In C++, multi-line comments are enclosed between `/` and `/`, allowing you to write comments that span multiple lines without affecting the execution of the code.
Here's a code snippet demonstrating a multi-line comment:
/*
This is a multi-line comment in C++.
It can span several lines.
*/
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Multi Line Comments in C++
What are Multi Line Comments?
Multi line comments in C++ are essential tools used by programmers to explain, document, or clarify sections of their code. Unlike single line comments that serve a quick purpose, multi line comments allow for in-depth explanations and documenting complex code that may span across several lines.
Syntax of a Multi Line Comment
The syntax for a multi line comment begins with `/` and ends with `/`. Everything in between these delimiters is ignored by the compiler. This feature makes multi line comments particularly useful for adding descriptive notes directly within the code without affecting its functionality.
Example Code Snippet
/* This is a multi line comment
It can span multiple lines
Useful for providing detailed information */
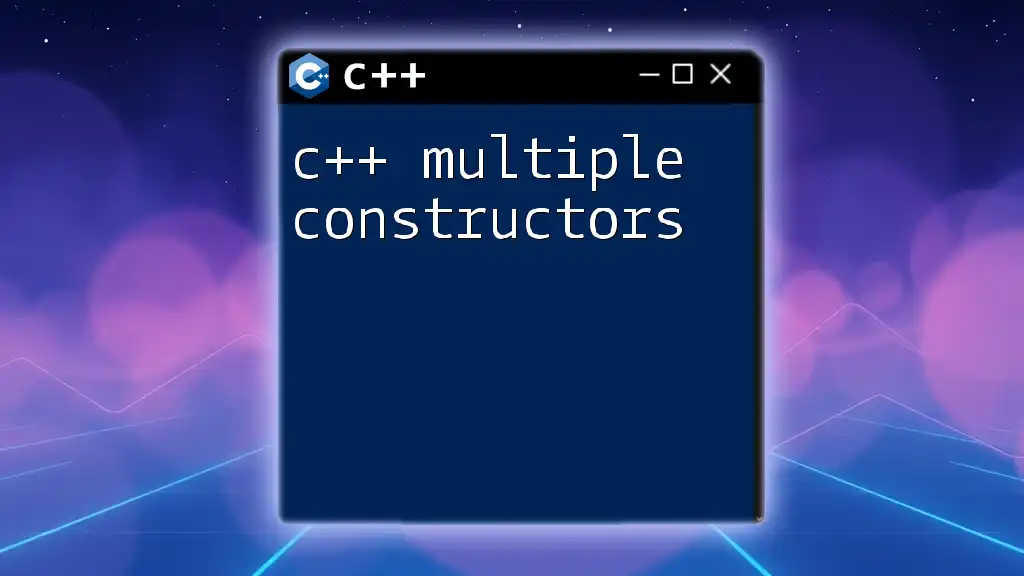
Practical Uses of Multi Line Comments
Documenting Complex Logic
One of the primary applications of multi line comments is to document complex functions or algorithms. Providing a clear explanation before the function can significantly enhance code readability. This is particularly important for future developers who may work on the code or even for the original author returning to it after time has passed.
Example Code Snippet
/* This function calculates the factorial of a number recursively.
It takes an integer input and returns the factorial as an integer.
*/
int factorial(int n) {
if (n <= 1)
return 1;
return n * factorial(n - 1);
}
In this example, the multi line comment succinctly explains what the `factorial` function does, what type of input it requires, and what it returns. This prevents confusion and saves time when revisiting the code.
Temporarily Disabling Code
Another common misuse of multi line comments is for debugging purposes. Developers often use multi line comments to disable portions of code temporarily while testing new features or troubleshooting. This allows them to isolate problematic code without permanently removing it.
Example Code Snippet
/*
int x = 10;
int y = 20;
cout << x + y; // This line is disabled
*/
In this scenario, the code block is commented out, meaning it won't execute, while still remaining easily accessible for future reference.
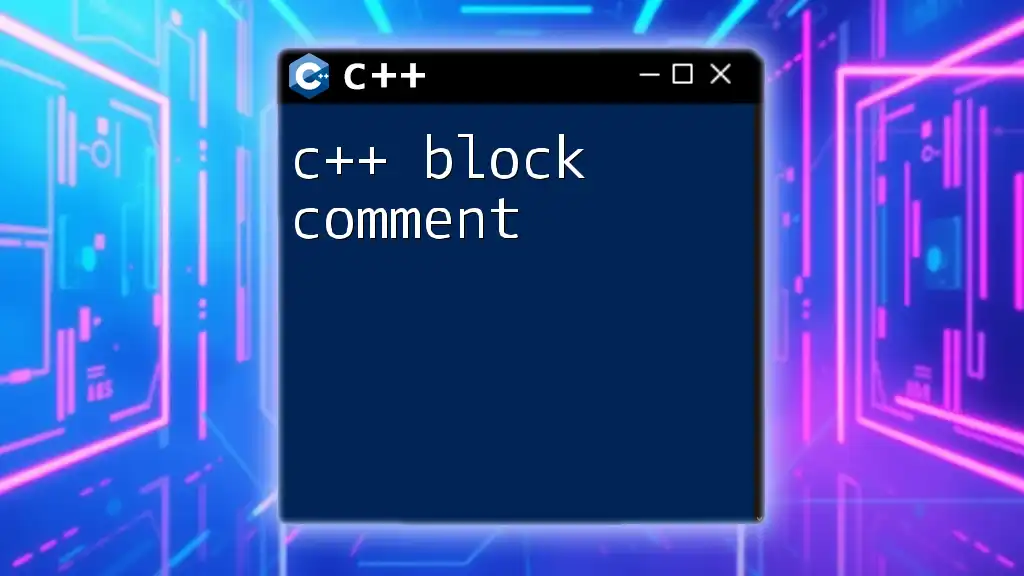
Best Practices for Using Multi Line Comments
Keep Comments Relevant
When using multi line comments, it is essential to ensure that they are relevant to the code they describe. This relevance aids in maintaining clarity and understanding. Avoid adding excessive information that may confuse rather than elucidate.
Avoid Over-Commenting
While comments are valuable, overuse can clutter the code, making it hard to read. Striking the right balance is crucial. Comments should enhance understanding, not detract from it. Focus on commenting complex logic rather than every single line of code.
Maintain Comment Consistency
A consistent commenting style throughout the codebase significantly improves readability. Try to adhere to established conventions within your team or project, including the formatting and phrasing of comments, to create a uniform coding environment.
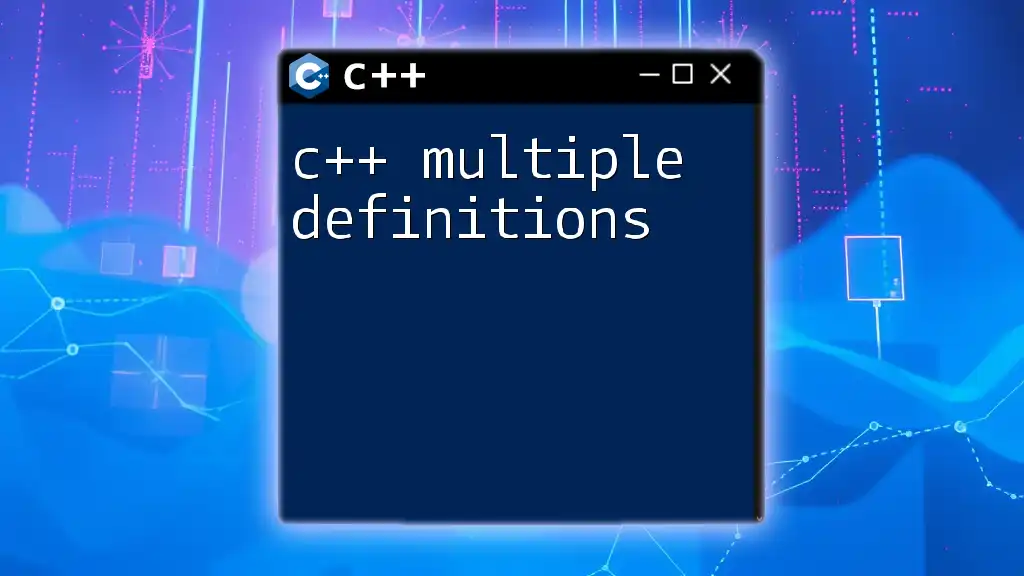
Differences Between Multi Line Comments and Single Line Comments
Single Line Comment Syntax
Single line comments in C++ are designated by `//`. They are typically used to write brief explanations or notes directly inline with the code. This makes them suitable for quick clarifications.
When to Use Each Type
Deciding when to use multi line versus single line comments often comes down to the complexity of the information. Use multi line comments for detailed descriptions and when explaining complex constructs. Reserve single line comments for short notes or quick reminders within the code.
Example Comparison
// This is a single line comment
int a = 5; // Initialize a
/* This is a multi line comment
it can provide more detailed descriptions */
int b = 10;
The examples showcase how in-line commentary can be quick and succinct, while multi line comments offer room for elaborate explanations.
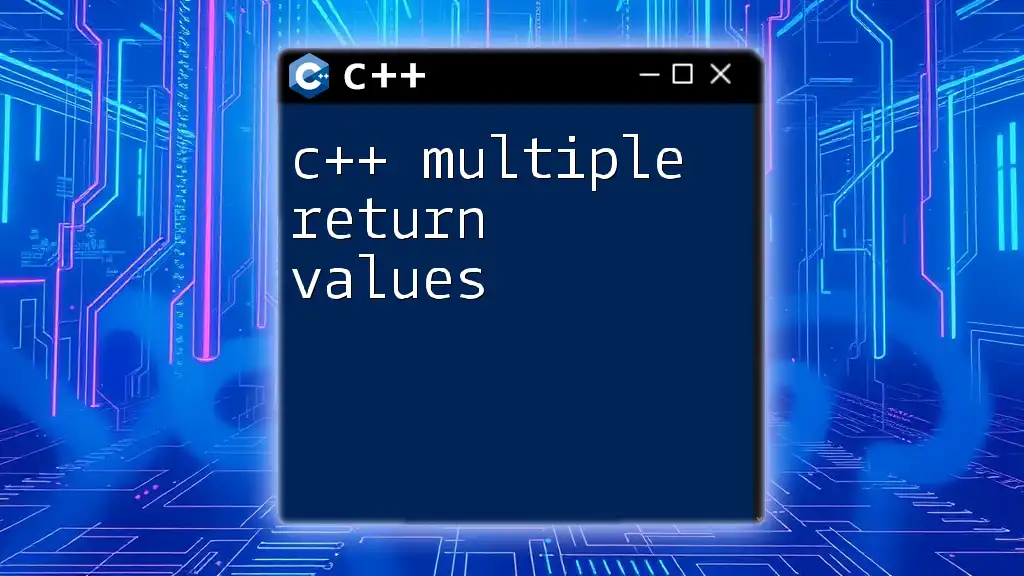
Common Mistakes When Using Multi Line Comments
Unclosed Comment Blocks
A frequent mistake among developers is neglecting to close a multi line comment properly. This can lead to compilation errors or unintended behavior, as the compiler will interpret everything following the opening delimiter as part of the comment.
Nested Comments
It’s also essential to be aware that C++ does not support nested multi line comments. Writing a multi line comment within another will create confusion and potentially lead to errors.
Code Example of Mistake
/* This function outputs hello world
cout << "Hello, World!"; // This may cause a compilation error
In the above example, failing to close the multi line comment correctly leads to issues during compilation and prevents the code from running correctly.
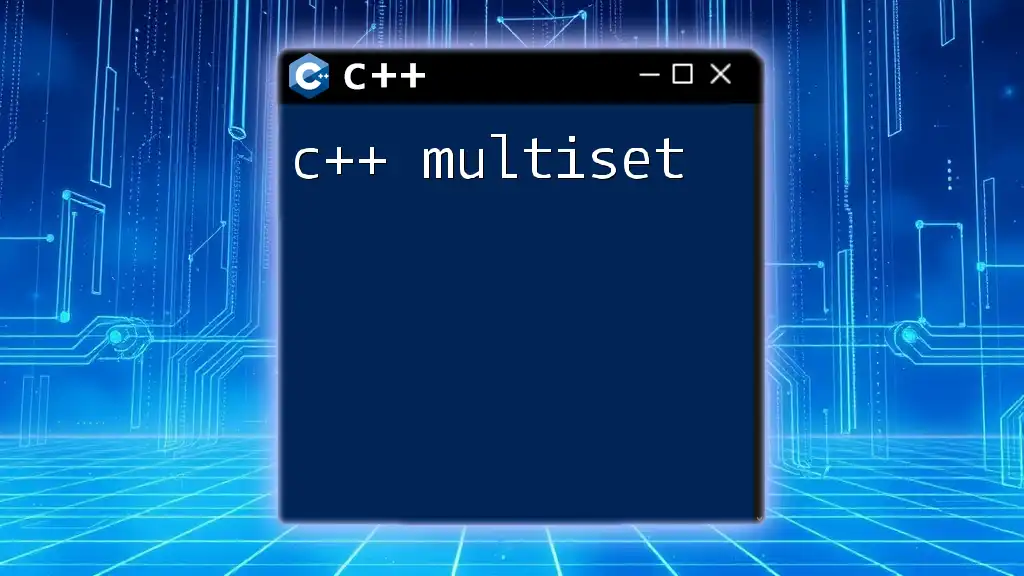
Conclusion
C++ multi line comments are a powerful feature that enhances code understanding and maintenance. By enabling developers to document logic and provide explanations, multi line comments become invaluable in writing clear, readable code. Adhering to best practices ensures that comments remain beneficial rather than distracting.
Effective commenting can significantly impact a project's long-term success by saving time, reducing errors, and improving collaboration among developers. Always strive to use comments wisely and in a manner that promotes clarity and comprehension.