In C++, `endl` and `\n` are both used to create a new line, but `endl` also flushes the output buffer, while `\n` does not.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Using endl to create a new line and flush the buffer
std::cout << "Hello, World!\n"; // Using \n to create a new line without flushing the buffer
return 0;
}
Understanding Output in C++
What is Output Stream?
In C++, output streams are the means through which data is presented to the user, typically via the console. The most commonly used output stream is `std::cout`, which allows developers to display messages to the console.
The Need for Line Breaks
Line breaks are crucial for enhancing the readability of console output. Without them, output data can appear jumbled, making it hard for users to decipher information. Therefore, inserting appropriate line breaks is essential for a pleasant user experience, especially during debugging or when providing critical information.
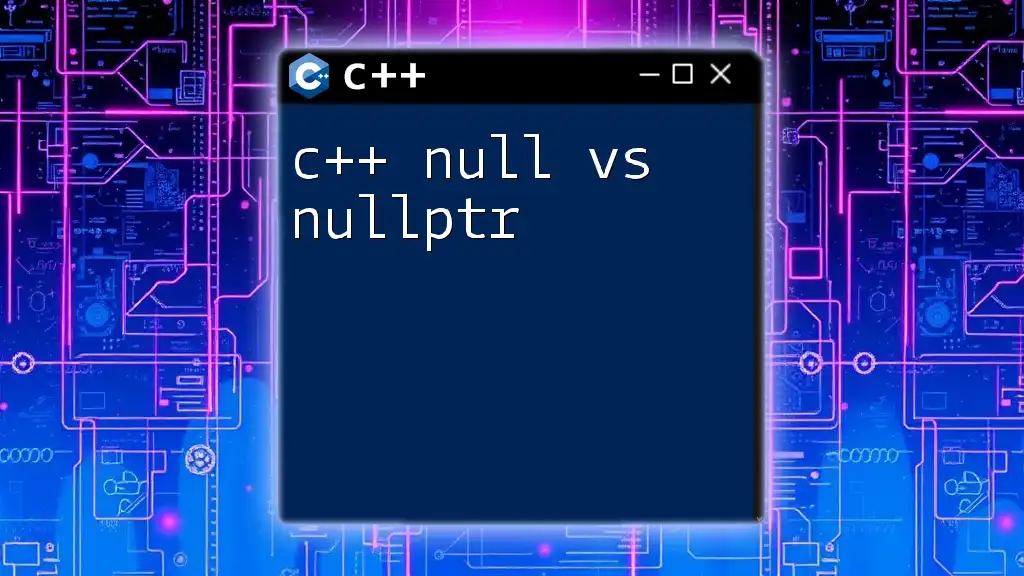
C++ endl
What is endl?
`endl` is a manipulator in C++ that inserts a new line into the output stream. When used with `std::cout`, it signals the end of a line. The purpose of `endl` goes beyond merely creating a new line; it also performs an additional function: flushing the output buffer.
Syntax of endl
Using `endl` is straightforward. Here’s how you would write it in a typical output situation:
std::cout << "Hello World!" << std::endl;
In this example, the string "Hello World!" is printed, followed by a new line and the immediate flushing of the output buffer.
Behavior of endl
Flushes the Output Buffer
One significant characteristic of `endl` is its ability to flush the output buffer. Flushing the buffer means that the output is sent to the console immediately instead of waiting for the buffer to fill up. This feature can be useful during debugging when you want to ensure that all output is visible at a given point in time. However, frequent flushing can lead to performance degradation, especially in loops or extensive output situations.
When to Use endl
Use `endl` when:
- You want to ensure that the user sees immediate output, particularly during debugging.
- The performance impact is negligible, such as in systems with low-frequency output.
Pros:
- Guarantees that messages are promptly displayed.
Cons:
- Can make programs significantly slower due to flushing.
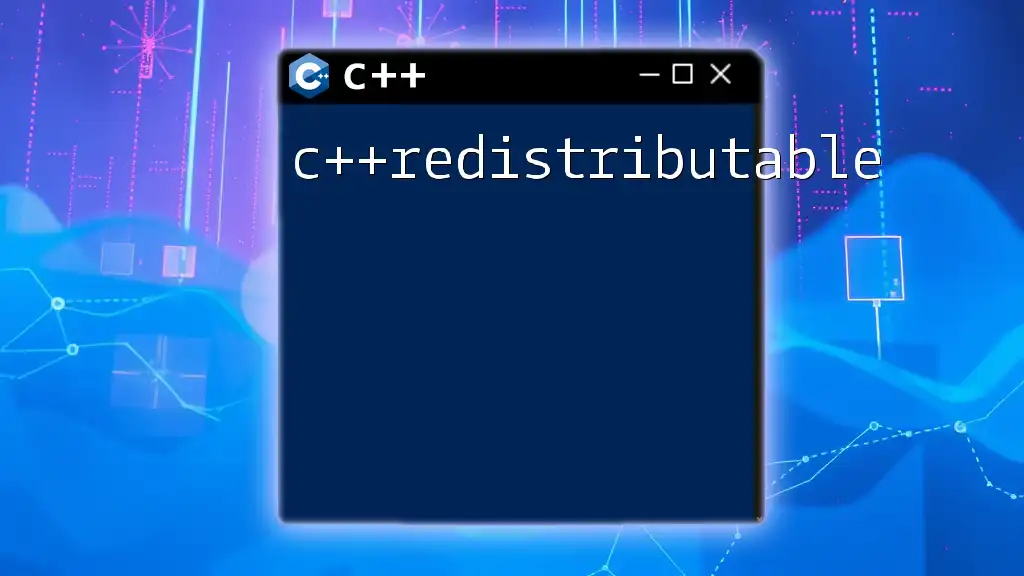
The n Character
What is \n?
The `\n` character, known as the newline character, represents a line break in C++. Unlike `endl`, it does not flush the output buffer. This characteristic can lead to better performance in applications that involve extensive output.
Syntax of \n
The syntax for using `\n` is also straightforward. Here’s a simple example:
std::cout << "Hello World!\n";
In this case, "Hello World!" is printed, followed by a new line, but without the flushing effect.
Behavior of \n
Does Not Flush the Output Buffer
Unlike `endl`, the `\n` character does not flush the output buffer. This means it adds a new line without hindering performance. In circumstances where a large amount of output is generated, using `\n` can significantly speed up execution time.
When to Use \n
Use `\n` when:
- You are working with large volumes of data where performance is critical.
- The output does not require immediate visibility, such as in batch processing.
Considerations:
- While it may seem less readable initially, maintaining quick output is often more important in extensive applications.
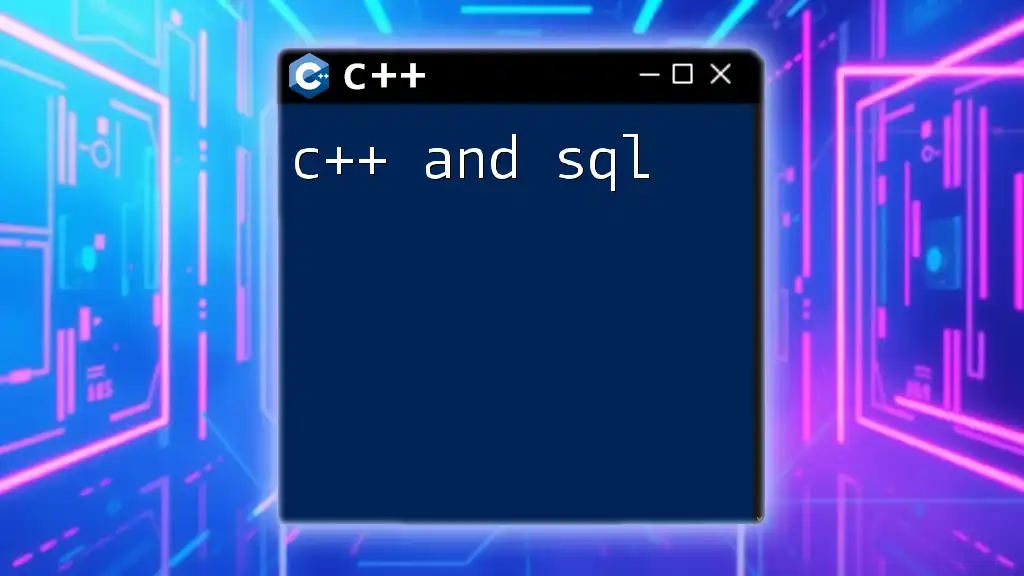
Comparing C++ endl vs n
Similarities
Both `endl` and `\n` serve the primary function of creating a new line in output. Their usage is common across various programming tasks where line breaks enhance readability.
Key Differences
Performance Considerations
The most crucial distinction lies in performance:
- `endl`: Flushes the output buffer, which can slow down the execution in cases involving multiple outputs.
- `\n`: Does not flush the buffer and, as a result, is typically more efficient for frequent outputs.
Readability and Code Clarity
Using `endl` can occasionally enhance readability, especially in smaller, isolated output tasks. In contrast, using `\n` may make the code look cluttered with escape sequences.
Example Comparison
Consider the following code snippets:
std::cout << "Using endl:" << std::endl;
std::cout << "Using n:\n";
While both snippets produce a new line in output, the performance implications of `\n` are advantageous in scenarios where speed is essential.
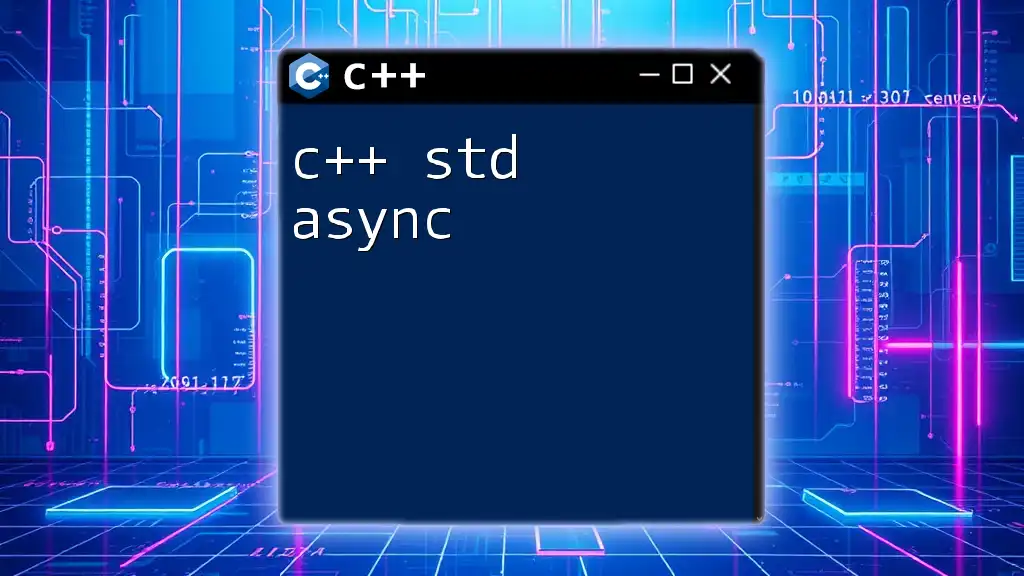
Best Practices
When working with C++, choose between `endl` and `\n` based on the context:
- For immediate output visibility (like debugging), lean towards `endl`.
- For performance-sensitive code, especially loops or frequent prints, use `\n`.
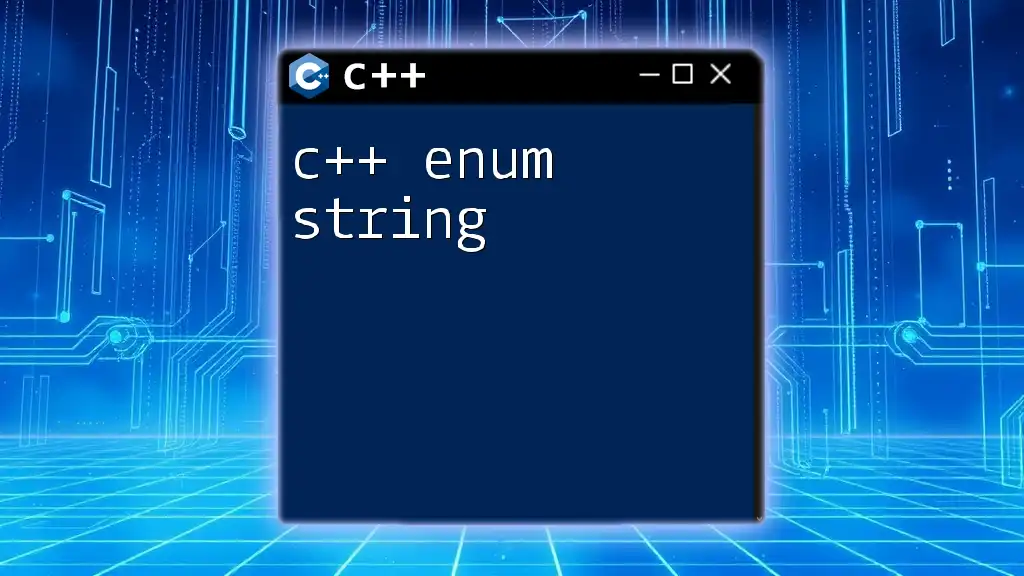
Conclusion
When navigating the c++ endl vs n debate, it is essential to consider the goals of your program. Choose `endl` for clarity and immediate output, while `\n` offers efficiency for performance-critical applications. Understanding when to employ each can significantly enhance your coding efficiency and program performance.
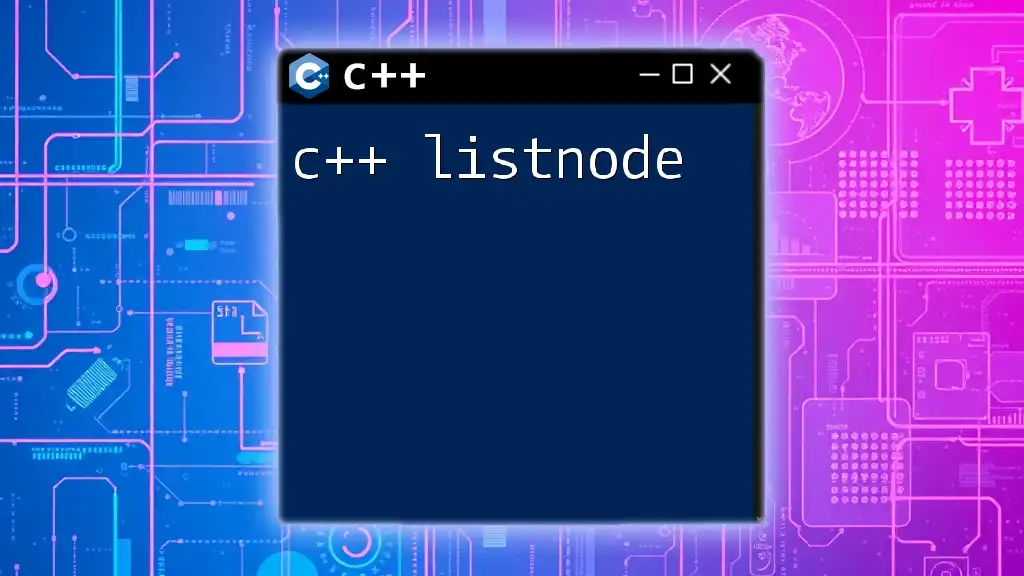
FAQs
Is it better to use endl over \n?
Choosing between `endl` and `\n` largely depends on your specific use case. If you require immediate output visibility, then `endl` is preferable. In contrast, if your program emphasizes performance, especially with frequent outputs, opt for `\n`.
Can I use both in my code?
Yes, you can use both `endl` and `\n` interchangeably in your program. However, it is crucial to apply them wisely based on the context to avoid performance hits or loss of clarity.
How do these choices affect larger applications?
In larger applications, especially those that yield substantial output, the choice between `endl` and `\n` can significantly affect performance and user experience. Using `\n` in such cases allows the application to run more efficiently while still maintaining adequate formatting for output.