Lambdas in C++ provide a convenient way to define inline functions for custom sorting algorithms, allowing you to easily specify the sorting criteria directly within the sort function.
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {5, 3, 9, 1, 4};
std::sort(numbers.begin(), numbers.end(), [](int a, int b) { return a > b; });
for (int n : numbers) std::cout << n << " "; // Output: 9 5 4 3 1
}
Understanding Sorting in C++
Sorting is a fundamental operation in programming, as it allows for organizing data in a specific order, making it easier to search and analyze. In C++, sorting can be done using various algorithms and functions, with the `std::sort` function from the Standard Library being the most commonly used.
The Importance of Sorting
Sorting is essential in many applications, from preparing data for analysis to optimizing search algorithms. Efficient sorting algorithms reduce the time complexity of searching operations, making programs run faster and enhancing user experience.
C++ Sorting Functions
The C++ Standard Library provides versatile sorting functions. Using `std::sort`, developers can quickly sort elements in an array or container. However, often there is a need for custom sorting criteria to meet specific requirements, and this is where lambdas excel.
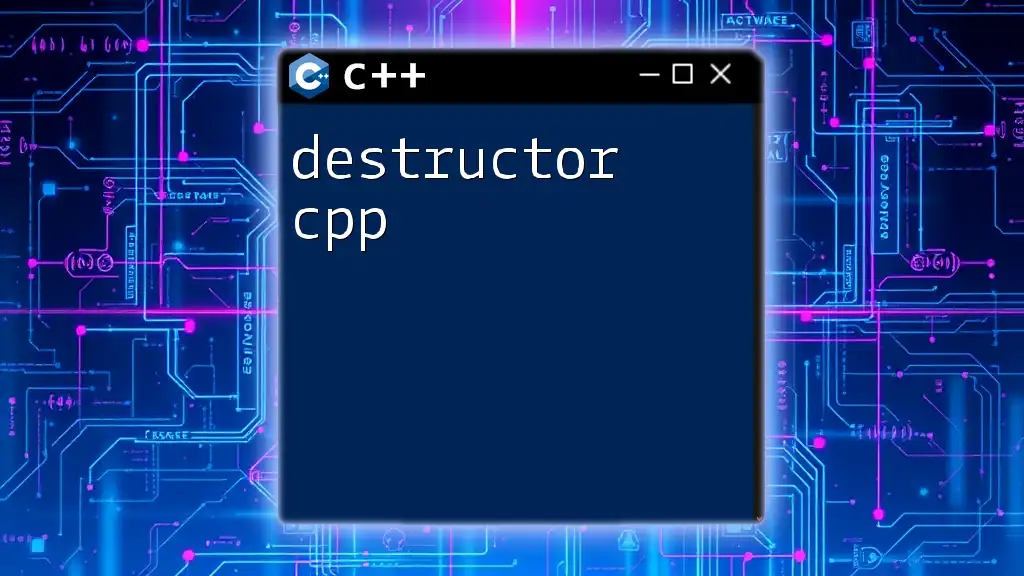
What is a Lambda Expression in C++?
Definition of Lambdas
Lambda expressions are a powerful feature that emerged in C++11, allowing programmers to define anonymous functions directly within their code. The basic syntax of a lambda expression looks like this:
[] (parameters) { body }
Advantages of Using Lambdas
Lambdas offer several advantages over traditional function pointers. They provide enhanced conciseness, eliminating the need for defining separate functions for simple operations. Moreover, lambdas can capture variables from their surrounding context, granting access to local variables without needing to pass them explicitly as parameters.
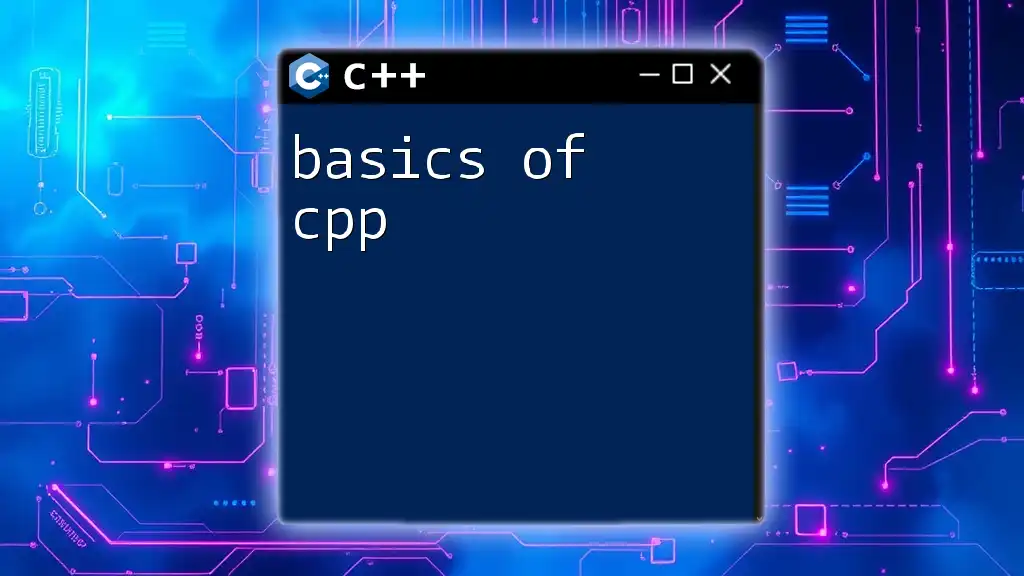
Using Lambdas for Custom Sorting
Custom Sorting with `std::sort`
To utilize custom sorting, we first need to understand how `std::sort` operates. This function requires two iterators and, optionally, a comparator function to determine the order. By default, `std::sort` sorts in ascending order.
Here’s a simple usage example:
std::vector<int> vec = {4, 1, 3, 2};
std::sort(vec.begin(), vec.end());
In this case, the vector `vec` is sorted in ascending order.
Implementing Custom Sort with Lambdas
Ascending Order
If we want to sort the elements in ascending order using a custom lambda, we can implement it as follows:
std::vector<int> vec = {4, 1, 3, 2};
std::sort(vec.begin(), vec.end(), [](int a, int b) { return a < b; });
Here, the lambda expression `[](int a, int b) { return a < b; }` serves as the comparator, explicitly stating that we want the sorting based on ascending order.
Descending Order
To achieve sorting in descending order, we can modify the lambda slightly:
std::sort(vec.begin(), vec.end(), [](int a, int b) { return a > b; });
In this code snippet, the comparison operator has been reversed, indicating that the sorting should now occur in descending order.
Sorting Complex Data Types
Example with Structs
When dealing with more complex data types such as structs, you can still leverage the power of lambdas for custom sorting. Consider the following `struct` definition:
struct Person {
std::string name;
int age;
};
Now let's say we have a vector of `Person` objects that we want to sort by age:
std::vector<Person> people = {{"Alice", 30}, {"Bob", 25}, {"Charlie", 35}};
std::sort(people.begin(), people.end(), [](const Person& a, const Person& b) {
return a.age < b.age;
});
In this example, the lambda captures two `Person` objects and compares their ages, allowing us to sort the `people` vector based on their age in ascending order.
Capturing External Variables in Lambdas
Lambdas can also capture external variables, providing additional flexibility. Let’s look at an example where we sort based on a threshold age defined in the surrounding scope:
int threshold = 30;
std::sort(people.begin(), people.end(), [threshold](const Person& a, const Person& b) {
return a.age < b.age && a.age < threshold;
});
In this case, the lambda captures the `threshold` variable, implying that only individuals younger than the threshold will be considered in the sorting criteria. Capturing allows for dynamic behavior based on external conditions, which can greatly enhance sorting functionality.
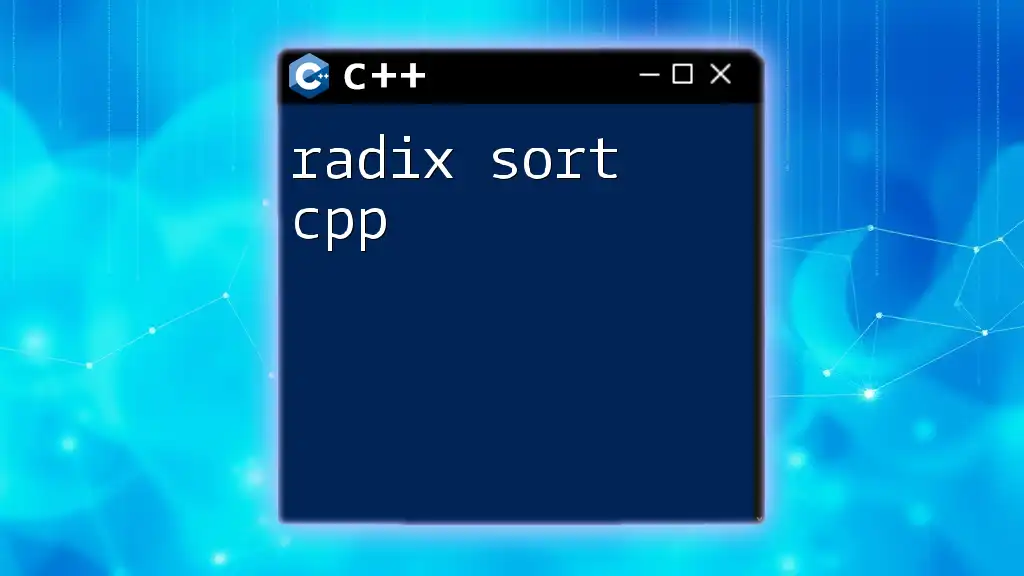
Best Practices for Using Lambdas in Sorting
Keep it Simple
When using lambdas, it is often best to keep the lambda body concise. Complex logic may confuse readers and diminish readability. If the logic becomes too convoluted, consider defining a separate comparator function. This can enhance clarity and maintainability.
Performance Considerations
While lambdas are convenient, it’s essential to be aware of any potential performance implications. Generally, the differences will be negligible for typical applications. However, performance-critical code should always be profiled to ensure that the chosen method is optimal.
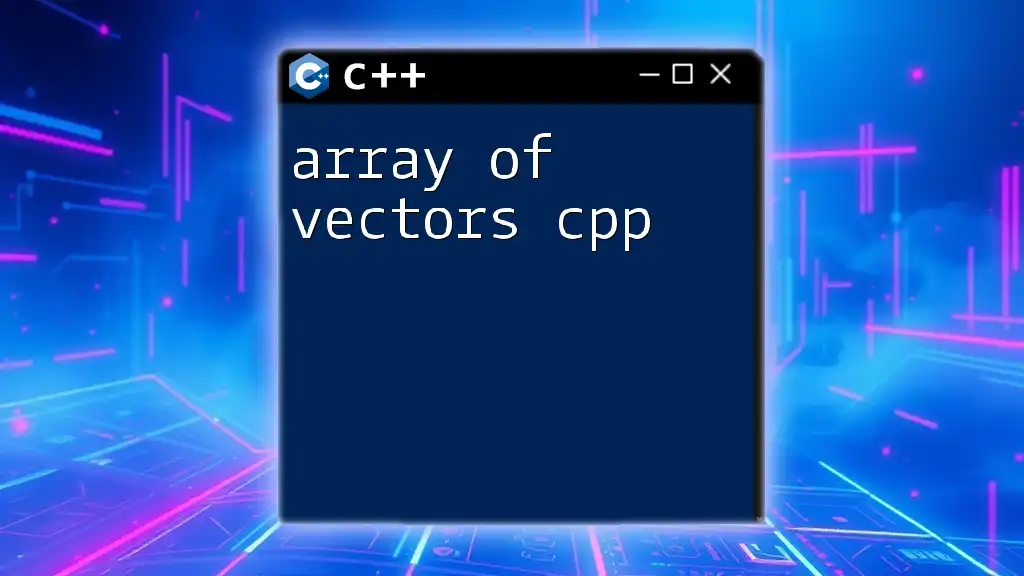
Conclusion
Lambdas are a powerful feature in C++ that greatly simplify custom sorting operations. They provide a way to efficiently define sorting criteria, leading to more readable and maintainable code. Whether you're sorting primitive data types or complex structures, lambdas for custom sort in C++ offer exceptional flexibility and ease of use.
Experiment with your own data types and sorting criteria to truly master this feature of modern C++. Happy coding!