To access private members of a class in C++, you can define a public member function within the class or use a friend function, as shown in the following code snippet:
#include <iostream>
using namespace std;
class MyClass {
private:
int privateVar;
public:
MyClass(int val) : privateVar(val) {} // Constructor
// Public function to access private member
int getPrivateVar() const { return privateVar; }
// Friend function to access private member
friend void accessPrivate(MyClass &obj);
};
void accessPrivate(MyClass &obj) {
cout << "Private Variable: " << obj.privateVar << endl;
}
int main() {
MyClass obj(42);
cout << "Access via public function: " << obj.getPrivateVar() << endl;
accessPrivate(obj); // Accessing via friend function
return 0;
}
Understanding Class Members
What are Class Members?
In C++, class members refer to the variables and functions that are defined within a class. These members play a crucial role in determining the behavior and characteristics of objects created from that class. There are three types of class members:
- Public members: Accessible from anywhere in the program.
- Protected members: Accessible only within the class and by derived classes.
- Private members: Accessible only within the defining class, providing a level of encapsulation.
The Role of Private Members
Private members serve as an essential tool in encapsulation, a fundamental principle of object-oriented programming. By restricting direct access to certain data, private members protect the integrity of the class's data and prevent unintended interference or misuse. Using private members allows for controlled interaction through public member functions, ensuring that the state of an object is maintained correctly.
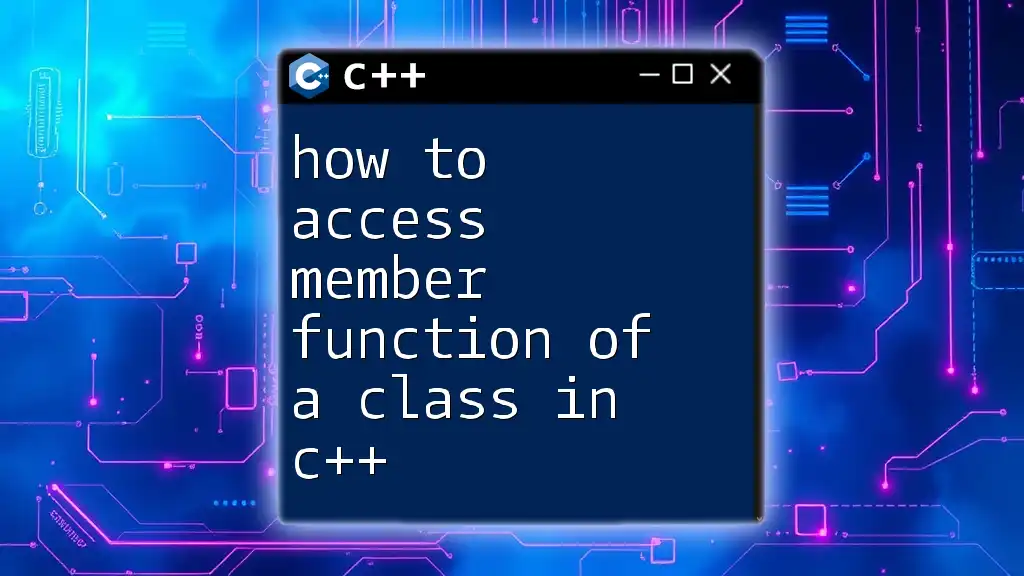
Accessing Private Members
When exploring how to access private members of a class in C++, there are a few widely accepted methods that maintain encapsulation while allowing necessary access.
Using Public Member Functions
One of the most common and straightforward methods to access private members is through public member functions. This approach allows external code to interact with private data without directly accessing it, which preserves the class's encapsulation.
Here’s an example to illustrate this:
class MyClass {
private:
int privateVariable;
public:
MyClass(int value) : privateVariable(value) {}
int getPrivateVariable() { return privateVariable; }
};
int main() {
MyClass obj(10);
std::cout << obj.getPrivateVariable(); // Accessing private member via public function
}
In this code snippet, the `getPrivateVariable()` function is a public member function that safely exposes the value of `privateVariable`. When you call `obj.getPrivateVariable()`, it retrieves the private member without violating encapsulation principles.
Friends of the Class
Another approach for accessing private members is by declaring a function or another class as a friend of the given class. Friend functions have the ability to access private and protected members directly.
Here's how this works:
class MyClass {
private:
int privateVariable;
public:
MyClass(int value) : privateVariable(value) {}
friend void accessPrivateMember(MyClass obj);
};
void accessPrivateMember(MyClass obj) {
std::cout << obj.privateVariable; // Accessing private member directly
}
int main() {
MyClass obj(10);
accessPrivateMember(obj);
}
In this example, the `accessPrivateMember` function is declared as a friend of `MyClass`. This means it can access the private member `privateVariable` directly, allowing for easy manipulation of private data. However, this method should be used with caution; becoming overly reliant on friend functions can lead to a breakdown of encapsulation.
Inheritance and Protected Members
Protected members come into play when dealing with inheritance. Although private members cannot be accessed by derived classes, they can be accessed indirectly through protected member functions.
Consider the following example:
class Base {
private:
int privateVariable;
protected:
Base(int value) : privateVariable(value) {}
int getPrivateVariable() { return privateVariable; }
};
class Derived : public Base {
public:
Derived(int value) : Base(value) {}
void showPrivateVariable() {
std::cout << getPrivateVariable(); // Accessing via protected member function
}
};
int main() {
Derived obj(10);
obj.showPrivateVariable();
}
In this case, the `Base` class has a private member `privateVariable`. Although `Derived` cannot directly access it, it can call the protected function `getPrivateVariable()` to retrieve its value. This approach maintains encapsulation while still allowing derived classes to access essential data.
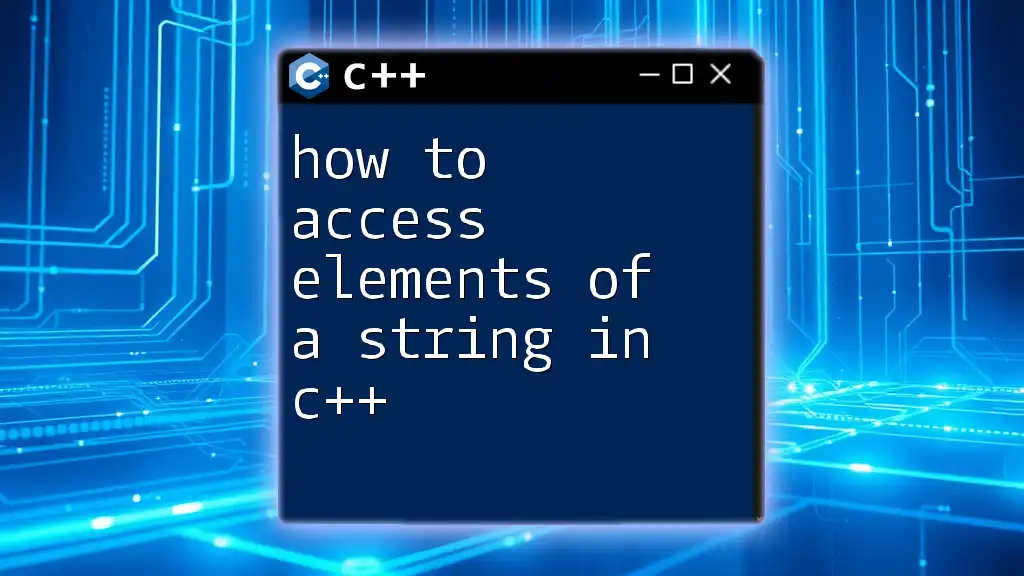
Best Practices for Accessing Private Members
When to Use Public Functions
When determining how to access private members of a class in C++, the first approach should always be through well-defined public member functions. These functions should be designed to allow controlled access to private data. This not only maintains encapsulation but also makes it easier to modify the underlying implementation without affecting external code that relies on the public interface.
Cautions with Friends
While friend functions and classes can provide powerful access to private members, they can also introduce risks. A key caution is that friends can bypass the encapsulation safeguards that a private member provides. Consequently, it is advisable to limit friendship to essential scenarios only, and developers should regularly evaluate whether a member truly needs to be a friend.
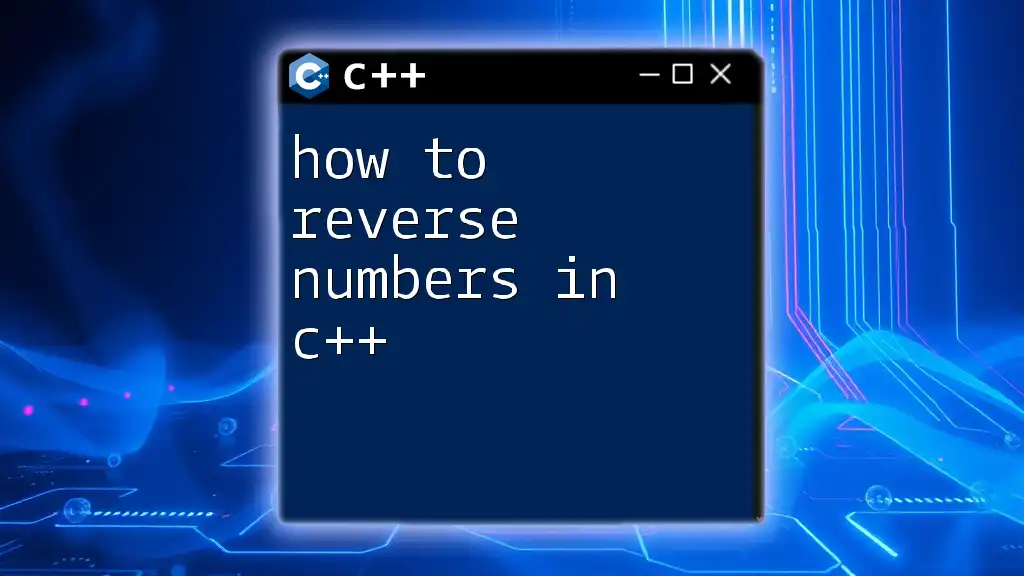
Conclusion
In summary, understanding how to access private members of a class in C++ is crucial for effective object-oriented programming. Whether you opt to use public member functions, friend functions, or protected members through inheritance, the essential goal remains the same: to maintain the integrity of your class's data while providing necessary access. By adhering to best practices and remaining aware of the implications of access control, you can design robust, maintainable C++ classes that adhere to the principles of encapsulation.