The "Advent of Code" is an annual event where participants solve coding challenges using various programming languages, including C++, which helps to sharpen their coding skills through fun and engaging tasks.
Here's a simple C++ code snippet that demonstrates reading input and printing output, a common task in these challenges:
#include <iostream>
int main() {
std::string input;
std::cout << "Enter something: ";
std::getline(std::cin, input);
std::cout << "You entered: " << input << std::endl;
return 0;
}
What is Advent of Code?
Advent of Code is an annual programming event that consists of a series of daily coding challenges during December. Each challenge is released at midnight EST and is designed to engage developers in problem-solving and coding in a fun and festive context. The significance of this event lies not only in enhancing coding skills but also in fostering a sense of community among developers around the world. Participants from various backgrounds and skill levels come together to tackle problems that often span a wide range of topics, including algorithmic thinking, data structures, and system design.
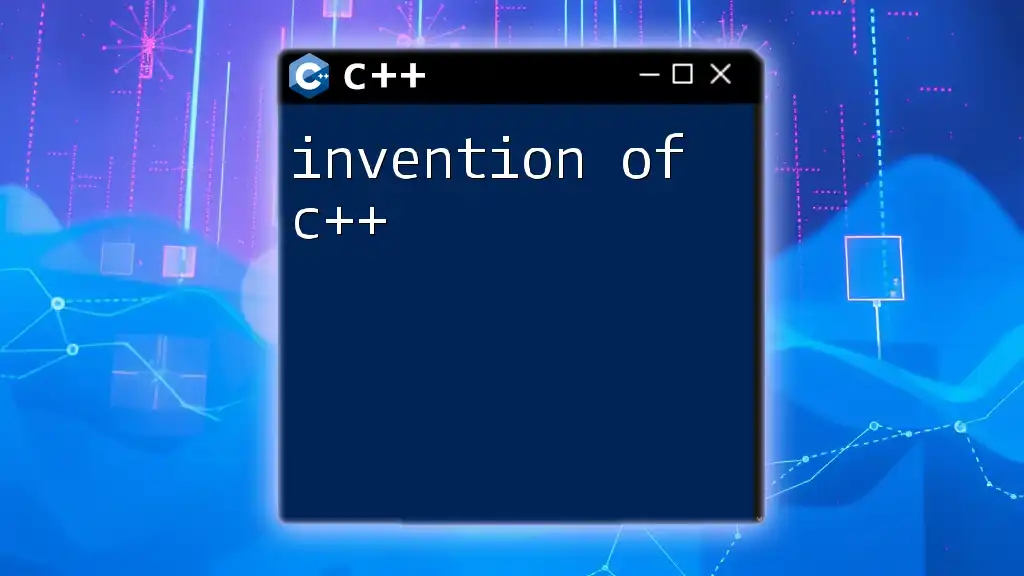
Why C++?
C++ is an excellent choice for tackling the challenges presented in Advent of Code. Its features include:
- Performance: As a compiled language, C++ offers significant speed advantages, making it suitable for time-sensitive problems.
- Control: C++ gives developers direct control over system resources, enabling optimization of memory usage and execution speed.
- Versatility: With its rich standard library, C++ provides various tools and utilities that can simplify problem-solving.
By using C++, participants can leverage these features to create efficient solutions to the puzzles presented during Advent of Code.
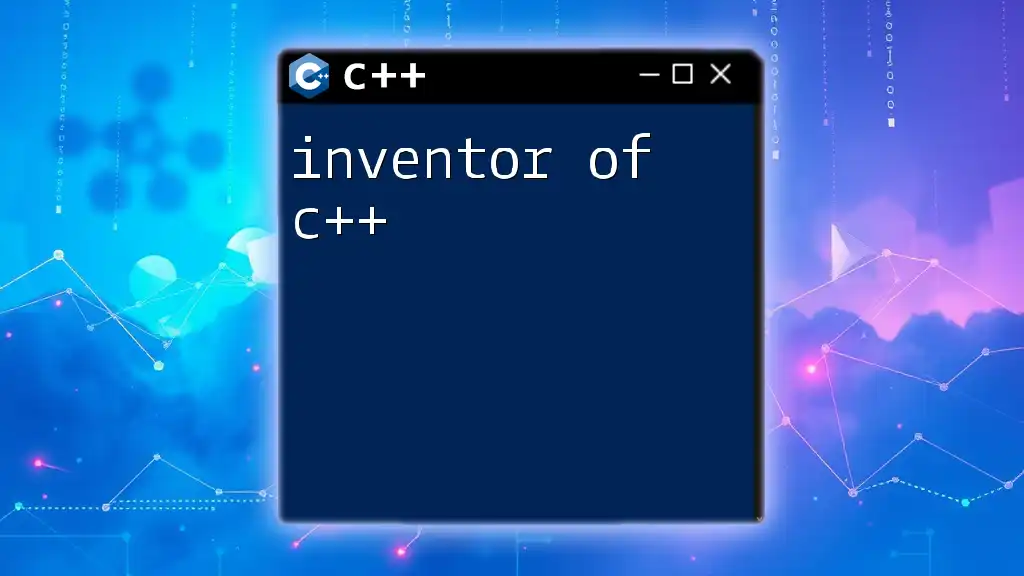
Getting Started with Advent of Code
Setting Up Your C++ Environment
To embark on your Advent of Code journey, you need to set up your C++ development environment.
Tools Required:
- Compiler Options: You can choose from compilers like GCC, Clang, or MSVC. Each has its own installation steps.
- IDEs: Integrated Development Environments like Visual Studio, CLion, or Code::Blocks enhance your coding experience with features like debugging, code suggestions, and integrated builds.
Sample Code Setup: Here's a simple program to test your setup:
#include <iostream>
using namespace std;
int main() {
cout << "Welcome to Advent of Code!" << endl;
return 0;
}
If you run this, it should print a friendly welcome message to the console.
Troubleshooting Environment Issues: When dealing with setup problems, consider checking your compiler paths, ensuring that your IDE is correctly configured, and looking out for syntax errors in your code.
Understanding Problem Statements
To solve challenges effectively, first, break down the problem statement. Focus on key elements, such as:
- Inputs: What data you need to process.
- Constraints: Any limitations on time or memory usage.
- Outputs: The required results that need to be returned.
Understanding these components will help you devise a robust solution.
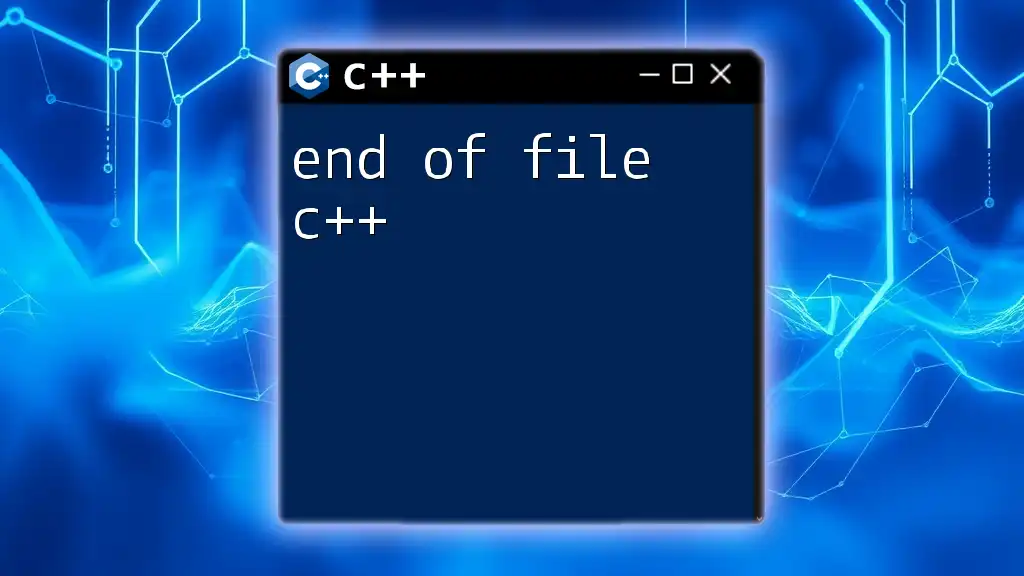
Solving Advent of Code Challenges with C++
Example Challenge Breakdown
Let’s consider a simple challenge to illustrate the problem-solving process effectively. Assume the task is to find the sum of an array of integers.
Code Walkthrough: Here’s how you could implement this in C++:
#include <iostream>
#include <vector>
using namespace std;
// Function to solve example problem
int sumOfVector(const vector<int>& numbers) {
int sum = 0;
for (int num : numbers) {
sum += num;
}
return sum;
}
int main() {
vector<int> exampleVector = {1, 2, 3, 4, 5};
cout << "The sum is: " << sumOfVector(exampleVector) << endl;
return 0;
}
Explanation of Code Logic: In the code above, we define a function that takes a vector of integers and returns their total sum. The `for` loop iterates through each element, accumulating their values into the `sum` variable. Finally, the program outputs the result with a message.
Common C++ Techniques for Advent of Code
Using STL (Standard Template Library): The STL provides a variety of data structures that can simplify coding tasks. For instance, vectors are dynamic arrays that can grow in size, making them ideal for unknown quantities of input data.
Input/Output Handling: Efficient handling of input and output can significantly affect performance. A simple example of reading an integer input:
int n;
cin >> n; // Reading a single integer input
This can be enhanced by reading multiple inputs in a single line, which is common in the Advent of Code problems.
Recursion and Iteration: Understanding when to use recursion versus iteration is vital. Recursion can simplify code for problems involving tree structures or combinations, while iteration usually performs better on loops that do not have a natural recursive structure.
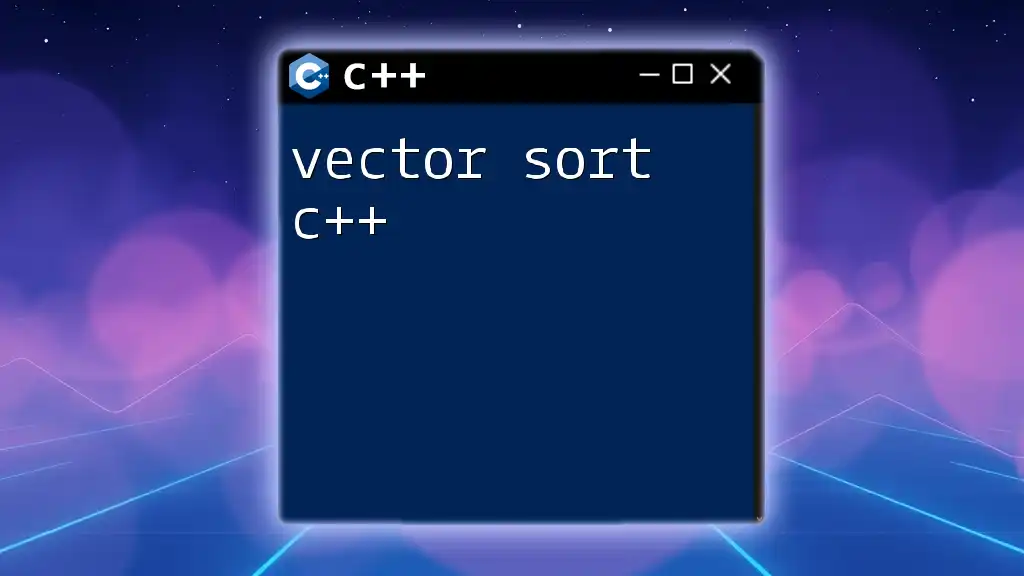
Advanced C++ Techniques for Complex Challenges
Optimization Strategies
Algorithm Complexity: Always assess the time and space complexity of your solutions. A fast solution may need efficient algorithms (like quicksort over bubble sort) to handle larger datasets effectively. For instance, if your algorithm has a time complexity of O(n^2), it may not be suitable for large inputs.
Data Structures Implementation: Sometimes, built-in data structures may not fit your needs perfectly. In such cases, it can be advantageous to implement custom data structures like trees or linked lists.
struct Node {
int data;
Node* next;
};
This struct can be the basis for your own linked list implementation, allowing for more tailored memory management and performance.
Debugging and Testing
Best Practices for Debugging C++ Code: Utilizing debugging tools like GDB or using integrated debugging features in your IDE can save time and help identify logical errors in your code.
Unit Testing in C++: Ensure your functions work as expected by implementing unit tests. A popular framework for C++ testing is Google Test, which allows you to write intuitive test cases.
#include <gtest/gtest.h>
TEST(SumTest, HandlesPositiveInput) {
EXPECT_EQ(sumOfVector({1, 2, 3}), 6);
}
By using unit tests, you can verify that your functions return expected results, ensuring reliability as you develop your solutions.
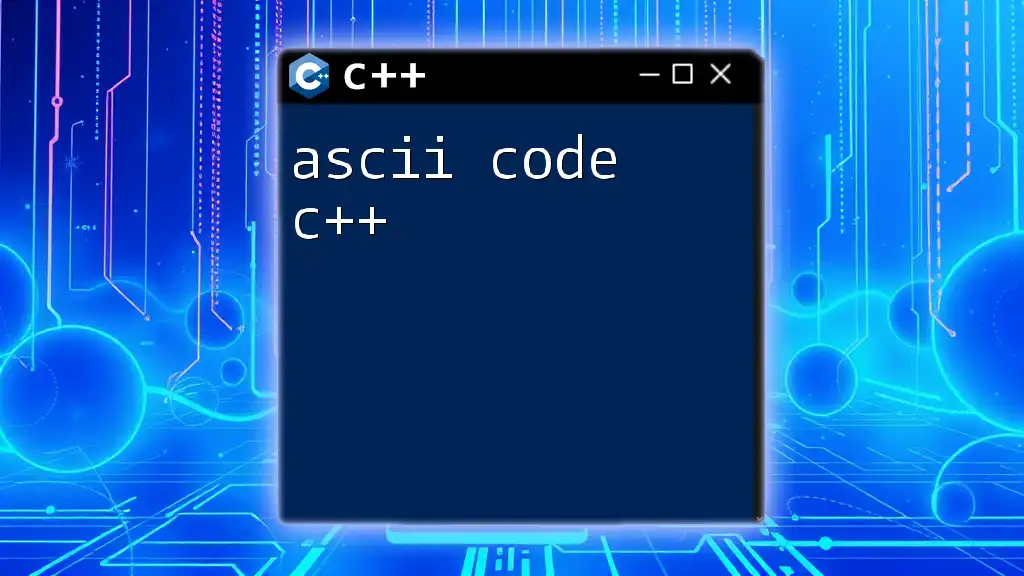
Collaborating with the Community
Sharing Solutions on GitHub
Once you've developed solutions, consider setting up a GitHub repository to share your work. This not only showcases your achievements but also allows others to learn from your code. Remember to include clear documentation and comments to explain your thought process.
Engaging with the Advent of Code Community
The Advent of Code community thrives on collaboration and sharing knowledge. Participate in online forums, join discussions on platforms like Reddit or Discord, and read through others' solutions to gain insights into different approaches. Networking with fellow participants can greatly enhance your problem-solving skills.
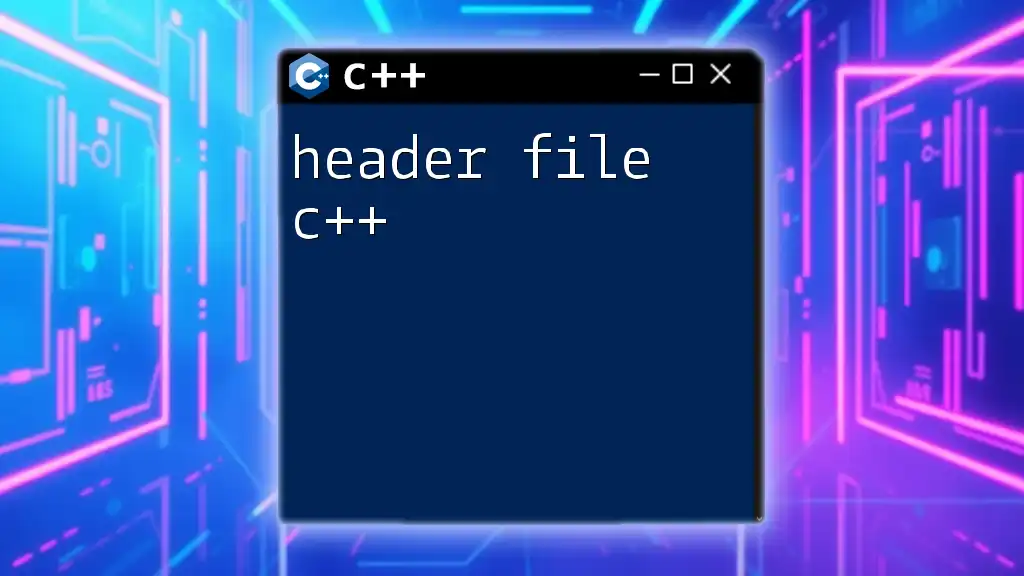
Conclusion
Participating in the Advent of Code while using C++ is a fantastic way to improve your programming capabilities. The challenges help you sharpen your skills in algorithm design, data structure utilization, and code optimization. Engaging in this event not only makes you a better developer but also connects you with a vibrant community of programmers eager to share knowledge and solutions.
As you continue to engage with the Advent of Code, remember that every problem solved is a step towards mastering C++. Don't hesitate to join a C++ learning community for additional tips and motivation. Embrace the challenges ahead and enjoy the journey!