In C++, "pid" typically refers to the Process ID, an identifier assigned by the operating system to distinguish between running processes, which can be retrieved using the `getpid()` function from the `<unistd.h>` library.
Here's a simple code snippet demonstrating how to obtain the Process ID:
#include <iostream>
#include <unistd.h>
int main() {
pid_t process_id = getpid();
std::cout << "Process ID: " << process_id << std::endl;
return 0;
}
Understanding PID in C++
What is PID?
PID stands for Proportional-Integral-Derivative, a control loop feedback mechanism widely used in industrial control systems. The purpose of PID is to continuously calculate an error value as the difference between a desired setpoint and a measured process variable. The PID controller applies a correction based on three distinct parameters: proportional, integral, and derivative.
An effective PID controller plays a crucial role in various applications like temperature control, speed regulation, and robotics, ensuring smooth and stable transitions towards the desired state.
The Role of C++ in Implementing PID
C++ is an excellent choice for implementing PID algorithms due to its combination of performance and flexibility. The language allows for fine-grained control over system resources and offers object-oriented features, enabling the creation of reusable and maintainable PID controller classes. In applications requiring real-time processing, C++ can handle computations efficiently, making it ideal for systems where timing and responsiveness are critical.
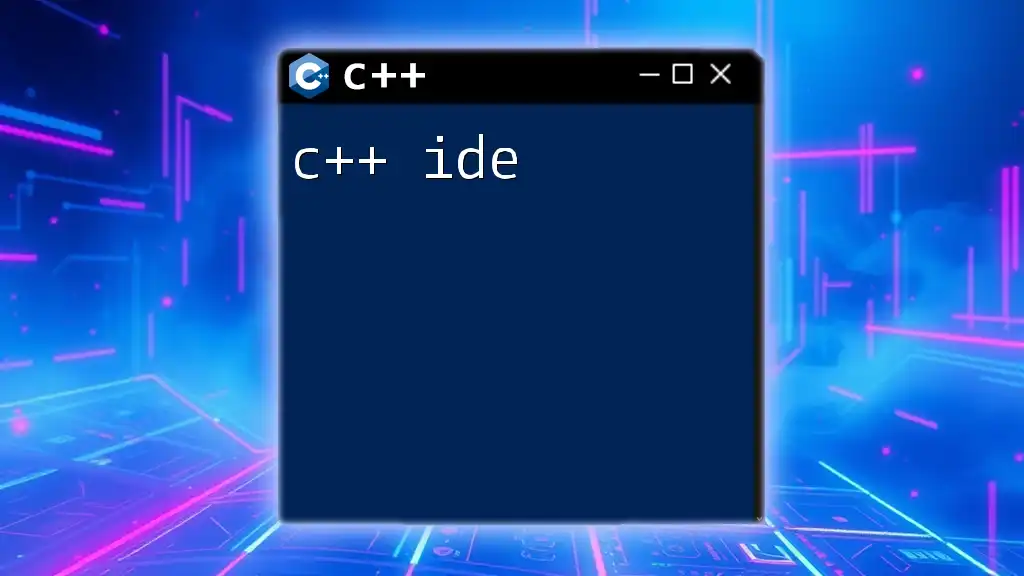
Basic Concepts of PID Control
Proportional Control
Proportional control determines the output based on the present error value. The proportional gain (Kp) indicates how strongly the controller responds to the current error.
A larger Kp value tends to minimize the error faster but can also cause overshooting and oscillations if set too high.
Example Code Snippet Illustrating Proportional Control
double proportionalControl(double Kp, double error) {
return Kp * error;
}
Integral Control
The integral control sums up past errors to eliminate steady-state errors that may persist over time. The integral gain (Ki) adds a corrective term based on the accumulation of error in the past, influencing the output to address long-term discrepancies.
One downside is the risk of integral windup, where excessive accumulation of errors leads to instability. Implementing a mechanism to reset the integral term when the output exceeds certain limits can mitigate this issue.
Example Code Snippet Demonstrating Integral Control
double integralControl(double Ki, double integral, double error, double dt) {
integral += error * dt;
return Ki * integral;
}
Derivative Control
Derivative control predicts future error by determining the rate of change of the error. This is achieved through the derivative gain (Kd), which dampens the system's response and enhances stability.
A well-tuned derivative term can significantly improve response time and minimize overshoot.
Example Code Snippet for Derivative Control
double derivativeControl(double Kd, double error, double prev_error, double dt) {
double derivative = (error - prev_error) / dt;
return Kd * derivative;
}
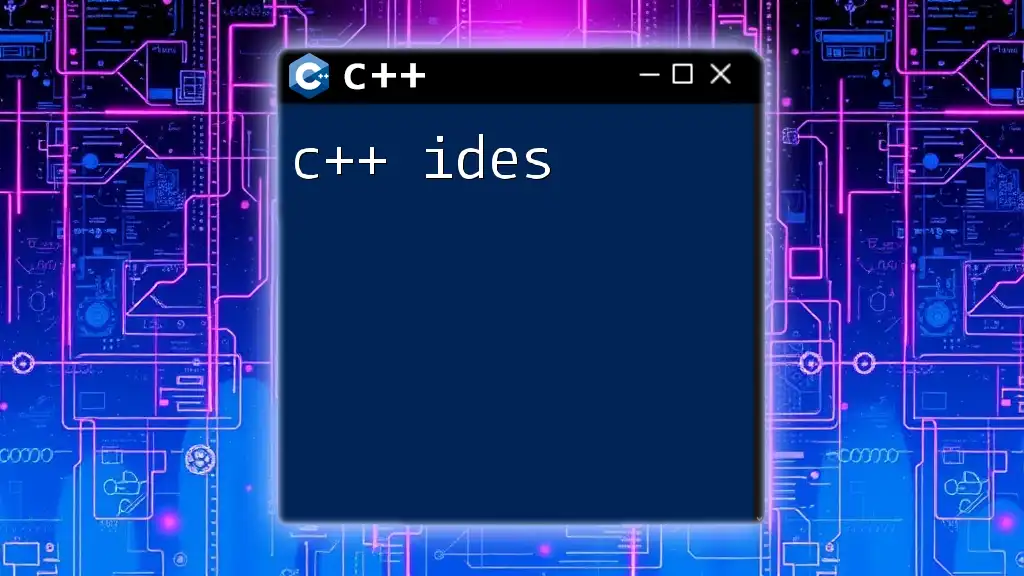
Developing a C++ PID Controller
Setting Up the PID Controller Class
To effectively manage the complexities of PID control, a structured C++ class can be established. This class should encapsulate all necessary components, including PID coefficients (Kp, Ki, Kd), previous error, and integral terms.
Code Snippet: Basic PID Class Structure
class PID {
private:
double Kp, Ki, Kd;
double prev_error, integral;
public:
PID(double Kp, double Ki, double Kd)
: Kp(Kp), Ki(Ki), Kd(Kd), prev_error(0), integral(0) {}
double compute(double setpoint, double actual, double dt);
};
Implementing the Compute Method
The core logic of the PID controller resides in the `compute` method. This function calculates the error between the desired setpoint and the actual measurement, and it subsequently applies the PID formula using the individual contributions of proportional, integral, and derivative terms.
Code Snippet: Compute Method Implementation
double PID::compute(double setpoint, double actual, double dt) {
double error = setpoint - actual;
integral += error * dt;
double derivative = (error - prev_error) / dt;
prev_error = error;
return Kp * error + Ki * integral + Kd * derivative;
}
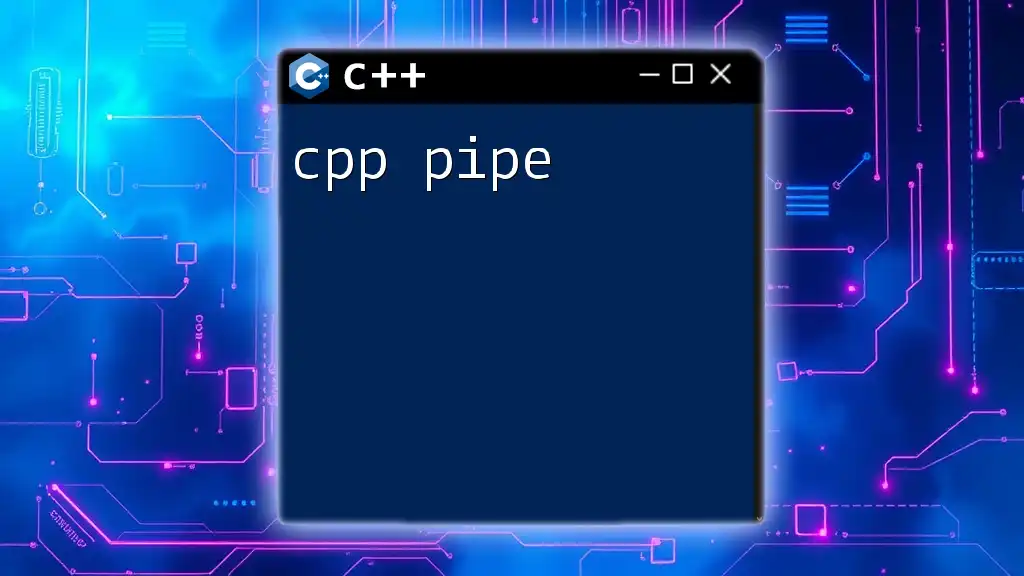
Practical Example of a PID Controller in C++
Application in Temperature Control System
To illustrate the practical application of a PID controller, consider a temperature control system. Here, the controller aims to maintain the temperature of a furnace at a desired setpoint by adjusting the power supplied to it.
The behavior of a PID controller can significantly mitigate the fluctuations that might arise from changing environmental conditions. Through continuous monitoring and adjustment, the controller ensures that the temperature approaches the designated setpoint with optimal stability.
Code Snippet: Implementing Temperature Control
PID tempPID(2.0, 5.0, 1.0); // Example PID coefficients
double currentTemp = 20.0; // Example current temperature
double setpointTemp = 25.0; // Desired temperature
// PID loop
while (true) {
double controlSignal = tempPID.compute(setpointTemp, currentTemp, dt);
// Assume applyControlSignal(controlSignal) applies the control to the system
currentTemp += ...; // Update current temperature based on control effect
}
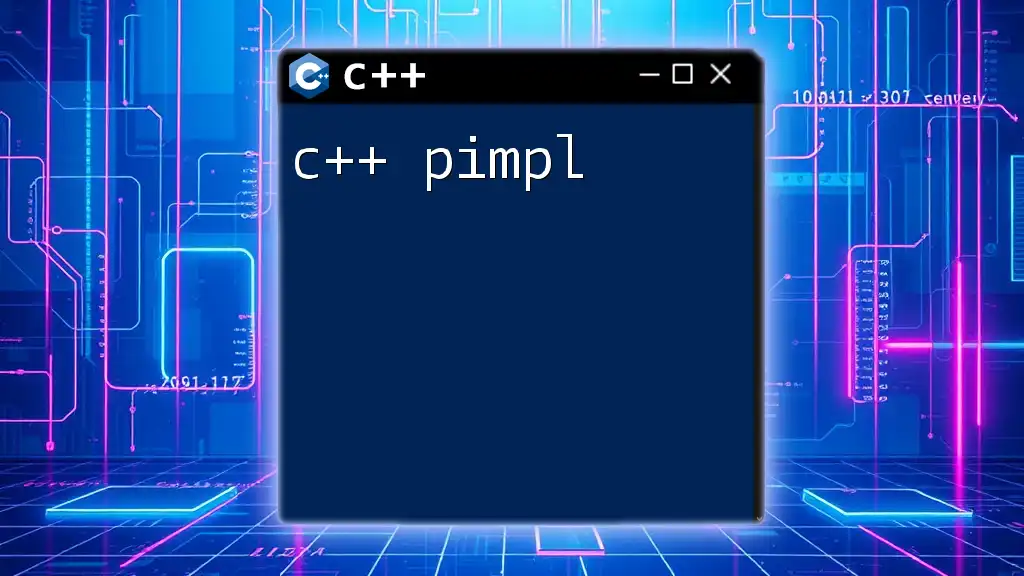
Tuning PID Controllers
Importance of Tuning
Tuning is essential for any PID controller, as improperly tuned parameters can lead to poor performance, instability, or oscillation. Proper tuning ensures that the PID controller responds optimally to changes in input and effectively minimizes error over time.
Common Tuning Methods
There are various methods for tuning PID controllers, with the most notable being:
- Ziegler-Nichols Method: This widely used method involves initially setting the integral and derivative gains to zero, then slowly increasing the proportional gain until sustained oscillation occurs. The values obtained are then used to set optimal parameters.
- Trial and Error: A more straightforward but time-consuming approach, where one tweaks the Kp, Ki, and Kd values iteratively while observing the system's response.
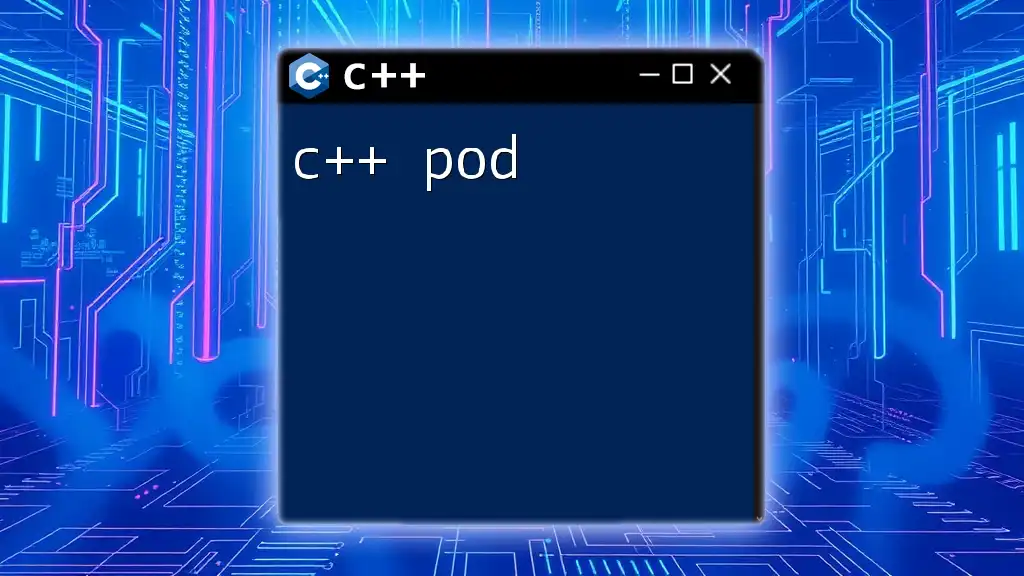
Challenges and Best Practices
Common Pitfalls in PID Implementation
Common issues in PID implementation include:
-
Integral Windup: This occurs when the integral term accumulates rapidly during prolonged error periods, potentially causing system instability. To counteract this, apply limits to the integral term or implement anti-windup strategies.
-
Oscillations and Instability: Highly aggressive tuning can lead to sustained oscillations or instability. When this occurs, it may indicate Kp values are set too high or that the integral term is causing excessive correction.
Best Practices for Implementing PID in C++
-
Use simulation tools for testing your PID controller in a controlled environment before deploying it in the real world. This step provides insight into performance without risking damage to physical systems.
-
Focus on code clarity and documentation. Writing well-organized code with comments explaining the functionality will ease future modifications and audits.
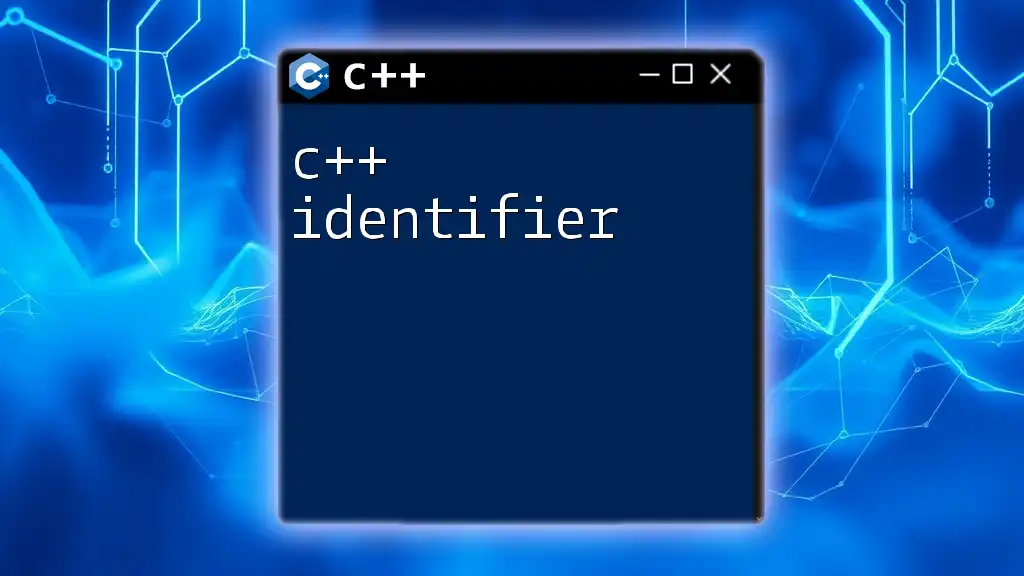
Conclusion
Summary of Key Points
In this guide, we explored the foundational aspects of the c++ pid controller, examining each component's role within the PID structure. We discussed the significance of PID in control systems, detailed how to create a PID controller class in C++, and provided practical examples. With an understanding of tuning techniques and best practices, you are well-equipped to implement PID controllers in your C++ applications.
Further Resources
For those interested in delving deeper into the subject, many resources are available, including textbooks on control theory, online courses, and forums featuring discussions on advanced PID tuning techniques and C++ programming insights.
Final Thoughts
Embrace the opportunity to experiment with PID control in real-world applications. As you gain more experience, challenge yourself with advanced topics to enhance your skills further and improve your understanding of control systems.