C++ can be used to bridge the gap to C by allowing C-style syntax and features, enabling developers to write C code within a C++ program seamlessly.
Here's an example of a simple C program written in C++:
#include <iostream>
extern "C" {
void myFunction() {
std::cout << "Hello from C function!" << std::endl;
}
}
int main() {
myFunction();
return 0;
}
Understanding the Differences Between C++ and C
Syntax Differences
C and C++ have developed significantly over time, resulting in some key syntactical variations. While both languages share many similarities, especially in their foundational structure, the particular nuances can become evident in their syntax.
For example, a simple declaration and print statement in C++ may look like this:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In contrast, the equivalent C code would be:
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
Object-Oriented Programming vs Procedural Programming
One of the most significant differences between C++ and C lies in their programming paradigms. C++ is built on object-oriented principles, while C remains a procedural language.
In C++, you can define a class to encapsulate data and functions, as shown in the following code snippet:
class Greeting {
public:
void sayHello() {
std::cout << "Hello from C++!" << std::endl;
}
};
int main() {
Greeting greet;
greet.sayHello();
return 0;
}
For converting this into C, you would typically use a function, as C does not support classes:
#include <stdio.h>
void sayHello() {
printf("Hello from C!\n");
}
int main() {
sayHello();
return 0;
}
Memory Management
Memory management also shows notable differences between C++ and C. C++ utilizes new and delete for dynamic memory allocation and deallocation, whereas C relies on malloc and free.
C++ example:
int* arr = new int[10]; // allocation
delete[] arr; // deallocation
In C, the equivalent would look like this:
int* arr = (int*)malloc(10 * sizeof(int)); // allocation
free(arr); // deallocation
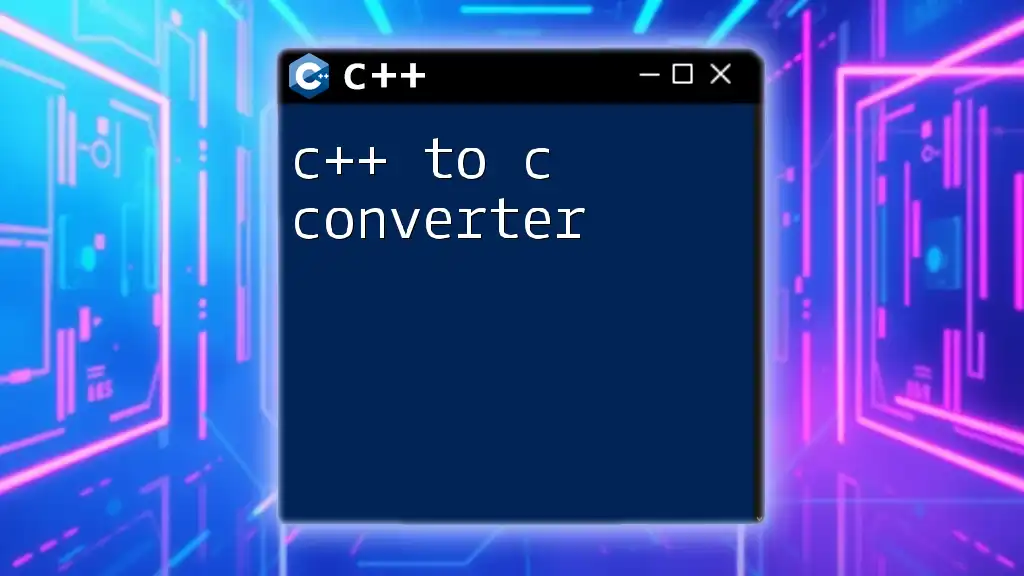
Converting C++ Code to C
Basic Conversion Techniques
When converting C++ code to C, the primary goal is to strip away C++ specific constructs. Start by identifying components specific to C++, such as classes, objects, and certain advanced data types.
Key Constructs in C++ Not Supported in C
Classes and Objects
When encountering C++ classes, convert them into C structs. For example, a simple C++ class:
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
};
can be translated to C as follows:
struct Point {
int x;
int y;
};
void initPoint(struct Point* p, int x, int y) {
p->x = x;
p->y = y;
}
Function Overloading
In C++, you can define multiple functions with the same name but different parameters—a feature called function overloading. For example:
void display(int i) {
std::cout << "Integer: " << i << std::endl;
}
void display(double d) {
std::cout << "Double: " << d << std::endl;
}
To convert this for C, you'll need to rename your functions:
void displayInt(int i) {
printf("Integer: %d\n", i);
}
void displayDouble(double d) {
printf("Double: %f\n", d);
}
Templates
Templates are another C++ feature not present in C. If a C++ function is templated, such as:
template <typename T>
void printArray(T arr[], int size) {
for (int i = 0; i < size; i++)
std::cout << arr[i] << " ";
}
You may need to define different functions for each data type in C. Here's a C example for integers:
void printIntArray(int arr[], int size) {
for (int i = 0; i < size; i++)
printf("%d ", arr[i]);
}
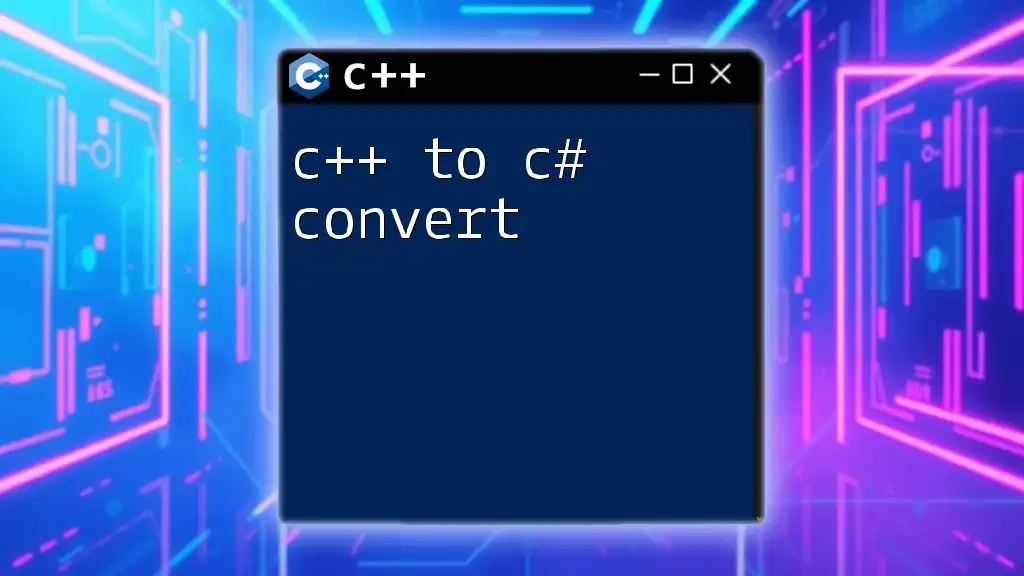
Using a C++ to C Code Converter
Introduction to C++ to C Code Converters
Automation can simplify the process of converting C++ code to C, especially for large projects. C++ to C code converters are specialized tools that help transition codebases with minimal manual work.
Popular C++ to C Code Converter Tools
Several converters such as Cxx2c and C2C have been developed to assist programmers in this task. These tools perform automated analysis of C++ code and provide equivalent C code as output.
How to Use a Code Converter
Let’s go through how to use a typical converter.
- Select the code snippet you wish to convert.
- Paste it into the converter tool.
- Review the generated C code, making manual adjustments as needed to ensure accuracy and efficiency.
Here’s an example of how a C++ code snippet:
#include <iostream>
class MyClass {
public:
void display() {
std::cout << "Displaying MyClass" << std::endl;
}
};
might be converted via a tool into:
#include <stdio.h>
struct MyClass {
};
void display(struct MyClass* obj) {
printf("Displaying MyClass\n");
}
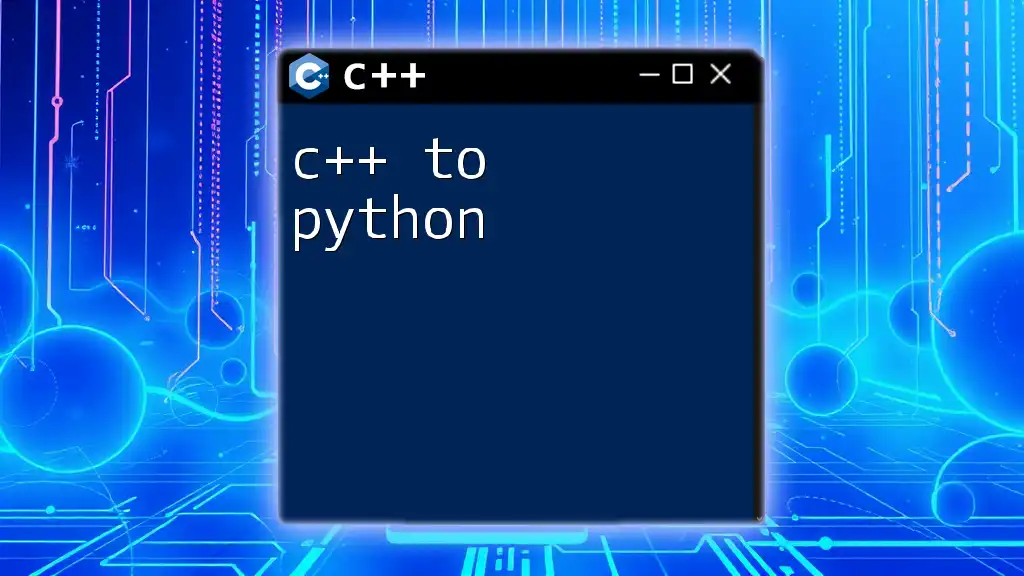
Best Practices for Efficient C++ to C Conversion
Refactoring C++ Code for Simplicity
Ensure that your C++ code is as concise and clear as possible before converting. Simplifying code can help facilitate a smoother transition. Look for opportunities to refactor and eliminate unnecessary complexities.
Testing Post-Conversion
After converting C++ to C, thorough testing is crucial to ensure that the functionality remains intact. This might involve creating a suite of unit tests specifically for the new C code.
Utilize testing and debugging tools like Valgrind for memory management verification and GDB for tracking down any runtime errors, as they can help in identifying potential issues that arise from the conversion process.
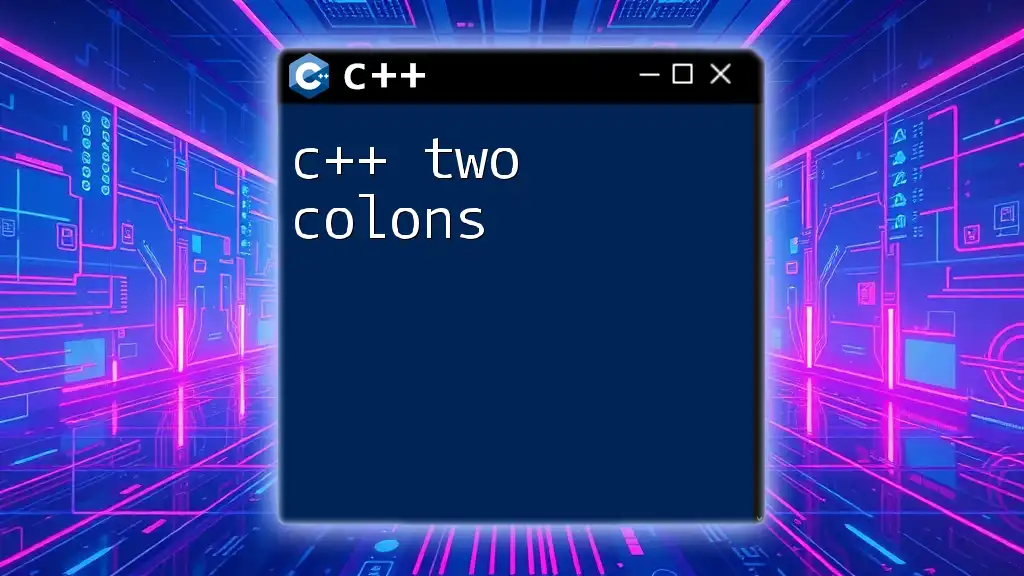
Conclusion
As you transition from C++ to C, keep in mind the numerous differences in syntax, programming paradigms, and code structure. The process can be complex, but with the availability of code converters and a solid understanding of both languages, achieving a successful conversion is entirely feasible.
By continuously refining your code and leveraging the community's resources, you’ll improve your proficiency in both C++ and C, ultimately enhancing your programming skill set.
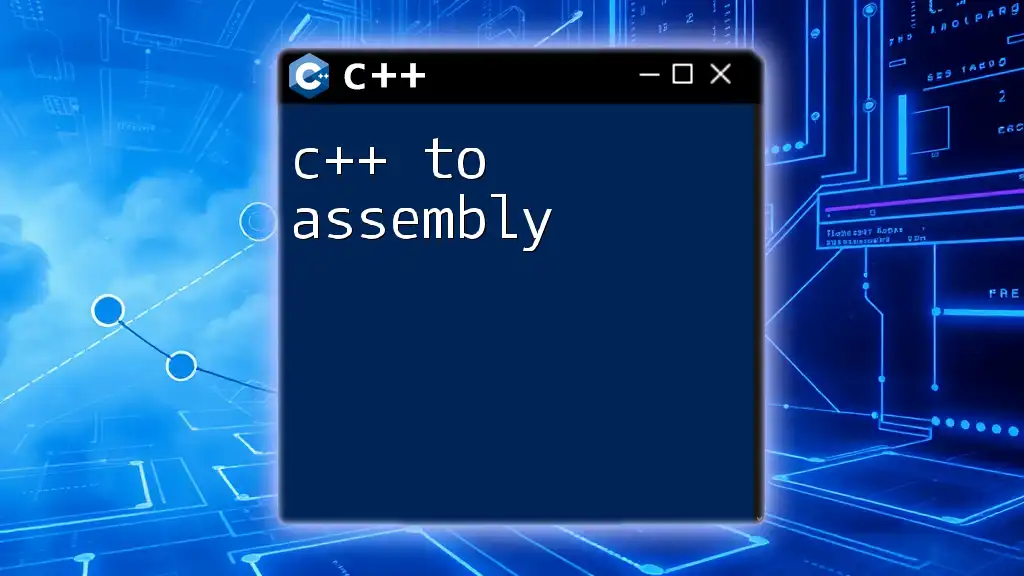
Additional Resources
Recommended Reading Materials
Dig into recommended books and online courses that focus on C and C++ programming for a deeper understanding and mastery of the languages.
Community and Forums for Further Learning
Engagement with communities on platforms such as Stack Overflow and Reddit’s C/C++ subreddits can provide invaluable support as you explore the intricacies of C and C++.