The error "was not declared in this scope" in C++ occurs when you attempt to use a variable or function that has not been defined or is out of the current scope, commonly resulting from missing declarations or typos.
Here’s an example that demonstrates this error:
#include <iostream>
int main() {
std::cout << myVariable; // Error: 'myVariable' was not declared in this scope
return 0;
}
What Does "Was Not Declared in This Scope" Mean?
The error message "was not declared in this scope" in C++ typically indicates that the compiler has encountered a variable, function, or identifier that it does not recognize in the current context. This common error can stem from various issues related to scope, which broadly refers to the visibility and lifetime of variables and functions in a program. Understanding this error is crucial because it helps programmers pinpoint issues and improves code reliability.

Common Causes of the Error
Variables Not Declared
One of the most frequent sources of the "was not declared in this scope" error is using a variable without declaring it first. In C++, every variable must be declared before it’s used in the code to inform the compiler about its type and location in memory.
For example:
#include <iostream>
int main() {
std::cout << myVariable; // Error: 'myVariable' was not declared in this scope.
return 0;
}
In this case, the variable `myVariable` was referenced before it was declared. To resolve this issue, simply declare the variable before you use it, like this:
int myVariable = 10;
Functions Not Declared
Another common cause of this error arises when functions are called before they are declared. In C++, it's essential to declare functions ahead of their invocation to inform the compiler about their existence.
For instance:
#include <iostream>
int main() {
myFunction(); // Error: 'myFunction' was not declared in this scope.
return 0;
}
void myFunction() {
std::cout << "Hello, World!";
}
Here, the `myFunction()` is called in `main()` before it’s declared. To fix this error, you can either move the function definition above its usage or provide a forward declaration, like so:
void myFunction();
int main() {
myFunction();
return 0;
}
Incorrect Namespace Usage
Namespaces in C++ help avoid name collisions. If you’re calling a function or variable that exists in a different namespace, failing to specify the correct namespace can also lead to a scope error.
For example:
#include <iostream>
int main() {
printHello(); // Error: 'printHello' was not declared in this scope.
return 0;
}
void printHello() {
std::cout << "Hello!";
}
Here, if `printHello` is defined in a different namespace and not specified in the `main` function, the compiler raises an error. You can fix this by either using the `using` directive or specifying the namespace directly if applicable.
Misnamed Identifiers
Typos or naming inconsistencies can also lead to this error. C++ is case-sensitive, so even minor differences in names can cause confusion.
Consider this example:
#include <iostream>
int main() {
int myNumber = 5;
std::cout << myNuber; // Error: 'myNuber' was not declared in this scope.
return 0;
}
In this code, `myNuber` is a typo, which causes the compiler to complain because it does not recognize it. Always double-check spelling to avoid this pitfall.
Conditional Compilation Issues
When using preprocessor directives such as `#ifdef` and `#ifdef`, you may encounter the "was not declared in this scope" error if the conditional compilation logic prevents certain functions or variables from being compiled.
For example:
#include <iostream>
#define FEATURE_ENABLED
int main() {
#ifdef FEATURE_ENABLED
std::cout << featureFunction(); // Error: 'featureFunction' was not declared in this scope.
#endif
return 0;
}
void featureFunction() {
std::cout << "Feature is enabled!";
}
In this case, if `featureFunction()` is defined outside the `#ifdef` block, the compiler won’t recognize it. Make sure that the relevant functions are defined within the blocks where they're expected to be used.
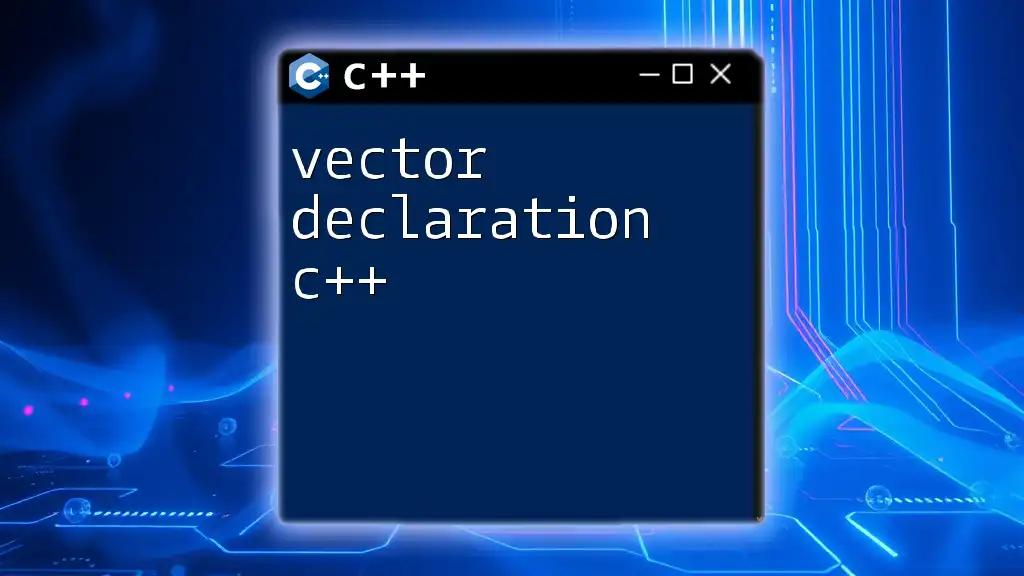
Best Practices to Avoid Scope Errors
Always Declare Before Use
The best way to avoid the "was not declared in this scope" error is simply to ensure that you declare all variables and functions before using them. This practice is fundamental to the clarity and functionality of your code, eliminating ambiguity.
Use Consistent Naming Conventions
Maintaining a consistent naming style reduces the possibility of errors due to typos or variable misrecognition. Adopt descriptive names for your variables and functions to clarify their purpose, enhancing overall code readability.
Modular Code Organization
Divide your code into smaller modules by using header files (`.hpp` or `.h`). This organizational method makes it easier to manage variable and function scopes effectively and can help prevent scope errors.
Leverage Compiler Warnings
Compilers like g++ offer warning flags that can help identify potential issues before they manifest as errors. Use the `-Wall` flag to turn on all warning messages, giving you insights into any problems with undeclared identifiers:
g++ -Wall myFile.cpp
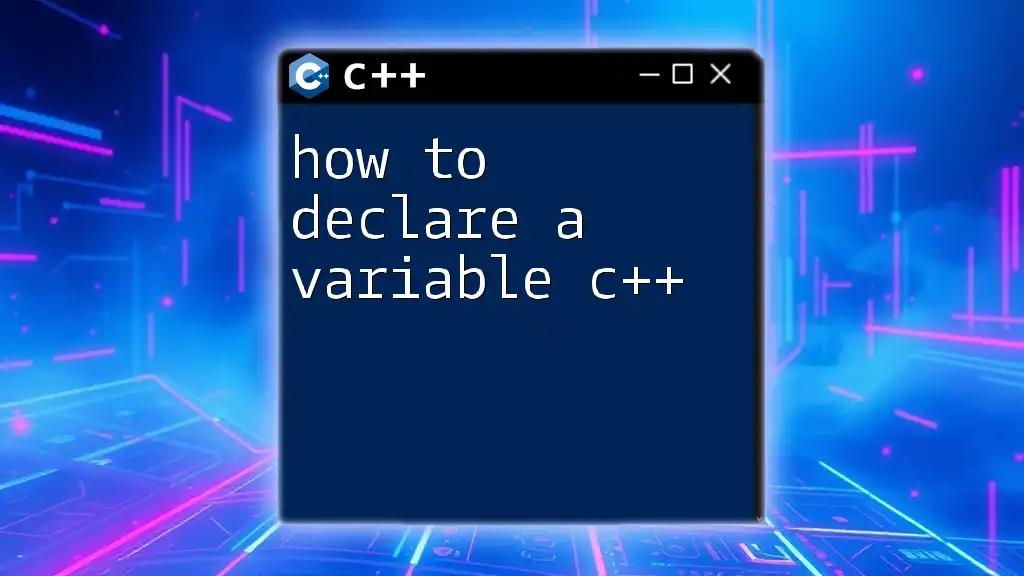
Conclusion
The "was not declared in this scope" error is a common yet solvable issue in C++ programming that emphasizes the importance of proper variable and function declarations, naming conventions, and awareness of scope. By understanding the causes of this error and applying best practices, you can avoid such pitfalls and improve your coding efficiency. Continue to practice and experiment with different C++ elements, ensuring that you gain a deeper and more effective understanding of scope in your programming journey.

Additional Resources
To expand your knowledge and tackle C++ efficiently, consider exploring the official C++ documentation, taking courses, and engaging in community forums. Learning from diverse resources will further enhance your programming skills and confidence.