C++ Plain Old Data (POD) refers to simple data structures that are compatible with C-style structures and have no user-defined constructors, destructors, or virtual functions, making them easy to manage and use in various programming contexts.
Here's a code snippet demonstrating a C++ POD struct:
struct Point {
int x;
int y;
};
Point p1 = {10, 20};
Understanding C++ Data Types
Fundamental Data Types
C++ offers several built-in types, commonly referred to as fundamental data types. These include:
- Integral Types: Such as `int`, `char`, `short`, `long`, and `bool`. Each has a specific range and size, depending on the system architecture.
- Floating Point Types: Including `float`, `double`, and `long double`. These types are crucial for representing real numbers, with varying degrees of precision.
In addition to built-in types, C++ also supports derived types, which include:
- Arrays: A collection of items stored at contiguous memory locations, all of the same type.
- Pointers: Variables that store memory addresses of other variables, providing a powerful way to work with dynamic memory.
- References: An alias for another variable, allowing a function or method to modify the variable’s value without making a copy.
Introduction to User-defined Types
C++ allows developers to create user-defined types, which are essential for representing complex data structures. Some of the most common user-defined types include:
-
Structures (`struct`): These are collections of various types grouped together under a single name. A `struct` allows you to bundle related data, enhancing code organization.
-
Unions: A union stores different data types in the same memory location. Only one member can contain a value at any given time, which helps save memory but requires careful management.
-
Enumerations (`enum`): Enums provide a way to define variables that can hold only a limited set of values. They enhance code readability by allowing the use of descriptive names instead of arbitrary numbers.
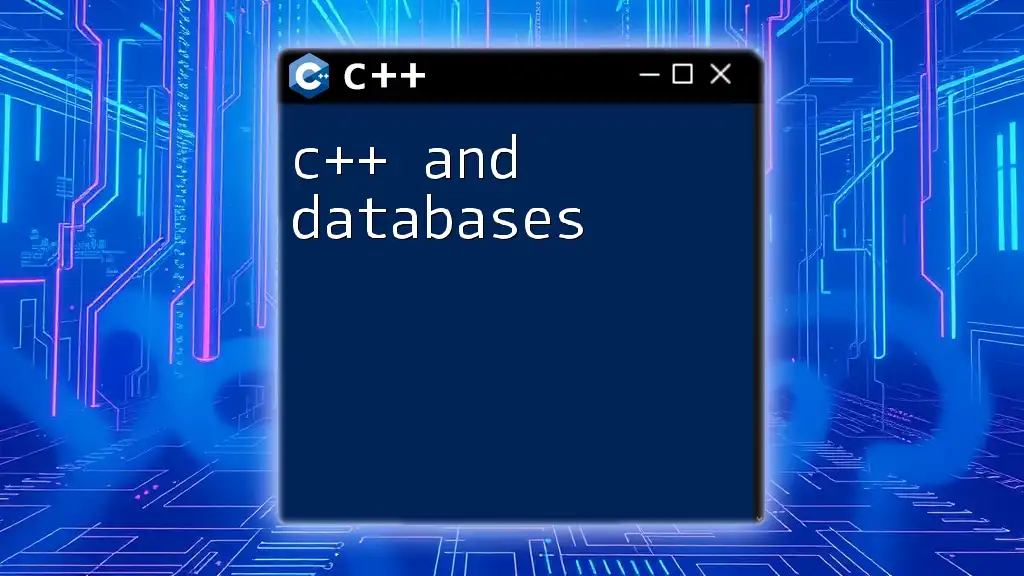
Characteristics of Plain Old Data
Definition of POD in C++
In C++, Plain Old Data (POD) types are defined as simple data types that adhere to specific characteristics. A type is considered POD if:
-
Trivial Types: These data types have trivial constructors, copy constructors, destructors, and assignment operators. This means that the default functionality handles them smoothly without developer-defined behavior.
-
Standard Layout: The layout of data in memory should be such that there are no surprising differences across compilers, making data structures predictable.
Understanding POD is vital because it allows for efficient memory layout and access patterns that enhance performance in C++ applications.
Differences Between POD and Non-POD Types
The distinction between POD and non-POD types is significant, particularly in C++ due to its complexity in memory management:
-
Memory Layout: POD types are often laid out contiguously in memory, which permits faster access due to improved cache performance. Non-POD types (like classes with virtual functions) can introduce additional overhead because of their memory layout.
-
Constructors and Destructors: One of the defining characteristics of POD types is their lack of user-defined constructors and destructors. This absence promotes simpler memory management since POD types demand less overhead.
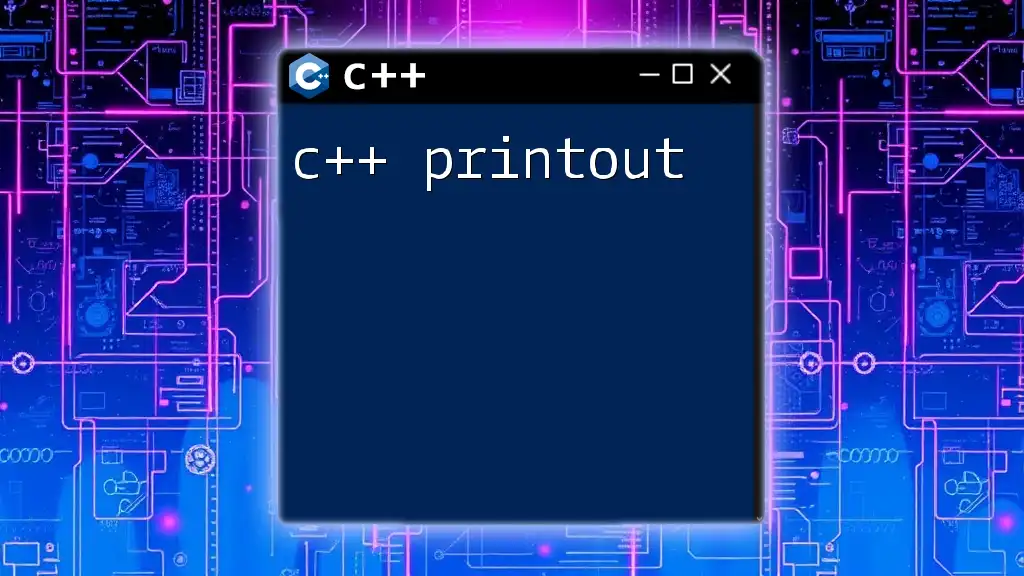
Creating Plain Old Data Structures in C++
Defining a Simple POD Structure
Creating a POD structure in C++ is straightforward. Here’s an example of a basic POD structure defining a two-dimensional point:
struct Point {
int x;
int y;
};
In this example, `Point` is a simple structure containing two integer components, `x` and `y`. These variables are straightforward data members, meeting the criteria of trivially constructible and destructible, which defines a POD type.
Using POD in Arrays
One of the advantages of using POD is the ability to create arrays of POD structures efficiently. For example:
Point points[5]; // Array of POD structures
This declaration creates an array `points` that can hold five `Point` structures. The usage of arrays with POD ensures that memory is allocated contiguously, leading to faster access times.
To access and modify elements within an array of POD structures, one can use simple indexing:
points[0].x = 10;
points[0].y = 20;
This snippet sets the `x` and `y` members of the first `Point` in the array.
POD with Functions
POD types are also efficient when passed to functions. Consider the following function that takes a reference to a `Point`:
void printPoint(const Point &p) {
std::cout << "Point(" << p.x << ", " << p.y << ")" << std::endl;
}
By passing the `Point` structure by reference, we avoid a copy of the `Point`, which further enhances performance and minimizes memory overhead. This approach underscores the versatility and efficiency of using POD types in C++.
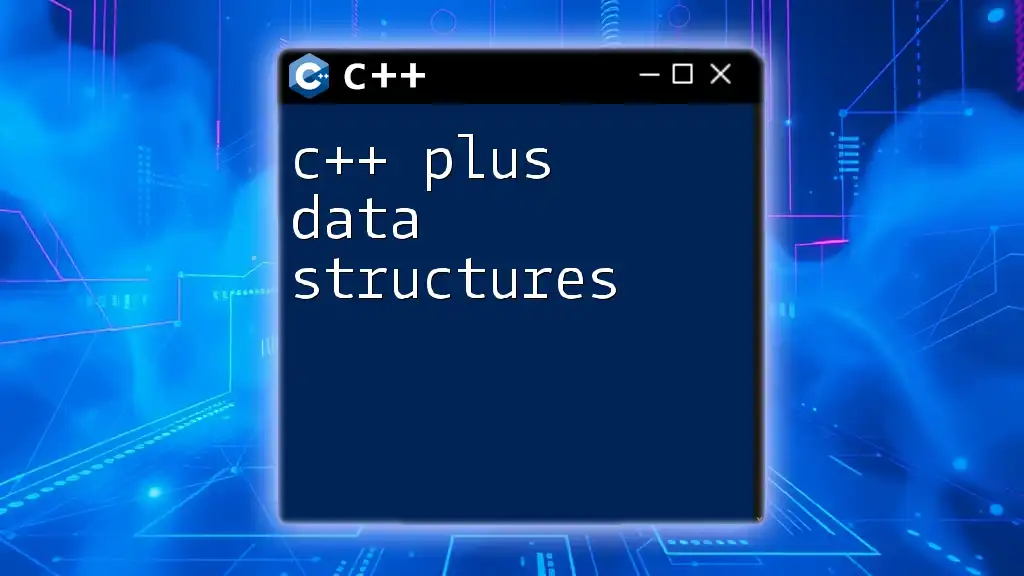
Best Practices for Utilizing Plain Old Data
When to Use POD
Understanding when to use POD types can greatly impact the performance of an application. When your data structures do not need the flexibility of classes, utilizing POD is often preferable. Performance considerations suggest that:
- Memory Management: With POD structures, memory management is typically less complex, meaning fewer chances for memory leaks. Also, the efficient memory layout contributes to overall improvements in performance.
Avoiding Common Pitfalls
In C++, certain pitfalls can arise when using POD. One key issue is object slicing. Consider the following illustration:
struct Base {
int data;
};
struct Derived : Base {
double extraData;
};
void func(Base b) { /* ... */ } // Object slicing occurs here
When `Derived` is passed to `func`, it’s sliced down to `Base`, losing the `extraData` member. To avoid this, always use pointers or references when dealing with derived types within POD contexts.
Furthermore, understanding how copy behavior works with POD types will prevent unwanted side effects. POD types can be copied safely without invoking complex copy constructors or destructors.
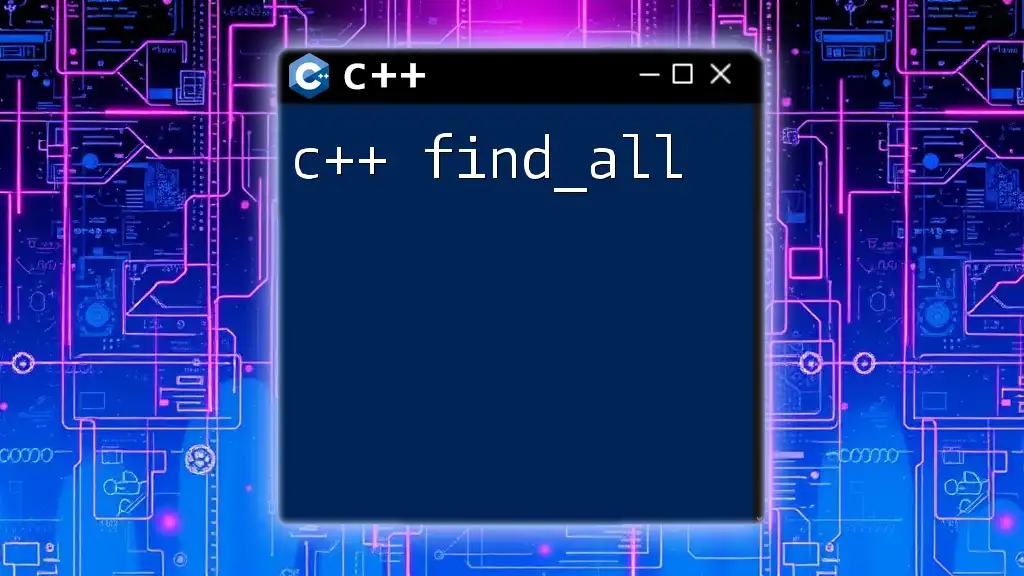
Conclusion
In summary, mastering C++ Plain Old Data is essential for writing efficient, understandable, and manageable code. Understanding the characteristics, applications, and best practices associated with POD allows developers to leverage the strengths of C++ by creating simple, efficient data structures tailored for performance. As the programming landscape continues to evolve, knowledge of POD will remain a valuable asset in a C++ developer's toolkit.