C++ is a versatile programming language that allows you to create high-performance applications, including system software, games, and real-time simulations, with direct control over hardware and memory.
#include <iostream>
int main() {
std::cout << "Hello, C++ World!" << std::endl;
return 0;
}
The Power of C++: An Overview
What Makes C++ Unique?
C++ is a multi-paradigm programming language that combines the advantages of high-level and low-level programming. One of its most vital features is Object-Oriented Programming (OOP), which allows developers to create complex applications with reusable code. Key OOP concepts include:
- Classes: Blueprints for creating objects that encapsulate data for the object and methods to manipulate that data.
- Inheritance: A mechanism to create new classes from existing classes, promoting code reuse and organization.
- Polymorphism: The ability to present the same interface for different data types, enhancing flexibility in programming.
C++ also offers low-level memory manipulation capabilities through the use of pointers, making it easier to access hardware resources directly. This combination of high performance and abstraction sets C++ apart from many other programming languages.
Performance Optimization
Another critical advantage of C++ is its ability to deliver optimal performance. This is particularly beneficial for systems where resource management is crucial. The language allows developers to fine-tune their code to achieve superior speed and efficiency, making it a preferred choice for applications requiring high-performance execution.

Applications of C++
Software Development
Desktop Applications
C++ is widely utilized for developing cross-platform desktop applications. Libraries like Qt and wxWidgets empower developers to create rich user interfaces while maintaining performance. For example:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, World!");
button.show();
return app.exec();
}
Mobile Applications
C++ can also be combined with mobile technologies. Using the Android NDK, developers can integrate C++ with Java or Kotlin to leverage native performance in mobile applications. This is particularly helpful in applications where speed is paramount, such as gaming or multimedia apps.
Game Development
Why C++ Dominates Game Development
C++ is the backbone of many prominent game engines like Unreal Engine and Unity. Its high performance is essential for real-time systems, allowing smoother gameplay experiences. The ability to manipulate hardware resources and manage memory efficiently is a massive advantage in this field.
Here's a simple example of a game loop in C++:
#include <iostream>
bool gameRunning = true;
void updateGameState() {
// Game logic goes here
}
void renderGraphics() {
// Drawing logic goes here
}
int main() {
while (gameRunning) {
updateGameState();
renderGraphics();
}
return 0;
}
Systems Programming
Operating Systems and Device Drivers
C++ plays a critical role in systems programming, particularly in developing operating systems and device drivers. Its ability to manipulate hardware and handle low-level tasks makes it indispensable in environments where performance and system resource management are crucial.
Writing a basic device driver in C++ allows for direct interaction with hardware, facilitating the creation of software that effectively communicates with peripherals.
Scientific and Engineering Applications
High-Performance Computing (HPC)
C++ is often utilized in scientific and engineering applications due to its speed and efficiency. It excels in numerical simulations and algorithms, making it ideal for applications in physics, bioinformatics, and complex modeling.
For example, a basic Monte Carlo simulation to model random processes may look like this:
#include <iostream>
#include <cstdlib>
double MonteCarloSimulation(int trials) {
int count = 0;
for (int i = 0; i < trials; ++i) {
double x = rand() / (double)RAND_MAX;
double y = rand() / (double)RAND_MAX;
if (x * x + y * y <= 1) count++;
}
return (double)count / trials * 4;
}
int main() {
int trials = 100000;
std::cout << "Estimated Pi: " << MonteCarloSimulation(trials) << std::endl;
return 0;
}

Web Development with C++
Advantages of C++ in Web Development
While not as common as other languages, C++ has a niche in web development, primarily with high-performance server-side applications. Frameworks like Wt and Crow make it possible to build responsive web applications using C++. C++'s efficiency in handling complex algorithms can greatly benefit data-intensive web applications.
Here is a simple example of a web server using the Crow framework:
#include "crow.h"
int main() {
crow::SimpleApp app;
CROW_ROUTE(app, "/")([](){
return "Hello, World!";
});
app.port(18080).multithreaded().run();
}
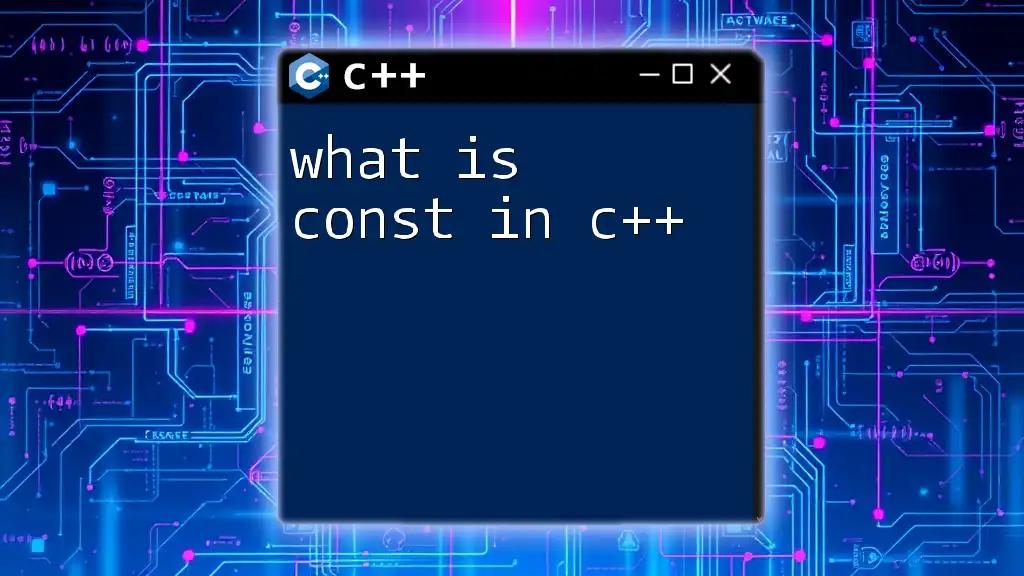
C++ in Financial Applications
Why C++ is Preferred in Finance
C++ is often the language of choice in financial sectors, particularly for quantitative finance and algorithmic trading due to its performance and reliability. Low-level manipulation allows for precise control over data structures and algorithm execution, which is essential in high-speed trading environments.
Financial applications may range from risk management systems to complex predictive algorithms, illustrating the versatility of C++ in processing vast amounts of financial data promptly.
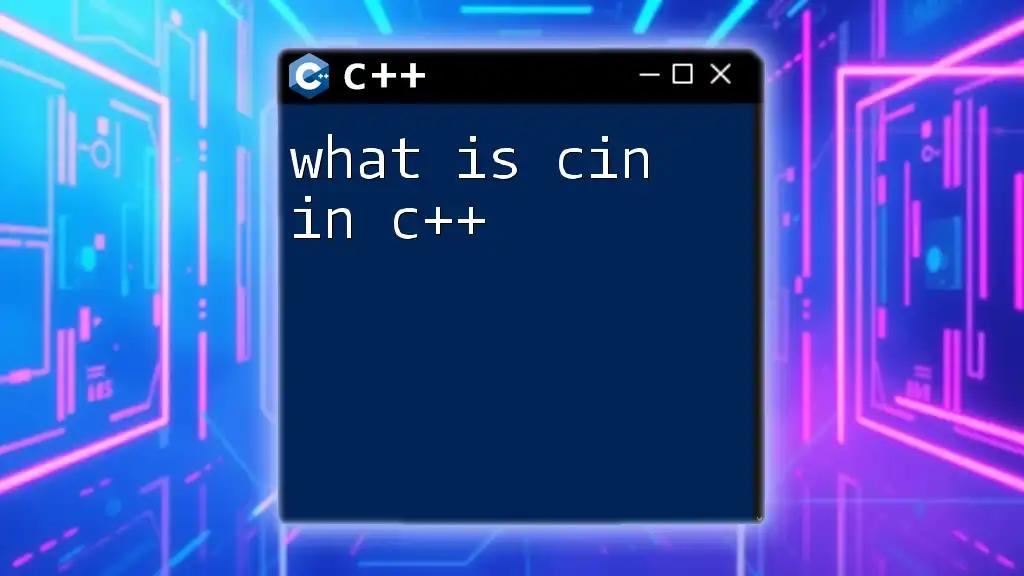
Conclusion
In this comprehensive overview, we've explored what you can do with C++, highlighting its versatility across various domains such as software development, game programming, systems programming, scientific computing, web development, and financial applications. C++'s unique capabilities make it an essential language for anyone looking to deepen their programming knowledge. By mastering C++, you open doors to countless opportunities in the tech landscape.
Whether you are a beginner or a seasoned developer, expanding your skills in C++ will undoubtedly propel your career and enhance your ability to create efficient and powerful applications.