In C++, you can generate a UUID by utilizing the <uuid/uuid.h> header in combination with the `uuid_generate` function, which creates a universally unique identifier.
Here's a code snippet demonstrating how to generate a UUID:
#include <iostream>
#include <uuid/uuid.h>
int main() {
uuid_t uuid;
uuid_generate(uuid);
char uuidString[37];
uuid_unparse(uuid, uuidString);
std::cout << "Generated UUID: " << uuidString << std::endl;
return 0;
}
Understanding UUID
What is a UUID?
A UUID (Universally Unique Identifier) is a 128-bit number used to identify information in computer systems. The primary goal of a UUID is to ensure that unique identifiers are generated, which do not need to be stored in a central database to eliminate duplication. UUIDs are often represented in hexadecimal format separated by hyphens and consist of five groups of hexadecimal digits—which totals 36 characters including the hyphens.
Why Use UUID in C++?
Using UUIDs in C++ provides several advantages over traditional identifiers, such as integers or sequential strings. Primarily, UUIDs are globally unique, meaning they can be generated independently in different locations or systems without any coordination necessary. This property makes them ideal for distributed systems, databases, and even for temporary identifiers in web applications.
Some common use cases include:
- Identifying objects in databases.
- Creating unique session tokens for web applications.
- Marking unique emails or accounts in user management systems.
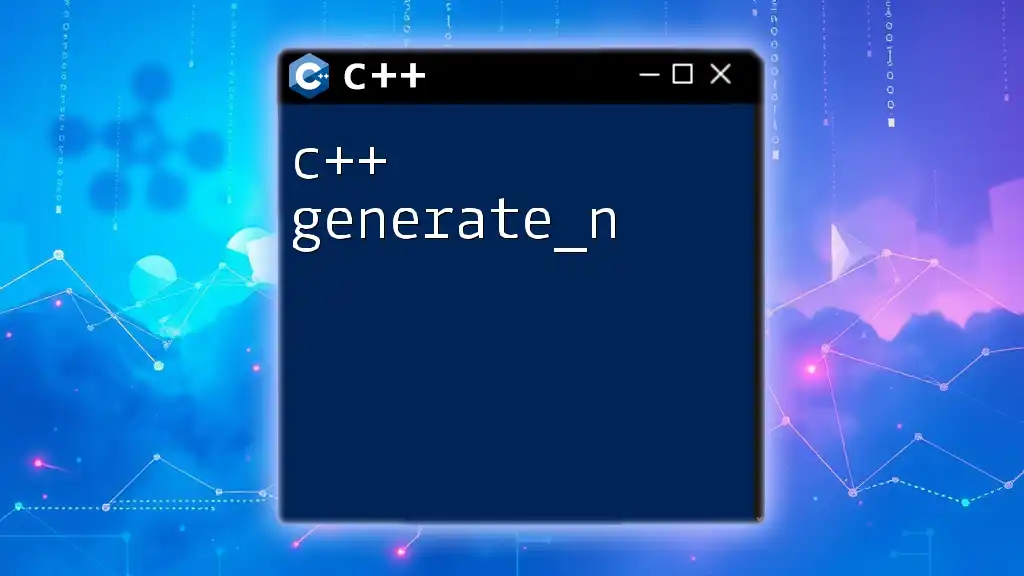
Generating UUID in C++
Overview of C++ UUID Libraries
C++ does not natively support UUID generation out of the box; however, several third-party libraries provide robust solutions. Some of the most recognized libraries include:
- Boost UUID: A powerful and widely-used library that includes UUID generation and manipulation capabilities.
- libuuid: Part of the e2fsprogs package, it is commonly used in Linux systems.
- C++20 std::uuid: A more recent addition to the C++ standard library, it simplifies UUID management if your compiler supports it.
Using Boost UUID Library
Setting Up Boost Library
To utilize the Boost UUID library, you first need to install Boost. You can install Boost via package managers, or by downloading it from [Boost's official website](https://www.boost.org/).
For instance, on Ubuntu, you can use:
sudo apt-get install libboost-all-dev
After installation, ensure you link the library in your C++ project by including the relevant header files and linking against the Boost library in your build configuration.
Basic Example of Generating UUID
Here is a basic code snippet demonstrating how to generate a UUID using Boost:
#include <boost/uuid/uuid.hpp>
#include <boost/uuid/uuid_generators.hpp>
#include <boost/uuid/uuid_io.hpp>
#include <iostream>
int main() {
boost::uuids::uuid my_uuid = boost::uuids::random_generator()();
std::cout << "Generated UUID: " << my_uuid << std::endl;
return 0;
}
In this code, we include necessary Boost headers and create a random UUID using the `random_generator()`. The generated UUID is then outputted to the console. This simple approach effectively illustrates how straightforward it is to generate a UUID in C++ using Boost.
Using C++20 std::uuid (If Applicable)
C++ Standard Library Enhancements
With the introduction of C++20, there is now a standard way to work with UUIDs via the `std::uuid` class, though it's important to check the support of your compiler. If your development environment supports C++20, generating a UUID can be even simpler using:
#include <uuid.h>
#include <iostream>
int main() {
uuid_t id;
uuid_generate_random(id);
char str[37];
uuid_unparse(id, str);
std::cout << "Generated UUID: " << str << std::endl;
return 0;
}
This snippet demonstrates a straightforward method for generating a UUID using `libuuid`. The `uuid_generate_random()` function creates a new UUID, while `uuid_unparse()` formats it into a readable string. Note that you will need the appropriate library linked during compilation.
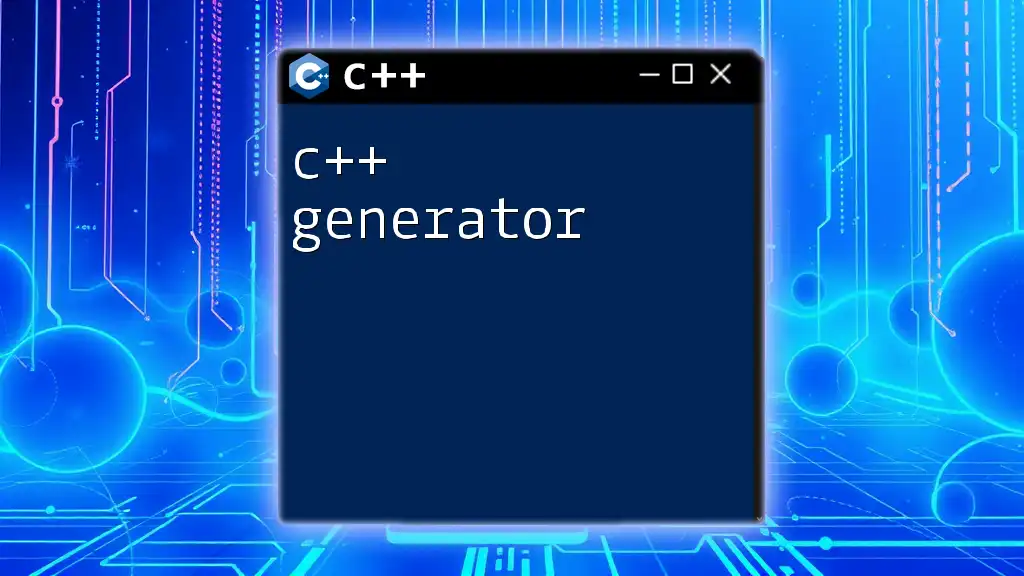
Validating and Storing UUIDs
Storing UUIDs in Databases
When integrating UUIDs into databases, it's crucial to choose the right data type. Most relational databases support UUID types, and you can store UUIDs as strings (VARCHAR) or as binary, depending on the requirements of your application.
For instance:
- In PostgreSQL, you can use the `UUID` type directly.
- In MySQL, you can store it as a `CHAR(36)` for the string representation, or `BINARY(16)` for a compact version.
Validating UUID Format in C++
To ensure proper UUID usage, you may want to validate the format of UUIDs in your applications. A good approach is utilizing regular expressions to verify that the string representation follows the UUID structure.
Here’s a sample code snippet for validating UUID format:
#include <regex>
#include <string>
bool is_valid_uuid(const std::string &uuid) {
std::regex uuid_regex("^[0-9a-f]{8}-[0-9a-f]{4}-[1-5][0-9a-f]{3}-[89ab][0-9a-f]{3}-[0-9a-f]{12}$");
return std::regex_match(uuid, uuid_regex);
}
This function uses a regular expression that checks if the string matches the standard format of a UUID, thus ensuring valid input.
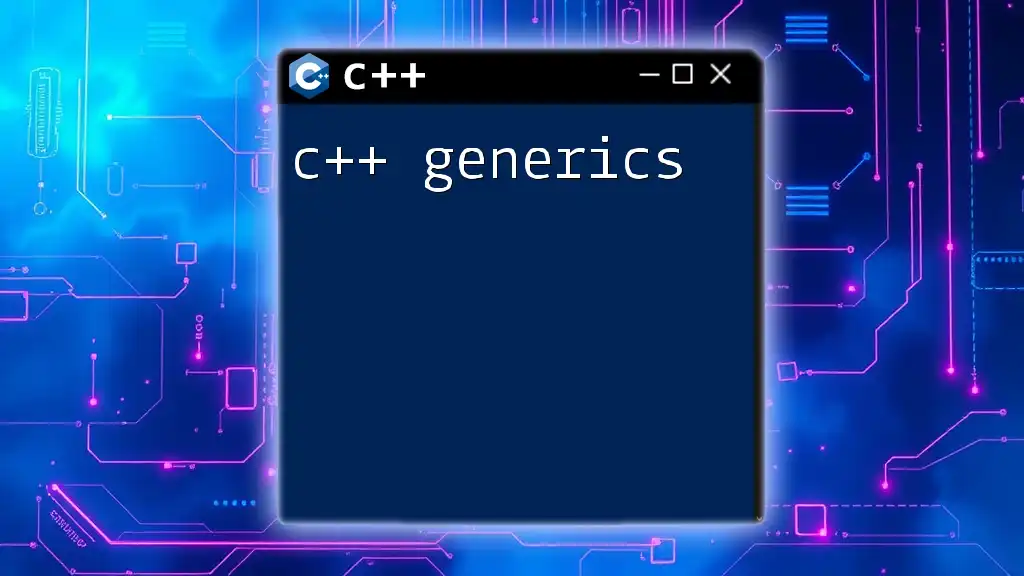
Best Practices When Using UUIDs
Efficiency Considerations
While UUIDs are immensely useful, they can introduce certain overheads in terms of efficiency. The 128-bit format is larger than standard integer types, which can affect storage and indexing, particularly in large datasets. It's advisable to weigh the use of UUIDs against the specific needs of your application and consider performance implications.
Application Scenarios
Consider the following real-world examples of UUID implementations:
- In a distributed database system, using UUIDs as primary keys ensures unique identifiers across multiple nodes.
- In a web application, session identifiers generated as UUIDs enhance security compared to sequential identifiers.
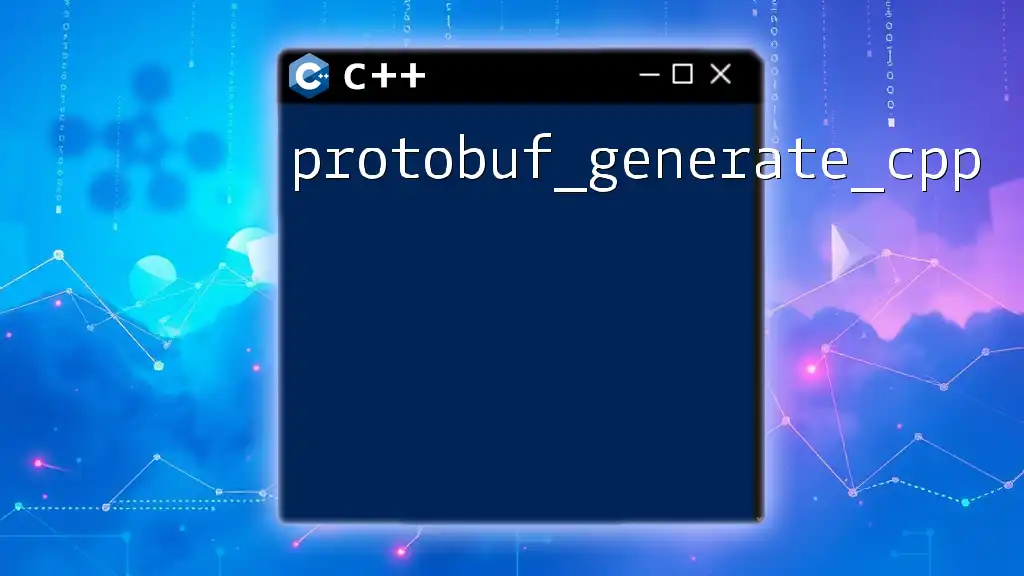
Troubleshooting Common Issues
Debugging UUID Generation Failures
When UUID generation fails, common issues usually stem from incorrect configurations or library incompatibilities. Ensure that libraries are correctly linked and verify that your development environment is polished. Utilize logging techniques to output error messages to assist in troubleshooting.
Compatibility Issues with Different Libraries
If utilizing multiple libraries in a project, compatibility issues may arise. Keep an eye on the documentation for any dependencies and ensure that UUIDs generated in one library can be interpreted by another, which may require conversions or specific formatting.
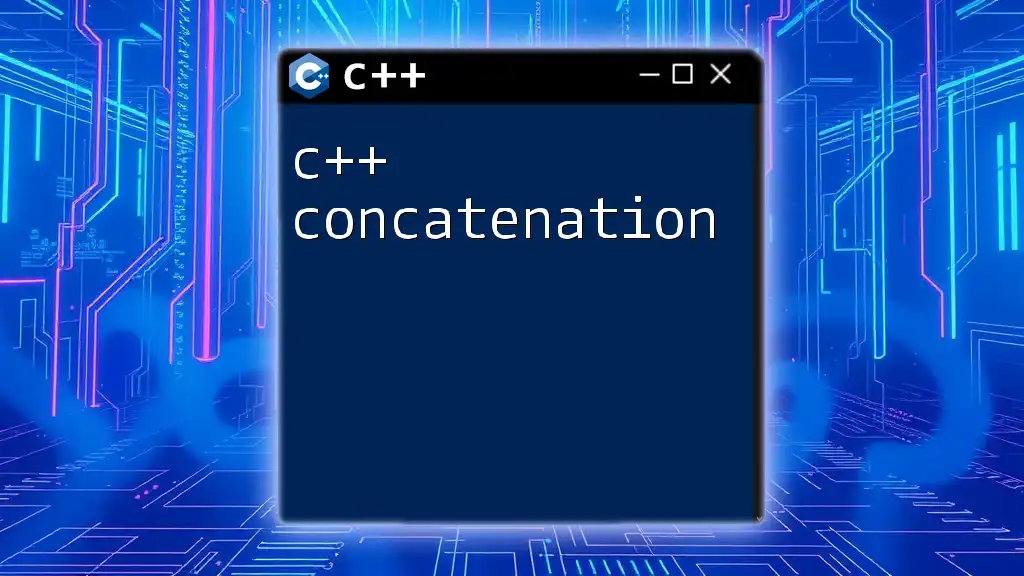
Conclusion
Generating UUIDs in C++ is a practical and efficient way to ensure global uniqueness within your applications. Whether using the Boost UUID library or the C++20 standard, developers can easily implement UUID generation and validation. By understanding both the structure of UUIDs and their practical applications, you can leverage their power in a variety of programming scenarios.
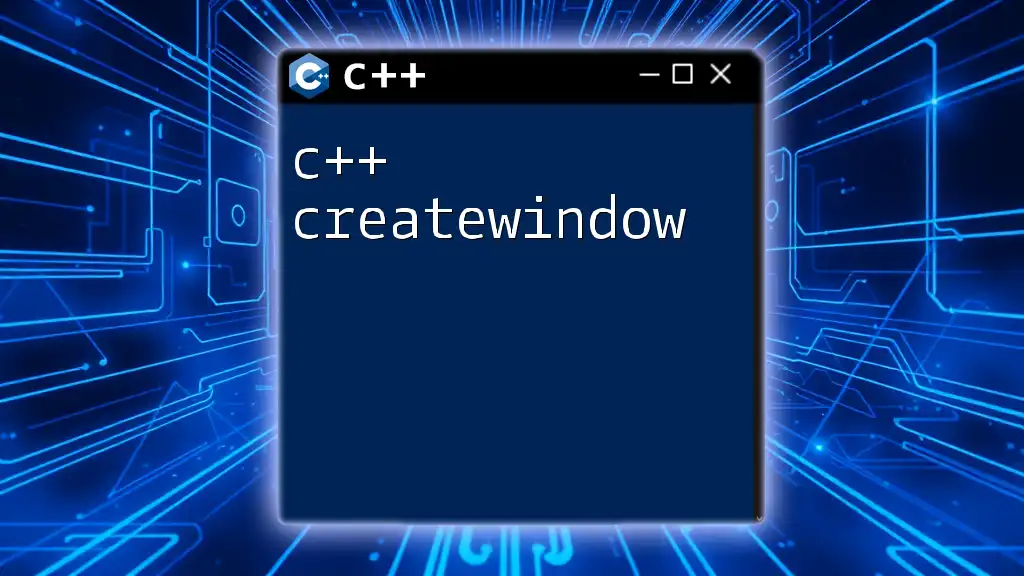
Additional Resources
For further information, consult the official documentation for your chosen libraries, such as [Boost UUID Documentation](https://www.boost.org/doc/libs/release/libs/uuid/) and the C++20 standards detailing `std::uuid`. Engaging with community forums and ongoing projects can enhance your understanding and application of UUIDs in C++.
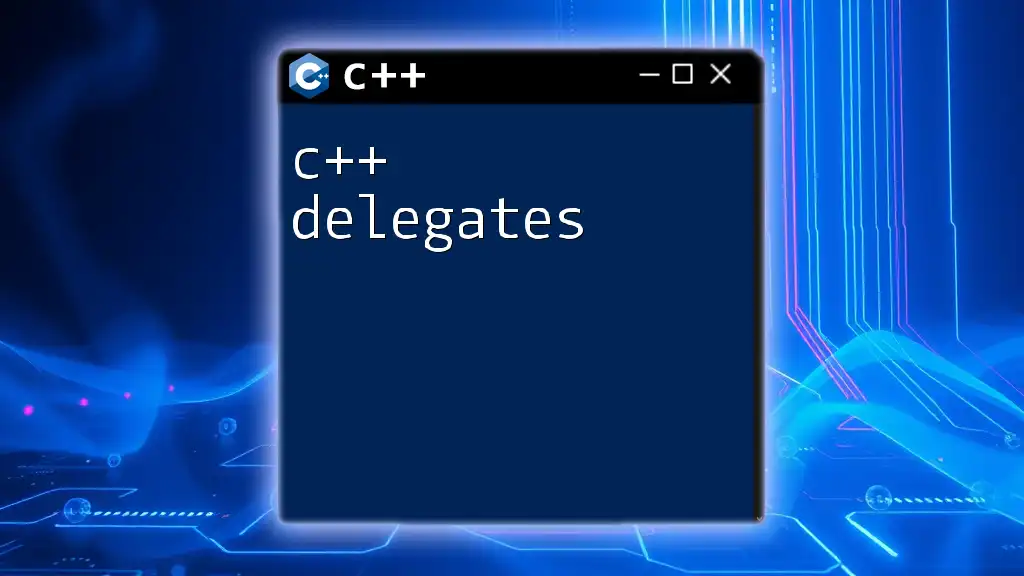
FAQs about UUID in C++
- What is the maximum length of a UUID? A UUID is always represented as a 36-character string (32 hexadecimal digits and 4 hyphens).
- Are UUIDs always truly unique? While the probability of duplication is extremely low, UUIDs are designed to be unique, but they are not infallible—handle them judiciously.
By incorporating UUIDs into your C++ projects, you will greatly enhance identifier management and maintain robustness across distributed systems and applications.