In C++, a 64-bit integer can be represented using the `long long` data type, which allows for storing large integer values ranging from −9,223,372,036,854,775,808 to 9,223,372,036,854,775,807.
#include <iostream>
int main() {
long long largeNumber = 9223372036854775807; // Maximum value for a 64-bit integer
std::cout << "The large number is: " << largeNumber << std::endl;
return 0;
}
What is a 64 Bit Integer?
A 64-bit integer, often referred to as `long long int` or `int64_t` in C++, is a data type that allows you to represent a wide range of integer values. Specifically, it can store values from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807. This range is significantly larger than that of a typical 32-bit integer, where the range is from -2,147,483,648 to 2,147,483,647.
Understanding when and why to use a 64-bit integer is crucial in programming. Applications such as scientific computations, financial modeling, and data analysis often require handling large numbers, making the 64-bit integer indispensable.
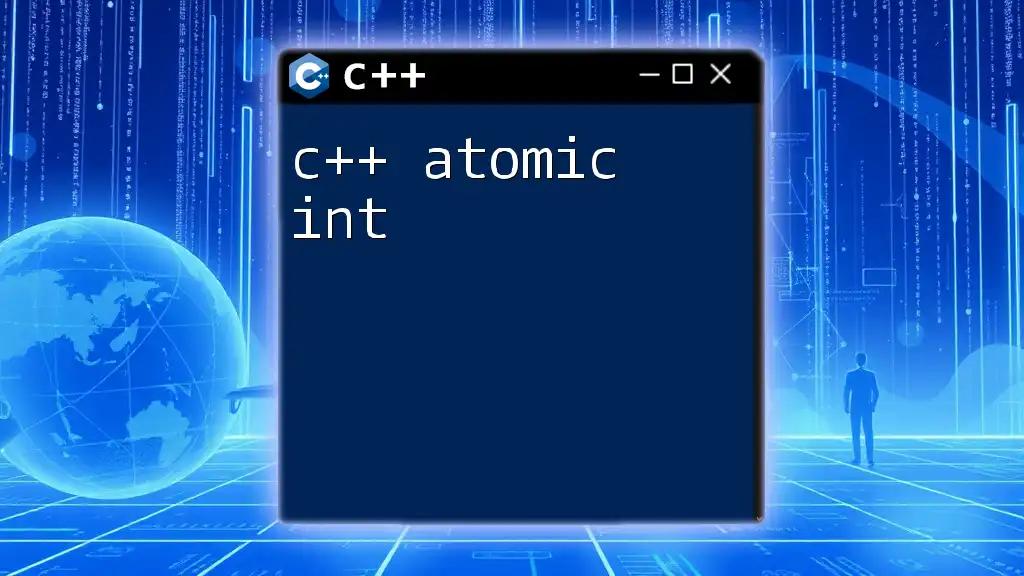
Understanding Data Types in C++
Before diving deeper into C++ 64 bit int, it's essential to have a foundational understanding of C++ data types. C++ provides a variety of data types, including char, int, float, and double, each serving different purposes.
The Need for Larger Integers
As systems evolve and datasets grow, the limitations of 32-bit integers can become problematic. For instance, if you're counting items in a large database or performing extensive mathematical calculations, you may encounter overflow errors with 32-bit integers because they lack the range required for such operations. This is where utilizing 64-bit integers becomes advantageous, as they reduce the risk of overflow significantly.

Declaring a 64 Bit Integer in C++
To use a 64 bit int in C++, you can declare it using different types:
Using Standard Data Types
In C++, you may declare a 64 bit integer using the `long long int` data type. Here’s a simple code snippet to illustrate this:
long long int largeNumber = 9223372036854775807; // Maximum value for 64-bit integer
This declaration allows the variable `largeNumber` to store very large integer values, making it ideal for applications needing a wide range of values.
Using Fixed Width Integer Types
Another effective way to declare a 64 bit integer is by using the `<cstdint>` library in C++, which standardizes integer sizes. Here’s how you do it:
#include <cstdint>
int64_t bigInteger = 1234567890123456789; // Using int64_t for a 64-bit integer
This method is preferred in multi-platform projects because it ensures a consistent size for your integer across different compilers and architectures.

Operations with 64 Bit Integers
Having established how to declare a 64 bit int, let's explore operational capabilities.
Arithmetic Operations
Basic arithmetic operations can be performed using 64-bit integers just like any other data type. This includes addition, subtraction, multiplication, and division.
Here’s a code snippet demonstrating these operations:
int64_t a = 8000;
int64_t b = 7000;
int64_t sum = a + b; // Example of addition
int64_t difference = a - b; // Subtraction
int64_t product = a * b; // Multiplication
int64_t quotient = a / b; // Division
Comparisons and Logical Operations
You can also perform comparison operations on 64-bit integers. This allows developers to write conditional statements based on the values stored in these variables.
For example:
if (a > b) {
std::cout << "a is greater than b";
}
This code checks if `a` is greater than `b`, making use of relational operators.
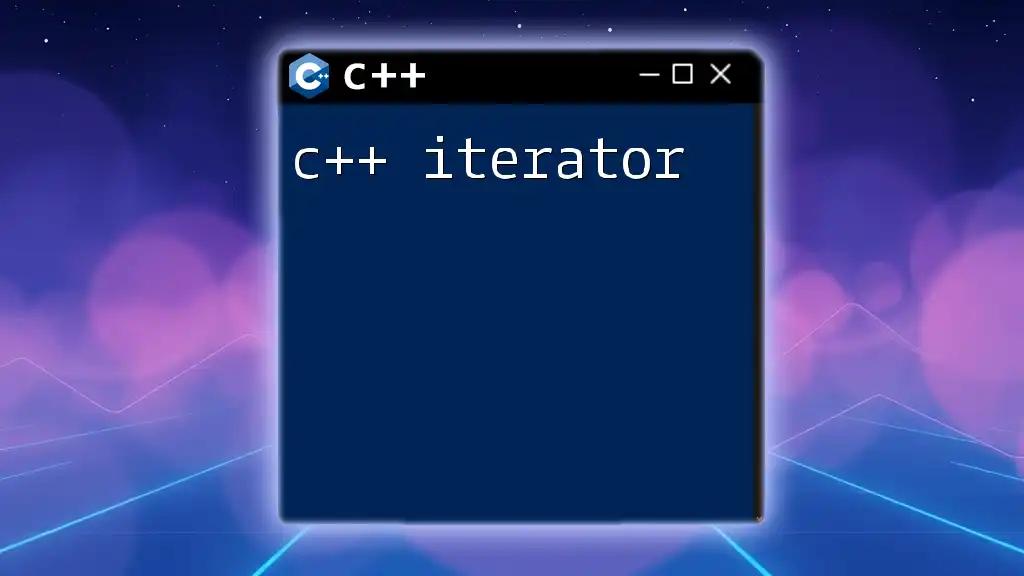
Common Pitfalls When Using 64 Bit Integers
Overflow Issues
Although 64-bit integers have a larger capacity to store values, they are not immune to overflow. An overflow occurs when an operation attempts to exceed the maximum value that can be represented by the 64-bit integer. Here’s an example:
int64_t overflowExample = 9223372036854775807 + 1; // This will cause overflow
In this scenario, adding 1 causes an overflow, resulting in unexpected outcomes. It is vital to handle such situations proactively.
Performance Considerations
While 64-bit integers offer extensive value ranges, they come with a potential performance trade-off. Using larger data types may incur a slight decrease in processing speed, particularly in systems where memory constraints exist. Therefore, understanding the specific requirements of your application is crucial in deciding whether to utilize 64-bit integers consistently.
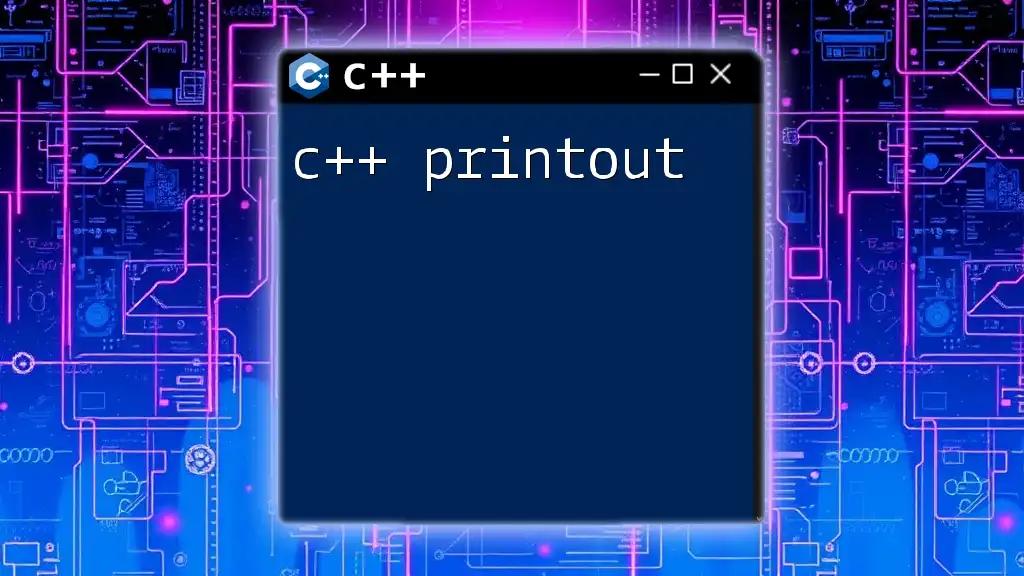
Best Practices for Using 64 Bit Integers in C++
Consistency and Readability
Maintain consistency in data type usage across your codebase to improve readability. For instance, if some calculations require 64 bit int, make it a point to use it throughout your program, unless smaller integers suffices for specific instances.
When to Use Fixed Width Types vs. Standard Types
Choosing between `int64_t` and `long long int` can depend on the context of your application. In cases where you need guaranteed fixed sizes across various platforms, `int64_t` is the preferred option. However, for general purposes and ease of use, `long long int` is sufficiently robust in most scenarios.

Use Cases for 64 Bit Integers
Handling Large Numbers in Applications
There are numerous use cases for C++ 64 bit int in real-world applications. For example, in financial applications, you might deal with large monetary values that exceed the limits of 32-bit integers. Similarly, in scientific computations involving extensive datasets, 64-bit integers ensure you can manage large values without running into overflow issues.
Performance Optimization Tips
To make the best use of 64-bit integers, consider optimizing your code to minimize unnecessary type conversions and limit the use of large integers when smaller types will suffice. Always analyze the specific needs of your application to ensure you balance performance with the need to handle larger datasets.
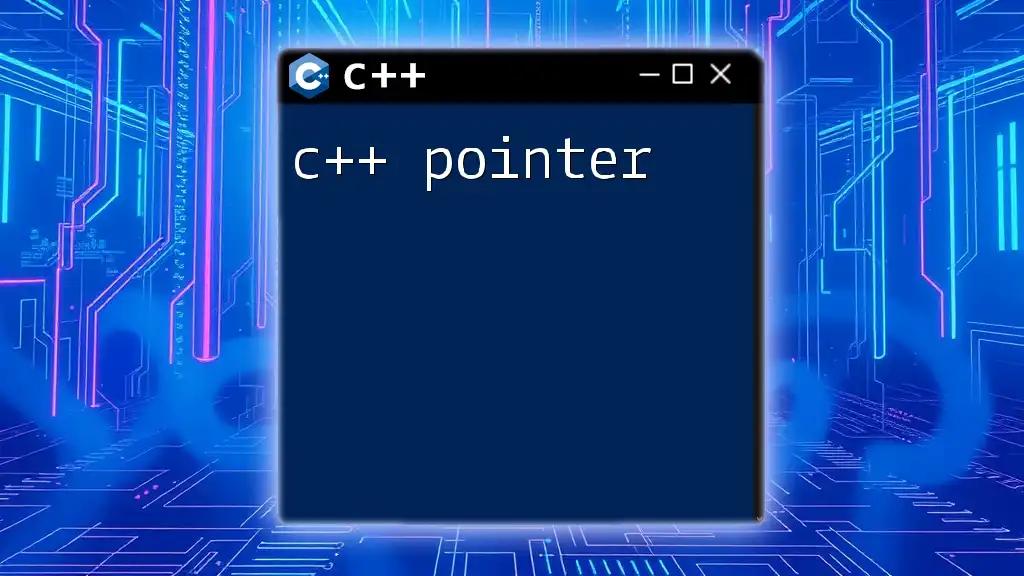
Conclusion
In summary, a C++ 64 bit int is a powerful data type that offers a solution for storing large integer values in your applications. By understanding its usage, operations, and best practices, you can effectively leverage 64-bit integers to enhance your programming projects.
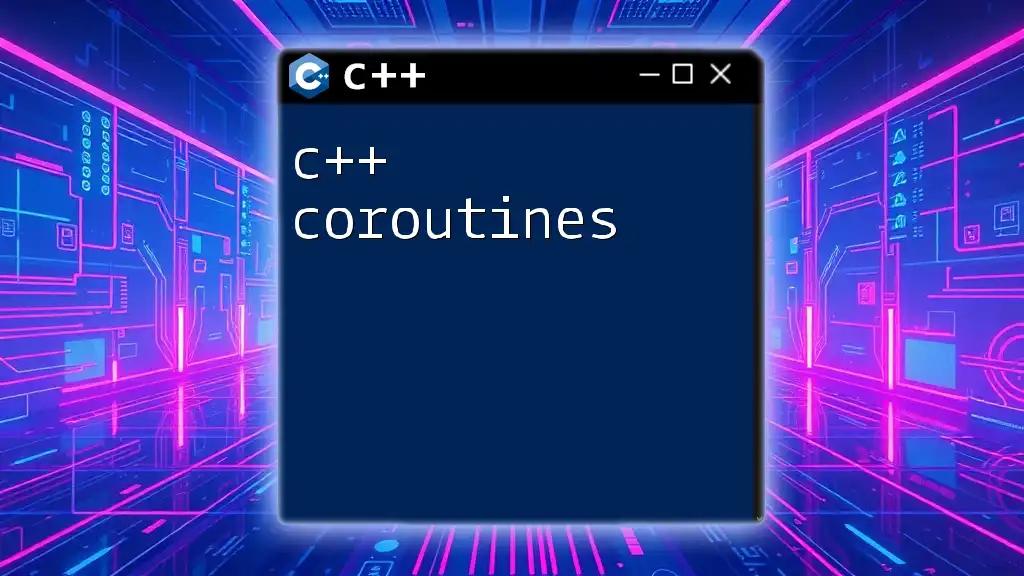
Frequently Asked Questions
What is the maximum value of a 64-bit integer in C++?
The maximum value of a 64-bit integer (specifically, `long long int` or `int64_t`) is 9,223,372,036,854,775,807.
How does C++ handle negative values in 64-bit integers?
C++ handles negative values in 64-bit integers using two's complement representation, where the most significant bit indicates the sign of the integer.
Should I always use 64-bit integers for calculations?
Not necessarily. You should use 64-bit integers only when the values you intend to handle exceed the range of 32-bit integers. For smaller numbers, using a standard int or long may be more efficient.
Can I mix 64-bit and 32-bit integers in calculations?
While you can mix them, it is advisable to use the same type in your calculations to avoid unintentional behavior, such as data loss or incorrect calculations due to type conversion issues.