In this post, we will demonstrate a simple C++ implementation of the classic Rock, Paper, Scissors game, where users can play against the computer by inputting their choice.
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
srand(static_cast<unsigned int>(time(0)));
std::string choices[3] = {"Rock", "Paper", "Scissors"};
int computerChoice = rand() % 3;
std::string userChoice;
std::cout << "Enter Rock, Paper, or Scissors: ";
std::cin >> userChoice;
std::cout << "Computer chose: " << choices[computerChoice] << std::endl;
if (userChoice == choices[computerChoice]) {
std::cout << "It's a tie!" << std::endl;
} else if ((userChoice == "Rock" && choices[computerChoice] == "Scissors") ||
(userChoice == "Paper" && choices[computerChoice] == "Rock") ||
(userChoice == "Scissors" && choices[computerChoice] == "Paper")) {
std::cout << "You win!" << std::endl;
} else {
std::cout << "You lose!" << std::endl;
}
return 0;
}
Understanding the Basics of C++
C++ is a powerful programming language known for its versatility and efficiency. Originally developed as an extension of the C programming language, C++ incorporates object-oriented features, which make it a popular choice among developers for a variety of applications, including game development.
Before diving into coding, it's crucial to set up your C++ environment. Some recommended Integrated Development Environments (IDEs) are Visual Studio, Code::Blocks, and CLion. Each provides tools to write, compile, and run C++ code effectively. Make sure you have a C++ compiler installed to execute your programs.
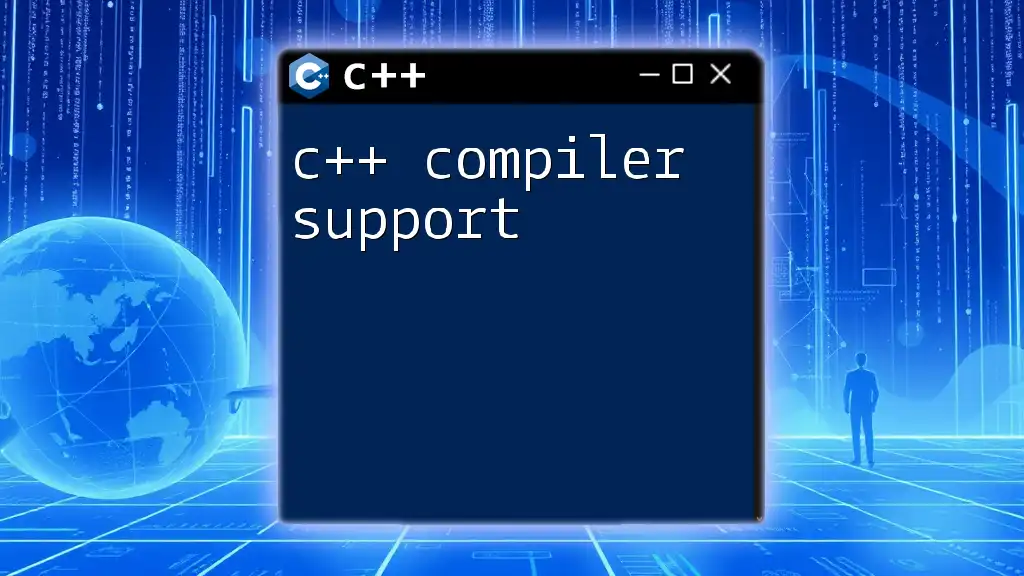
Getting Started with the C++ Rock Paper Scissors Game
To effectively implement a C++ rock paper scissors game, it’s vital to first understand the game’s mechanics. The game is straightforward: players choose one of three options—rock, paper, or scissors—and compare their selections. The rules are simple:
- Rock crushes scissors.
- Scissors cuts paper.
- Paper covers rock.
Once you grasp these basics, it's time to conceptualize your C++ project structure. A well-organized project usually includes a single source file (let’s call it `rock_paper_scissors.cpp`) where you’ll implement all necessary code.
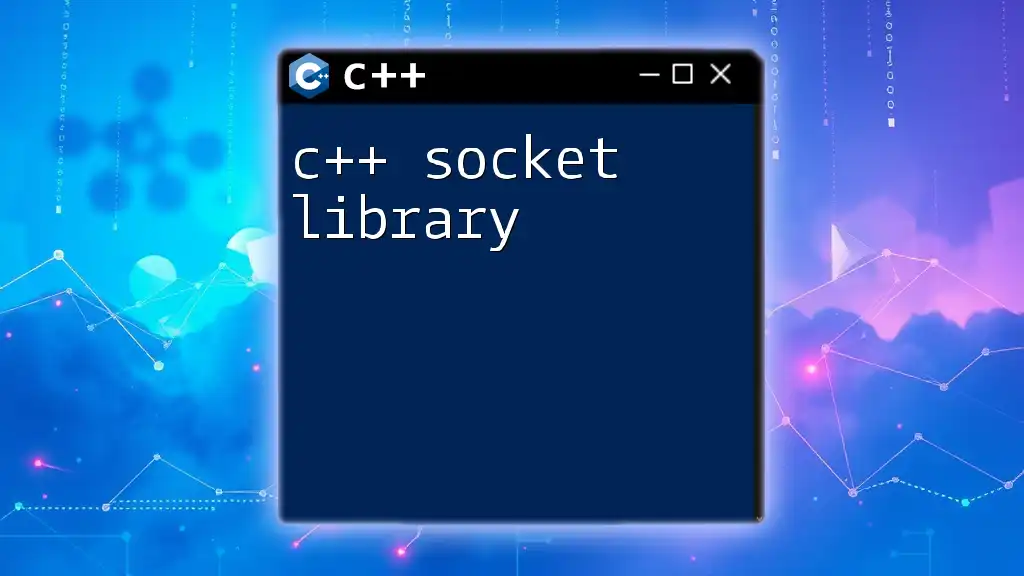
Coding the Rock Paper Scissors Game
Initializing the Game
To start implementing the game, you will need to utilize constants and variables. Constants will represent the different choices: Rock, Paper, and Scissors. Here's an example snippet:
const int ROCK = 0;
const int PAPER = 1;
const int SCISSORS = 2;
These constants help make your code more readable. Instead of using numbers directly, using defined constants makes it easier to understand what each value represents.
Gathering Player Input
After initializing your variables, the next step is to gather input from the player. We will use the `cin` function for this purpose:
int playerChoice;
std::cout << "Enter your choice (0 = Rock, 1 = Paper, 2 = Scissors): ";
std::cin >> playerChoice;
It’s important to validate the input to ensure the player enters a valid number (0, 1, or 2). You can add error-checking logic to handle invalid entries, which is essential in building robust programs.
Generating Computer Choice
To enhance the gaming experience, the computer must also make a choice. C++ allows you to use random number generation to simulate the computer’s selection. Here’s how to do it:
srand(time(0)); // Seed the random number generator
int computerChoice = rand() % 3; // Generate a number between 0 and 2
The `srand(time(0))` line seeds the random number generator with the current time, ensuring that you get different results each time the program runs.
Determining the Winner
Next, you need to implement the logic that determines the winner of each round. This is where condition statements come into play. Here’s how you can structure your code:
if (playerChoice == computerChoice) {
std::cout << "It's a tie!" << std::endl;
} else if ((playerChoice == ROCK && computerChoice == SCISSORS) ||
(playerChoice == PAPER && computerChoice == ROCK) ||
(playerChoice == SCISSORS && computerChoice == PAPER)) {
std::cout << "You win!" << std::endl;
} else {
std::cout << "Computer wins!" << std::endl;
}
This block of code checks the player’s choice against the computer’s choice to determine the outcome of the game.
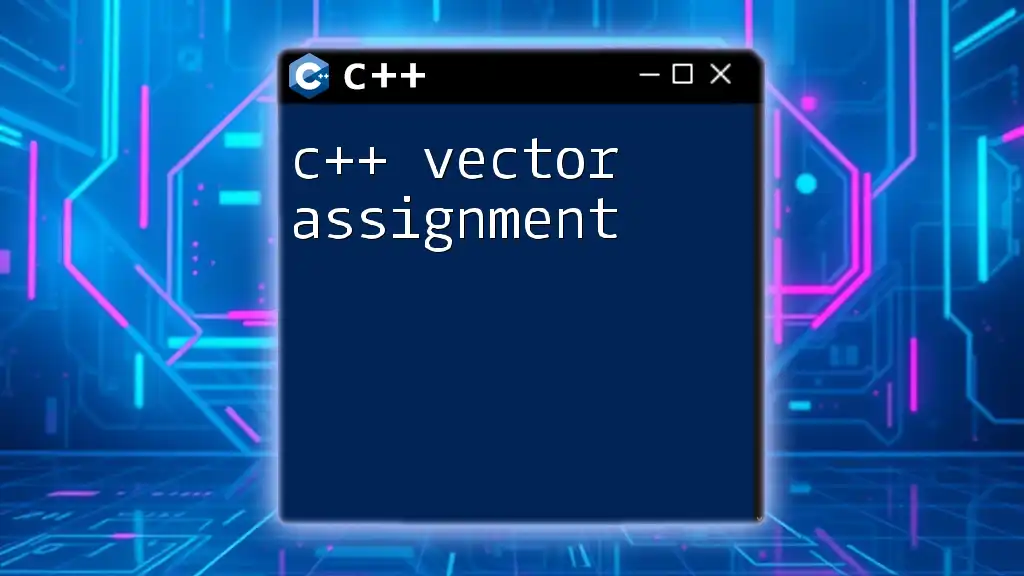
Complete Code Walkthrough
Now that you have all the components, let’s put them together into a single program. Below is the full implementation of a simple C++ rock paper scissors game:
#include <iostream>
#include <cstdlib>
#include <ctime>
const int ROCK = 0;
const int PAPER = 1;
const int SCISSORS = 2;
int main() {
srand(time(0));
int playerChoice;
std::cout << "Enter your choice (0 = Rock, 1 = Paper, 2 = Scissors): ";
std::cin >> playerChoice;
int computerChoice = rand() % 3;
if (playerChoice == computerChoice) {
std::cout << "It's a tie!" << std::endl;
} else if ((playerChoice == ROCK && computerChoice == SCISSORS) ||
(playerChoice == PAPER && computerChoice == ROCK) ||
(playerChoice == SCISSORS && computerChoice == PAPER)) {
std::cout << "You win!" << std::endl;
} else {
std::cout << "Computer wins!" << std::endl;
}
return 0;
}
This complete code provides the core functionality of the C++ rock paper scissors game.
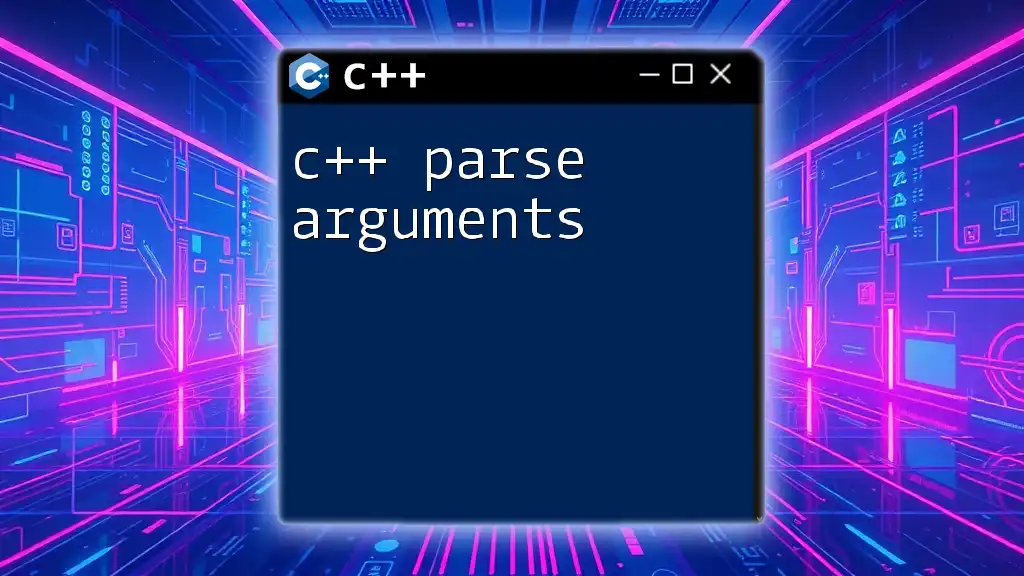
Enhancements and Extensions
Adding Replay Functionality
To make your game more engaging, you can add the option for players to replay. This can be achieved by implementing a loop. Consider the following code snippet:
char playAgain;
do {
// Game logic here
std::cout << "Play again? (y/n): ";
std::cin >> playAgain;
} while (playAgain == 'y');
This loop allows the player to play multiple rounds until they choose to stop.
Introducing Scoring System
You can elevate your game by incorporating a scoring system that tracks the number of wins, losses, and ties. This enhances engagement and makes the game more competitive. You can modify the game logic like this:
int playerScore = 0;
int computerScore = 0;
// Update scores based on game outcomes
This simple enhancement adds a layer of complexity and fun to the game, encouraging players to aim for higher scores.
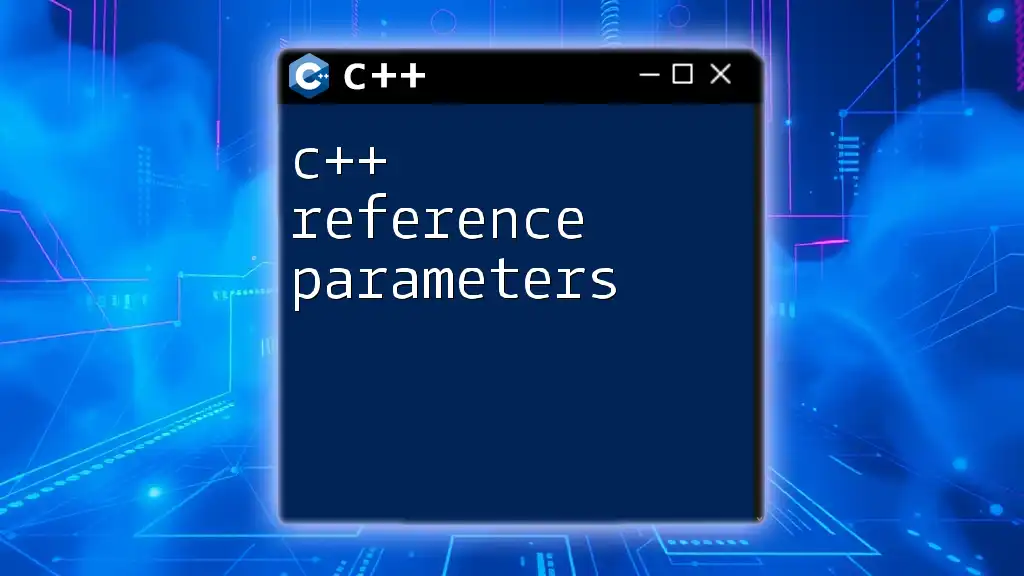
Conclusion
Through this article, you’ve gained a comprehensive understanding of how to build a C++ rock paper scissors game. From conceptualizing the game mechanics to coding the complete program, each step reinforced key programming concepts.
By working on small projects like this, you can sharpen your C++ skills effectively. Feel free to experiment—try adding new features, improving the user interface, or even converting it into a more complex game.
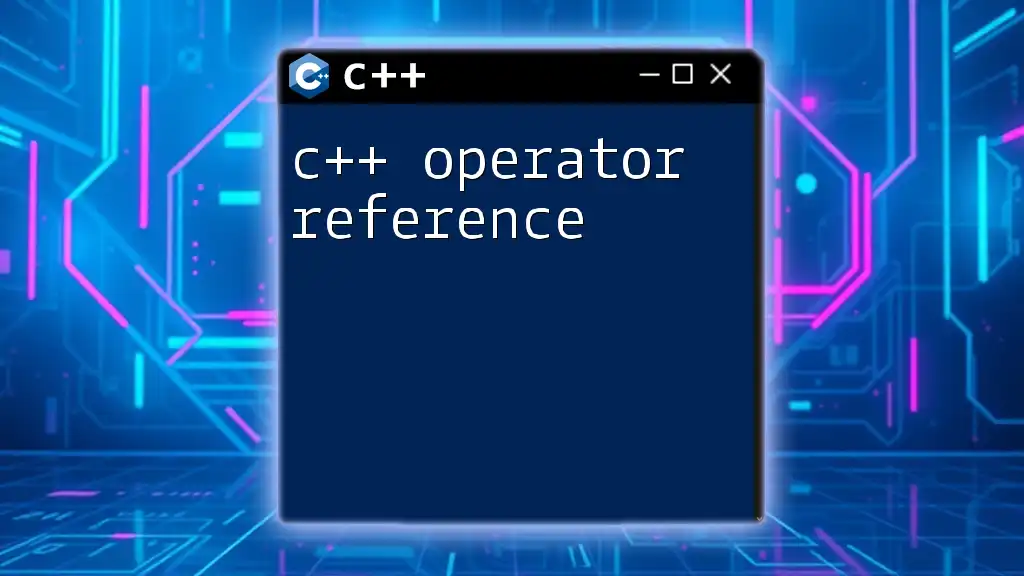
Call to Action
We invite you to share your versions of the game or any questions you might have! Engage with fellow learners and explore additional resources to further your journey in C++ programming and game development.