C++ version 1.13 is considered outdated and lacks many features and improvements found in newer versions, which can enhance performance and ease of use.
Here's a simple code snippet demonstrating a basic "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Overview of C++ 1.13
What is C++ 1.13?
C++ 1.13 is a notable version of the C++ programming language that emerged during its early development. It represents a stage where several foundational features were solidified and integrated into the language, paving the way for future enhancements. Understanding its role in the evolution of C++ helps to appreciate the advancements made in later versions and the programming paradigms they introduced.
Key Features of C++ 1.13
One of the defining elements of C++ 1.13 is its updates to the standard library, which enhanced functionality and usability. These enhancements include additional data structures and algorithms that were foundational for modern programming.
- Compiler Improvements: In this version, several optimizations were made to improve the efficiency of code compilation, reducing compile times and enhancing the performance of the generated executables. These improvements represent a significant leap toward the eventual optimizations seen in later releases.
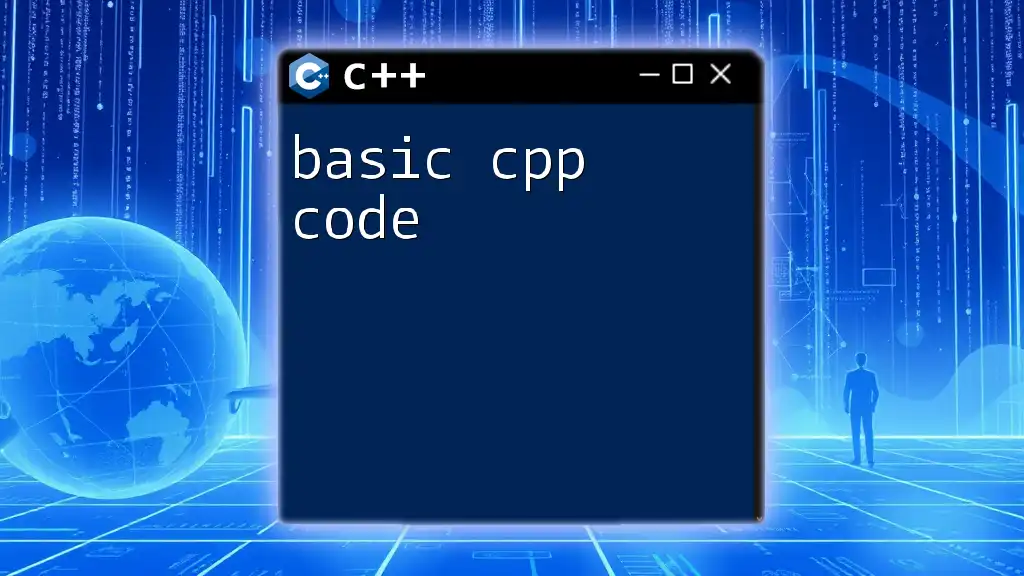
Comparing C++ 1.13 with Other Versions
Major Improvements Over Previous Versions
C++ 1.13 presented several improvements that distinguished it from C++ 1.12 and earlier versions. One significant advancement was syntax changes that added clarity and flexibility to the code. For instance, improvements in operator overloading and type conversion made it easier for developers to work with complex data types.
Example: New Syntax Changes
With the enhancements in C++ 1.13, developers gained access to better arrays and pointers management. Here’s a brief example demonstrating how adaptable arrays became:
#include <iostream>
int main() {
int arr[5] = {1, 2, 3, 4, 5};
for (int i = 0; i < 5; i++) {
std::cout << arr[i] << " ";
}
return 0;
}
This syntax provided a more intuitive way for programmers to handle arrays and loops.
Example: Performance Metrics
Benchmarking during this phase often revealed significant performance improvements, with C++ 1.13 displaying faster execution times in various algorithms compared to its predecessors. Such performance metrics are important for developers making decisions about which version to adopt.
Limitations of C++ 1.13
Despite its improvements, C++ 1.13 is not without its limitations. One of the primary concerns is its lack of compatibility with newer C++ standards. As development progressed, many modern features and updates were introduced that have become staples in C++ programming today. By choosing C++ 1.13, developers may miss out on:
- Advanced type inference
- Smart pointers and memory management improvements
- Enhanced standard libraries and language features introduced in later versions.
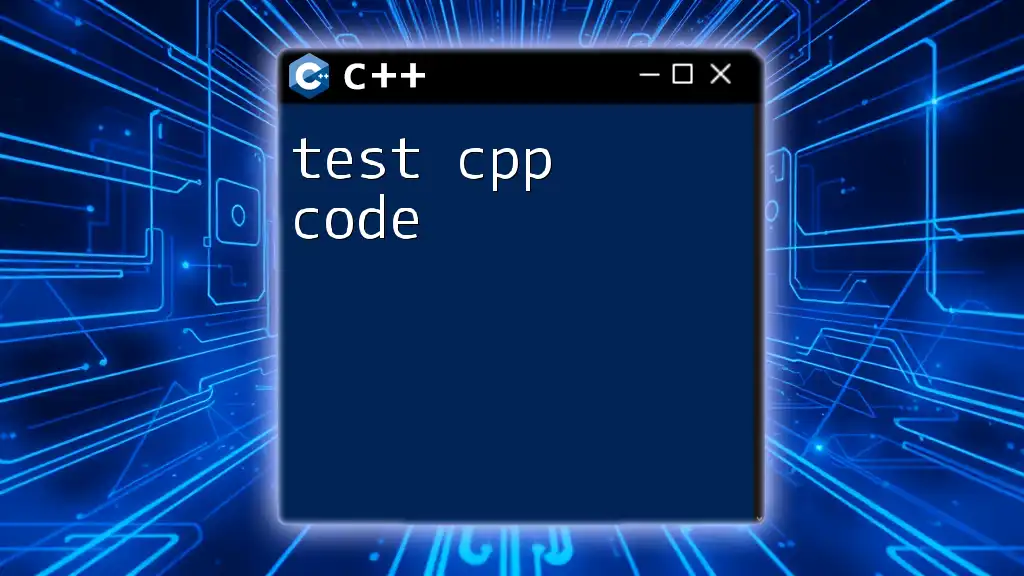
Practical Applications of C++ 1.13
Ideal Use Cases for C++ 1.13
C++ 1.13 is particularly suitable for specific applications, particularly in resource-constrained environments. For instance:
- Systems Programming: The version is still functional for low-level programming, making it suitable for operating systems or hardware interfaces.
- Game Development: Early game engines that do not require modern graphics or extensive libraries can efficiently utilize C++ 1.13.
Code Examples
Here are some code examples to illustrate the capabilities of C++ 1.13:
Basic Syntax Example
One straightforward program was to print "Hello, World!" and demonstrate basic I/O:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This simple snippet highlights the essential structure of C++ programming, which remains relevant across various versions.
New Features in Action
Here’s another example involving the use of updated data structures like arrays:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
This demonstrates a key capability of C++ 1.13 in managing collections of data effectively.
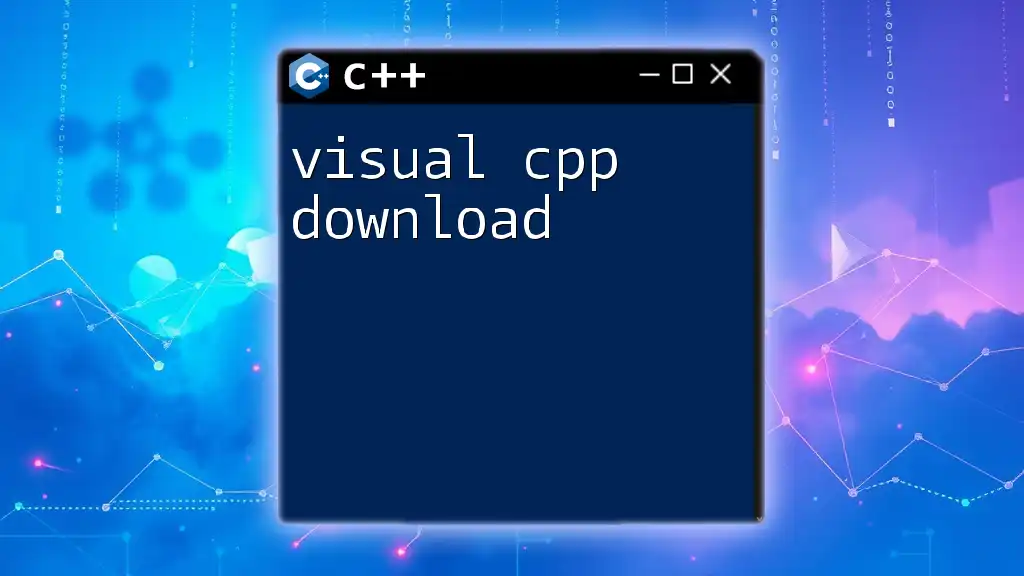
Community Feedback and Adoption
Developer Opinions
Community feedback regarding C++ 1.13 has been mixed. While some developers appreciate its simplicity and the lower learning curve it provides for newcomers, others criticize it for lacking modern features. Forums, blogs, and discussions across platforms reveal varying perspectives, often depending on developer background and project requirements.
Adoption Rates
Stats indicate that C++ 1.13 was never overwhelmingly popular in large-scale applications. As the programming landscape evolved with the introduction of C++11 and beyond, many developers transitioned to these newer options for their superior features and performance enhancements.
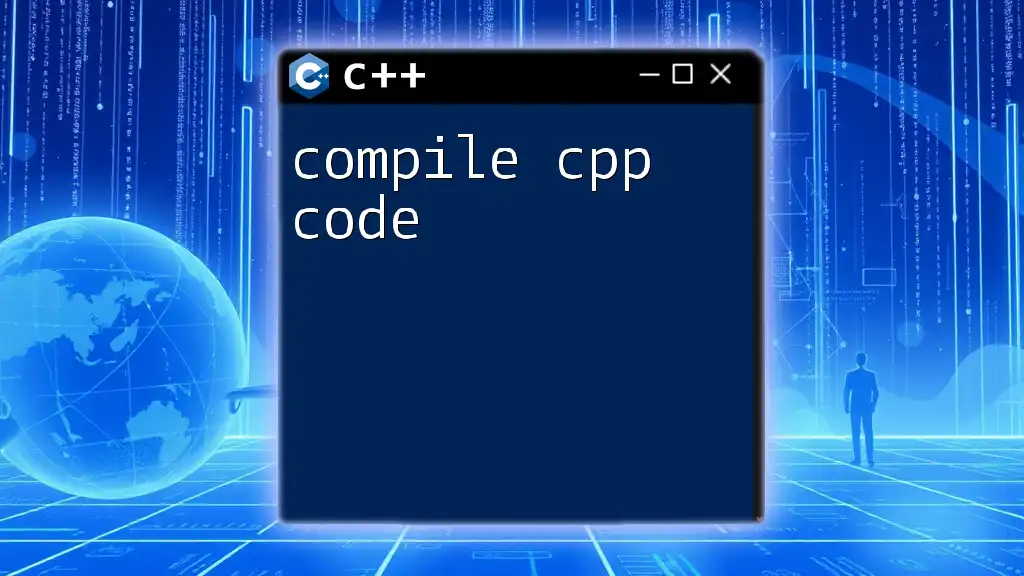
Should You Use C++ 1.13?
Pros and Cons
-
Pros:
- Simplicity and ease of use for those new to programming, making it approachable for beginners.
- Reliable performance for certain applications that do not require the latest features or optimizations.
-
Cons:
- Lack of modern features, compared to more recent versions like C++11 and C++14, which incorporate significant advancements.
- Potential issues with long-term support as newer versions gain traction, leaving C++ 1.13 less maintained.
Best Practices for Using C++ 1.13
If you decide to work with C++ 1.13, consider employing the following best practices:
- Focus on building foundational skills in C++, which will serve as a great base for mastering newer versions later.
- Stay aware of compatibility issues that may arise if you decide to transition to a more modern C++ version in the future, particularly if you begin a new project.
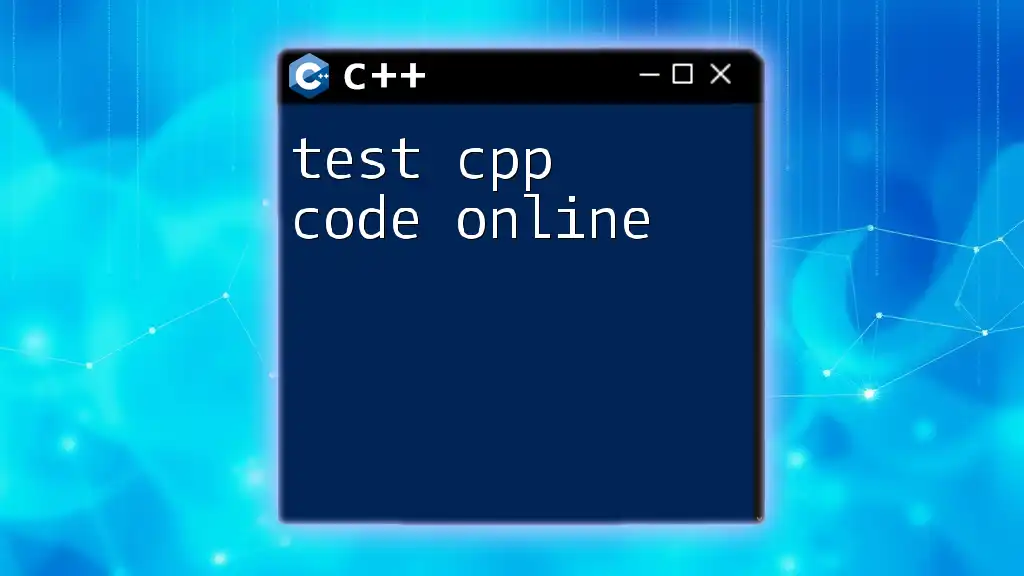
Conclusion
In summary, is 1.13 cpp good? It's clear that C++ 1.13 has its merits, especially for certain types of applications. However, its limitations and lack of modern features inevitably lead many developers to choose more recent versions of C++. Understanding its role in the evolution of C++ can significantly influence your decision on whether to adopt it for your projects.
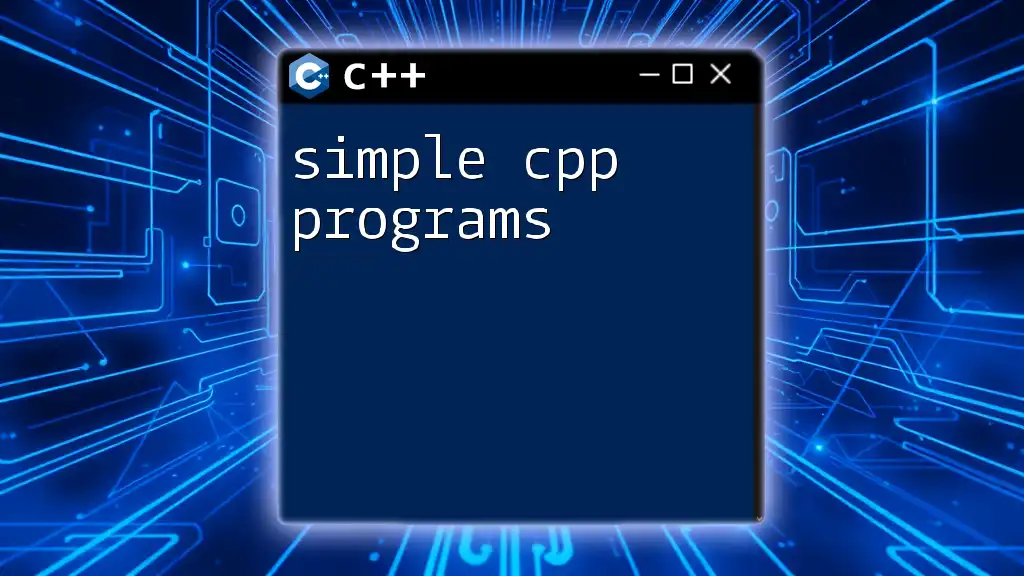
Call to Action
We encourage you to share your experiences and thoughts about C++ 1.13 in the comments below. Have you used this version in your projects, and what has been your experience? Also, consider signing up for our newsletter for more articles and insights into C++ and command usage. Your feedback helps shape the content we create!