Breadth-First Search (BFS) in C++ is an algorithm used to traverse or search through graph and tree data structures level by level, and can be implemented using a queue to keep track of nodes to visit.
Here’s a simple C++ code snippet demonstrating BFS using an adjacency list representation:
#include <iostream>
#include <vector>
#include <queue>
void bfs(const std::vector<std::vector<int>>& graph, int start) {
std::queue<int> q;
std::vector<bool> visited(graph.size(), false);
q.push(start);
visited[start] = true;
while (!q.empty()) {
int node = q.front();
q.pop();
std::cout << node << " ";
for (int neighbor : graph[node]) {
if (!visited[neighbor]) {
visited[neighbor] = true;
q.push(neighbor);
}
}
}
}
int main() {
std::vector<std::vector<int>> graph = {
{1, 2}, // Neighbors of node 0
{0, 3}, // Neighbors of node 1
{0, 4}, // Neighbors of node 2
{1}, // Neighbors of node 3
{2} // Neighbors of node 4
};
std::cout << "BFS starting from node 0:" << std::endl;
bfs(graph, 0);
return 0;
}
Understanding the Fundamentals of BFS in C++
What is a Graph?
A graph is a data structure consisting of a set of vertices (or nodes) and a set of edges connecting pairs of vertices. Graphs can be used to represent various systems forming a connected structure, such as social networks, transportation systems, and network topologies.
Graphs can be categorized into two main types:
- Directed Graphs: In these graphs, edges have a direction, meaning they point from one vertex to another.
- Undirected Graphs: Edges in these graphs do not have a direction; the connection between vertices is bidirectional.
In C++, graphs can be represented using different structures, such as adjacency lists or adjacency matrices. An adjacency list is particularly useful for efficiently storing sparse graphs.
BFS Algorithm Explained
The Breadth First Search (BFS) algorithm traverses a graph or tree level by level, exploring all neighbor nodes at the present depth before moving on to nodes at the next level. This makes BFS ideal for scenarios where exploring the nearest nodes first is desirable.
Key features of BFS include:
- It uses a queue data structure to facilitate the traversal.
- BFS is particularly useful for finding the shortest path in unweighted graphs.
BFS is often contrasted with Depth First Search (DFS), which explores as far as possible down one branch before backtracking. While BFS gives you the shortest path in unweighted graphs, DFS is typically more memory efficient for large graphs.
Applications of BFS in Real-Life Problems
BFS is applicable in numerous real-world scenarios, including:
- Shortest path finding: BFS can be employed to determine the shortest path between two nodes.
- Network broadcasting: In networking, BFS can effectively broadcast messages to all nodes.
- Finding connected components: BFS helps in identifying different connected components in a graph.
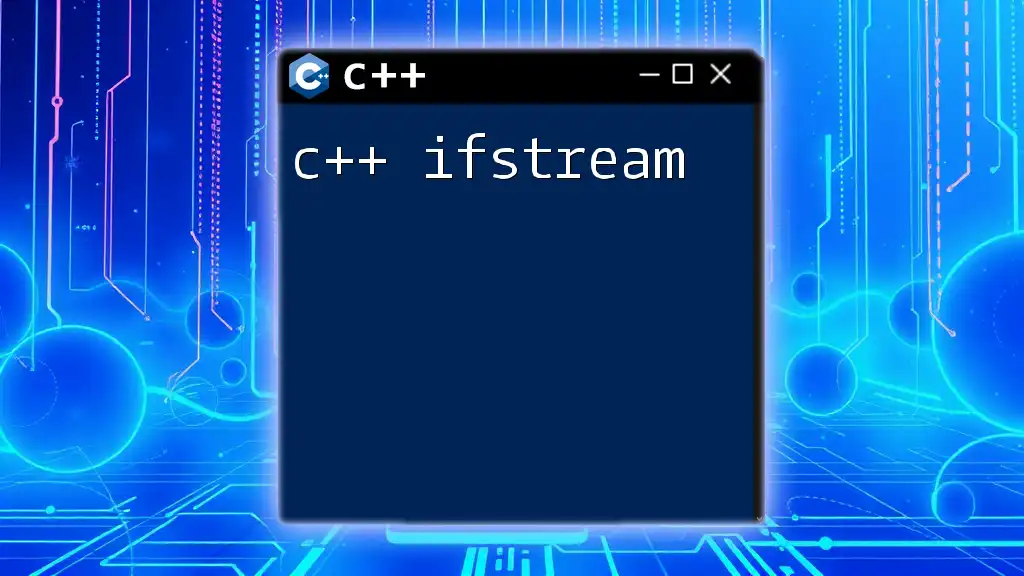
Implementing BFS in C++
Setting Up the C++ Environment
Before you can start implementing BFS in C++, make sure you have your development environment set up. You will need a C++ compiler and standard libraries for input-output and data structures like vectors and queues.
BFS Algorithm Implementation in C++
Building the Graph
To implement BFS, you first need to define a graph structure. Below is a simple representation of a graph using an adjacency list through a vector of vectors.
#include <iostream>
#include <vector>
#include <queue>
using namespace std;
class Graph {
public:
int vertices;
vector<vector<int>> adjList;
Graph(int v) {
vertices = v;
adjList.resize(v);
}
void addEdge(int v1, int v2) {
adjList[v1].push_back(v2);
adjList[v2].push_back(v1); // Comment this line for directed graphs
}
};
In this code snippet, we define a `Graph` class that initializes a graph with a specified number of vertices and provides a method to add edges.
Performing BFS
The BFS algorithm can be implemented using a queue to keep track of the vertices to be explored. The algorithm marks nodes as visited and processes each node by exploring its neighbors.
Here's the implementation of the BFS function:
void bfs(Graph &g, int startVertex) {
vector<bool> visited(g.vertices, false);
queue<int> q;
visited[startVertex] = true;
q.push(startVertex);
while (!q.empty()) {
int vertex = q.front();
q.pop();
cout << vertex << " ";
for (int neighbor : g.adjList[vertex]) {
if (!visited[neighbor]) {
visited[neighbor] = true;
q.push(neighbor);
}
}
}
}
In this implementation, we initiate a `visited` vector to keep track of visited nodes. The queue helps us process each vertex while exploring its neighboring nodes.
Example: Using BFS to Traverse a Graph
To see BFS in action, let's create a sample graph and call the BFS function from the main function.
int main() {
Graph g(5); // Total of 5 vertices
g.addEdge(0, 1);
g.addEdge(0, 2);
g.addEdge(1, 3);
g.addEdge(1, 4);
g.addEdge(2, 4);
cout << "BFS starting from vertex 0: ";
bfs(g, 0); // Expected Output: 0 1 2 3 4
return 0;
}
In this code, we create a graph with five vertices and several edges. When calling the BFS function starting from vertex 0, the expected output is `0 1 2 3 4`, demonstrating how BFS traverses the graph level by level.
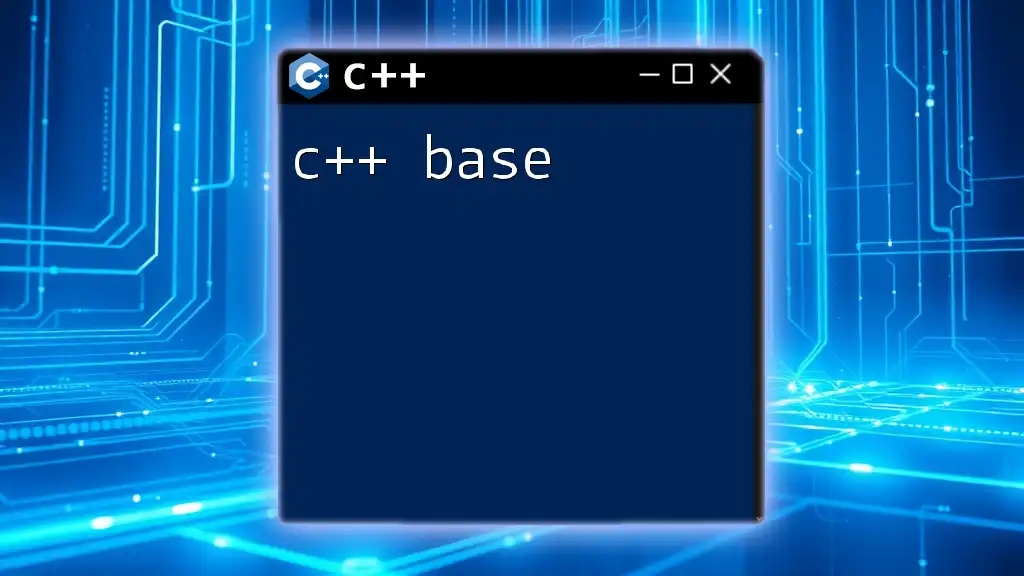
Advanced Topics in BFS
BFS for Shortest Path
BFS can be adapted to solve the shortest path problem in unweighted graphs. To modify BFS to return the shortest path, we can maintain a distance array that keeps track of the distance of each vertex from the starting point.
BFS in Trees vs. Graphs
While BFS can be applied to both trees and graphs, the implementation can vary slightly. In the case of binary trees, BFS can leverage the property of child nodes, using each level for traversal.
Below is an example code snippet for BFS on a binary tree:
class TreeNode {
public:
int value;
TreeNode* left;
TreeNode* right;
TreeNode(int val) : value(val), left(nullptr), right(nullptr) {}
};
void bfsTree(TreeNode* root) {
if (!root) return;
queue<TreeNode*> q;
q.push(root);
while (!q.empty()) {
TreeNode* current = q.front();
q.pop();
cout << current->value << " ";
if (current->left) q.push(current->left);
if (current->right) q.push(current->right);
}
}
In this tree traversal using BFS, `bfsTree` function operates similarly to the graph implementation, visiting each node level by level.
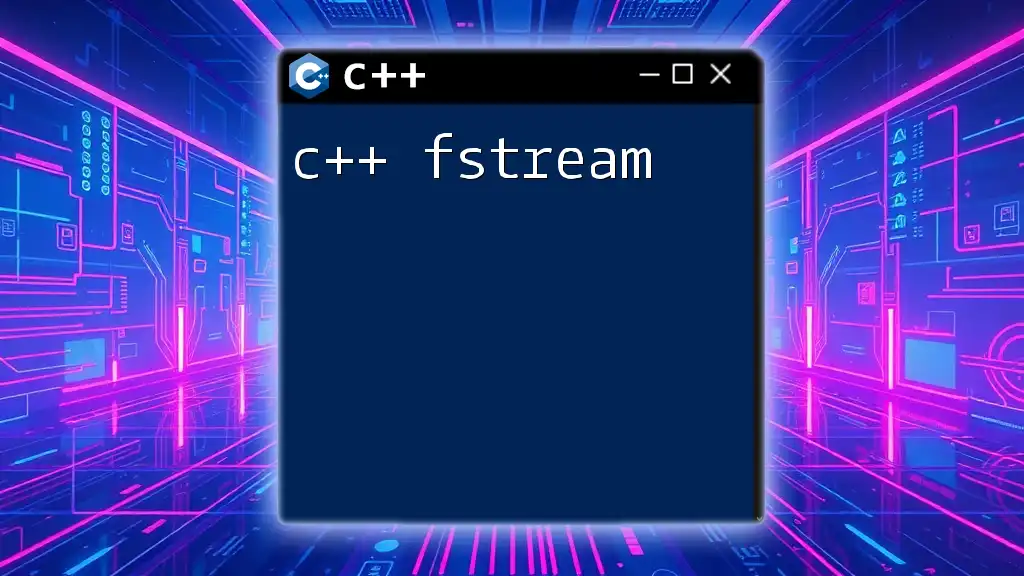
Common Pitfalls and Best Practices
While implementing BFS, keep the following considerations in mind:
- Performance: BFS has a time complexity of O(V + E), where V is the number of vertices and E is the number of edges. Ensure that your graph structures are optimized to support this complexity.
- Avoiding Infinite Loops: Make sure your visited array prevents revisiting nodes, which can lead to infinite loops.
- Cycle Detection: Incorporate checks to detect cycles in graphs if you're working with cyclic structures.
Overall, implementing C++ BFS not only enhances your understanding of graph algorithms but also establishes a strong foundational skill for tackling various computational problems in programming.
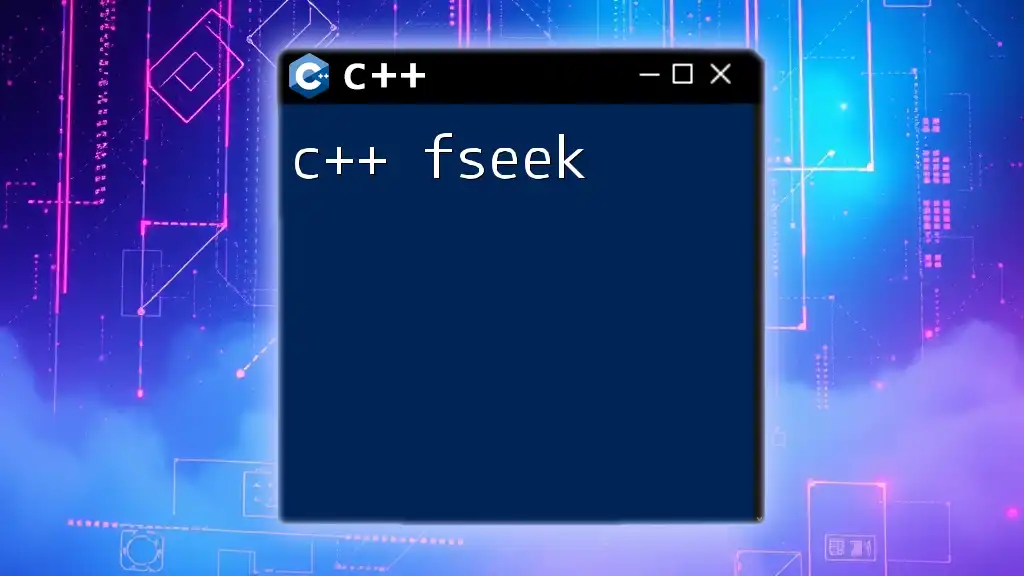
Conclusion
The C++ BFS algorithm is a powerful tool for navigating through graphs or trees, offering efficient traversal, shortest path identification, and various applications in real-world scenarios. The knowledge gained from understanding and implementing BFS can significantly improve your programming efficacy and problem-solving skills. As you explore more complex graph structures, practice implementing BFS across different challenges to further solidify your understanding.
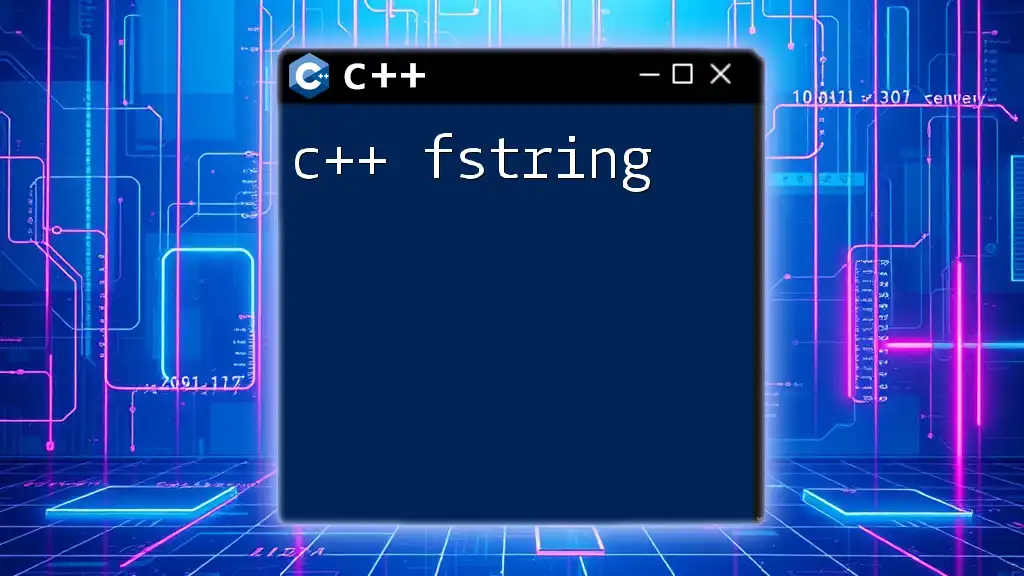
Frequently Asked Questions
What is the Time Complexity of BFS?
The time complexity of BFS is O(V + E), where V represents the number of vertices and E represents the number of edges in the graph.
Can BFS Work on Weighted Graphs?
BFS is primarily designed for unweighted graphs. For weighted graphs, algorithms like Dijkstra's or Bellman-Ford are more appropriate.
How to Optimize BFS?
You can optimize BFS by ensuring the use of appropriate data structures, managing memory efficiently, and avoiding unnecessary computations, especially in large graphs.