In C++, a relationship between objects can be established using class composition, allowing one class to include objects of another class, which promotes a "has-a" relationship.
class Engine {
public:
void start() {
// Engine starting logic
}
};
class Car {
private:
Engine engine; // Car "has-a" Engine
public:
void startCar() {
engine.start(); // Starting the car by starting the engine
}
};
What are Relationships in C++?
Relationships in C++ define how different classes or objects interact with each other within the framework of object-oriented programming (OOP). Understanding these relationships is crucial for designing software systems that are robust, maintainable, and extendable. In C++, we primarily observe four types of relationships: association, aggregation, composition, and inheritance.
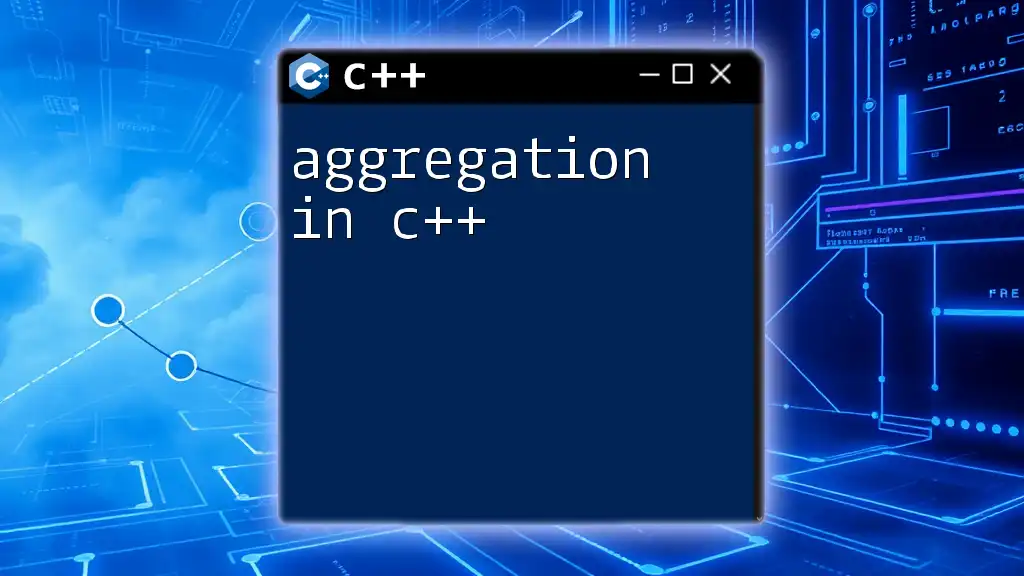
Types of Relationships
Association
Association is a relationship where one class is associated with another. This can be thought of as a "uses-a" relationship, meaning one class uses the functionalities of another but does not own it.
For example, consider the following classes — `Driver` and `Car`:
class Driver {
public:
void drive() { /* driving logic */ }
};
class Car {
private:
Driver *driver;
public:
Car(Driver *d) : driver(d) {}
void operate() { driver->drive(); }
};
In this example, the `Car` class relies on the `Driver` class to perform its operations. However, the `Car` does not own the `Driver`, indicating an association relationship. When creating associations, it's important to keep the classes loosely coupled to enhance flexibility and manageability.
Aggregation
Aggregation represents a "whole-part" relationship. It's stronger than association but still signifies that the contained object can exist independently of the container.
Consider the relationship between a `Car` and its `Engine`:
class Engine {
public:
void start() { /* start engine logic */ }
};
class Car {
private:
Engine *engine; // Car has an Engine
public:
Car(Engine *e) : engine(e) {}
void startCar() { engine->start(); }
};
Here, the `Car` has an `Engine`, but the `Engine` can exist without the `Car`. This illustrates aggregation, wherein the lifetime of `Engine` is not tied to that of `Car`. Using aggregation appropriately promotes clarity in structures, helping with system organization.
Composition
Composition, unlike aggregation, indicates a stronger relationship where the contained objects cannot exist independently of the container. This is often termed as a "has-a" relationship.
For instance, let’s see the relationship between `Car` and `Wheel`:
class Wheel {
public:
Wheel() { /* Wheel creation logic */ }
};
class Car {
private:
Wheel wheel; // Car contains a Wheel (composition)
public:
Car() { /* Car creation logic */ }
};
In this case, the `Car` contains a `Wheel`, and once `Car` is destroyed, the `Wheel` is also destroyed. Composition enforces strong ownership, which can simplify object lifecycle management and enhance encapsulation.
Inheritance
Inheritance facilitates a relationship where one class (the derived class) inherits attributes and behaviors from another class (the base class). This concept promotes reusability and the establishment of hierarchical relationships.
For instance:
class Vehicle {
public:
void start() { /* vehicle starting logic */ }
};
class Car : public Vehicle { // Car inherits from Vehicle
public:
void honk() { /* honk logic */ }
};
The `Car` class inherits the characteristics of `Vehicle`, allowing it to inherit properties and functions. This mechanism reduces code duplication and allows similar classes to share common behaviors, leading to cleaner and more efficient designs.
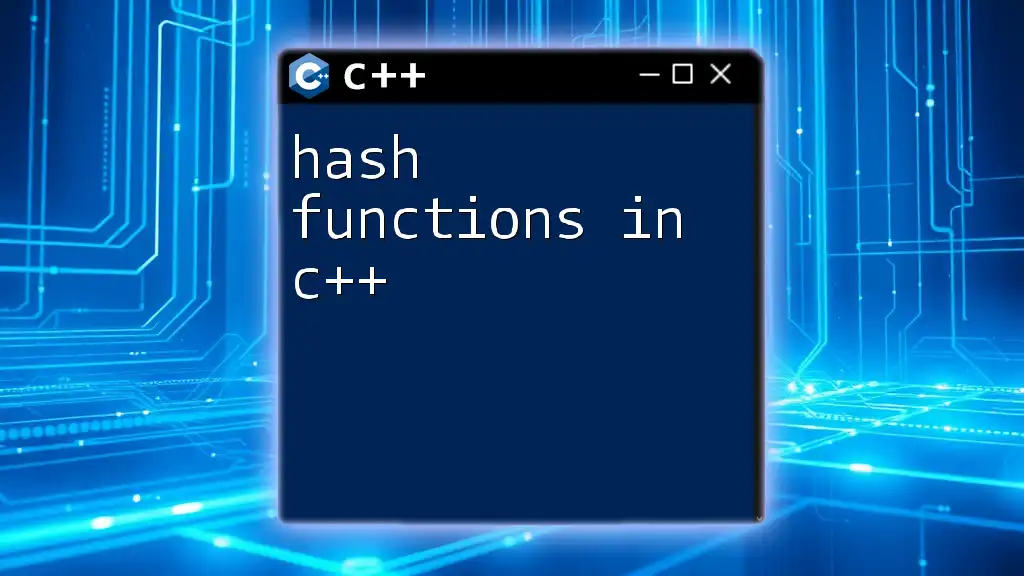
Understanding Polymorphism
Polymorphism is a core tenet of OOP that allows objects to be treated as instances of their parent class. This feature enables a single function or method to operate in different ways, depending on the object that invokes it.
What is Polymorphism?
Polymorphism comes in two forms: function overloading and function overriding.
Function Overloading
With function overloading, multiple functions can have the same name but differ in their parameter types or counts.
class Printer {
public:
void print(int i) { /* print integer logic */ }
void print(double d) { /* print double logic */ }
};
The `Printer` class has two `print` methods differentiated by parameters. This allows for intuitive usage while providing flexibility.
Function Overriding
In contrast, function overriding enables a derived class to provide a specific implementation of a method that is already defined in its base class.
class Animal {
public:
virtual void sound() = 0; // Pure virtual function
};
class Dog : public Animal {
public:
void sound() override { /* bark logic */ }
};
class Cat : public Animal {
public:
void sound() override { /* meow logic */ }
};
With polymorphism, you can work with base class pointers or references that point to derived class objects, allowing dynamic method resolution at runtime. This is powerful for creating flexible and scalable code.
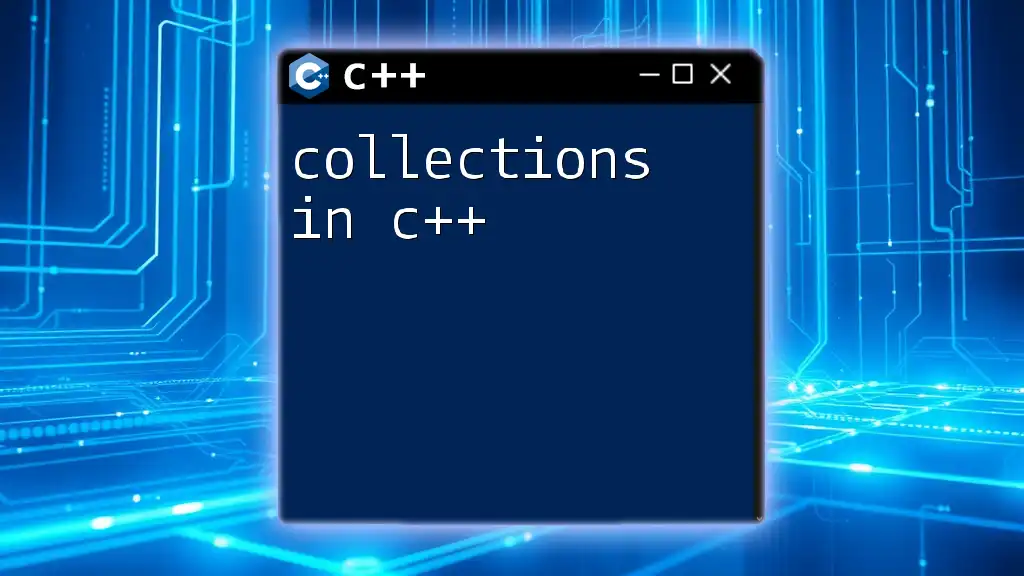
Real-World Examples of Relationships
Let’s examine a practical scenario: a Library Management System. In this case, relationships between `Library`, `Book`, and `Member` can be illustrated through associations and aggregations.
class Book {
// Book properties like title and author
};
class Member {
// Member's properties like name and membership ID
};
class Loan {
Book book; // A loan encompasses a Book
Member member; // A loan is associated with a Member
// Logic for managing the loan period, etc.
};
In this example, the `Loan` class has an aggregation relationship with `Book` and an association relationship with `Member`. Upon closing a loan, the `Loan` system manages returning the book and updating the member’s records without altering the fundamental properties of `Book` or `Member`.
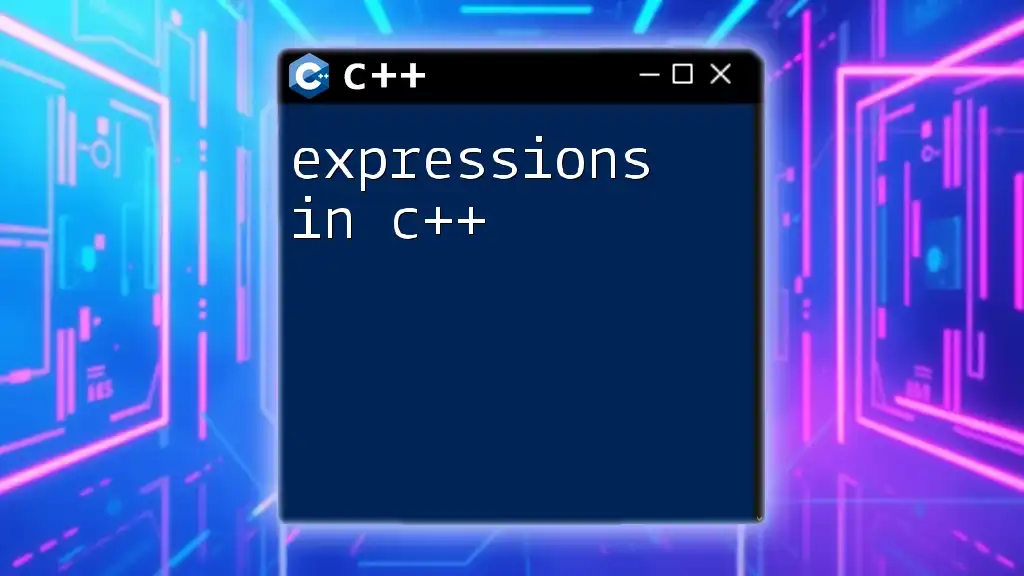
Best Practices in Managing Relationships
Use of Smart Pointers
To avoid the pitfalls of manual memory management in C++, it’s recommended to utilize smart pointers such as `std::shared_ptr` and `std::unique_ptr`. These tools help maintain ownership semantics and prevent memory leaks, enhancing the robustness of relationships within your classes.
Design Patterns to Consider
Adopting design patterns can lead to effective management of relationships. Patterns like Singleton, Factory, or Builder elucidate various ways to structure classes and handle relationships effectively, thereby providing clarity and reusability to your codebase.
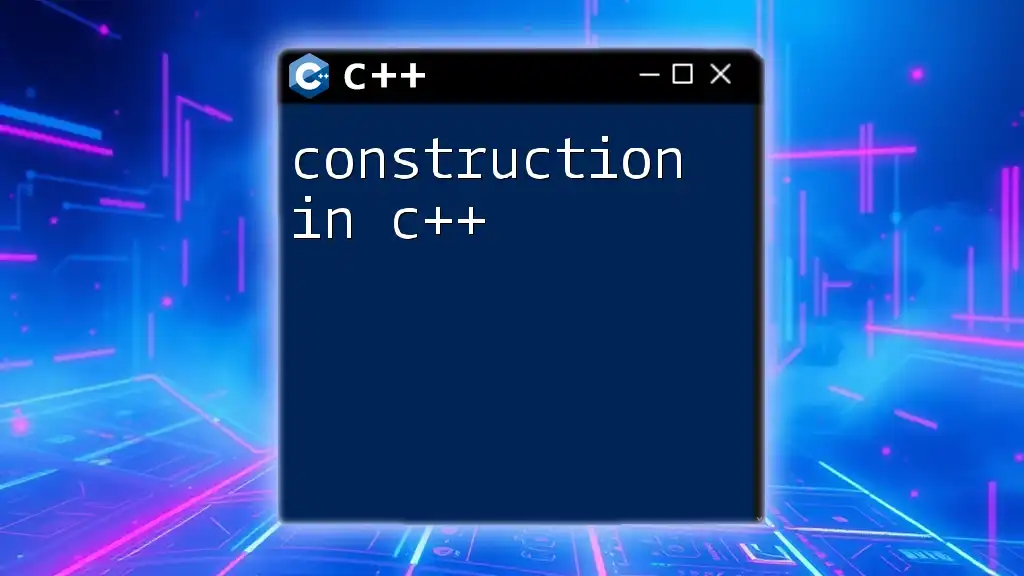
Common Mistakes to Avoid
Tight Coupling
One of the most common mistakes in managing relationships is tight coupling. This occurs when changes in one class induce changes in another, making the system fragile and harder to maintain. Aim for loose coupling that allows each class to operate independently.
Circular Dependencies
Another critical issue is circular dependencies, which arise when two classes depend on each other directly or indirectly. This can lead to complications during compilation and runtime. To avoid this, consider separating interface from implementation and utilizing forward declarations when necessary.
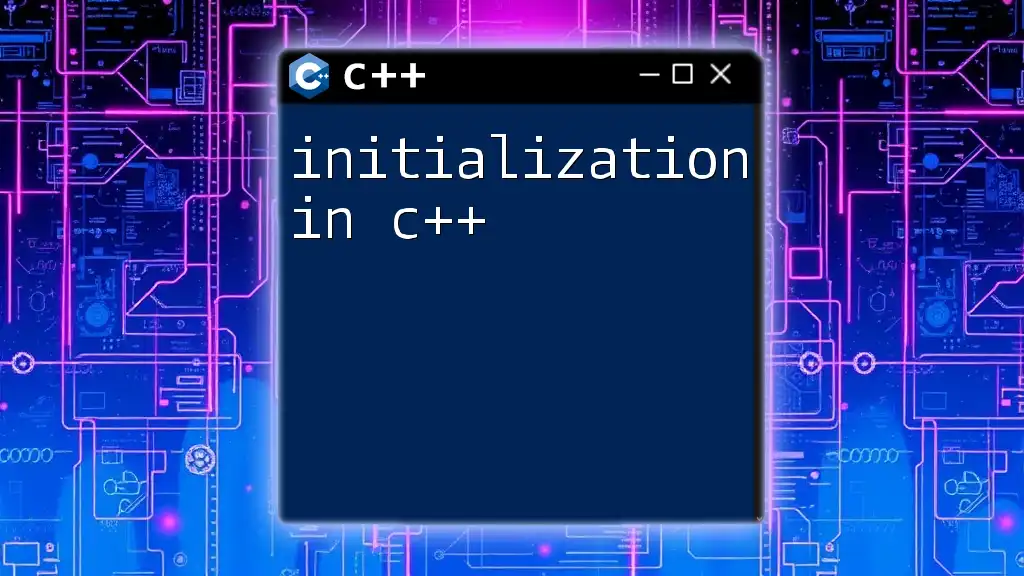
Conclusion
In summary, understanding how classes have a relationship in C++ is vital for effective object-oriented programming. By recognizing the different types of relationships—association, aggregation, composition, and inheritance—you can better manage interactions and interactions in your software systems. As you work with these relationships, integrate practices that enhance clarity and maintainability to create robust C++ applications.