The `n` command in C++ is typically used as a placeholder for new-line input manipulation, especially in combination with output streams, which allows you to format your output on multiple lines.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Inserts a new line after the output
return 0;
}
Understanding the "n" Command in C++
C++ is a powerful programming language that allows you to express complex ideas simply and efficiently. One common variable used in C++ programming is "n," which typically stands for a numeric value, especially in the context of loops, arrays, and other data structures. Understanding how to effectively use "n" can significantly enhance your coding proficiency and productivity.
What Does "n" Represent in C++?
In many programming scenarios, "n" is utilized as a placeholder for numbers, particularly when dealing with size or counts. For example, it might represent the number of iterations in a loop or the size of an array. The significance of "n" is that it allows for flexibility and adaptability in your code, making it easier to handle data structures of varying sizes.
Why Use "n" in C++?
Using "n" can be particularly beneficial for several reasons:
- Efficiency: By using a variable like "n," you can define sizes and limits dynamically, which leads to more reusable code.
- Readability: It clarifies the intentions of your code, indicating that you are working with a collection of elements, sizes, or counts.
- Maintainability: If you decide to change the size of an array or the limit of a loop, modifying the variable "n" allows you to make these changes with minimal disruption to your code.
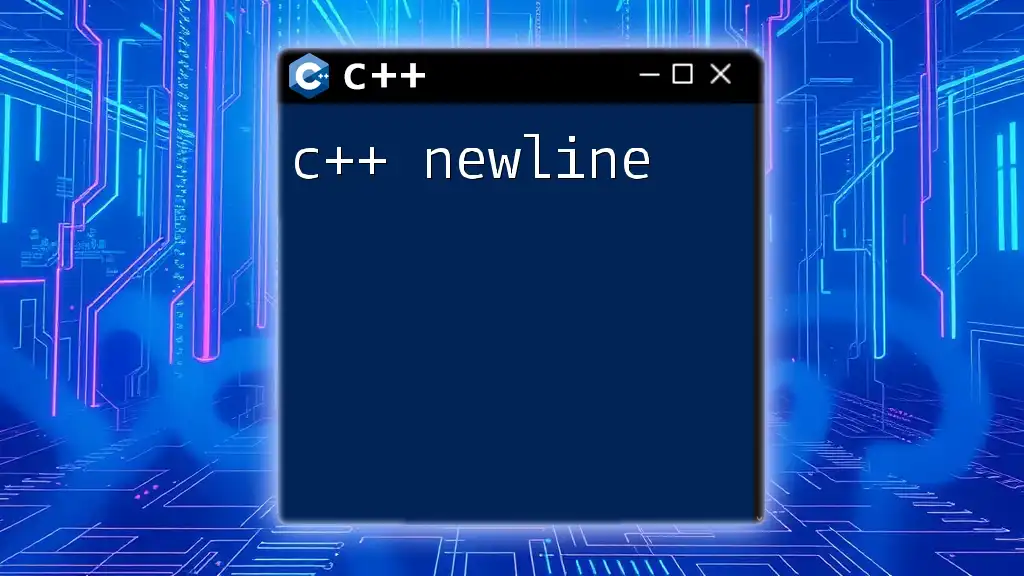
How to Use "n" in C++
Basic Usage
Using "n" in your code is straightforward. It typically involves declaring "n" and utilizing it wherever a numeric value is needed.
Here’s an example code snippet demonstrating the basic usage of "n":
#include <iostream>
int main() {
int n = 5; // Declare and initialize n
std::cout << "The value of n is: " << n << std::endl;
return 0;
}
Using "n" with Data Types
Integers and Floating Point Numbers
"n" can represent various data types, and by understanding this, you'll be able to enhance your coding practices significantly. For instance:
#include <iostream>
int main() {
int n = 10; // Integer
float nFloat = 10.5; // Floating point
std::cout << "Integer n: " << n << ", Floating point n: " << nFloat << std::endl;
return 0;
}
This code snippet shows how "n" can be used as both an integer and a floating-point number, showcasing its versatility.
Using "n" in Arrays
An important application of "n" is its use in defining the size of arrays. You can declare an array using "n" to create a dynamic-size array based on your variable.
#include <iostream>
int main() {
int n = 5;
int arr[n]; // Declare an array of size n
for (int i = 0; i < n; i++) {
arr[i] = i * 2; // Assigning values
}
std::cout << "Array values: ";
for (int i = 0; i < n; i++) {
std::cout << arr[i] << " "; // Print array values
}
return 0;
}
In this example, the size of the array is determined by the variable "n," which makes your code adaptable to changes.
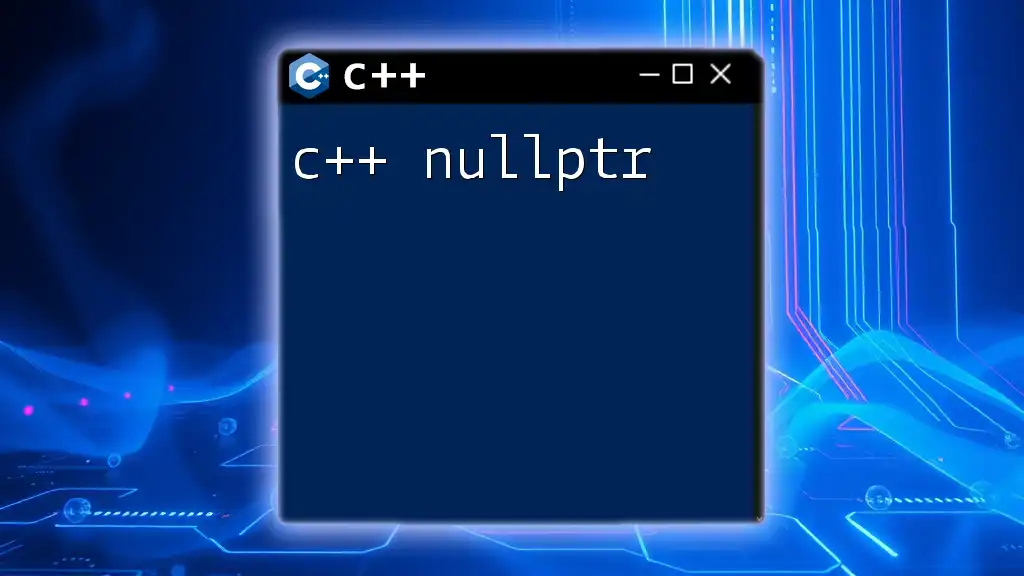
Practical Applications of "n" in C++
Looping Structures
Loops are one of the primary areas where "n" finds its utility. Being able to iterate "n" times allows for efficient and dynamic data processing. Consider the following example of using "n" in a for-loop:
#include <iostream>
int main() {
int n = 5;
std::cout << "Counting up to n: ";
for (int i = 0; i < n; i++) {
std::cout << i << " ";
}
std::cout << std::endl;
return 0;
}
This code demonstrates how you can utilize "n" to dictate the number of iterations in the loop, making it easy to adjust the counting limit by simply changing "n."
Function Parameters
"n" can also serve as a function parameter, allowing you to write more generic and reusable functions. Here’s a simple example:
#include <iostream>
void printN(int n) {
std::cout << "The value of n is " << n << std::endl;
}
int main() {
int n = 10;
printN(n); // Calling the function with n
return 0;
}
In this example, the function `printN` accepts "n" as a parameter, which allows you to pass different values whenever you call the function, enhancing its reusability.
Conditional Statements
Using "n" in conditional statements is also straightforward and effective. You can check the value of "n" to execute specific logic in your code.
#include <iostream>
int main() {
int n = 8;
if (n > 10) {
std::cout << "n is greater than 10" << std::endl;
} else {
std::cout << "n is 10 or less" << std::endl;
}
return 0;
}
This code snippet checks the value of "n" and responds accordingly, demonstrating how conditionals can utilize "n" directly.
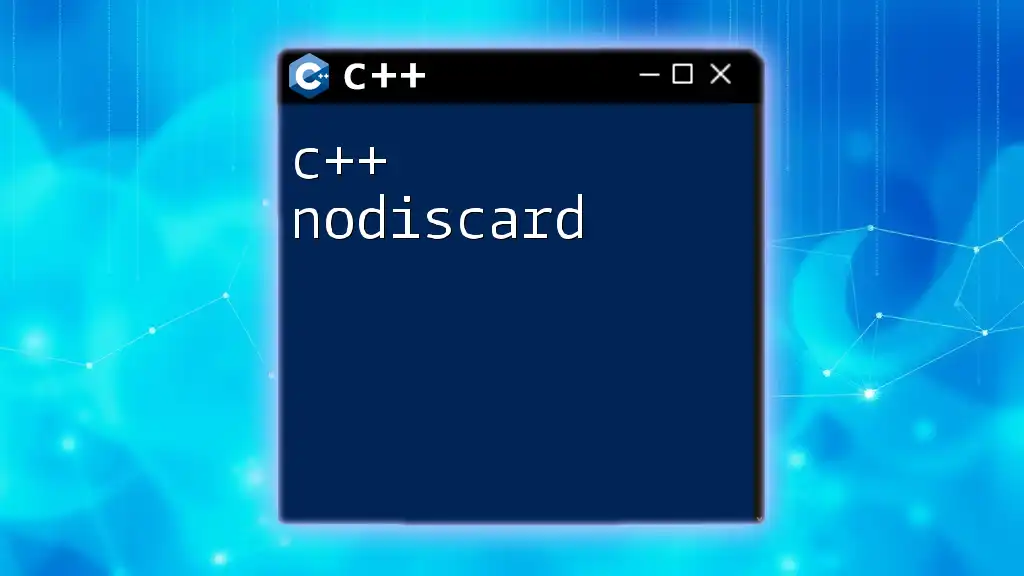
Common Mistakes When Using "n" in C++
While "n" is beneficial, there are common pitfalls to be wary of.
Uninitialized Variables
One critical mistake is using "n" without initializing it. Attempting to use an uninitialized variable can lead to unpredictable behavior in your code. For example:
#include <iostream>
int main() {
int n; // n is uninitialized
std::cout << "The value of n is: " << n << std::endl; // Undefined behavior
return 0;
}
To avoid this, always ensure that "n" is properly initialized before usage.
Out-of-Bounds Errors in Arrays
Using "n" to define the size of an array without checking its validity can result in out-of-bounds errors. Always validate "n" before using it to construct arrays to prevent such issues.
#include <iostream>
int main() {
int n = -5; // Invalid size
if (n > 0) {
int arr[n]; // Dangerous if n is not validated
} else {
std::cout << "Invalid array size" << std::endl;
}
return 0;
}
Here, it is essential to validate "n" to ensure it's a positive integer before using it as an array size.
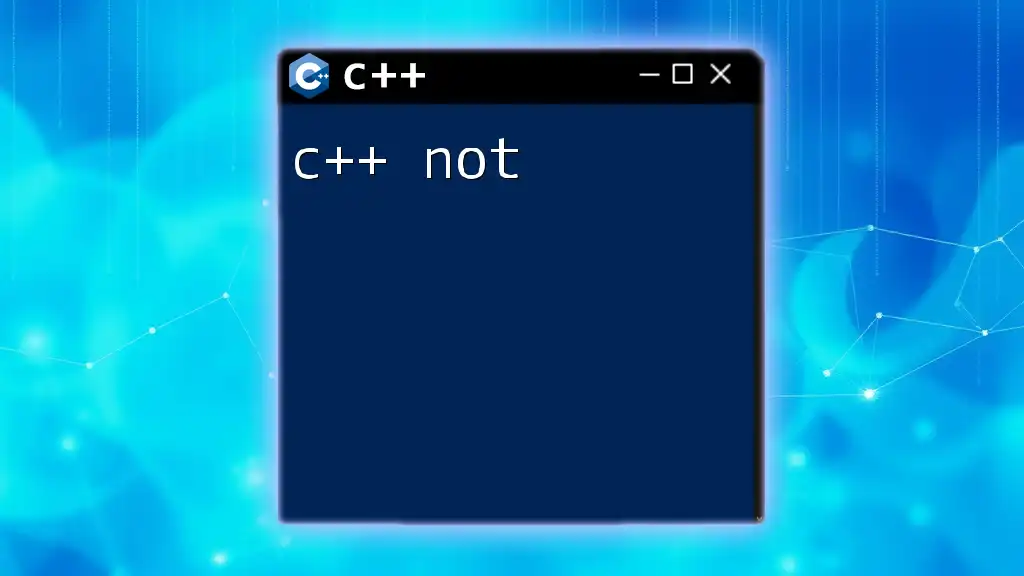
Advanced Tips for Using "n" in C++
Optimizing Performance with "n"
When working with large datasets or numerous iterations, consider optimizing how you use "n." Instead of repeatedly recalculating the value of "n," store it in a constant if it's unlikely to change, which can save processing time.
Combining "n" with STL (Standard Template Library)
Integrating "n" with the Standard Template Library (STL) can streamline your code further. For example, consider how "n" can be used to create dynamic vectors:
#include <iostream>
#include <vector>
int main() {
int n = 5;
std::vector<int> vec(n); // Create a vector of size n
for (int i = 0; i < n; i++) {
vec[i] = i * 3; // Assigning values
}
std::cout << "Vector values: ";
for (auto value : vec) {
std::cout << value << " "; // Print vector values
}
return 0;
}
In this snippet, "n" dictates the size of the vector, making operations on collections easier with STL.
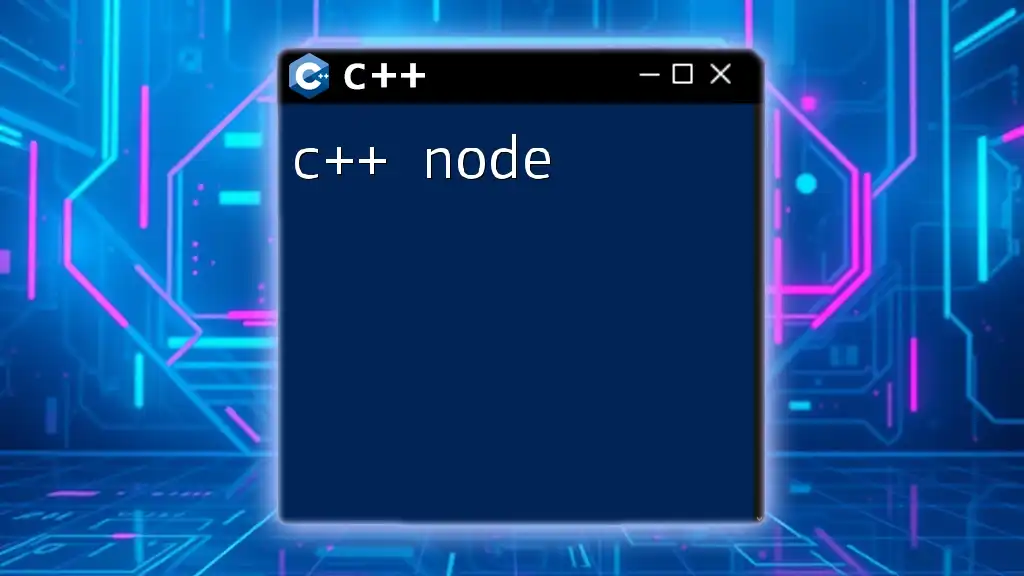
Conclusion
Mastering the usage of "n" in C++ programming is crucial for developing efficient, readable, and maintainable code. By understanding its applications—from basic data handling to advanced function parameters—you can significantly improve your coding skills and execution. Take practice and strive to incorporate these techniques in your projects. Always remember to initialize "n," validate its use in arrays, and leverage the power of STL for enhanced performance. As you use "n" more often, watch as your coding efficiency and fluency grow.