In C++, you can convert an enum to an integer by simply assigning the enum value to an integer variable, as shown in the following example:
enum Color { Red, Green, Blue };
int main() {
Color myColor = Green;
int colorValue = myColor; // colorValue will be 1
return 0;
}
Understanding C++ Enums
What is an Enum?
An enum, short for enumeration, is a user-defined type in C++ that consists of a set of named integral constants. Enums provide a way to define and group related values, making your code more readable and maintainable.
Here’s the basic syntax for declaring an enum:
enum Color { RED, GREEN, BLUE };
With the above declaration, the constants `RED`, `GREEN`, and `BLUE` are assigned integer values starting from `0` by default. Therefore, `RED` is `0`, `GREEN` is `1`, and `BLUE` is `2`.
Types of Enums in C++
Unscoped Enums
Unscoped enums are the traditional form of enums in C++. They can be used without any qualification but can lead to naming conflicts. For instance:
enum Direction { NORTH, SOUTH, EAST, WEST };
In the example above, `NORTH`, `SOUTH`, `EAST`, and `WEST` will be accessible directly in the scope where the enum is defined, potentially resulting in name collisions.
Scoped Enums (enum class)
C++11 introduced scoped enums, or `enum class`, to address some of the limitations of unscoped enums. Scoped enums do not implicitly convert to integers and require qualified names, providing better type safety and reducing the risk of name conflicts.
Here is an example of a scoped enum:
enum class Status { SUCCESS, FAILURE };
In this case, to access the constants, you must use the enum name, such as `Status::SUCCESS`.
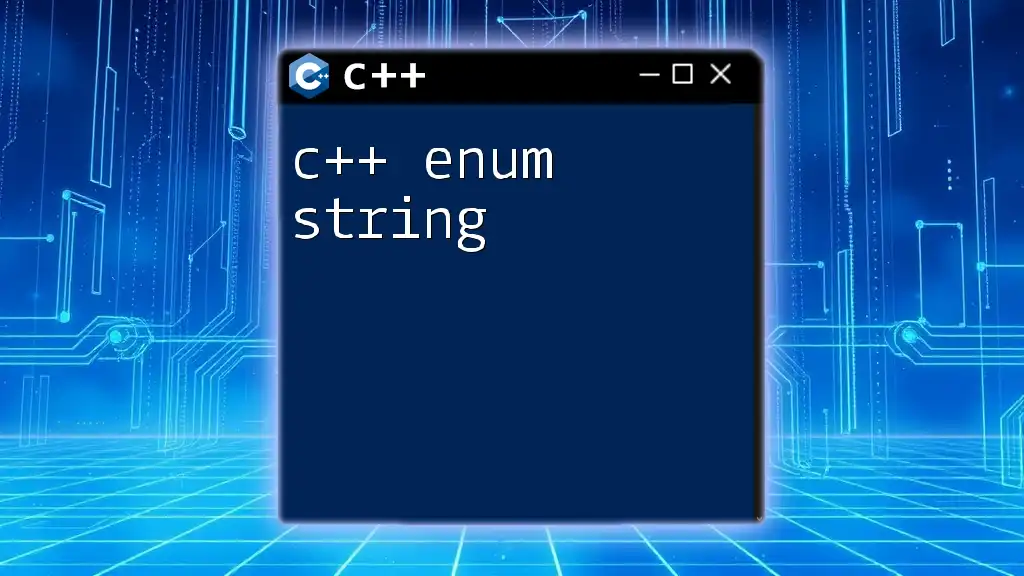
Converting Enum to Int in C++
Implicit Conversion
C++ allows for implicit conversion of unscoped enums to integers. This means you can directly assign an enum member to an integer variable without any conversion syntax. This feature makes unscoped enums convenient but can sometimes lead to unclear code.
For example:
enum Fruit { APPLE, BANANA, CHERRY };
int appleValue = APPLE; // Implicit conversion to int
In this case, `APPLE` is assigned the integer value of `0`, and `appleValue` will hold that integer implicitly.
Explicit Conversion
Scoped enums do not allow implicit conversion. You must use explicit conversion when working with scoped enums. This conversion enhances type safety by preventing unintended conversions and promoting clearer code practices.
To convert a scoped enum to an integer, you use `static_cast`. Here’s how it looks:
enum class TrafficLight { RED, YELLOW, GREEN };
int lightValue = static_cast<int>(TrafficLight::RED); // Explicit conversion
In this example, `TrafficLight::RED` is converted to its underlying integer representation, which is `0`.
Risks of Implicit Conversion
While implicit conversion of unscoped enums can be convenient, it has inherent risks. The most significant concern is the potential for unclear code interpretation. It can become difficult to discern what values represent merely by looking at the code. This ambiguity can lead to bugs that are hard to trace, especially in larger codebases. Furthermore, this conversion compromises type safety; developers can accidentally pass enum values inappropriately, leading to runtime errors.
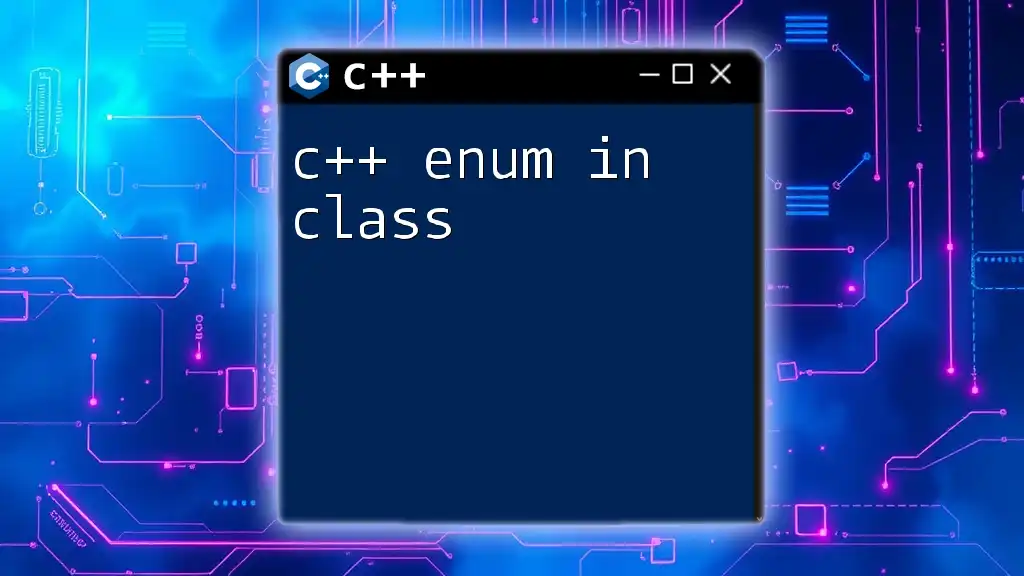
Practical Examples
Using Enums in Switch Cases
Enums can be especially useful in switch statements, enhancing code clarity and providing a straightforward way to handle different cases. Using enums in this context avoids the use of magic numbers and helps developers understand the intent behind each condition.
Consider the following example:
enum class Operation { ADD, SUBTRACT, MULTIPLY };
Operation op = Operation::ADD;
switch (op) {
case Operation::ADD:
// Add code block
break;
case Operation::SUBTRACT:
// Subtract code block
break;
}
By doing this, each case clearly corresponds to a meaningful name rather than an arbitrary number, making the code easier to read and maintain.
Enum to Int for Array Indexing
Enums can also be effectively used for indexing arrays, leading to cleaner and more understandable code. By allowing enum values to serve as indices, you can directly relate the indexing logic to meaningful names, avoiding magic numbers in array accesses.
Here’s an illustrative code snippet:
enum ColorIndex { RED, GREEN, BLUE };
int colors[3] = { 255, 0, 0 };
int selectedColor = colors[ColorIndex::GREEN]; // Using enum as index
In the snippet above, `ColorIndex::GREEN` clearly indicates the intended index, making your code more intuitive and less error-prone.
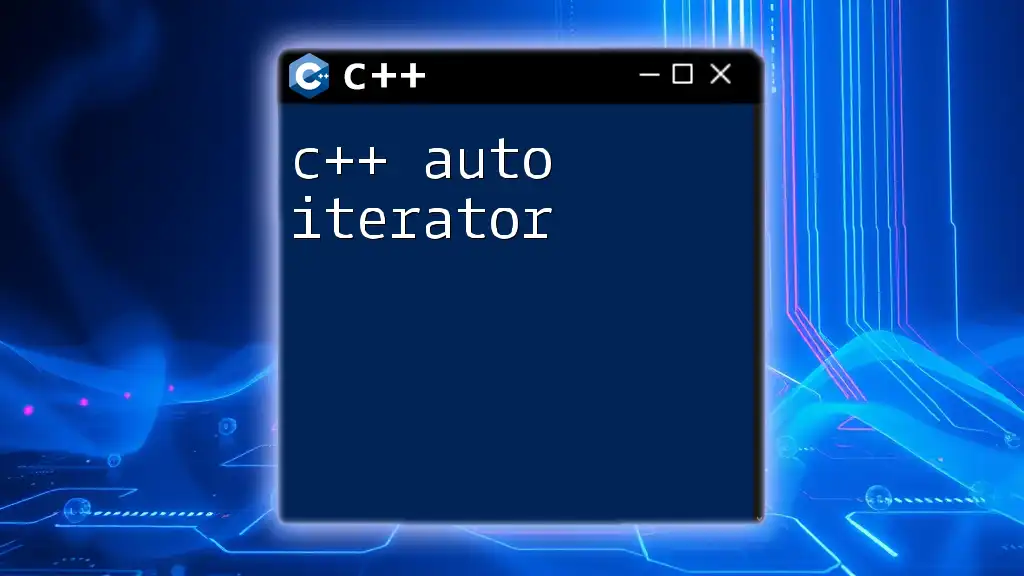
Best Practices
When to Use Enums
Using enums can significantly improve code readability and maintainability. They are ideal for representing a fixed set of related constants. Guideline includes:
- Group related options: Use enums instead of preprocessor directives to group related string or integer constants.
- Clarify choices: Use enums in scenarios involving conditions or states that represent a limited number of possibilities.
Avoiding Magic Numbers
Using enums helps avoid magic numbers, which are hardcoded values that appear in your code without any explanation of their significance. By defining these numbers as enums, you provide context and make the code easier to understand. This quality leads to fewer errors and greater maintainability.
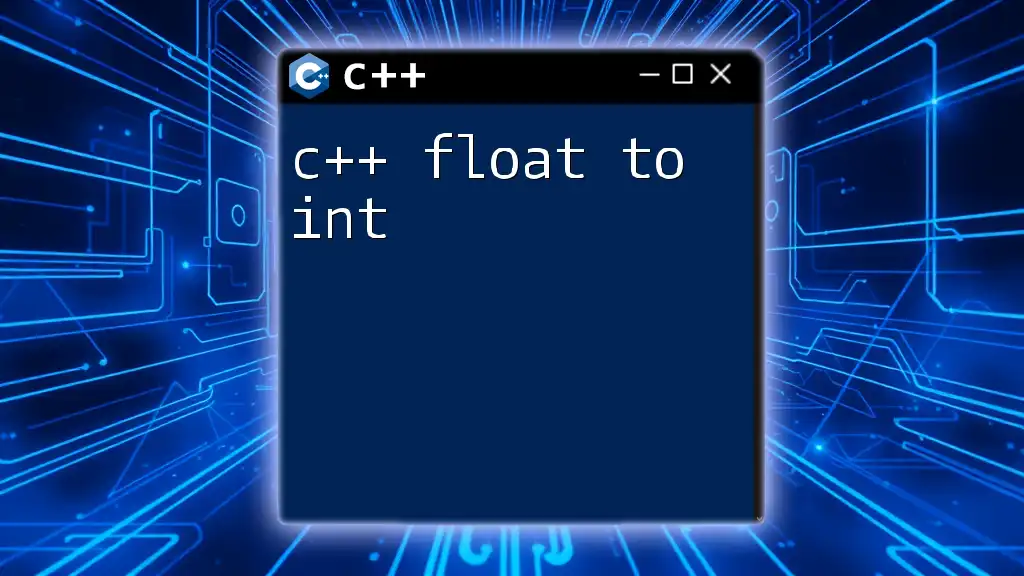
Conclusion
In summary, understanding how to convert C++ enums to int is crucial for effective programming. Both unscoped and scoped enums have their appropriate use cases, and each comes with its advantages and restrictions regarding conversion. By utilizing enums judiciously, you can write clearer, more reliable, and safer C++ code. Practice implementing these techniques in your projects, and explore more advanced topics such as enforcing better type safety with templates.
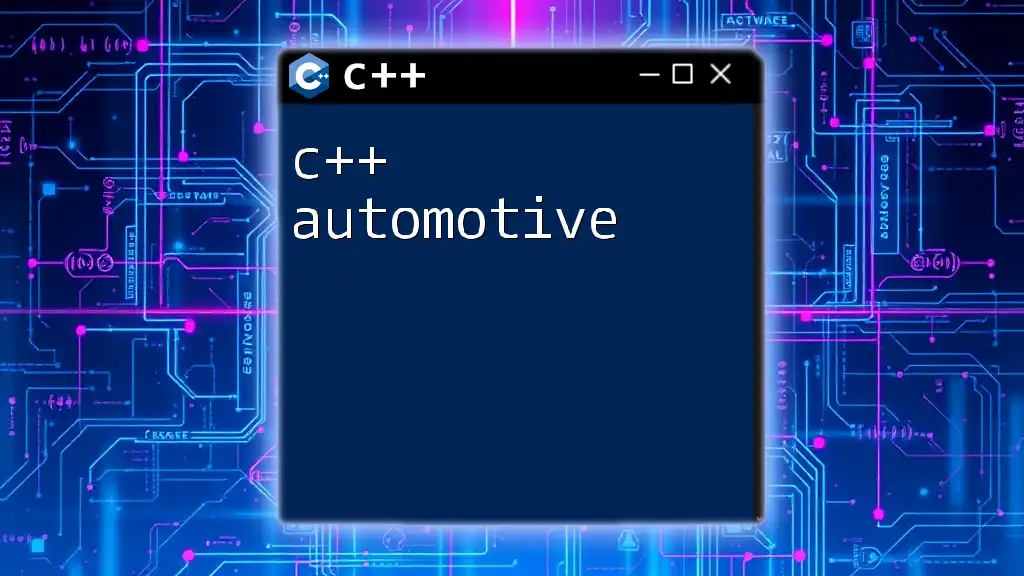
Additional Resources
For further reading, consult the official C++ documentation on enums and consider analytics or resources that dive deeper into type safety and advanced language features. Educational books and tutorials on modern C++ development can also provide valuable insights into using enums effectively in real-world applications.