A buffer overrun in C++ occurs when data exceeds the allocated memory buffer, potentially leading to unexpected behavior or vulnerabilities by overwriting adjacent memory.
Here's a simple example of a buffer overrun:
#include <iostream>
#include <cstring>
int main() {
char buffer[10];
std::strcpy(buffer, "This string is too long for the buffer!"); // Buffer overrun
std::cout << buffer << std::endl;
return 0;
}
What is a Buffer Overrun?
A buffer overrun occurs when a program writes more data to a block of memory, or buffer, than it was allocated for. Buffers are temporary storage locations in memory. When data is written to a buffer, there is a specified limit on how much data can be stored. An overrun happens when data exceeds this limit, which can corrupt adjacent memory, leading to erratic behavior or security vulnerabilities.
How Buffer Overruns Occur
Buffer overruns often stem from misunderstandings of memory management principles in C++. In C++, arrays have a fixed size defined at compile time, meaning developers need to be mindful of their limits while writing code. When insufficient consideration is given to how much data will be written to a buffer, overruns can easily happen.

Common Causes of Buffer Overruns in C++
Insufficient Buffer Size
A frequent cause of buffer overruns is providing an insufficient buffer size. When a developer fails to account for the length of the data being processed, it can lead to excess data being written beyond allocated memory.
Consider this example:
char buffer[10];
strcpy(buffer, "This string is too long for the buffer");
In this case, the buffer is only 10 bytes, but the string written to it exceeds this size. This can lead to undefined behavior, with the program potentially overwriting critical data.
Poor Input Validation
Input validation is crucial for securing a program against errors and vulnerabilities. When user input is not validated, a malicious user could attempt to input excessive data, which can lead to a buffer overrun.
Look at the following code:
std::string userInput;
std::cin >> userInput; // No validation, could lead to overflow
Without validation checks, a user can enter an overly long string, leading to potential overruns.
Improper Use of Functions
Functions that do not automatically check buffer sizes are also frequent culprits behind buffer overruns. For example, functions like `strcpy` and `strcat` can easily lead to unsafe scenarios if developers are unaware of the destination buffer's size.
Take this example:
char src[] = "Source";
char dest[5];
strcpy(dest, src); // Unsafe
Here, `src` has more characters than `dest` can accommodate, which will result in a buffer overrun.
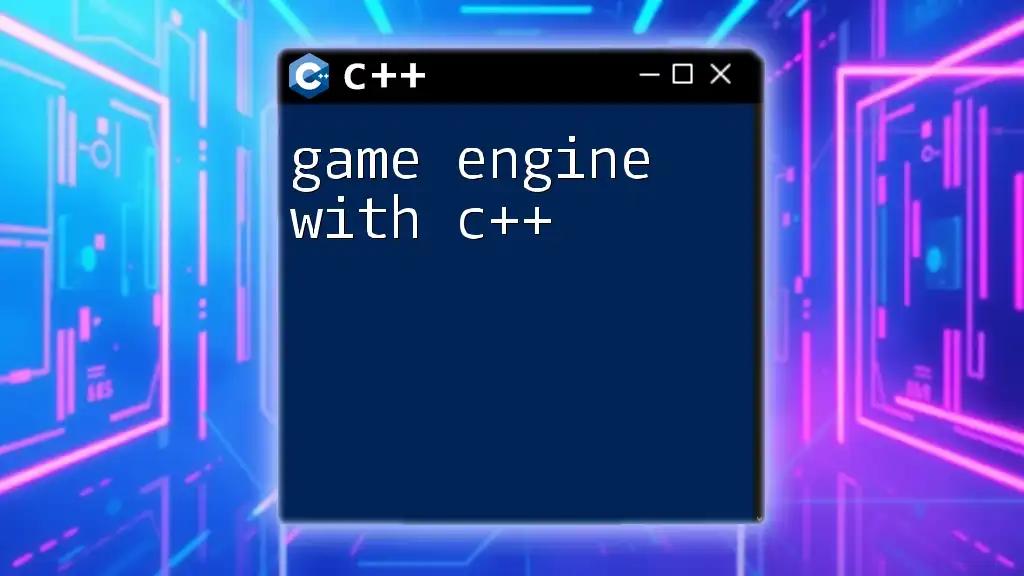
Security Implications
Real-World Examples of Buffer Overruns
Several notorious security vulnerabilities arose from buffer overruns. Notable examples include the Code Red Worm and the Melissa Virus, which exploited these weaknesses to infect systems and deliver malicious payloads. Such events underscore how crucial it is to understand and mitigate buffer overrun issues.
How Attackers Exploit Buffer Overruns
Attackers often exploit buffer overruns to inject arbitrary code into a system. One common technique known as stack smashing allows attackers to overwrite the return address in a stack frame, redirecting the code execution flow to malicious code. This can compromise the security of an entire system.

Preventing Buffer Overruns in C++
Proper Use of Standard Libraries
Using safer alternatives from the C++ Standard Library can significantly reduce the risk of buffer overruns. Consider using `std::string` instead of character arrays, as it handles memory management automatically.
Here’s an example:
std::string safeBuffer;
std::cin >> safeBuffer; // Automatically manages size
By leveraging `std::string`, developers can avoid many common pitfalls associated with manual memory management.
Implementing Input Validation Techniques
Preventative programming techniques, such as input validation, are essential to maintain code integrity. Implementing checks to limit input length can eliminate unsafe input scenarios.
For instance, consider the following code:
std::string userInput;
std::cin >> userInput;
if (userInput.size() > 10) {
std::cout << "Input too long!" << std::endl;
}
In this example, the program checks user input length and responds appropriately if it exceeds a safe limit.
Using Compiler Warnings and Static Analysis
Compiler warnings can alert developers to potential issues before runtime. By enabling warnings with commands such as:
g++ -Wall -Wextra yourfile.cpp
developers can catch unsafe practices early in the development process. Additionally, using static analysis tools further identifies potentially unsafe constructs in the code.

Debugging Buffer Overruns
Tools for Detecting Buffer Overruns
Effective debugging tools are essential for identifying buffer overruns. Tools like Valgrind and AddressSanitizer are invaluable for memory debugging and can automatically pinpoint memory management issues.
For instance, running a program with Valgrind’s memory checker can yield valuable insights into overrun errors, allowing developers to fix them proactively.
Analyzing Crash Dumps
When a program crashes due to a buffer overrun, analyzing crash dumps can offer insights into what went wrong. Developers should look for specific patterns erring toward memory manipulation and buffer management.

Conclusion
Buffer overruns while writing to C++ code represent a significant risk that can lead to security breaches and erratic program behavior. Understanding their causes, prevention methods, and debugging techniques is crucial for producing safe and effective C++ applications. By adopting these best practices and being vigilant about memory management, developers can greatly reduce the risk of buffer overruns in their code. For those keen to learn more about C++ safety, exploring further resources and courses can enhance your knowledge and coding proficiency.