The "linker command failed with exit code 1" error in C++ typically occurs when the linker cannot find a definition for a declared function or variable, indicating an issue with unresolved symbols during the linking stage.
// Example of a definition missing for a declared function
#include <iostream>
void myFunction(); // Declaration without definition
int main() {
myFunction();
return 0;
}
What is a Linker?
In the realm of C++, a linker is a crucial tool that combines various pieces of code and data into a single executable file. This process follows the compilation stage, where individual source files are translated into object files. The linker resolves references between these object files, allowing them to work in concert within the final program. Understanding the linker's role helps developers diagnose issues that may arise, such as the “linker command failed with exit code 1 c++” error.
What Does Exit Code 1 Mean?
Exit codes are integers returned by processes to signify their completion status. An exit code of 1 typically indicates a general error has occurred during the execution of a program. In the context of linking, this often means the linker encountered issues that prevented it from successfully creating the executable. Common triggers for exit code 1 may include missing symbols, duplicate definitions, or misconfigured build settings.
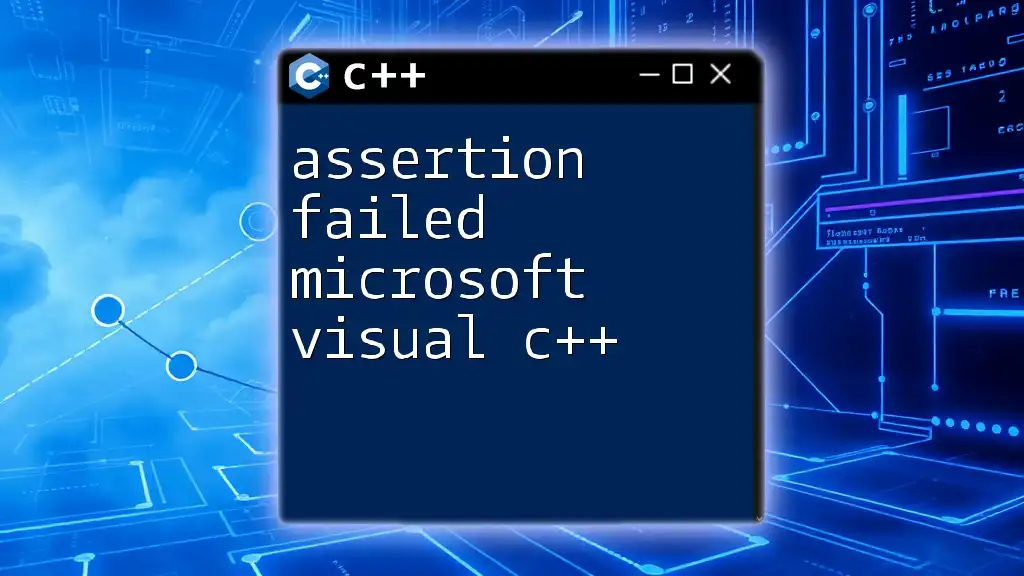
Common Causes of the "Linker Command Failed with Exit Code 1" Error
Missing Symbols
Missing symbols occur when the linker cannot find a definition for a referred function or variable in the object files being processed. This leads to linker errors as the linker is unable to resolve references necessary for code execution.
Example: Undefined Reference
Consider the following code snippet:
void exampleFunction();
int main() {
exampleFunction(); // If exampleFunction is not defined, this causes a linker error
return 0;
}
In this case, since `exampleFunction` lacks a corresponding definition, attempting to link the program will produce an error indicating an undefined reference. To resolve this, ensure that all declared functions or variables have proper definitions.
Duplicate Symbols
Having duplicate symbols is another frequent cause of linker errors. When two or more object files declare the same global variables or functions, the linker becomes confused, as it cannot ascertain which definition to use.
Example: Multiple Definition
// File1.cpp
int var = 5;
// File2.cpp
int var = 10; // Causes duplicate symbol error when both files are linked
In the above example, `var` is defined in both `File1.cpp` and `File2.cpp`. When linking these files together, the linker throws an error about duplicate symbols. One solution is to use the `extern` keyword in header files to define the variable only once.
Incorrect Build Configuration
Linker errors can also arise due to inconsistent build configurations across different modules. If the flags used for compiling and linking differ, this can lead to unresolved references or incompatible object files.
Example: Using Different Compiler Flags
g++ -Wall -o program1 File1.cpp
g++ -o program2 File2.cpp // Missing the -Wall flag might lead to inconsistent behavior
Inconsistent flags might not directly cause a link error but can result in varied behavior among compiled files, complicating the linking process. Always ensure consistent settings across your project's modules.
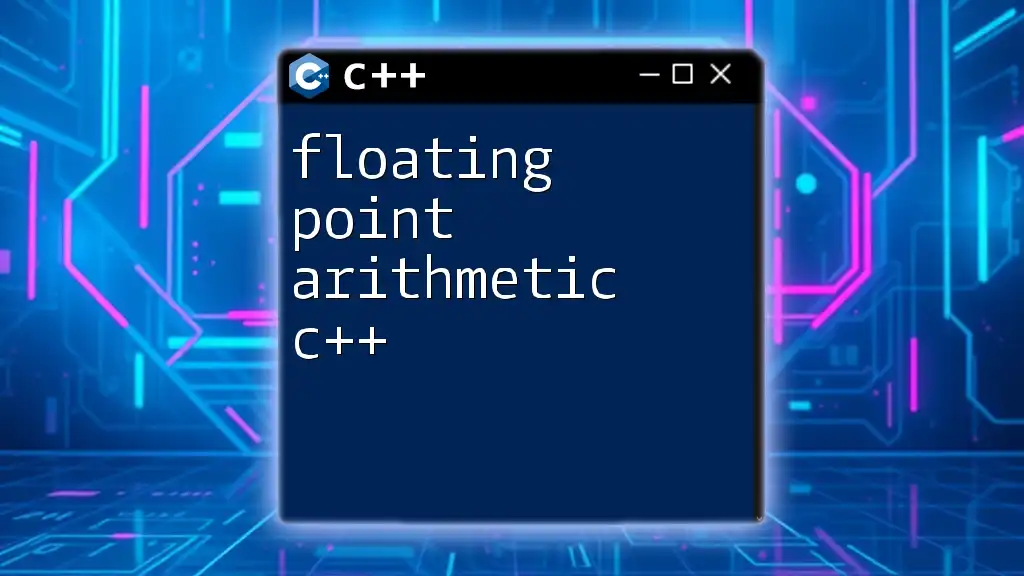
Steps to Troubleshoot Linker Errors
Check Your Build Output
Always begin by examining the build output closely. Linker errors often contain messages that point directly to the issue at hand. Understanding these messages can significantly streamline troubleshooting efforts.
Review Your Code
Take a moment to check your code for signs of missing or duplicate symbols. Ensure all function declarations have corresponding definitions. Additionally, inspect for any file mistakenly included multiple times.
Verify Dependencies
Make sure all required libraries are included in your build configuration. Missing or incorrectly specified libraries often results in linker errors that can halt compilation. Validate that all library paths are correctly set.
Clean and Rebuild Your Project
Performing a clean build can help eliminate obsolete files that lead to linker issues. Most IDEs provide an option to clean the build, which removes object files and forces the project to rebuild from scratch, potentially resolving lingering linker errors.
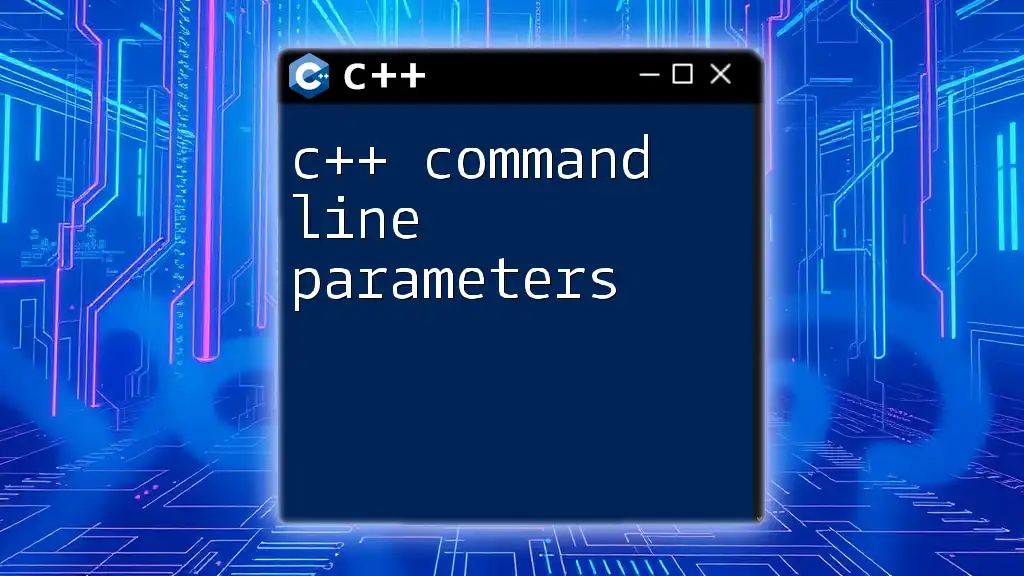
Solutions to Common Linker Errors
Resolving Missing Symbols
To tackle missing symbols, locate the function or variable causing the issue and ensure it is defined properly. If a function is declared in a header but missing in a source file, be sure to implement it.
Fixing Duplicate Symbols
To resolve issues with duplicate symbols, centralize definitions to avoid overlap. Use the `extern` keyword wisely to indicate shared variables.
Example: Using `extern`
// Header.h
extern int var;
// File1.cpp
#include "Header.h"
int var = 5;
// File2.cpp
#include "Header.h" // Includes declaration to avoid duplicate definition
In this example, `var` is defined in `File1.cpp` and declared with `extern` in `Header.h`, preventing a duplicate symbol error.
Adjusting Build Configuration
Ensure your build configuration settings are uniform across the project. If you're using an IDE, it should provide options to review and adjust compiler settings easily. In command-line compilations, maintain consistent flags to avoid conflicts.
Using Linker Options
Employ linker options, such as `-L` for specifying library paths and `-l` for naming libraries, to resolve linking issues caused by missing references.
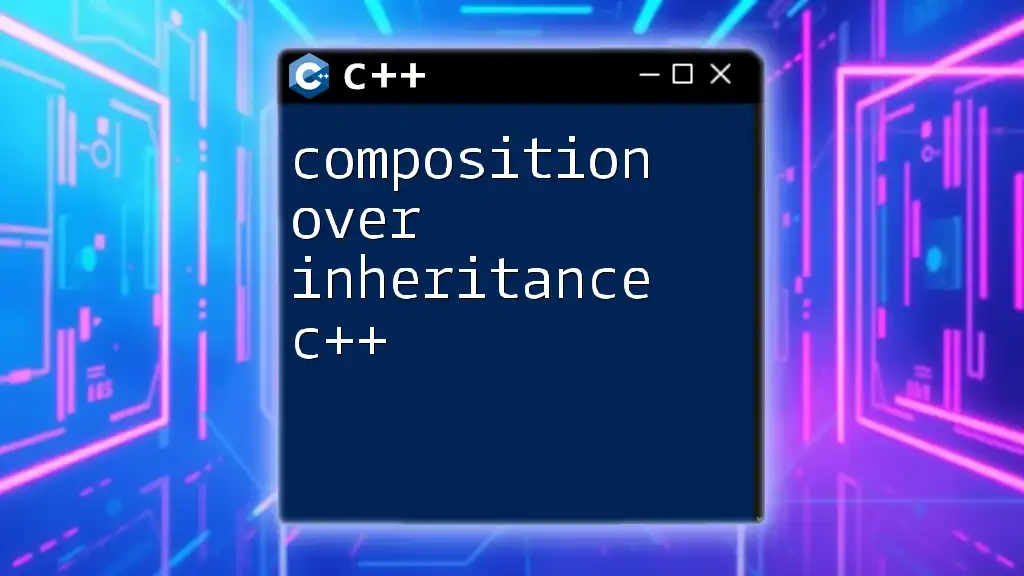
Real-World Examples and Scenarios
Case Study: Fixing a Common Linker Error
Imagine a scenario where you are attempting to link two object files, `File1.o` and `File2.o`, but encounter the "linker command failed with exit code 1" error due to a missing function. A detailed examination of both files reveals that a function declared in `File1.o` is never defined. Resolving this by providing a definition eliminates the error, showing the importance of careful coding practices.
Experiencing Linker Issues in Large Projects
Linker issues often multiply in larger codebases due to numerous interdependencies. To mitigate this, utilize a build system like CMake to manage dependencies effectively. This allows automatic resolution of linking paths and makes it easier to maintain a consistent build environment.
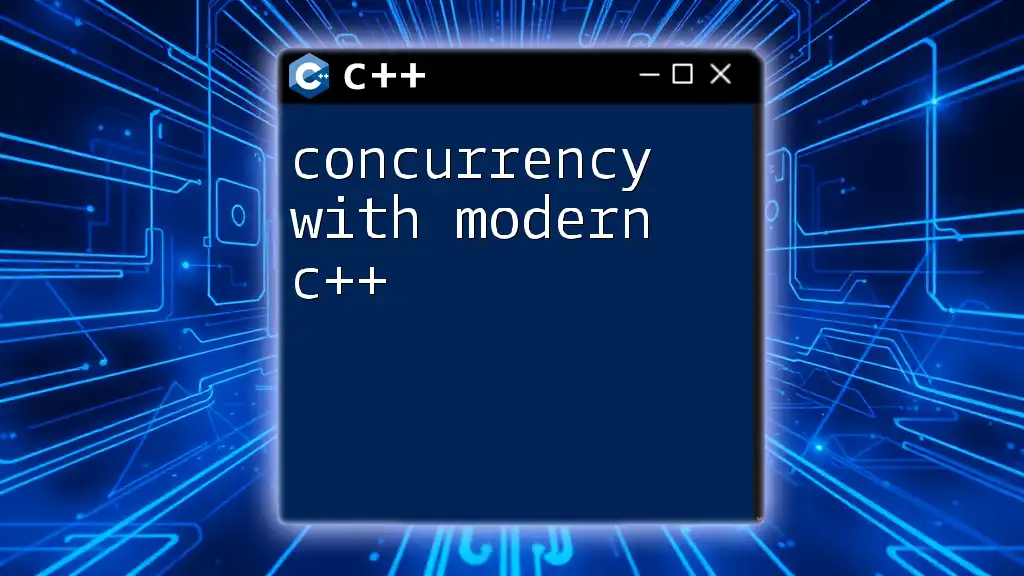
Conclusion
Understanding the nuances of linker errors can greatly enhance your productivity as a C++ developer. By familiarizing yourself with common causes, troubleshooting effectively, and implementing robust coding practices, you can prevent the daunting "linker command failed with exit code 1 c++" error from disrupting your workflow.
Additional Resources
For further reading on overcoming linker errors and enhancing your C++ knowledge, explore comprehensive guides and resources specific to your environment. Consider leveraging online forums and communities where you can ask questions and share insights with fellow developers.
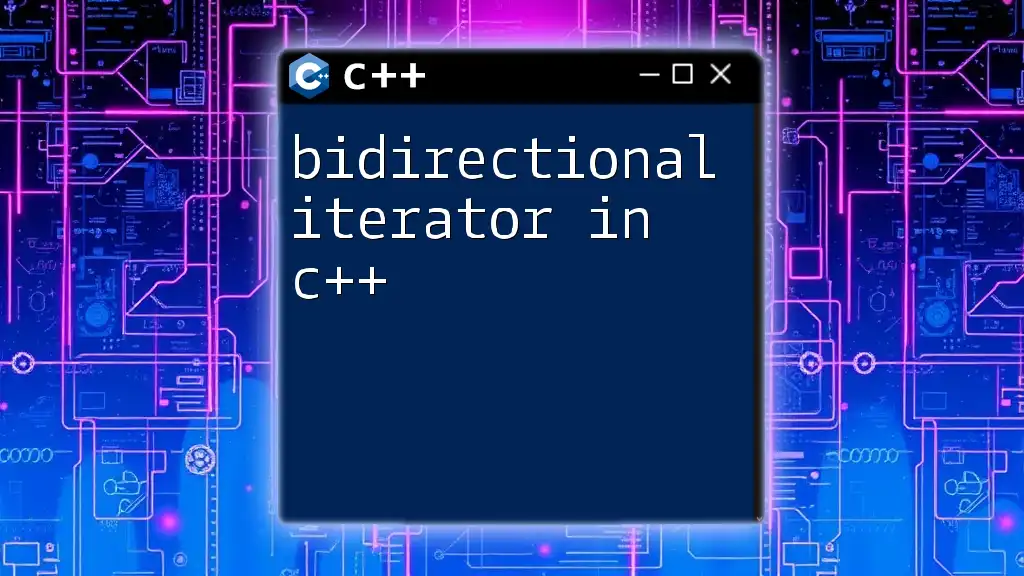
FAQs About Linker Errors in C++
What if I still can't resolve my linker error?
If the error persists despite troubleshooting, consider seeking community help or consulting documentation related to your IDE or compiler.
Can IDEs help in resolving these issues automatically?
Many modern IDEs provide automatic dependency resolution, linking, and flag management features that simplify the process and reduce human error.
How does the linker differ across various platforms?
Linkers may vary in behavior depending on the platform and the tools used. Always refer to platform-specific documentation for nuances in linking behavior and options available.