AOT (Ahead-of-Time) compilation in C# allows programs to be compiled to native code before execution for improved performance, while C++ natively compiles to machine code, providing similar performance benefits without the need for a separate compilation step.
Here’s a simple C++ program as an example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C# AOT
What is C#?
C# is a modern, object-oriented programming language developed by Microsoft. It is primarily used within the .NET ecosystem and leverages the Common Language Runtime (CLR) for managing application execution. Here are some of the key characteristics of C#:
- Object-Oriented Programming: C# promotes the use of classes and objects, making it easier to create modular and reusable code.
- Managed Environment: Thanks to the CLR, C# abstracts many low-level operations, enhancing security and memory management.
- Versatile Use Cases: C# is widely utilized for building web applications with ASP.NET, desktop applications with Windows Forms and WPF, and even game development using Unity.
What is AOT Compilation?
AOT (Ahead-of-Time) compilation refers to the process where source code is compiled into native machine code before the program is run. Unlike JIT (Just-In-Time) compilation, which compiles code at runtime, AOT compilation results in pre-compiled binaries that can be executed directly by the operating system.
The benefits of AOT compilation include:
- Improved Performance: AOT eliminates the startup overhead associated with JIT compilation.
- Greater Predictability: Because the code is pre-compiled, developers can analyze and optimize performance during the compilation phase.
Benefits of C# AOT
One significant advantage of C# AOT is performance optimization. Applications that leverage AOT can achieve faster startup times as the required code has already been compiled and is ready to run.
C# AOT also enhances memory usage, as unused code and data can be stripped away during the compilation process. AOT enables developers to build applications that exhibit cross-platform capabilities while maintaining consistent and efficient performance across different environments.

Understanding C++
What is C++?
C++ is a powerful, low-level programming language that extends the C programming language with object-oriented features. It allows for fine control over system resources and memory. Notable characteristics include:
- Multi-Paradigm Support: C++ supports procedural, object-oriented, and functional programming styles, allowing developers to choose the best approach for their specific needs.
- Versatile Use Cases: C++ is heavily used in system and software development, real-time simulation, and high-performance game development. Its performance makes it suitable for tasks requiring significant computational resources.
Compilation in C++
The compilation process in C++ involves multiple stages: preprocessing, compiling, assembling, and linking. This process plays a crucial role in transforming source code into a final executably file.
In C++, AOT compilation is the standard, as the source code is compiled directly into native machine code. This results in optimized performance as there is no interpreter or runtime overhead during execution.
Benefits of C++
One of the standout benefits of C++ is its performance and control over system resources. C++ grants developers the ability to write highly efficient algorithms while providing direct access to hardware-level features.
Additionally, C++ applications run as native code, meaning they do not require intermediary layers such as virtual machines or interpreters. This reduces latency, which is vital for applications demanding low-response times, such as video games or real-time systems.
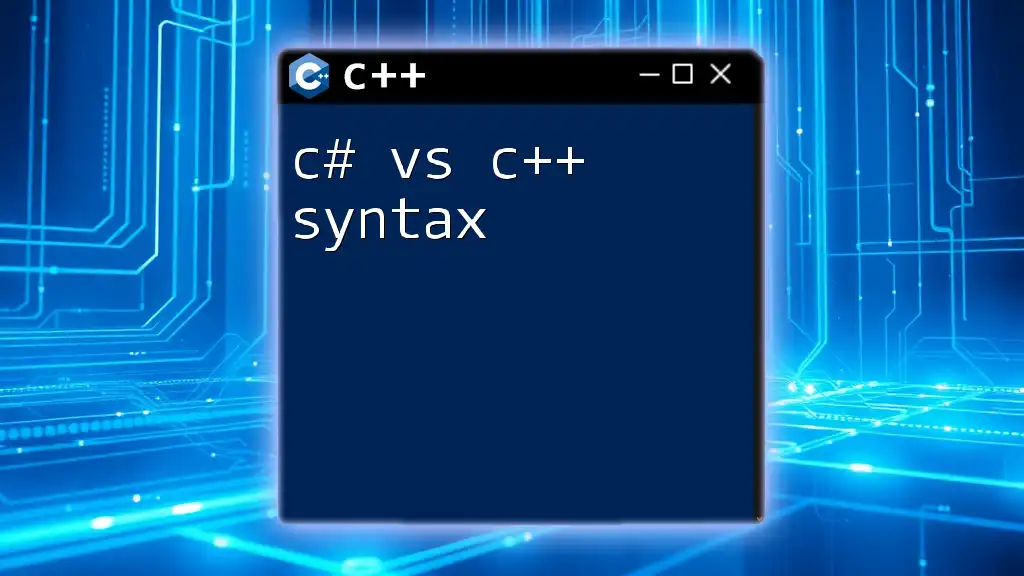
Comparing C# AOT and C++
Performance Comparison
When discussing execution speed, C++ typically outperforms C# AOT due to its native compilation and close-to-hardware execution. However, C# AOT’s performance has significantly improved over time, making it a suitable choice for many applications.
Startup times are another critical factor. AOT compilation in C# narrows the gap between it and native compiled C++ applications, as the code is ready to run immediately without the JIT overhead.
Development Experience
In terms of ease of use, C# often has an edge due to its higher-level abstractions and robust development environments, like Visual Studio. The language's syntax is often considered more readable and easier to learn for beginners.
Conversely, C++ requires a more profound understanding of programming concepts, especially concerning memory management and pointers. While this might steepen the learning curve, it allows for greater control for advanced developers.
Ecosystem and Libraries
Both C# and C++ have strong ecosystems but differ significantly in their frameworks and libraries:
- C# relies heavily on the .NET framework, which provides extensive libraries for web, desktop, and mobile applications.
- C++ benefits from a broad range of libraries, such as STL (Standard Template Library) and Boost, enabling diverse functionalities from data structures to advanced mathematical calculations.
Finally, community support for both languages remains strong. Both C# and C++ have thriving communities that produce ample resources, tutorials, and forums for developers seeking help or sharing knowledge.
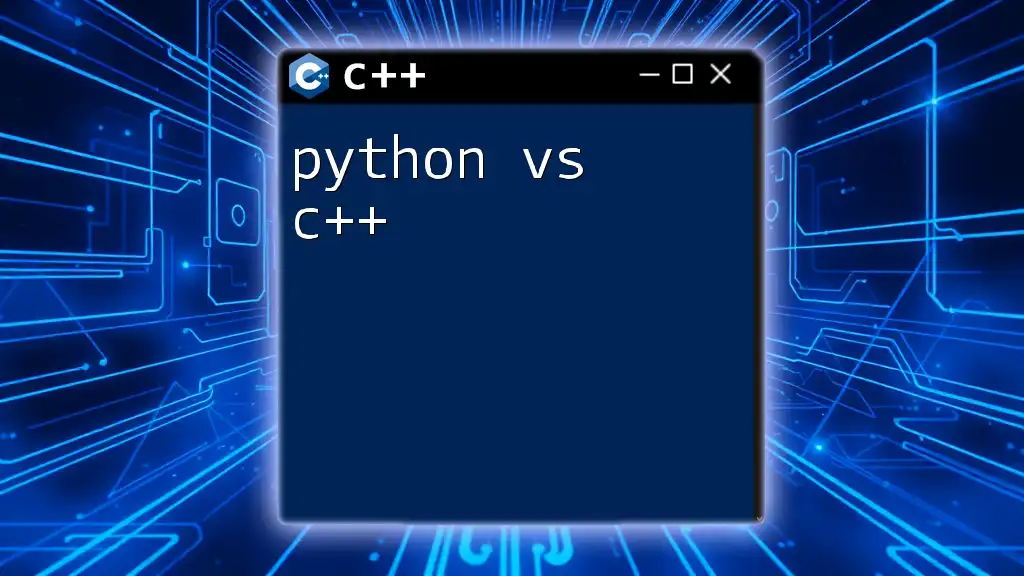
Use Cases and Applications
When to Use C# AOT
C# AOT shines in scenarios where cross-platform development is essential, such as mobile or web applications that need to run optimally on various systems, including Windows, macOS, and Linux. AOT’s ability to minimize startup time makes it ideal for applications needing quick responsiveness for users.
When to Use C++
C++ is ideal for applications demanding high performance and real-time capabilities. If you are developing system-level software, games, or simulations that require fine control over performance and resource management, C++ is the preferred choice.
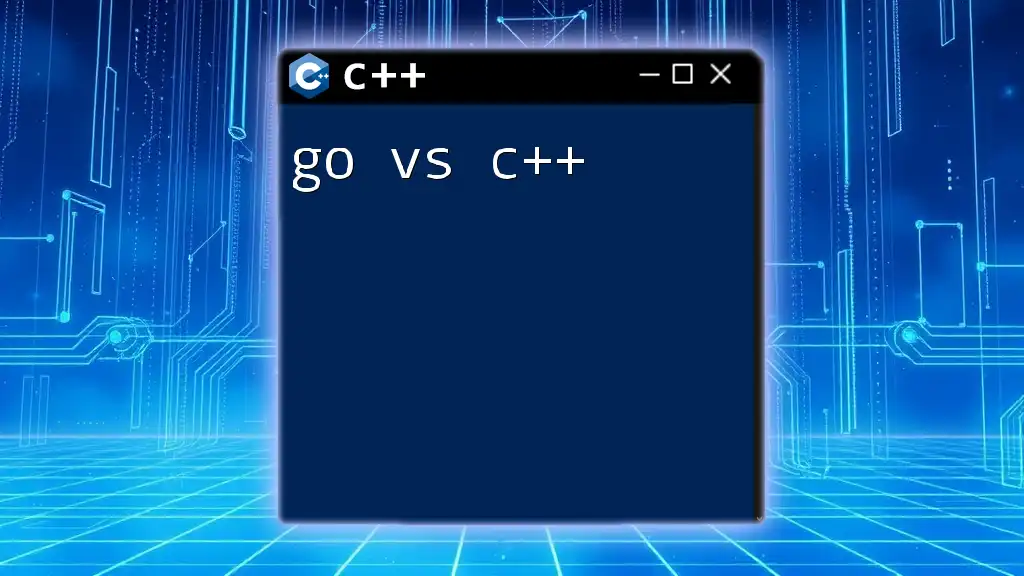
Real-world Examples
C# AOT in Action
For example, consider a C# AOT application that processes data from multiple sources for real-time analysis. Here’s a simple code example showing how C# AOT allows setup at compile time:
public class HelloWorld {
public static void Main() {
Console.WriteLine("Hello, C# AOT Compilation!");
}
}
C++ in Action
In contrast, a C++ application could be a game engine where speed and performance are critical. A simple C++ program might look like this:
#include <iostream>
int main() {
std::cout << "Hello, C++ Compilation!" << std::endl;
return 0;
}
Both languages have proven effective in different domains, showcasing their strengths depending on project requirements.
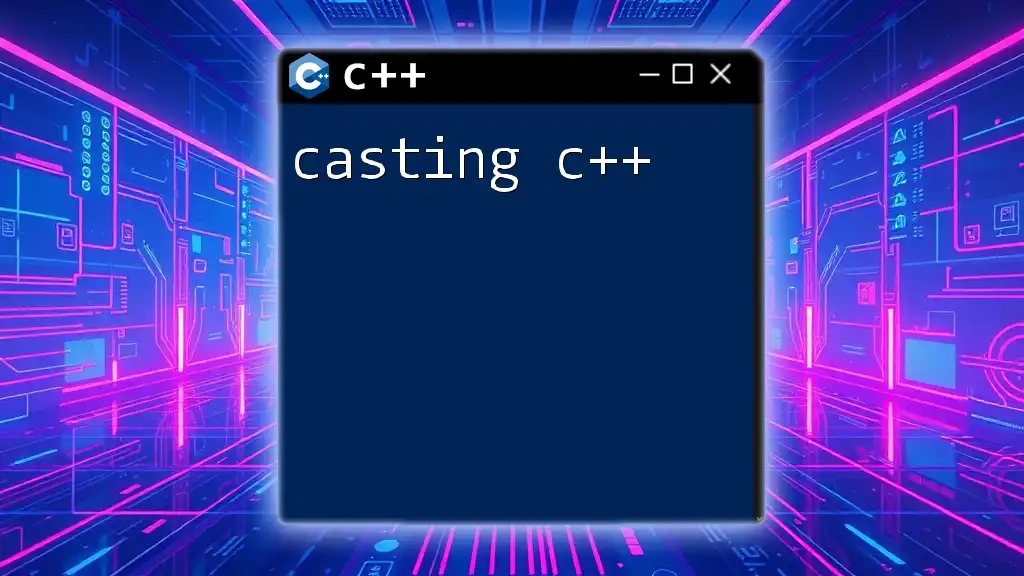
Conclusion
In summary, the comparison between C# AOT vs C++ reveals distinct characteristics and advantages for each language. C# AOT is geared toward establishing a rapid development cycle with efficient performance for cross-platform applications, while C++ is the leader for performance-critical software that requires close-to-hardware execution.
Final Thoughts
Choosing between C# AOT and C++ depends on the project objectives, the performance needs, and the development environment. By analyzing each language's features and capabilities, developers can make informed decisions that ensure their applications are both efficient and robust.
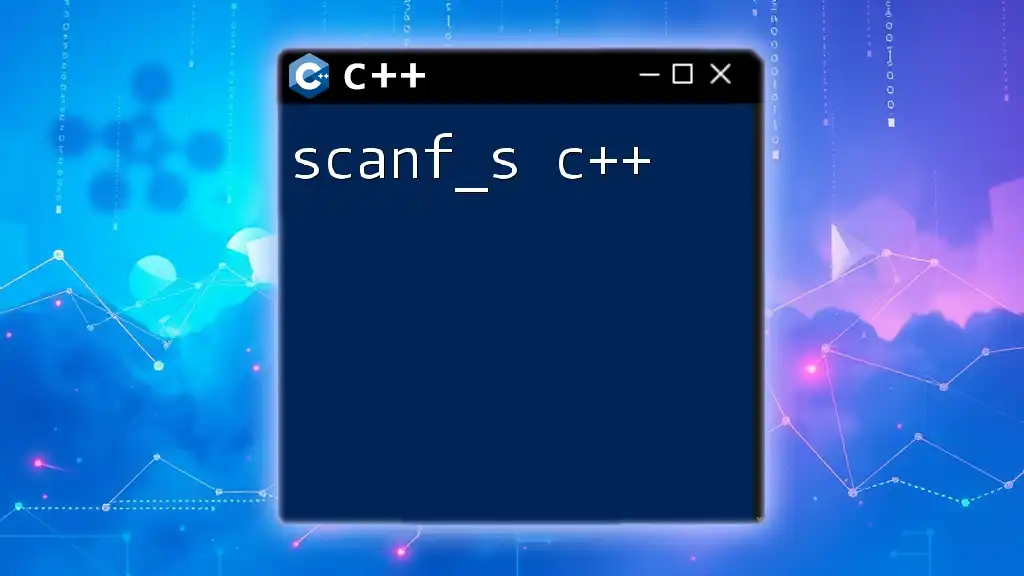
FAQs
What are the main differences in syntax between C# and C++? C# is generally more straightforward and readable, featuring built-in garbage collection. In contrast, C++ offers deeper control of memory but requires manual management.
Is C# AOT faster than C++? While C# AOT improves startup times significantly, C++ usually has an advantage in execution speed due to its nature as a compiled language.
What kind of support can developers expect for each language? Both languages benefit from large communities and extensive documentation, providing ample resources for both beginners and experienced developers.