To extract a character from a string in C++, you can use the `at()` method or the array subscript operator, providing the index of the character you wish to access.
Here's a code snippet demonstrating both methods:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
char firstChar = str.at(0); // Using at() method
char secondChar = str[1]; // Using array subscript operator
std::cout << "First character: " << firstChar << std::endl;
std::cout << "Second character: " << secondChar << std::endl;
return 0;
}
Understanding Strings in C++
What is a String?
In C++, a string is a sequence of characters used to represent text. There are primarily two types of strings: C-style strings and C++ strings.
- C-style strings are arrays of characters terminated by a null character (`'\0'`). They are less convenient because you have to manage memory and string termination manually.
- C++ strings, on the other hand, come from the `std::string` class, which is part of the C++ Standard Library. This class provides various functions to manipulate strings easily and safely.
For example, you can create a string in C++ like this:
std::string myString = "Hello, World!";
Why Extract Characters?
Extracting characters from strings is a common operation in many programming tasks. Real-world scenarios include:
- Data parsing: Extracting specific characters to analyze data formats.
- User input handling: Processing user input by grabbing specific letters or symbols for validation or comprehension.
- Text analysis: Analyzing content for a particular character occurrence or formatting.
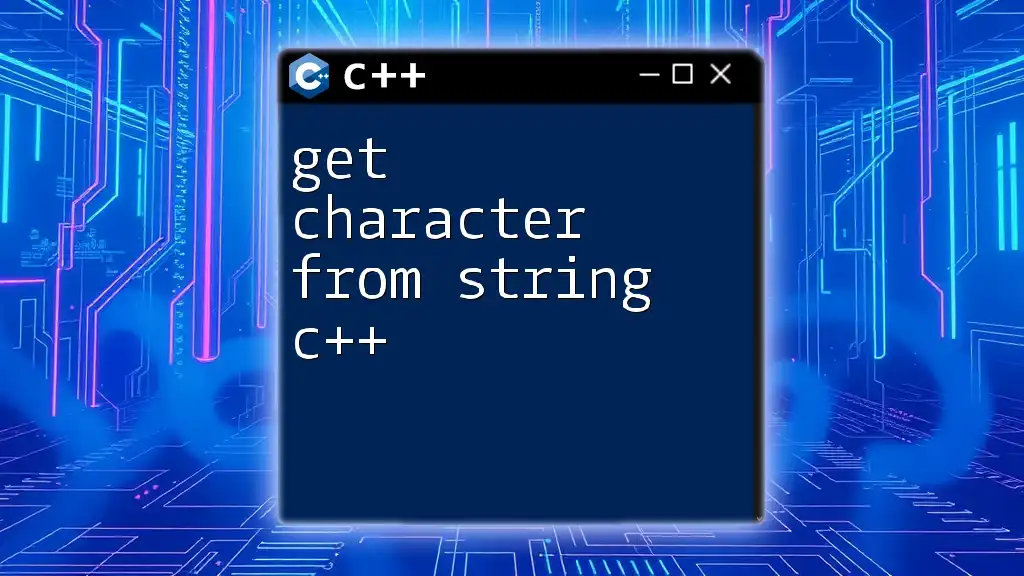
Methods to Extract Characters
Using the `at()` Method
The `at()` method is a member function of the `std::string` class that allows you to safely access a character at a specified index. This method performs bounds checking, meaning it will throw an exception if you attempt to access an index outside the string's range.
For instance:
char extractedChar = myString.at(7); // Extracts 'W'
If you try to access an index out of range:
try {
char extractedChar = myString.at(15); // Throws exception
} catch (const std::out_of_range& e) {
std::cerr << e.what() << std::endl; // Prints the error message
}
This exception handling ensures that your program can provide feedback rather than crash unexpectedly.
Using the Subscript Operator (`[]`)
The subscript operator (`[]`) allows for a more straightforward way to access characters in a string. While it is convenient, it does not perform bounds checking. This can lead to undefined behavior if used improperly.
Here is how you can use it:
char extractedChar = myString[7]; // Extracts 'W'
However, keep in mind the risks. For example:
char extractedChar = myString[15]; // Undefined behavior (may cause a crash)
Iterating Through a String
You can also extract characters by iterating through the string using loops. This is especially useful if you wish to process each character individually.
For example, using a simple for loop:
for (size_t i = 0; i < myString.length(); ++i) {
std::cout << myString[i] << std::endl; // Prints each character
}
Alternatively, you can use a range-based for loop:
for (char ch : myString) {
std::cout << ch << std::endl; // Prints each character
}
Using `std::string::find` Method
The `find` method can be used when you want to locate a specific character or substring. If the character is found, it returns its position; if not, it returns `std::string::npos`.
You could use it as follows:
size_t pos = myString.find('W'); // Finds position of 'W'
if (pos != std::string::npos) {
char extractedChar = myString[pos]; // Extracts 'W' if found
std::cout << "Found: " << extractedChar << std::endl;
} else {
std::cout << "'W' not found" << std::endl;
}
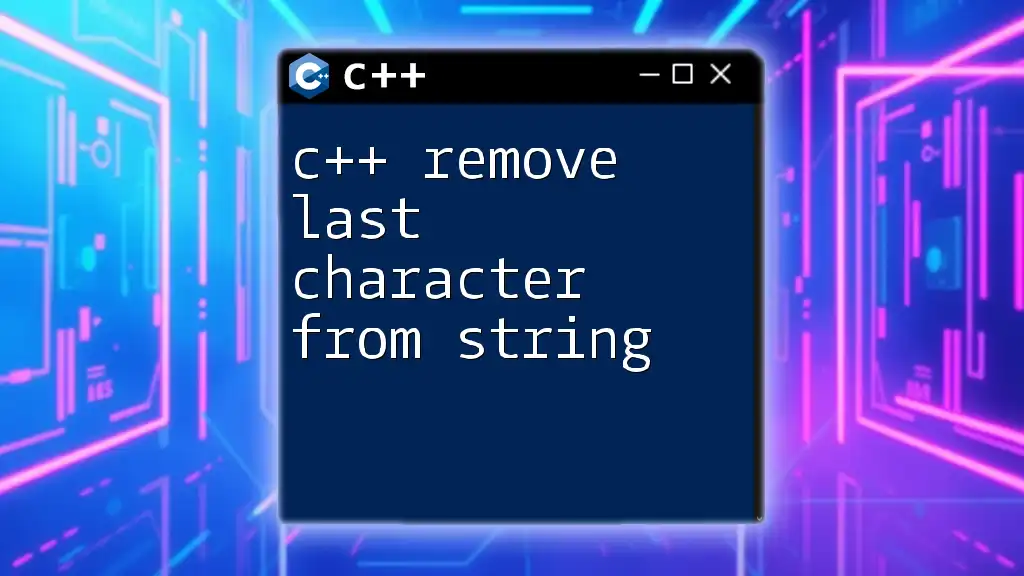
Practical Example: Extracting from User Input
In many real-world applications, you'll be working with user-provided strings. Here’s how to extract characters from a string entered by the user:
-
Prompting the user for input: You can use `std::getline` to capture the entire line of input, including spaces.
-
Validating input: Always ensure your string has characters before attempting to extract.
-
Extracting characters based on user-defined criteria:
std::string userInput;
std::cout << "Enter a string: ";
std::getline(std::cin, userInput);
if (!userInput.empty()) {
char extracted = userInput.at(0); // Extracts the first character
std::cout << "The first character is: " << extracted << std::endl;
} else {
std::cout << "Input string is empty." << std::endl;
}
This check avoids accessing out-of-bounds characters and enhances your application's stability.
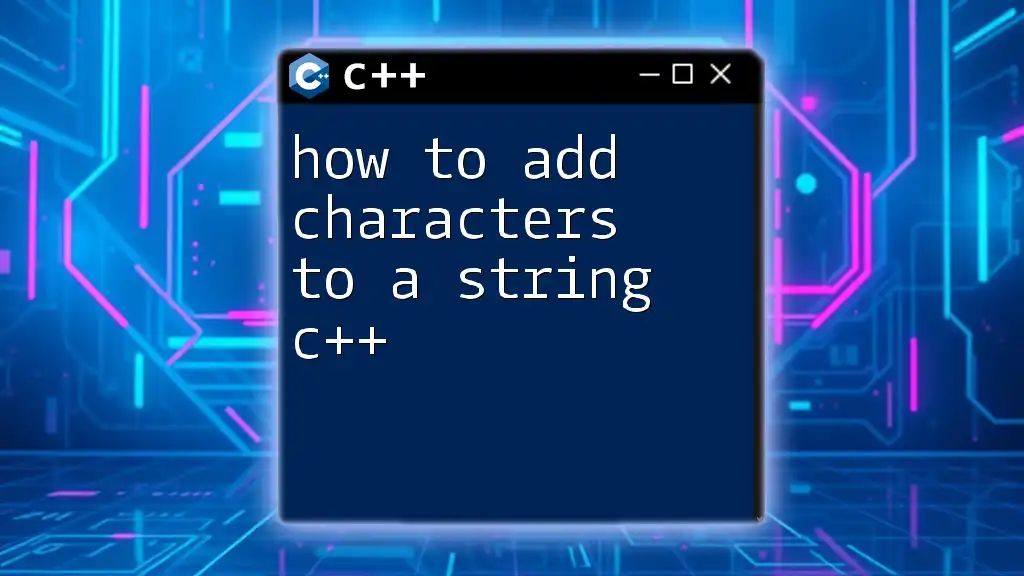
Best Practices for String Manipulation
Validating Indices
Always validate indices when extracting characters to prevent accessing invalid memory locations. Using `at()` helps with this, but be mindful when using the `[]` operator.
Leveraging Standard Library Features
C++ Standard Library provides various string manipulation functions. Familiarize yourself with them to write cleaner, safer code.
Performance Considerations
While `at()` provides safety, if performance is a critical concern in a loop where bounds are guaranteed, the subscript operator may be more efficient. However, always balance safety with performance.
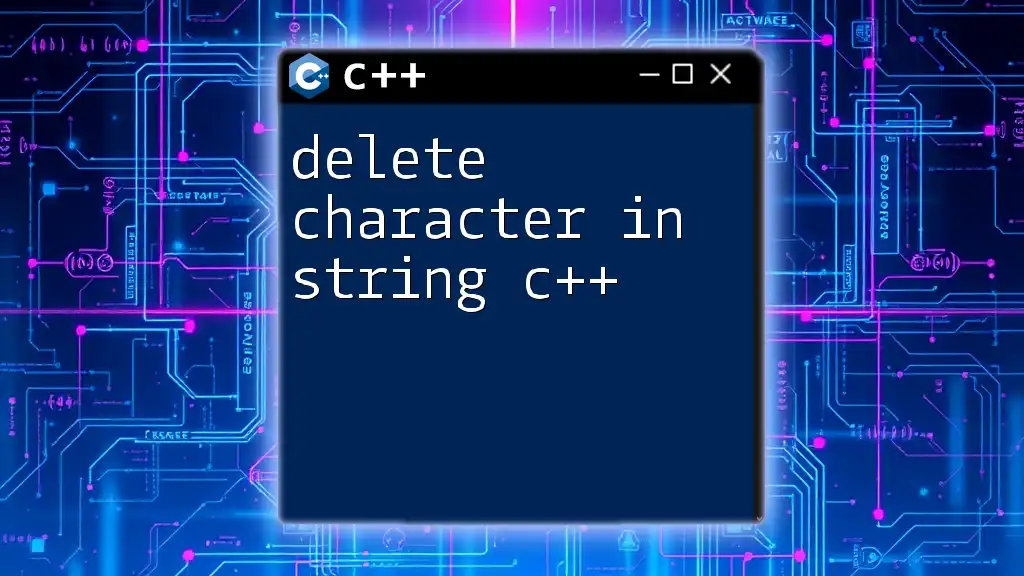
Conclusion
In this guide, we explored how to extract a character from a string in C++ using various methods such as `at()`, the subscript operator, iteration, and the `find` method. We illustrated the importance of safety by handling out-of-bounds access, especially when dealing with user input. Armed with this knowledge, you can manipulate strings more effectively in your C++ applications.
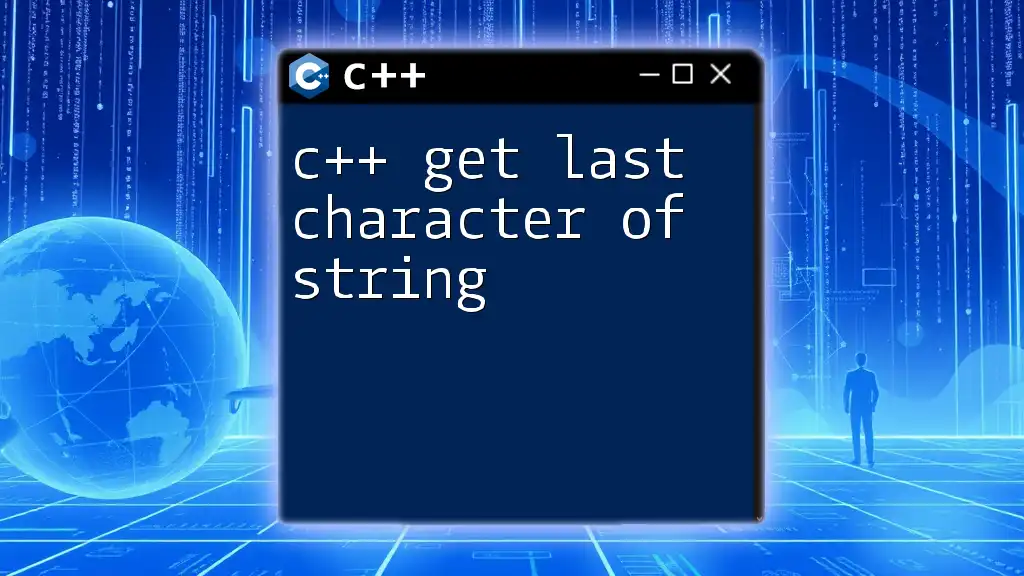
Additional Resources
Recommended Reading
Familiarize yourself with the C++ Standard Library documentation for the `std::string` class to explore additional functions and methods available for string manipulation.
Community Engagement
We encourage you to practice these techniques and share your experiences. Join the discussion on forums or social media channels where C++ enthusiasts connect and learn!