Conway's Game of Life is a cellular automaton devised by mathematician John Conway, where each cell in a grid can be alive or dead based on simple rules that determine the state of the next generation.
Here's a simple C++ implementation of Conway's Game of Life:
#include <iostream>
#include <vector>
#include <unistd.h>
const int WIDTH = 10;
const int HEIGHT = 10;
void printGrid(const std::vector<std::vector<int>>& grid) {
for (const auto& row : grid) {
for (int cell : row) {
std::cout << (cell ? 'O' : '.') << " ";
}
std::cout << std::endl;
}
}
int countNeighbors(const std::vector<std::vector<int>>& grid, int x, int y) {
int count = 0;
for (int i = -1; i <= 1; ++i) {
for (int j = -1; j <= 1; ++j) {
if ((i == 0 && j == 0) || x + i < 0 || x + i >= HEIGHT || y + j < 0 || y + j >= WIDTH)
continue;
count += grid[x + i][y + j];
}
}
return count;
}
void updateGrid(std::vector<std::vector<int>>& grid) {
std::vector<std::vector<int>> newGrid = grid;
for (int i = 0; i < HEIGHT; ++i) {
for (int j = 0; j < WIDTH; ++j) {
int aliveNeighbors = countNeighbors(grid, i, j);
if (grid[i][j] == 1) {
newGrid[i][j] = (aliveNeighbors < 2 || aliveNeighbors > 3) ? 0 : 1;
} else {
newGrid[i][j] = (aliveNeighbors == 3) ? 1 : 0;
}
}
}
grid = newGrid;
}
int main() {
std::vector<std::vector<int>> grid(HEIGHT, std::vector<int>(WIDTH, 0));
grid[1][2] = grid[2][3] = grid[3][1] = grid[3][2] = grid[3][3] = 1; // initial state
while (true) {
printGrid(grid);
updateGrid(grid);
usleep(500000); // pause for half a second
}
return 0;
}
Understanding the Basics of the Game
The Rules of the Game
Conway's Game of Life is a fascinating simulation of how cells evolve over time based on predetermined rules. The game involves a grid of cells, where each cell can either be alive or dead. The transition from one generation to the next is governed by a set of simple rules:
- Any live cell with fewer than two live neighbors dies: This is due to underpopulation.
- Any live cell with two or three live neighbors lives on to the next generation: This indicates a stable environment.
- Any live cell with more than three live neighbors dies: This represents overpopulation.
- Any dead cell with exactly three live neighbors becomes a live cell: This means reproduction.
Understanding these rules is crucial for implementing the logic in your C++ program, as they create engaging and often unpredictable patterns.
Overview of Cellular Automata
Cellular automata are discrete, abstract computational systems that can model a wide variety of phenomena. Conway's Game of Life is a prime example of this concept. By understanding cellular automata, you can appreciate how simple rules can lead to complex behaviors.
In the Game of Life, each cell's state changes based on its neighbors, which can lead to surprising and sometimes beautiful patterns. This concept is important for both theoretical and practical applications, from biology to computer graphics.
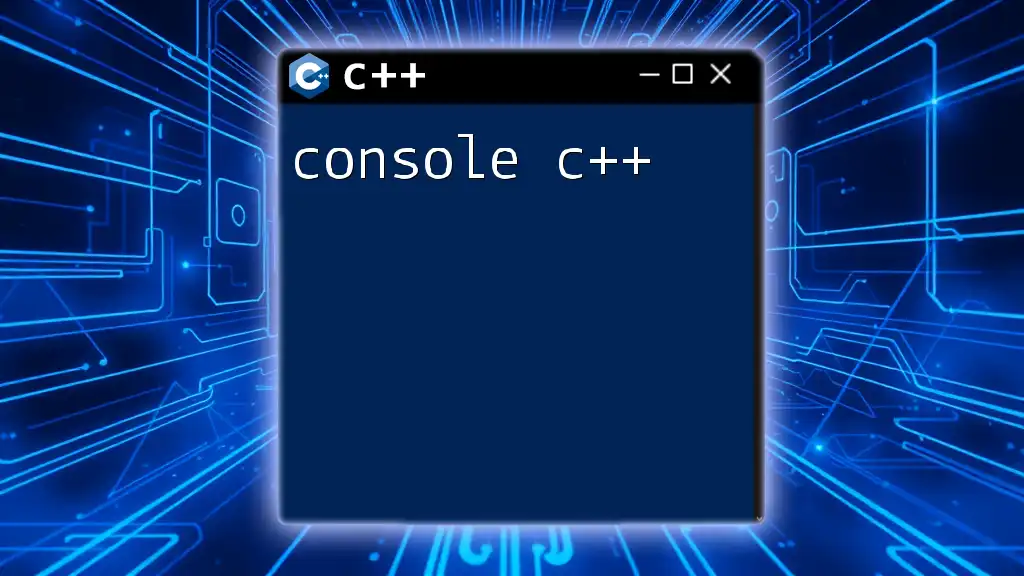
Setting Up the C++ Environment
Required Tools and Software
Before coding, you'll need the right tools. Here are some recommendations for setting up your C++ development environment:
- Use popular IDEs such as Visual Studio, Code::Blocks, or CLion. Each of these provides robust support for C++ development.
- Ensure you have a C++ compiler installed, such as GCC or Clang, to run your code efficiently.
- Consider using libraries like SFML or SDL if you want to implement graphical displays for your cells, which can elevate the simulation.
Initializing a C++ Project
To create a new project, follow these steps:
- Open your chosen IDE.
- Create a new project and select a console application template.
- Set up a basic file structure that includes main files such as `main.cpp`, and, potentially, header files for functions.
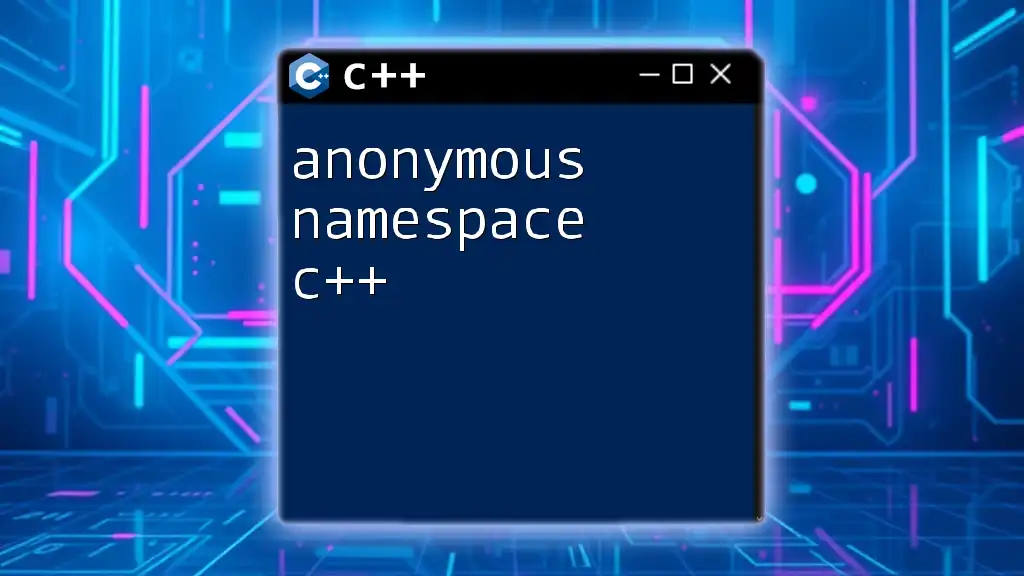
Implementing Conway's Game of Life in C++
Defining the Grid
The next step is to create a way to represent the grid. In C++, this can be done using a 2D array or a vector to denote the cells. Below is a simple illustration for defining a grid of 20x20 cells:
const int width = 20;
const int height = 20;
bool grid[width][height] = { false };
You can manipulate the dimensions according to your requirements, but keeping it manageable is essential, especially while debugging.
Creating the Game Logic
Cell State and Neighbors
The core of the Game of Life is the management of each cell's state. Cells will need to know their neighbors to apply the rules effectively. To count the living neighbors of a given cell, you can implement the following function:
int countNeighbors(int x, int y) {
int count = 0;
for (int i = x - 1; i <= x + 1; i++) {
for (int j = y - 1; j <= y + 1; j++) {
if (i == x && j == y) continue; // Skip the cell itself
if (i >= 0 && i < width && j >= 0 && j < height && grid[i][j]) {
count++;
}
}
}
return count;
}
This function iterates through the surrounding cells to count how many are alive, returning that number to guide state transitions.
Applying the Game Rules
Now, to apply the rules of Conway's Game of Life, you will need to create a function to update the entire grid:
void updateGrid() {
bool newGrid[width][height] = { false };
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
int neighbors = countNeighbors(x, y);
if (grid[x][y]) {
// Cell is alive
newGrid[x][y] = (neighbors == 2 || neighbors == 3); // Survive
} else {
// Cell is dead
newGrid[x][y] = (neighbors == 3); // Reproduction
}
}
}
// Update the main grid
memcpy(grid, newGrid, width * height * sizeof(bool));
}
This function uses the neighbor count to determine if each cell should live, die, or become alive.
Displaying the Game
Text-Based Display
For initial testing and exploration, a simple text-based output can help visualize the grid. You can use the following function to display the current state of the grid in the console:
void displayGrid() {
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
std::cout << (grid[x][y] ? "O" : ".") << " ";
}
std::cout << std::endl;
}
}
In this output, alive cells are represented by "O", while dead cells are represented by ".". This makes it easy to see how the state of the game changes over time.
Graphical Display (Optional)
If you want to take your simulation a step further, consider implementing a graphical output. Libraries such as SFML or SDL can facilitate this process. Here’s a brief snippet demonstrating how you might begin setting up a graphical output:
#include <SFML/Graphics.hpp>
void graphicalDisplay() {
sf::RenderWindow window(sf::VideoMode(800, 800), "Conway's Game of Life");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed) window.close();
}
window.clear();
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
if (grid[x][y]) {
sf::RectangleShape cell(sf::Vector2f(20, 20));
cell.setPosition(x * 20, y * 20);
cell.setFillColor(sf::Color::Green);
window.draw(cell);
}
}
}
window.display();
}
}
In this example, an 800x800 window shows the grid with each cell represented as a rectangle.
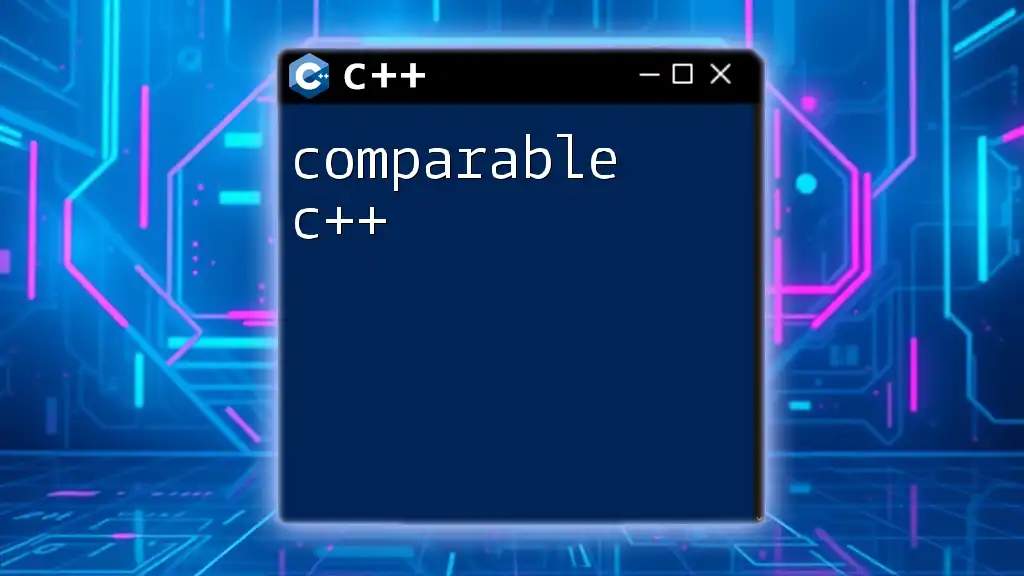
Running the Game
Game Loop Implementation
To bring everything together, implement a game loop that calls the `updateGrid()` and `displayGrid()` functions sequentially:
int main() {
// Initialize your grid, perhaps with a pattern
while (true) {
updateGrid();
displayGrid();
// Delay to control speed
std::this_thread::sleep_for(std::chrono::milliseconds(100));
}
return 0;
}
This loop will continue to run indefinitely, updating and displaying the grid according to Conway's Game of Life rules.
Example Implementation
By combining all segments above, you can achieve a fully functional implementation of Conway's Game of Life in C++. Review the entirety of your code and optimize it as necessary, ensuring efficiency and performance.
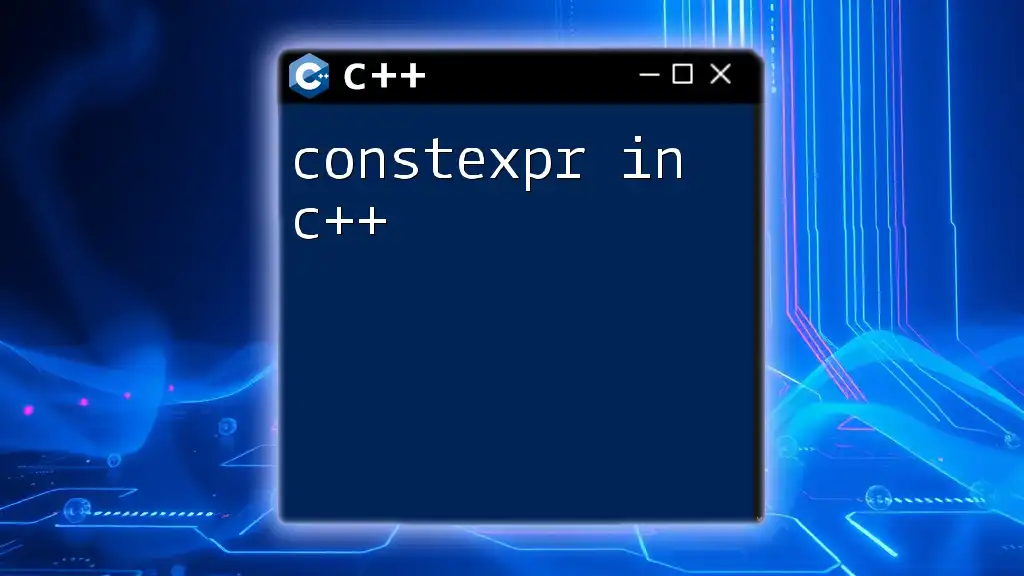
Conclusion
Conway's Game of Life serves as an excellent introduction to cellular automata and offers a foundation for understanding complex systems in C++. By creating this simulation, you not only enhance your programming skills but also appreciate the intricate patterns that arise from simple rules.
As you continue your journey in programming, explore further variations of the Game of Life or consider its applications in various fields, from biology to artificial intelligence. You'll find that each new challenge enhances your understanding of both C++ and the underlying concepts of computation.
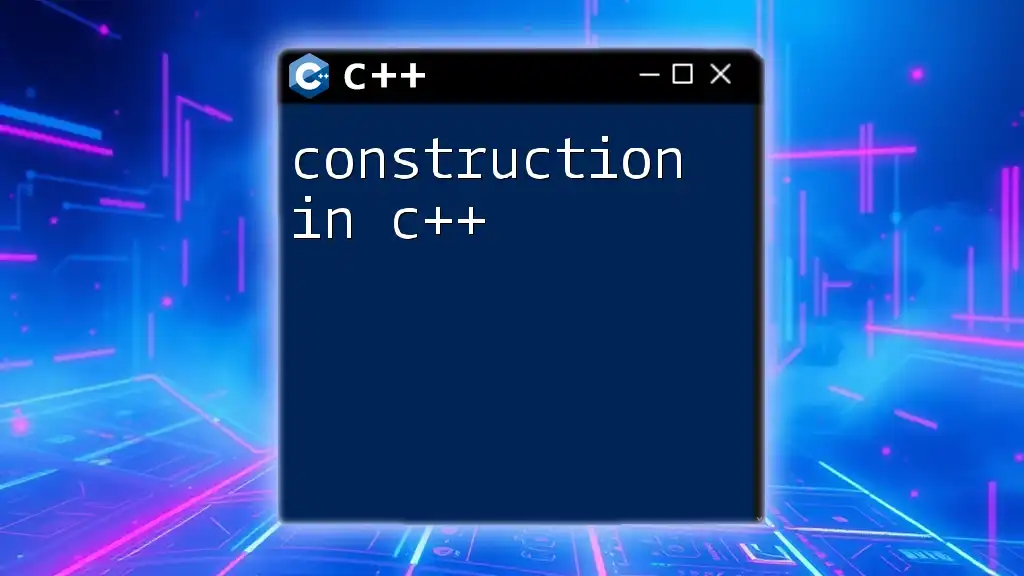
FAQs about Conway's Game of Life in C++
What is Conway's Game of Life?
Conway's Game of Life is a zero-player game that simulates the evolution of a grid of cells based on specific rules governing their states.
How can I visualize the Game of Life in C++?
You can visualize it using text-based interfaces or graphical libraries such as SFML or SDL for a more engaging experience.
What are some common patterns in the Game of Life?
Common patterns include oscillators, gliders, and still lifes. These can emerge from the initial configurations of live cells and lead to fascinating behaviors.
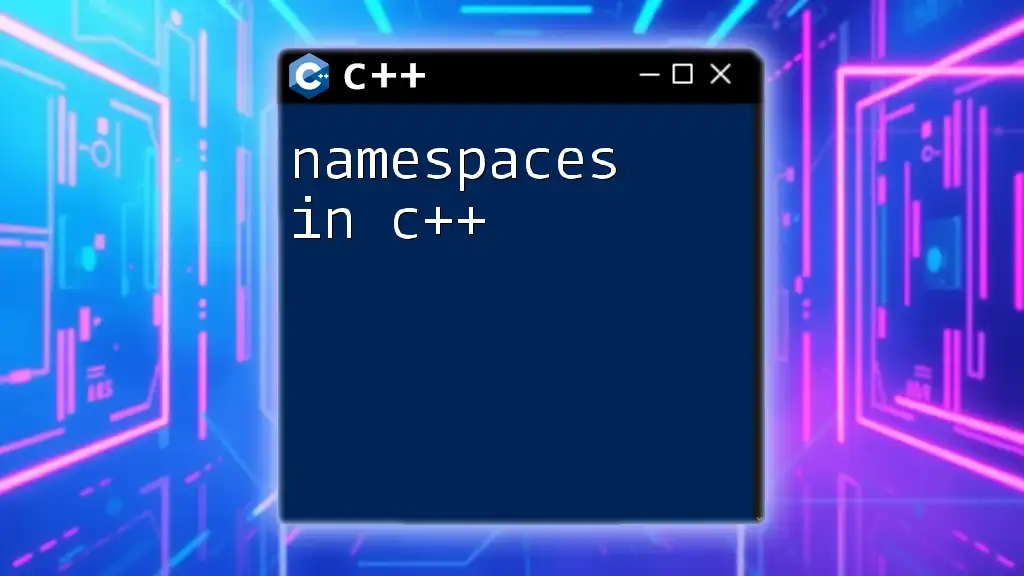
Additional Resources
To further your understanding, consider exploring online resources, forums, and communities focused on C++ and cellular automata. Learning from others and sharing your implementation can provide valuable insights and inspiration for your projects.
Feel free to experiment, modify, and take your version of Conway’s Game of Life in C++ in new directions!