C++ is a powerful programming language used for system/software development, and understanding its core concepts is essential for effective coding; for example, here’s how to declare and initialize a variable:
int main() {
int age = 25; // Declare and initialize an integer variable
return 0;
}
Understanding C++ Basics
What is C++?
C++ is a powerful programming language that evolved from C in the early 1980s and introduced object-oriented programming (OOP) principles. It offers features that enhance the capabilities of procedural programming while also providing robust tools for developing complex applications. Known for its efficiency and control over system resources, C++ is widely used in areas such as game development, systems programming, and high-performance applications.
Setting Up the Environment
Choosing the Right IDE
When starting with C++, selecting an Integrated Development Environment (IDE) can significantly enhance your programming experience. Here are a few popular IDEs:
- Visual Studio: Best suited for Windows users, it provides powerful debugging and development tools.
- Code::Blocks: A lightweight, open-source option that is easy to set up and use.
- CLion: A cross-platform IDE by JetBrains, great for advanced development with built-in tools.
Installation Instructions
Setting up your environment is straightforward. Just follow these steps:
- Download the preferred IDE from its official website.
- Follow the installation instructions specific to your operating system.
- Ensure you have the C++ compiler installed (many IDEs come bundled with it).
Hello World in C++
To truly understand C++, your journey starts with writing your first program: the classic "Hello, World!" example. Here’s a simple code snippet:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code:
- `#include <iostream>` brings in the input-output stream library needed for console I/O.
- `int main()` is the entry point for any C++ program.
- `std::cout` is used to print to the console, and `std::endl` adds a new line and flushes the output buffer.
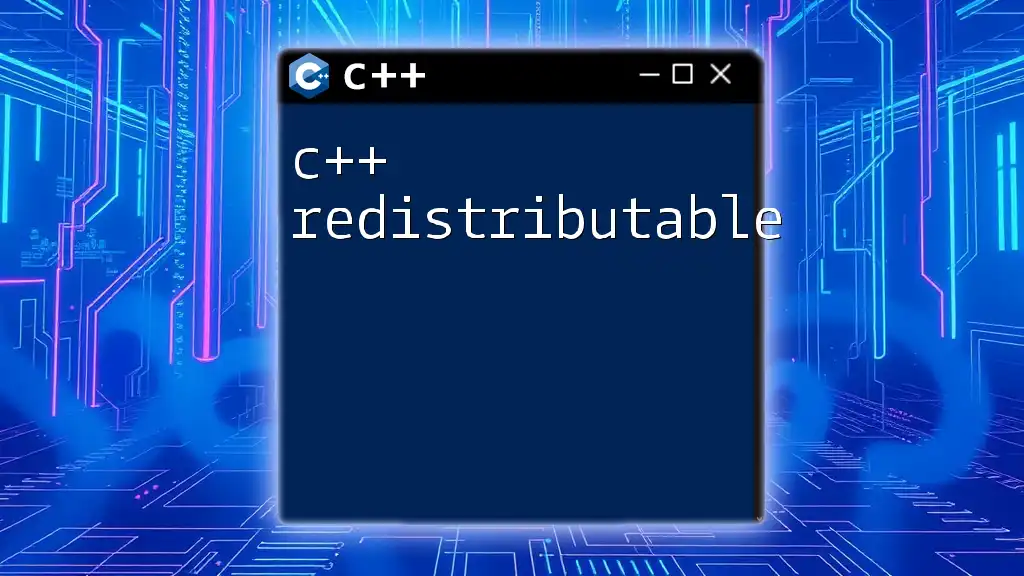
Core Concepts of C++
Variables and Data Types
Understanding Variables
Variables are essentially storage units for data, and every variable in C++ has a data type that determines its size and operations allowed.
Common Data Types in C++
- `int`: Represents integer values.
- `float`: Used for single-precision floating-point numbers.
- `char`: Represents single characters.
- `string`: A sequence of characters.
Here's an example of defining different variables:
int age = 30;
float salary = 55000.50;
char grade = 'A';
std::string name = "John Doe";
Const and Volatile Qualifiers
- const: When a variable is declared as constant, its value cannot be changed after its initial assignment. This is useful for defining fixed values.
- volatile: It indicates that a variable may be changed by other components outside of the program's control (e.g., hardware), preventing the compiler from optimizing away accesses to it.
Control Structures
Conditional Statements
Conditional statements allow you to execute code based on specific conditions. C++ offers `if`, `else if`, and `else` constructs. For example:
if (age > 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
Loops
Loops are essential for executing a block of code multiple times. The `for`, `while`, and `do-while` loops are common in C++. Here’s how they work:
- For Loop:
for (int i = 0; i < 5; i++) {
std::cout << i << " ";
}
- While Loop:
int i = 0;
while (i < 5) {
std::cout << i << " ";
i++;
}
- Do-While Loop:
int i = 0;
do {
std::cout << i << " ";
i++;
} while (i < 5);
Functions in C++
Functions in C++ are blocks of code designed to perform a particular task. They help organize code and promote reusability. Here's how you can define and call a function:
void greet() {
std::cout << "Welcome to C++!" << std::endl;
}
int main() {
greet(); // Calling the function
return 0;
}
Object-Oriented Programming (OOP)
Introducing OOP Concepts
C++ is heavily focused on OOP, which brings concepts like encapsulation, inheritance, and polymorphism to life.
Class and Object
Classes serve as blueprints for objects. An object is an instance of a class.
class Car {
public:
std::string model;
void display() {
std::cout << "Model: " << model << std::endl;
}
};
// Creating an object
Car myCar;
myCar.model = "Tesla Model S";
myCar.display();
Inheritance
Inheritance allows a class to inherit properties and methods from another class. It promotes code reusability.
Single vs Multiple Inheritance:
- Single Inheritance:
class Vehicle {
public:
void start() {
std::cout << "Vehicle started." << std::endl;
}
};
class Car : public Vehicle {
// Inherits from Vehicle
};
- Multiple Inheritance:
class Engine {
public:
void run() {
std::cout << "Engine running." << std::endl;
}
};
class Car : public Vehicle, public Engine {
// Inherits from both Vehicle and Engine
};
Polymorphism
Polymorphism enables functions to use objects of different classes through interfaces. It’s categorized as compile-time and run-time polymorphism.
Compile-time Polymorphism through Function Overloading:
void display(int value) {
std::cout << "Integer: " << value << std::endl;
}
void display(double value) {
std::cout << "Double: " << value << std::endl;
}
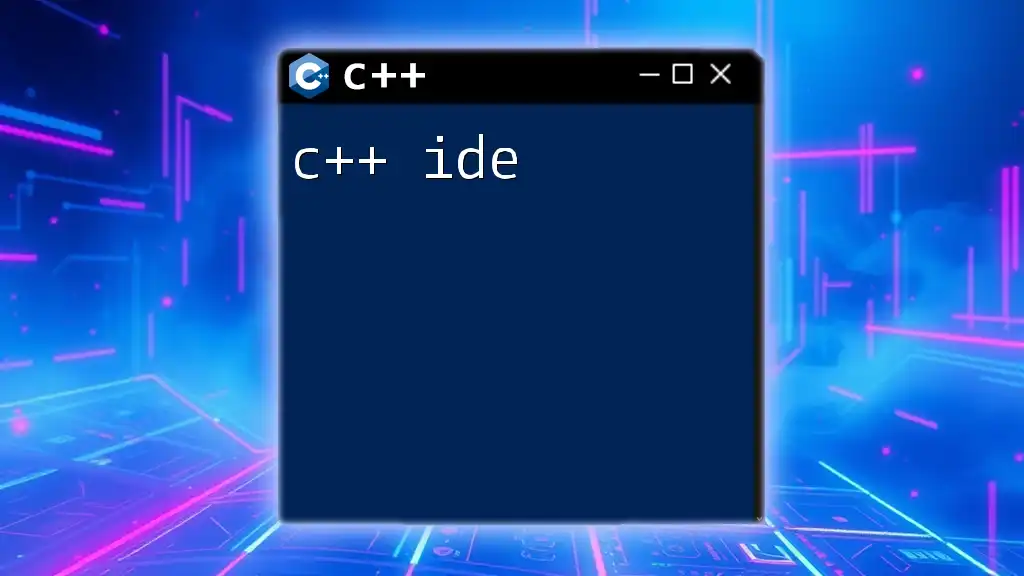
Advanced C++ Concepts
Templates
Templates allow functions or classes to operate with generic types. This promotes code reusability and type safety.
Example of a function template:
template <typename T>
T add(T a, T b) {
return a + b;
}
Exception Handling
Exception handling is crucial for maintaining the stability of software. C++ provides `try`, `catch`, and `throw` to handle errors gracefully.
try {
throw std::runtime_error("An error occurred!");
} catch (const std::exception &e) {
std::cout << e.what() << std::endl;
}
C++ Standard Library
Introduction
The C++ Standard Library (STL) provides a rich set of functionalities, including algorithms, data structures, and input/output operations. Familiarity with STL can significantly enhance productivity and code quality.
Example of Using Vectors
Vectors are dynamic arrays that can grow in size. Here’s how you can utilize them:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Using `std::vector` allows for convenient handling of collections without manual memory management.
Smart Pointers
Smart pointers like `unique_ptr`, `shared_ptr`, and `weak_ptr` provide automatic memory management, helping avoid memory leaks.
Example:
#include <memory>
std::unique_ptr<int> ptr(new int(10));
std::cout << *ptr << std::endl;
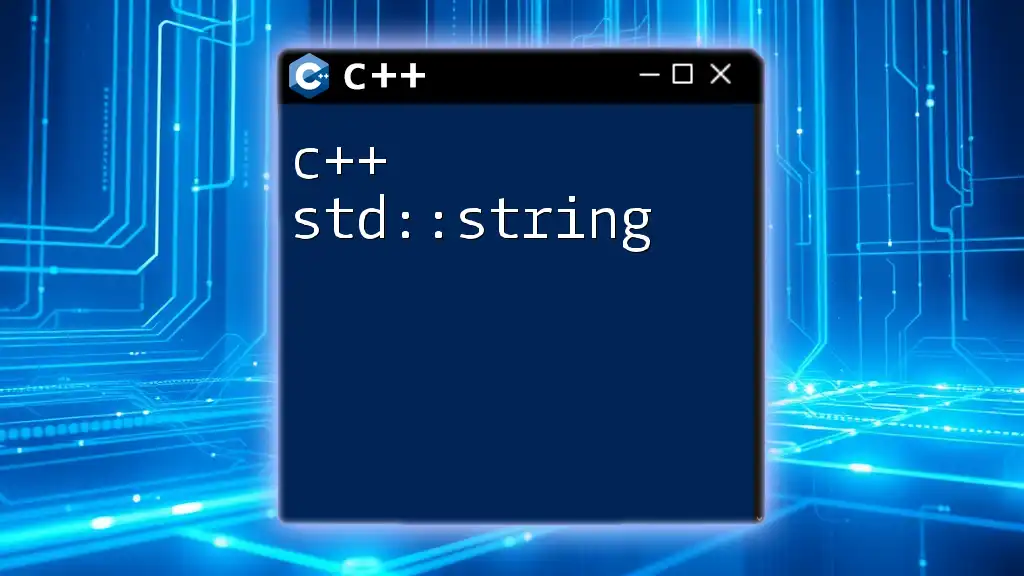
Best Practices in C++
Coding Standards
Importance of Coding Standards
Consistent coding practices create more readable and maintainable code. Adhering to standards like indentation, naming conventions, and commenting improves teamwork and project scalability.
Memory Management
Understanding Memory Leaks
Memory management is a critical aspect of C++ programming. Failing to deallocate memory properly can lead to memory leaks. Using tools like `valgrind` can help identify and fix such issues.
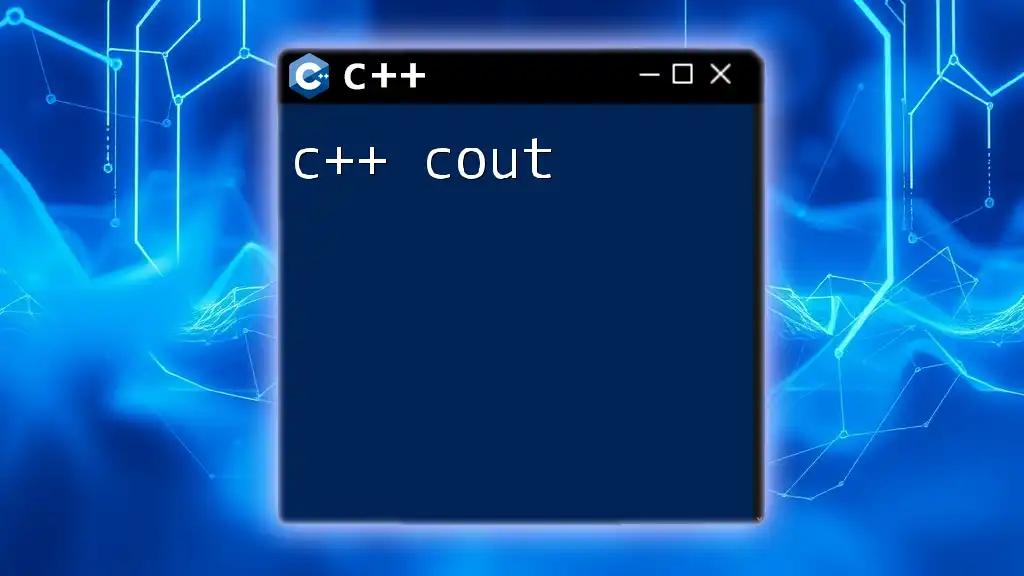
Conclusion
In this comprehensive guide, we’ve explored various fundamental and advanced C++ concepts, including object-oriented programming, templates, and exception handling. Mastering these topics will prepare you for tackling complex programming challenges and developing robust applications.
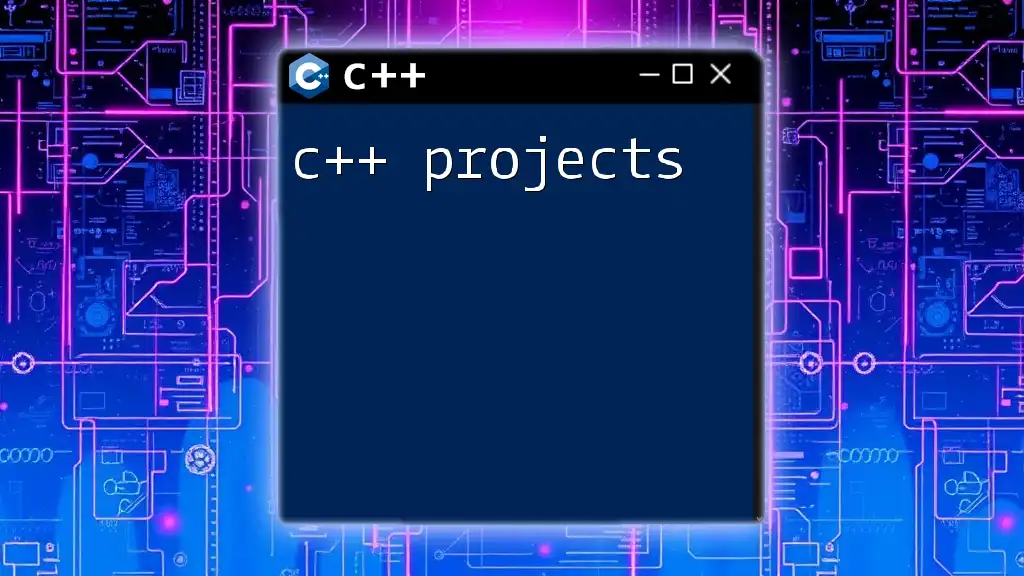
Call to Action
Engage with our community! We invite you to share your thoughts, ask questions, or discuss your experiences with C++ in the comments below. Your insights can greatly enrich the learning experience for everyone!