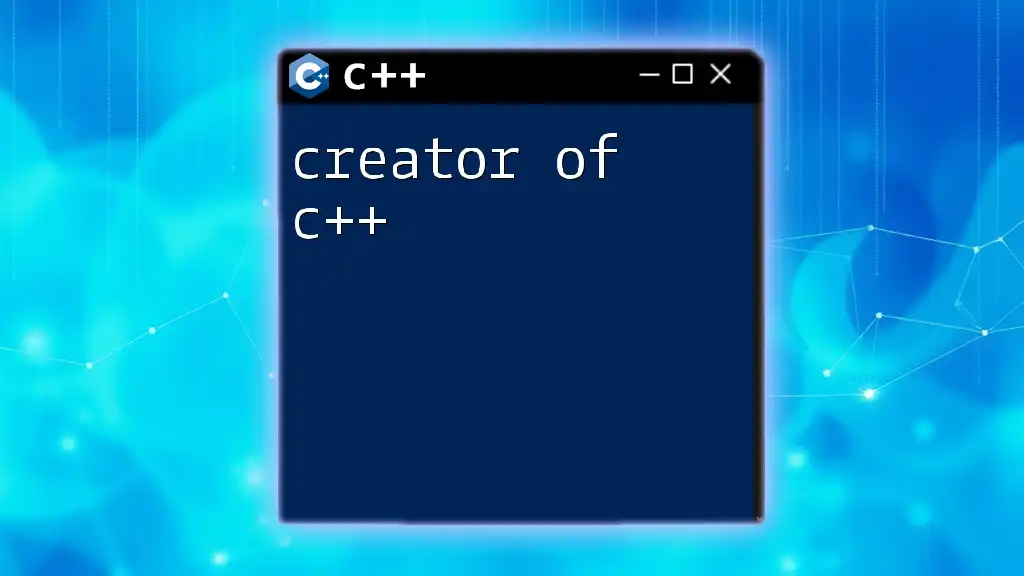
Conclusion
Understanding the power of 2 in C++ offers countless benefits, from efficient computations to enhanced memory management. By mastering how to calculate powers of two, check if a number is a power of two, and apply these principles in programming, you can significantly improve your coding skills and contribute effectively to performance-critical applications. Embrace the versatility of powers of two and practice implementing these strategies to elevate your programming skills!
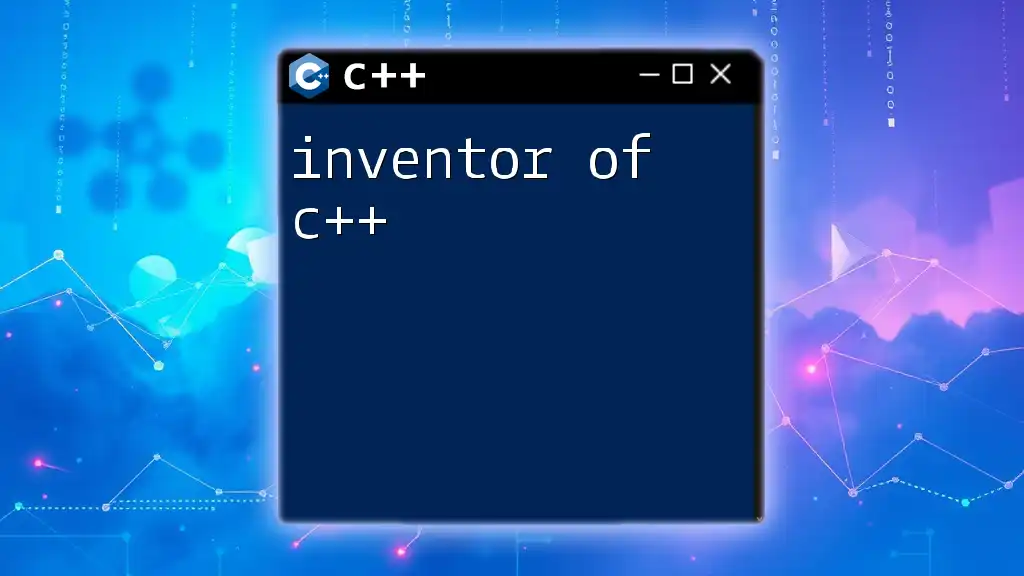
Additional Resources
For further learning on this topic, consult resources like C++ documentation, online programming tutorials, and coding challenge platforms that focus on data structures and algorithms related to powers of two. These tools will deepen your understanding and provide practical applications of the concepts discussed in this article.