To set up debugging for a C++ project in Visual Studio Code using a `launch.json` configuration, you can create or modify the file in your project's `.vscode` directory as follows:
{
"version": "0.2.0",
"configurations": [
{
"name": "C++ Launch",
"type": "cppdbg",
"request": "launch",
"program": "${workspaceFolder}/a.out",
"args": [],
"stopAtEntry": false,
"cwd": "${workspaceFolder}",
"environment": [],
"externalConsole": false,
"MIMode": "gdb",
"setupCommands": [
{
"description": "Enable pretty-printing for gdb",
"text": "-enable-pretty-printing",
"ignoreFailures": true
}
],
"preLaunchTask": "build",
"miDebuggerPath": "/usr/bin/gdb",
"miDebuggerArgs": "",
"setupCommands": [],
"logging": {
"engineLogging": false
}
}
]
}
Understanding `launch.json`
What is `launch.json`?
The `launch.json` file is a crucial configuration file in Visual Studio Code (VS Code) that dictates how programs are debugged. It serves as a setup for the debugger, allowing developers to customize the environment in which their applications run. This file enables detailed control over various parameters, ensuring that the debugging process is tailored to the developer's needs.
Though VS Code supports various programming languages, the usage of `launch.json` is particularly significant for C++ development. The complexity of C++ applications makes an effective debugging setup essential for successful development and troubleshooting.
Typical Use Cases for `launch.json` in C++
There are several scenarios where `launch.json` becomes invaluable, particularly when working with C++ projects:
- Debugging C++ applications: The main purpose of `launch.json` is to facilitate the debugging process, making it easier to track down issues in your code.
- Configuring build and runtime parameters: This allows you to customize various aspects of how your code is compiled and run, influencing performance and behavior during testing.
- Customizing debugging sessions: Developers can specify specific conditions and contexts for their debugging, making the process more efficient.
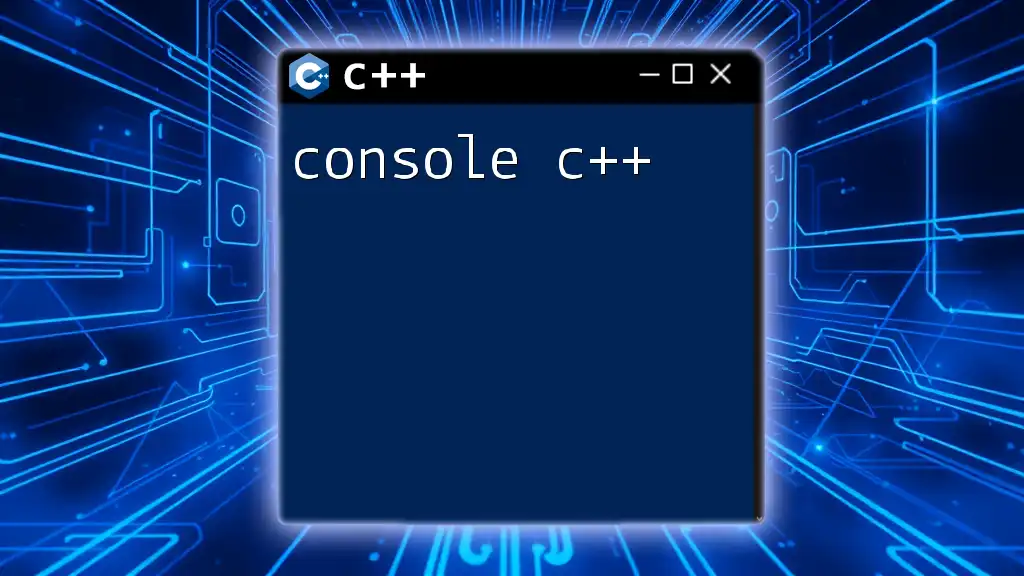
Setting Up C++ Debugging with `launch.json`
Installing Necessary Extensions
Before creating a `launch.json` file, it’s essential to have the appropriate extensions installed:
- C/C++ Extension: This is the primary extension required for C++ debugging in VS Code. It provides features like IntelliSense, code navigation, and essential debugging tools.
To install the C/C++ extension:
- Open the Extensions view (`Ctrl+Shift+X`).
- Search for "C/C++" and install the one provided by Microsoft.
Creating a New `launch.json` File
To create your `launch.json` file, follow these steps:
- Open your C++ project in VS Code.
- Navigate to the Run and Debug view (click the play icon on the sidebar or press `Ctrl+Shift+D`).
- Click "create a launch.json file" when prompted.
- Select "C++ (GDB/LLDB)" or "C++ (Windows)" based on your operating system.
You can find the `launch.json` file in the `.vscode` folder within your workspace.
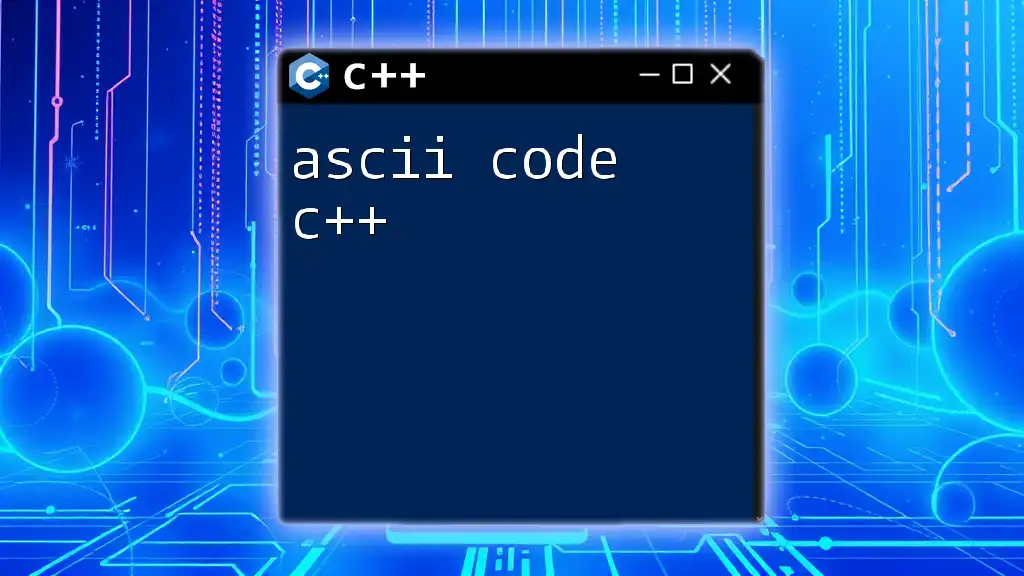
Writing Your First `launch.json` Configuration
Basic Configuration Structure
The `launch.json` file starts with a basic structure that looks like this:
{
"version": "0.2.0",
"configurations": []
}
This structure contains two key components:
- version: Defines the schema version.
- configurations: An array where you'll define various debugging configurations.
Key Fields Explained
Name
The `name` field allows you to set a recognized label for each debugging configuration, making it easier to identify later. For instance:
"name": "C++ Launch"
Type
The `type` field is crucial because it indicates the debugger to be used. For C++ applications, you should set it as follows:
"type": "cppvsdbg"
Request
The `request` field specifies whether you want to launch or attach to a running application. For most debugging scenarios, the value should be:
"request": "launch"
Program
This field points to the compiled executable you want to debug. It's essential to provide the correct path. For example, if your generated executable is in a `bin` folder, you would write:
"program": "${workspaceFolder}/bin/yourExecutable"
Pre-launch Tasks
You can configure tasks to build your C++ code before launching the debugger. This can be beneficial to ensure that you are always working with the latest changes. Here’s a snippet to add a pre-launch task:
"preLaunchTask": "build"
Complete Example of a `launch.json` for C++
Here's a comprehensive example of a `launch.json` configuration for a C++ program:
{
"version": "0.2.0",
"configurations": [
{
"name": "Launch C++ Program",
"type": "cppvsdbg",
"request": "launch",
"program": "${workspaceFolder}/bin/yourExecutable",
"args": [],
"stopAtEntry": false,
"cwd": "${workspaceFolder}",
"environment": [],
"externalConsole": false,
"MIMode": "gdb",
"setupCommands": [
{
"description": "Enable pretty-printing for gdb",
"text": "-enable-pretty-printing",
"ignoreFailures": true
}
]
}
]
}
Each component of this configuration is essential for effective debugging, and understanding each option helps in customizing it to your specific needs.
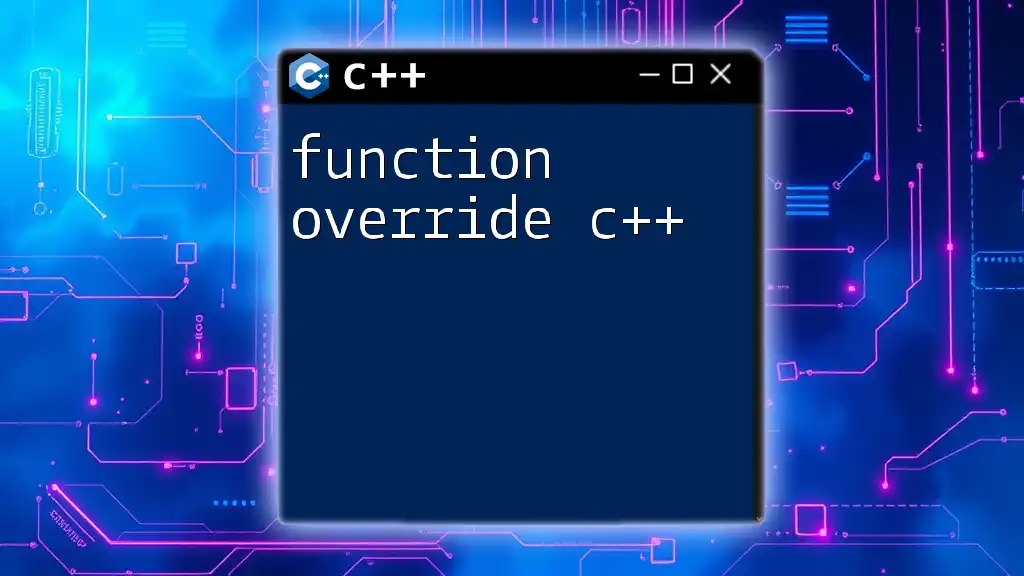
Advanced Configuration Options
Debugging with Arguments
Passing command-line arguments to your C++ application is straightforward. Use the `args` field to specify these arguments. For example, if your application expects two arguments, you would set it up like this:
"args": ["arg1", "arg2"]
Setting Environment Variables
Environment variables are often crucial for C++ applications, allowing you to configure settings dynamically. You can set these variables using the `environment` field as follows:
"environment": [
{ "name": "MY_ENV_VAR", "value": "value" }
]
Working with Multiple Configurations
For projects that require different debugging setups, you can include multiple configurations within the `configurations` array. This allows you to easily switch between setups based on your needs. For example, the following shows how to add another configuration:
{
"name": "Debug Another C++ Program",
"type": "cppvsdbg",
"request": "launch",
"program": "${workspaceFolder}/bin/anotherExecutable"
}
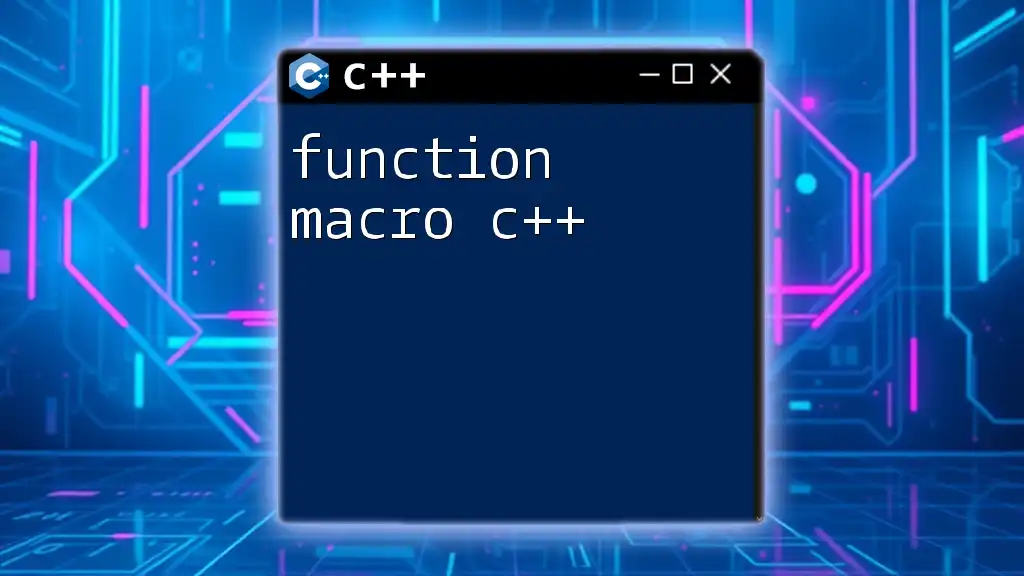
Debugging Best Practices in C++
Leveraging Breakpoints
Breakpoints are one of the most powerful features when debugging C++ code. You can set breakpoints in your code by clicking in the left margin next to the line numbers in VS Code. This allows you to pause execution at specific lines and inspect the current state of the application.
Inspecting Variables
During debugging sessions, VS Code provides robust tools for inspecting variables. You can view variable values, call stacks, and scopes in the debug panel, helping you to understand how data changes over time.
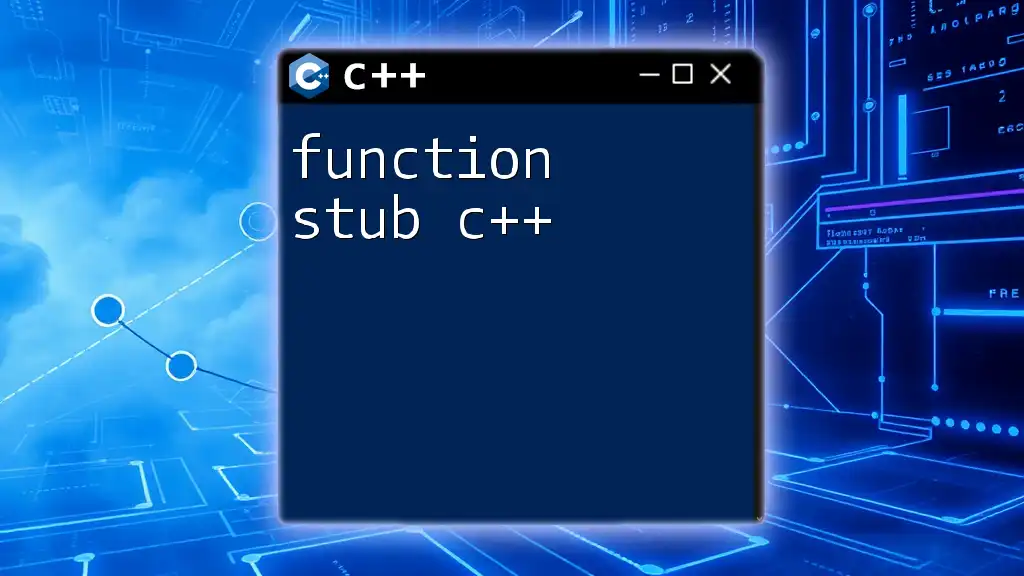
Conclusion
Incorporating a well-configured `launch.json` file into your C++ development workflow is essential for an efficient debugging experience in VS Code. By understanding the structure and functionalities available, you can tailor your configurations to fit your specific project needs, leading to faster debugging and improved code quality. Experimenting with your configurations will deepen your proficiency in using VS Code for C++ programming, enhancing your overall development skills.
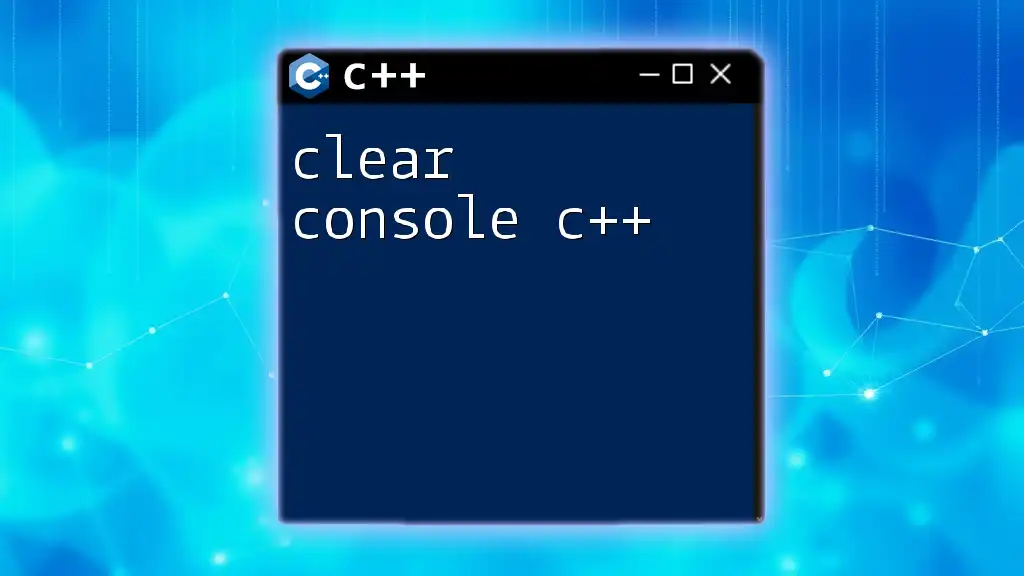
Additional Resources
For further reading and exploration, consider visiting the official VS Code documentation, where you can find more detailed explanations on `launch.json` and C++ development resources. Engaging with community forums and discussion boards can also provide answers to specific questions or challenges you may face in your development journey.