In C++, the value `0` is considered `false` in boolean contexts, while any non-zero value is considered `true`.
Here's a code snippet demonstrating this:
#include <iostream>
int main() {
int value = 0;
if (value) {
std::cout << "True" << std::endl;
} else {
std::cout << "False" << std::endl; // This will be printed
}
return 0;
}
Understanding Boolean Values in C++
The `bool` type in C++ is fundamental for managing the flow of programs. This type can hold one of two possible values: `true` or `false`. Understanding how these values work, especially in relation to numeric values like 0, is crucial for effective programming.
In C++, expressions that yield a boolean value can affect the control structure of your code, particularly in conditional statements and loops. Knowing what constitutes a truthy or falsy value helps in writing clear and logical code.
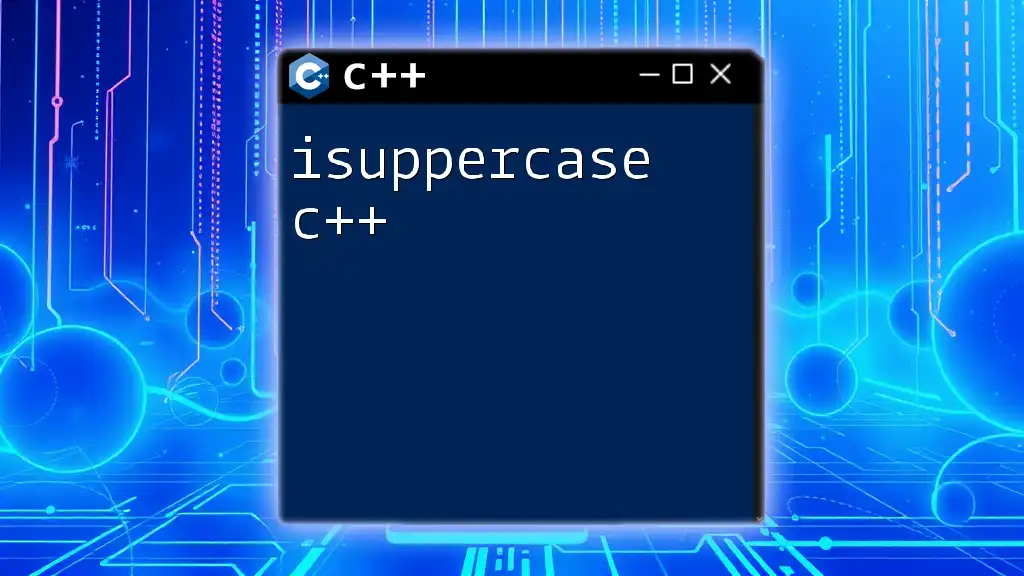
The Role of Zero in C++
Zero's representation in C++ is straightforward and can lead to interesting behavior when it comes to boolean evaluation.
Zero as a Numeric Value
In C++, zero can be represented both as an integer and as a floating-point number. Regardless of its form, zero always logically equates to false when evaluated in a boolean context. This fundamental principle makes it essential for programmers to recognize how zero operates.
Zero and Boolean Evaluation
When zero is used in conditional statements, C++ interprets it as `false`. Here is a simple illustration:
int value = 0;
if (value) {
// This block will not execute
} else {
// This block will execute, as value is false
}
In this example, since `value` is zero, the condition evaluates to false, and the corresponding block under else will be executed. This behavior exemplifies the principle that zero evaluates to false in the realm of boolean logic in C++.
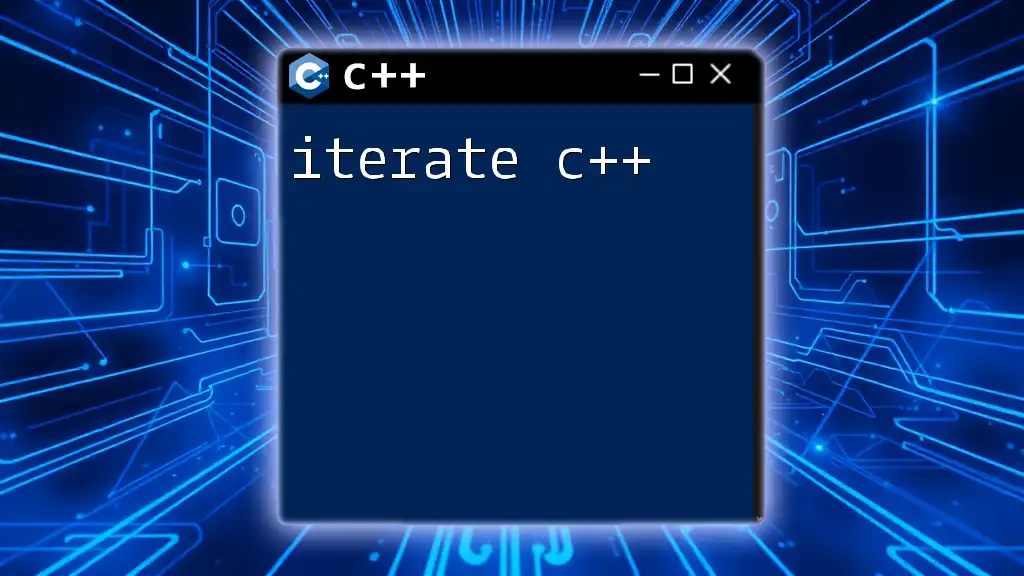
Real-world Examples of Zero in Conditionals
Understanding how zero behaves in conditional statements can help you avoid familiar pitfalls when writing or interpreting C++ code.
Zero in Loops and Conditionals
Consider how zero can influence a loop condition. The following example demonstrates that using zero will result in the loop not executing:
int counter = 0;
while (counter) {
// This block will not execute
counter--;
}
Here, the condition `while (counter)` evaluates to false because `counter` is zero. Hence, the loop body does not execute at all. It emphasizes that using a variable set to zero will lead to immediate exit from the loop.
Comparative Evaluation with Non-zero Values
In contrast, non-zero values are treated as true. The following code snippet highlights this behavior:
int value = 5;
if (value) {
// This block will execute
}
With `value` set to 5, the condition evaluates to true, and the corresponding block of code executes. This demonstrates how non-zero integers affirmatively trigger execution, reinforcing the general rule: non-zero is true, whereas zero is false.
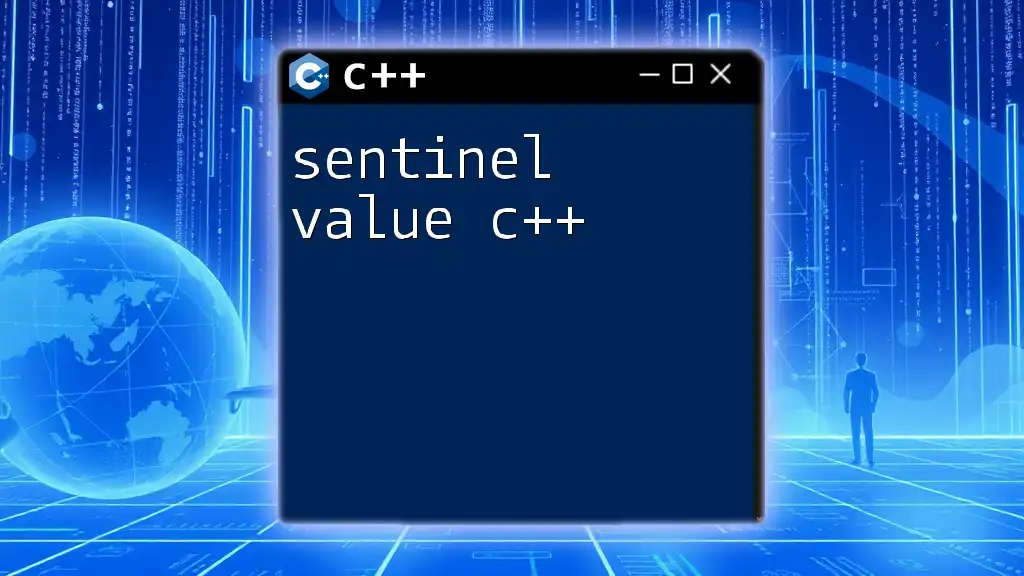
Misconceptions Regarding Zero in C++
Misunderstanding zero's boolean nature can lead to frustrating and erroneous behaviors in C++ programming. Some common misconceptions might include:
-
Assuming Zero Can Be True: Some novice programmers mistakenly believe that using zero in logical expressions will yield a true result. This misunderstanding leads to logic errors and unexpected behavior in code execution.
-
Confusing Contexts: Beginners might confuse contexts where zero could be accepted as true in different programming languages; however, in C++, zero is consistently evaluated as false.
Recognizing these misconceptions can enhance debugging skills and improve overall programming confidence.
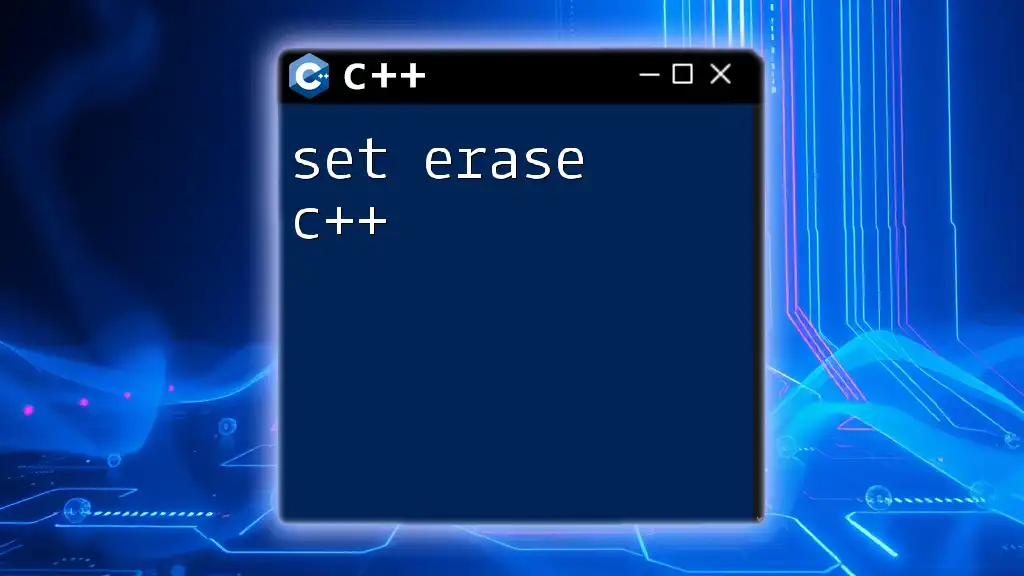
Best Practices in C++
With a clearer understanding of how zero operates within boolean contexts, there are best practices to consider:
-
Explicitly Use `bool` Types: For clarity, utilize the `bool` type whenever possible for true/false logic. This practice reduces ambiguity about the intention of your code.
-
Be Cautious with Numeric Comparisons: Avoid using numeric types in place of boolean expressions. It not only makes code harder to read but can also lead to unexpected behavior.
By following these best practices, you can create cleaner, more understandable, and more effective C++ code.
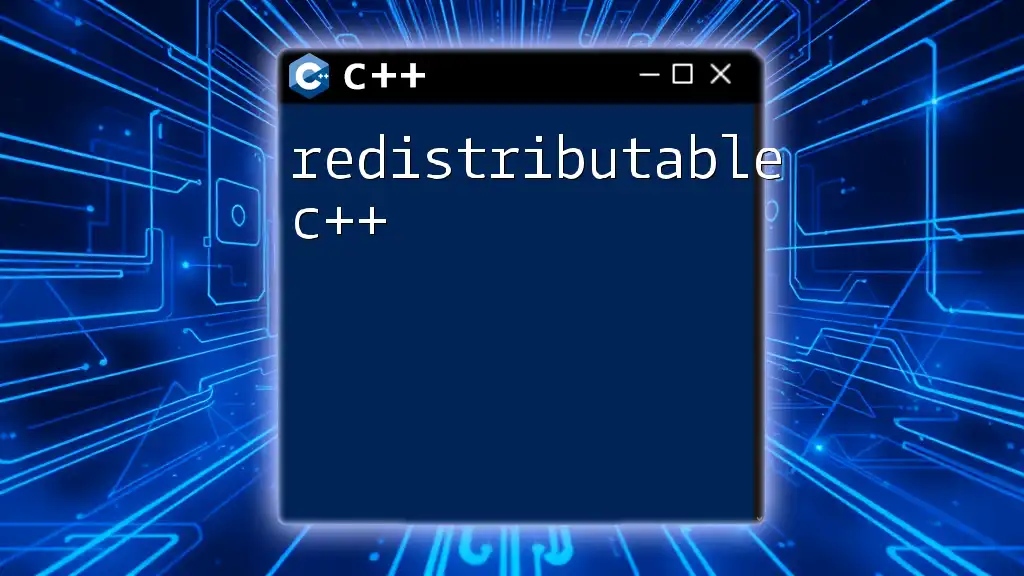
Conclusion
In summary, the question of is 0 true or false in C++? is answered succinctly: zero is false. A strong grasp of boolean evaluation in C++ is vital for effective programming. Understanding how numeric values correlate with boolean logic will enhance code quality and help avoid common pitfalls in control structures.
To further your understanding of these concepts, engage with code examples, review documentation, and consider experimenting with different values in various contexts. Embracing this knowledge will undoubtedly make you a more proficient C++ developer.
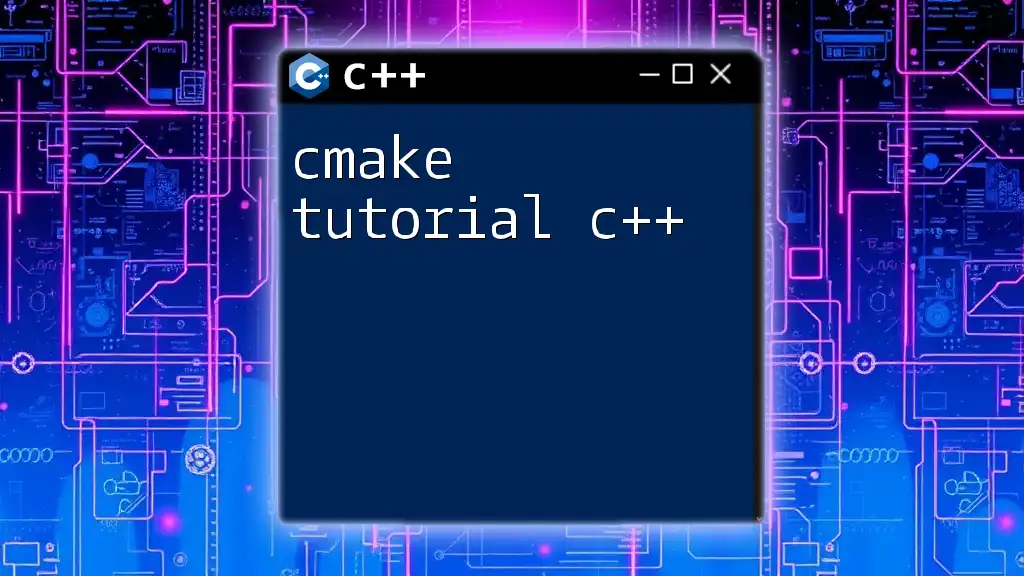
Additional Resources
For those looking to deepen their understanding of C++, consider exploring books, online courses, and tutorials that cover more advanced topics. Your journey into C++ programming will only be enhanced by practicing what you learn and iterating on your code consistently.