Big O analysis in C++ is a method used to classify algorithms based on their worst-case runtime complexity as the input size grows, enabling developers to choose the most efficient algorithm for their needs.
Here's a simple example of sorting an array using Bubble Sort, which has a time complexity of O(n^2):
#include <iostream>
using namespace std;
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n-1; i++)
for (int j = 0; j < n-i-1; j++)
if (arr[j] > arr[j+1])
swap(arr[j], arr[j+1]);
}
int main() {
int arr[] = {64, 34, 25, 12, 22, 11, 90};
int n = sizeof(arr)/sizeof(arr[0]);
bubbleSort(arr, n);
cout << "Sorted array: ";
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
return 0;
}
Understanding Big O Notation
What is Big O Notation?
Big O notation is a mathematical concept used to describe the performance and efficiency of an algorithm. It provides a high-level understanding of how an algorithm's run time or space requirements grow relative to the size of the input data. In essence, Big O notation enables programmers and computer scientists to communicate the efficiency of algorithms in a standardized manner.
Key terminology in Big O Analysis includes:
- Upper limit: Represents the worst-case scenario for an algorithm's performance.
- Time complexity: Refers to the amount of computational time that an algorithm takes based on the size of the input data.
How Big O Notation Works
Big O notation classifies algorithms according to their growth rates. More specifically, it helps indicate how the run time of an algorithm increases as the input size (`n`) increases. Understanding this classification allows developers to make more informed decisions when selecting or designing algorithms. The impact of Big O notation becomes evident specifically when dealing with large data sets, where inefficiencies can significantly slow down performance.
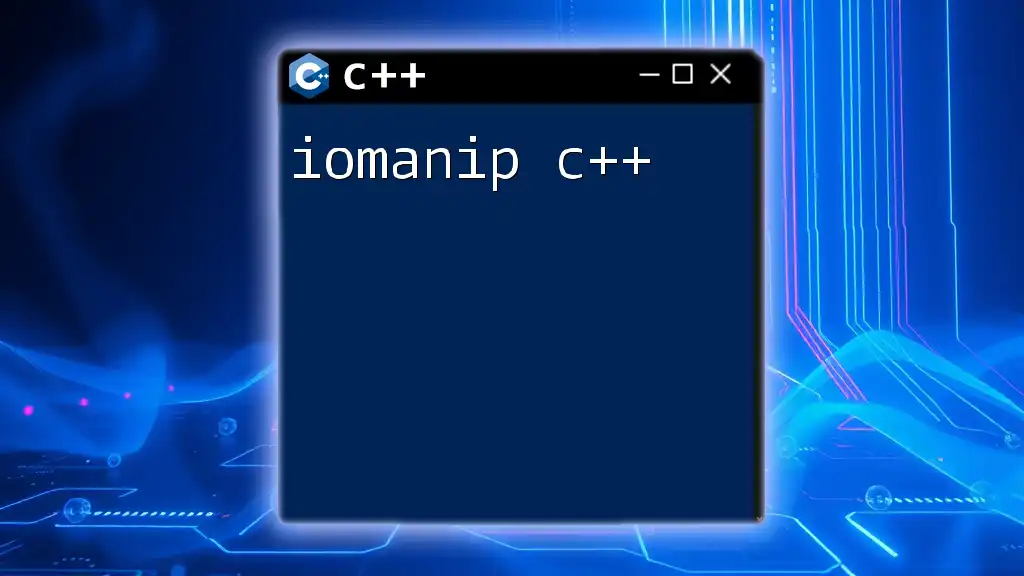
Common Big O Notation Types
Constant Time - O(1)
An algorithm is said to have constant time complexity when its run time does not change regardless of the input size. This is represented as O(1).
Example in C++:
int getFirstElement(int arr[]) {
return arr[0];
}
In this example, accessing the first element of an array takes the same amount of time no matter how large the array is. Therefore, the time complexity is O(1). This efficiency is preferred as it indicates that the algorithm executes in a predictable and fast manner.
Linear Time - O(n)
An algorithm exhibits linear time complexity (O(n)) when its run time increases linearly with the increase in input size.
Example in C++:
void printArray(int arr[], int size) {
for (int i = 0; i < size; i++) {
std::cout << arr[i] << " ";
}
}
Here, as the size of the array increases, the number of operations required to print the array elements grows proportionally. Consequently, linear time complexity is intuitive and easy to understand, impacting both performance and algorithm choice.
Quadratic Time - O(n^2)
When describing the quadratic time complexity of an algorithm, it means that the run time increases with the square of the input size (O(n²)). This often occurs in scenarios where nested loops are required.
Example in C++:
void printAllPairs(int arr[], int size) {
for (int i = 0; i < size; i++) {
for (int j = 0; j < size; j++) {
std::cout << "(" << arr[i] << ", " << arr[j] << ")" << std::endl;
}
}
}
In this example, for every iteration of the outer loop, the inner loop iterates through the entire array. Therefore, if the size of the array is `n`, the total number of operations becomes `n*n`, leading to O(n²) complexity. Quadratic time complexity can quickly lead to performance bottlenecks, particularly with larger datasets.
Logarithmic Time - O(log n)
Logarithmic time complexity (O(log n)) arises when an algorithm reduces the problem size significantly with each step. This is commonly seen in searching algorithms.
Example in C++:
int binarySearch(int arr[], int left, int right, int target) {
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) return mid;
else if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
In binary search, the size of the search space is halved with each comparison. This remarkable efficiency makes logarithmic time complexity particularly advantageous in scenarios where large datasets are involved, maintaining effective performance.
Exponential Time - O(2^n)
Exponential time complexity signifies that the run time grows exponentially with the increase in input size, represented as O(2^n). Algorithms with exponential time complexity can become impractical swiftly as the size of the input grows.
Example in C++:
int fibonacci(int n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
In this Fibonacci sequence implementation, the function splits into two recursive calls for each value of `n`. The run time grows exponentially, making algorithms of this complexity inefficient for large `n`. Being aware of exponential time complexities is crucial for developers to identify and refactor potentially harmful code.
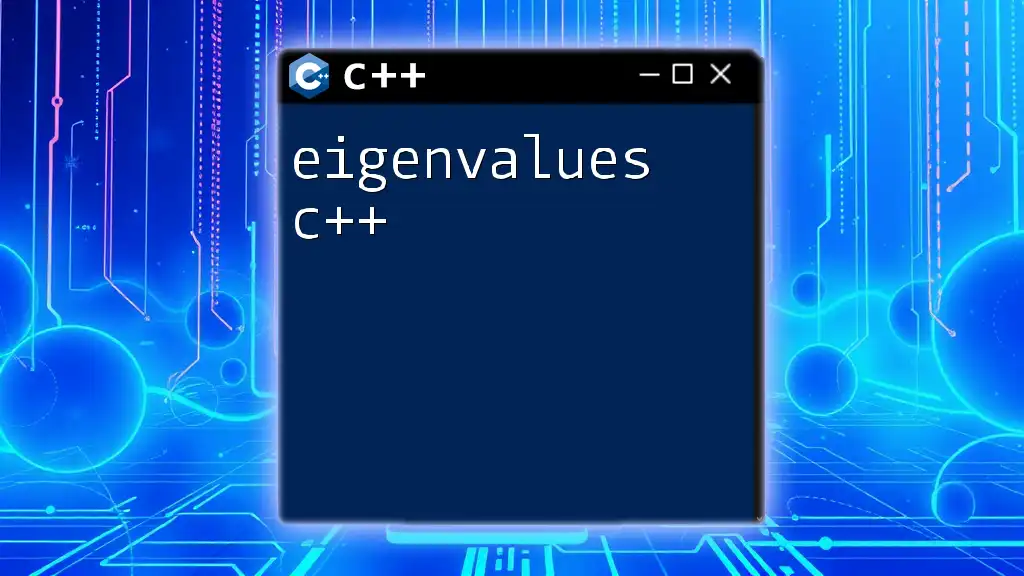
Real-World Applications of Big O Analysis
Importance in Software Development
Effective Big O analysis in algorithm design is critical for delivering high-performing applications. Poorly optimized algorithms not only degrade performance but can also lead to user dissatisfaction due to slow response times. For instance, an O(n²) algorithm could be feasible for small datasets but can become problematic as the dataset size explodes into millions of entries, crippling the application.
Performance Testing in C++
To ensure favorable performance, C++ developers often leverage various tools for analyzing complexity. For example, using the `<chrono>` library allows programmers to measure execution time effectively.
Example of measuring execution time:
#include <iostream>
#include <chrono>
void sampleFunction() {
// Sample code to measure
}
int main() {
auto start = std::chrono::high_resolution_clock::now();
sampleFunction();
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double> duration = end - start;
std::cout << "Execution time: " << duration.count() << " seconds\n";
return 0;
}
By intentionally testing algorithms with various input sizes, developers can gain insights into performance characteristics, helping them refine their code.
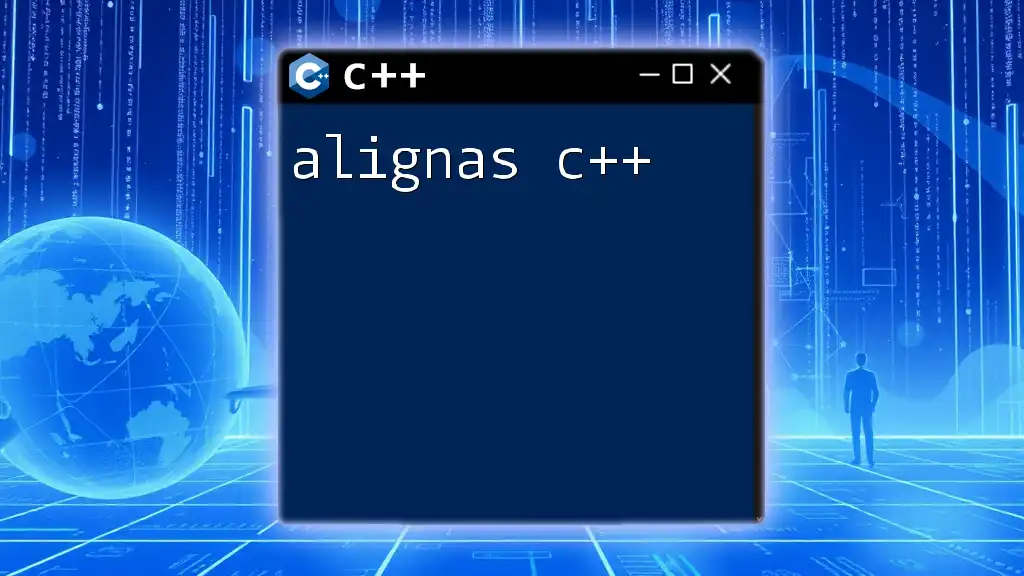
Tips for Analyzing Big O in Your Code
When evaluating the performance of your algorithms, consider the following:
- Identify the basic operations: Focus on the operations that will have the largest impact on execution time as the data size grows.
- Count control structures: Analyze loops and recursive calls and their effects on run time.
- Ignore constant factors: When expressing Big O, coefficients and lower-order terms do not matter, so simplify your expression to its leading term.
- Test and profile: Utilize profiling tools and benchmarking to compare the execution time of different implementations and verify your analysis.
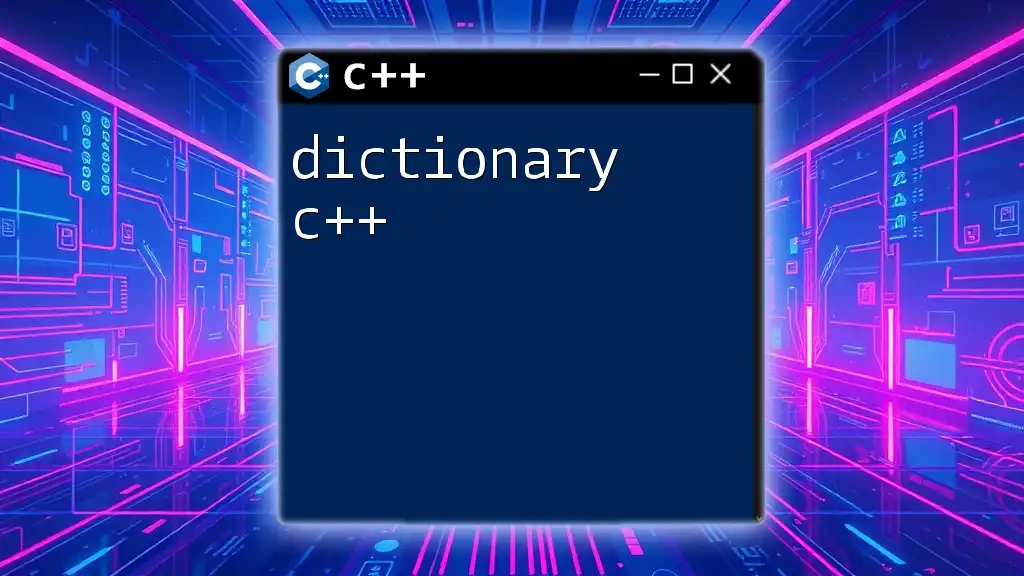
Conclusion
Understanding Big O analysis in C++ is essential for every programmer striving to write efficient code. By grasping the various time complexities, the implications of algorithm choice, and effective testing methods, you can significantly improve application performance and ensure a positive user experience. Continual learning and optimization are key in the ever-evolving landscape of software development.
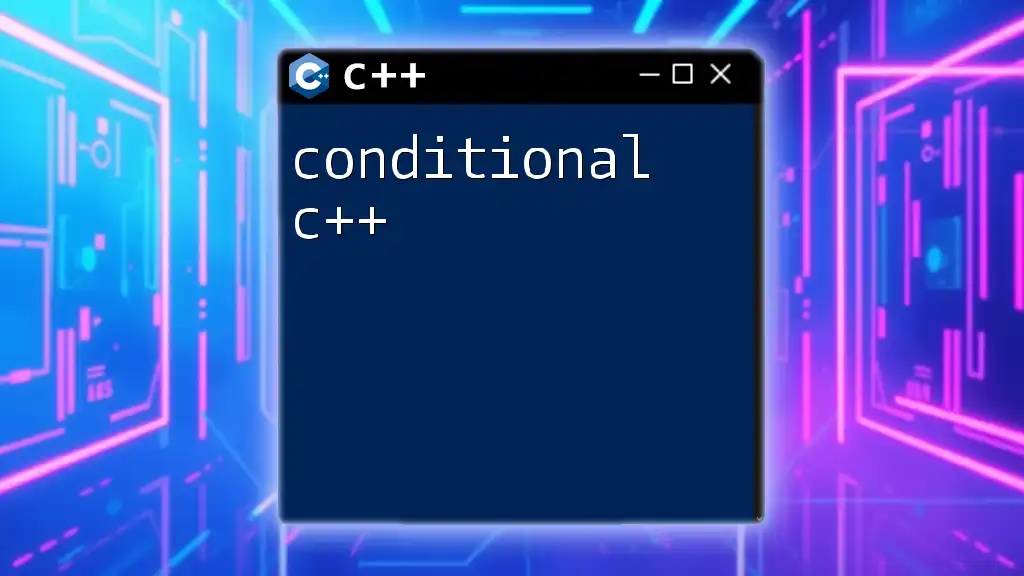
Further Reading and Resources
For those looking to deepen their understanding of Big O analysis in C++, consider exploring recommended books, online courses, and communities that focus on algorithm design and performance optimization. Engaging with the broader programming community can enhance your knowledge and help you stay updated on best practices.