"C++ from C" refers to the transition from C, a procedural programming language, to C++, which introduces object-oriented programming features while maintaining the foundational syntax of C.
Here’s a simple code snippet demonstrating how you can create a class in C++ that resembles struct usage in C:
#include <iostream>
// C-style struct
struct Point {
int x;
int y;
};
// C++ class
class PointCPP {
public:
int x;
int y;
// Constructor
PointCPP(int x, int y) : x(x), y(y) {}
void display() {
std::cout << "Point(" << x << ", " << y << ")" << std::endl;
}
};
int main() {
Point pointC = {1, 2};
PointCPP pointCPP(3, 4);
std::cout << "C Point: (" << pointC.x << ", " << pointC.y << ")" << std::endl;
pointCPP.display();
return 0;
}
Understanding C and C++: Key Differences
Syntax and Structure
C and C++ share a similar syntax since C++ was developed as an extension of C. However, C++ introduces new features and constructs that enhance programming capabilities.
In C, a basic structure typically resembles the following:
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
In C++, while you can still use the C style, you often see object-oriented constructs added:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
While both examples print "Hello, World!", the second utilizes C++'s `iostream` library and its output stream `cout`, reflecting the emphasis on objects in C++.
Data Types and Variables
Both languages use similar primitive data types such as `int`, `float`, and `double`. However, C++ expands upon these with the introduction of classes and more sophisticated data types.
In C, strings are handled using character arrays, which can be cumbersome and error-prone:
char str[20] = "Hello";
C++, on the other hand, provides the `std::string` class, simplifying string manipulation:
#include <string>
std::string str = "Hello";
Using `std::string` allows for safer and more versatile operations compared to C-style strings.
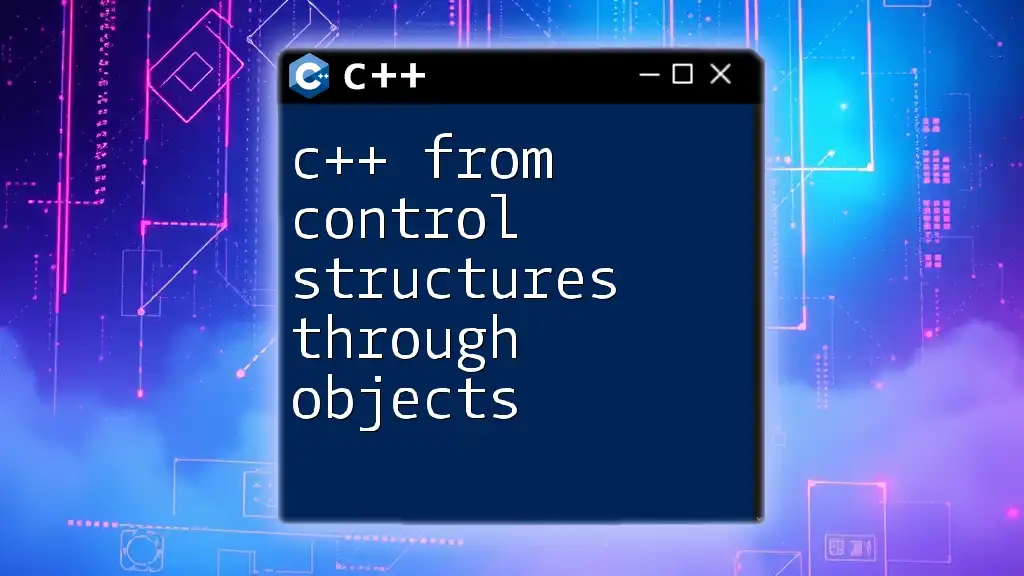
Transitioning Concepts: Functions and OOP
Functions in C vs. C++
A function in C is defined as follows:
int add(int a, int b) {
return a + b;
}
In C++, while function definitions remain quite similar, the introduction of function overloading allows defining multiple functions with the same name, differentiated by their parameter lists:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
This feature enhances code clarity and reduces the need for multiple function names that perform similar tasks.
Introduction to Object-Oriented Programming (OOP)
OOP is one of the most significant advancements when transitioning from C to C++. It offers several advantages such as encapsulation, inheritance, and polymorphism.
Classes and Objects
In C, data structures help group related data but have limitations in encapsulation:
struct Point {
int x;
int y;
};
In C++, you can define a class that encapsulates both data and functions:
class Point {
public:
int x, y;
void setCoordinates(int xVal, int yVal) {
x = xVal;
y = yVal;
}
};
This encapsulation allows for better data management and modular code.
Constructor and Destructor
C++ introduces the concepts of constructors and destructors for resource management:
class MyClass {
public:
MyClass() { // Constructor
std::cout << "Object created." << std::endl;
}
~MyClass() { // Destructor
std::cout << "Object destroyed." << std::endl;
}
};
In this example, constructors automatically initialize resources when an object is created, and destructors clean up when an object is no longer needed.
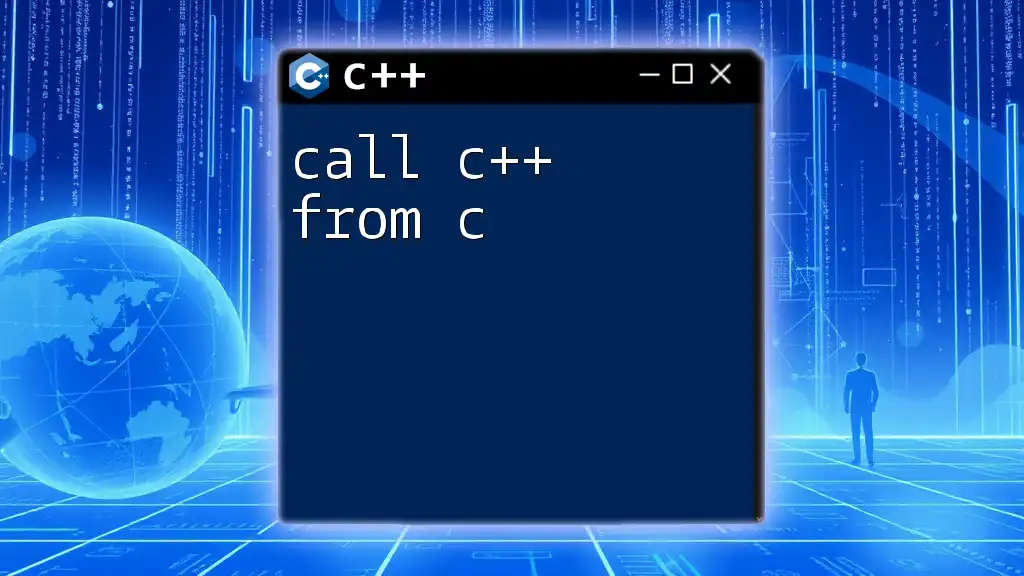
Essential C++ Concepts for C Programmers
Standard Template Library (STL)
The STL is a powerful feature of C++ that provides a collection of template classes and functions, enabling developers to use generic functionality without reinventing the wheel.
Using Vectors and Maps
The `std::vector` provides a dynamic array that can automatically resize:
#include <vector>
std::vector<int> numbers;
numbers.push_back(1);
numbers.push_back(2);
`std::map` is a key-value pair associative container:
#include <map>
std::map<std::string, int> age;
age["Alice"] = 30;
age["Bob"] = 25;
These structures save time and reduce complexity compared to manually managing arrays or linked lists.
Exception Handling
C traditionally handles errors using return codes and global variables, a method that can lead to confusing code. C++ introduces a clearer strategy through exception handling:
Try-Catch Block
In C++, you can manage errors using `try`, `catch`, and `throw`:
try {
throw std::runtime_error("An error occurred!");
} catch (const std::runtime_error& e) {
std::cout << e.what() << std::endl;
}
This mechanism allows you to separate error-handling logic from regular code flow, enhancing clarity and maintainability.
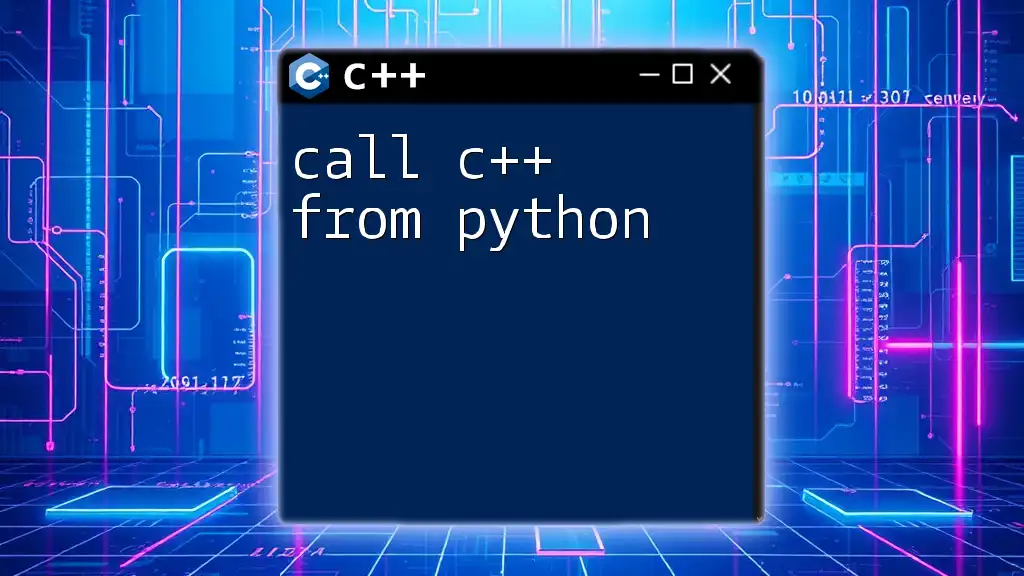
Advanced Topics: Templates and Lambda Functions
Introduction to Templates
Templates enable defining functions and classes with generic types, making code more reusable.
Function Templates and Class Templates
Here's a simple function template for swapping two values:
template<typename T>
void swap(T& a, T& b) {
T temp = a;
a = b;
b = temp;
}
For a class template example, consider:
template<typename T>
class Box {
private:
T data;
public:
Box(T data) : data(data) {}
T getData() { return data; }
};
Templates allow you to write code that works with any data type, promoting code reusability and flexibility.
Lambda Functions
Lambda functions are a feature introduced in C++11, allowing you to write anonymous functions directly in the code where they are needed.
Lambda Basics
A simple lambda function to add two numbers is as follows:
auto add = [](int a, int b) { return a + b; };
std::cout << add(3, 5) << std::endl; // Outputs 8
Uses of Lambda Functions
These functions can simplify code by reducing the need for separate named functions, especially for straightforward operations such as sorting:
std::vector<int> vec = {3, 1, 4, 1, 5};
std::sort(vec.begin(), vec.end(), [](int a, int b) {
return a < b;
});
In this example, the lambda function is passed directly to `std::sort`, improving code readability.
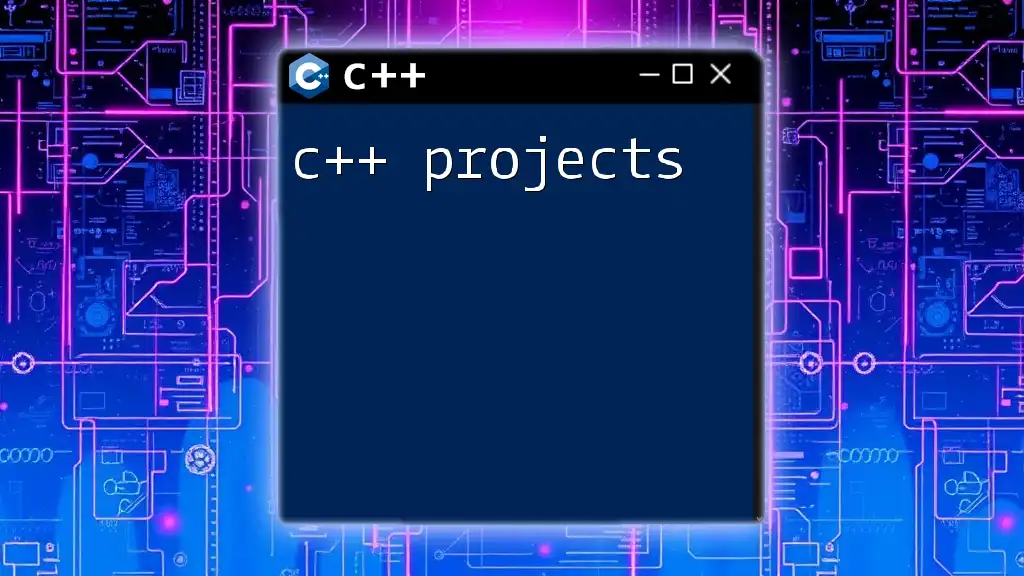
Practical Application: Converting C Code to C++
Example 1: Simple C Program
Here is a simple C program that calculates the factorial of a number using recursion:
#include <stdio.h>
int factorial(int n) {
if (n == 0) return 1;
return n * factorial(n - 1);
}
int main() {
int num = 5;
printf("Factorial: %d\n", factorial(num));
return 0;
}
Converted C++ Code
This can be enhanced in C++ as follows:
#include <iostream>
class Factorial {
public:
static int calculate(int n) {
if (n == 0) return 1;
return n * calculate(n - 1);
}
};
int main() {
int num = 5;
std::cout << "Factorial: " << Factorial::calculate(num) << std::endl;
return 0;
}
By wrapping the function in a class and promoting it to `static`, we allow for logical grouping and potential extension in the future.
Example 2: Struct to Class
A simple struct in C might look like this:
struct Rectangle {
int width;
int height;
};
While functional, this does not encapsulate behavior. In C++, this can be converted to:
class Rectangle {
private:
int width, height;
public:
Rectangle(int w, int h) : width(w), height(h) {}
int area() {
return width * height;
}
};
Using a class allows for better organization of related attributes and methods while protecting the integrity of the data.
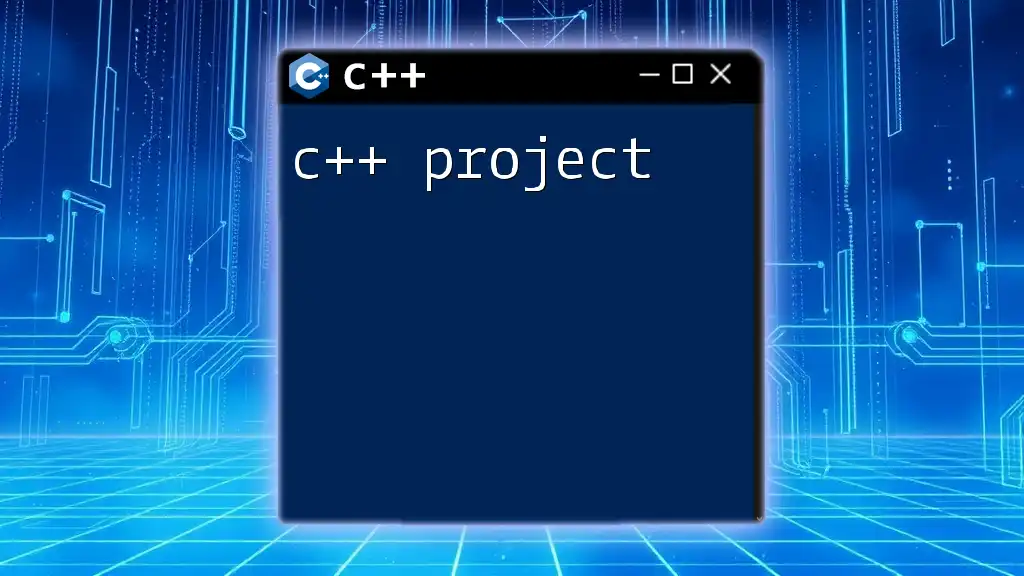
Best Practices for C to C++ Transition
Coding Style
Maintaining a consistent and clear coding style is vital during the transition from C to C++. Utilize meaningful naming conventions, consistent indentation, and comments to improve code readability. Adopting older C-style practices can create confusion in a C++ context, so it’s beneficial to adapt to C++ conventions.
Using Standard Libraries
One of the best practices is to leverage C++'s powerful standard libraries when possible. Instead of reinventing data structures and algorithms, utilize the STL, ensuring your code is both efficient and concise.
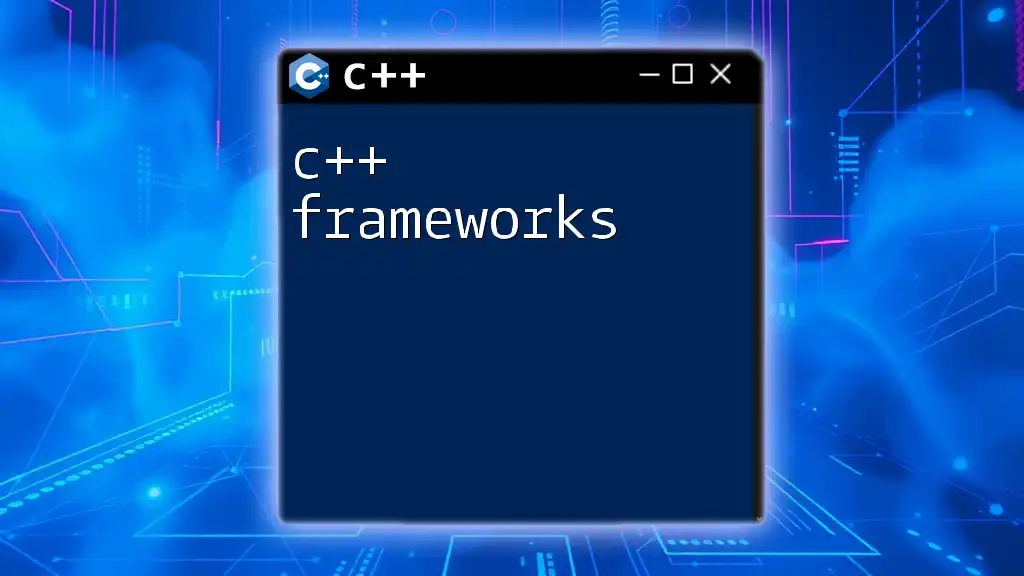
Performance Considerations
Memory Management
C++ provides sophisticated memory management options with `new` and `delete`, as well as smart pointers such as `std::unique_ptr` and `std::shared_ptr`. These prevent memory leaks and enhance safety when handling dynamic memory:
#include <memory>
std::unique_ptr<int> ptr = std::make_unique<int>(5);
Compiler Differences
When transitioning from C to C++, recognizing the differences in compilers and their optimizations is important. C++ compilers often have more advanced capabilities for optimizations, impacting execution speed and memory utilization positively.
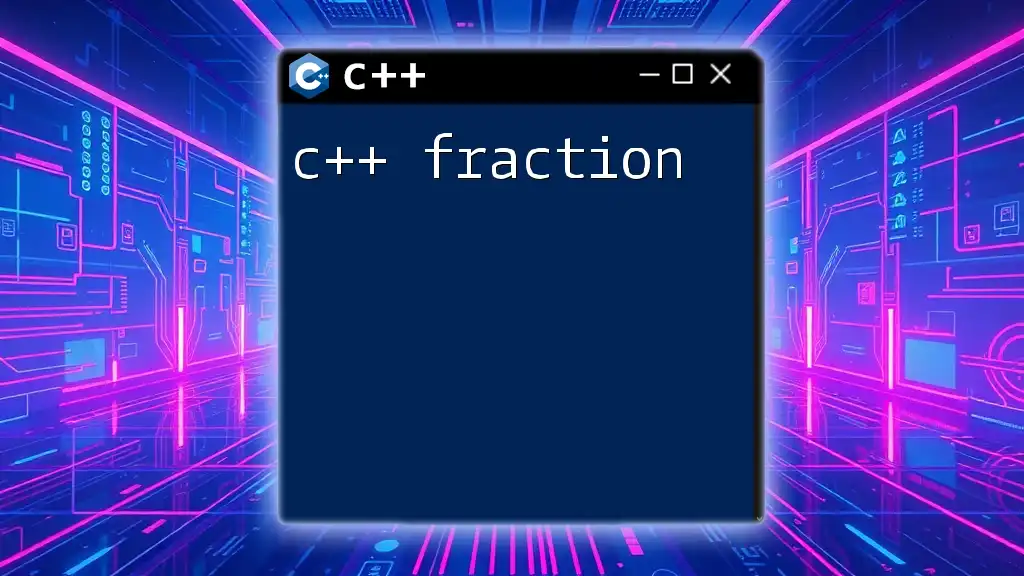
Conclusion
Transitioning from C to C++ opens up a wealth of new programming constructs and enhanced capabilities that improve both productivity and code quality. This guide has paved the way for understanding the essential differences and similarities, along with practical applications to ease the shift.
Encouraging programmers to explore the extensive features of C++ will foster better coding practices and lead to modern, efficient software development. As each individual continues their learning journey, the exploration of resources and community support will be invaluable.