In C++, "cpp 1" typically refers to the foundational concept of the C++ programming language, emphasizing its basic syntax and structure, such as the declaration of a simple "Hello, World!" program.
Here's a code snippet demonstrating this:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Basic Syntax and Structure
The Structure of a C++ Program
Understanding the structure of a C++ program is crucial for beginners. Each program begins with the preprocessor directive `#include`, which allows the program to use libraries, like `iostream` for input and output. Following this is the `main` function, which serves as the entry point of the program.
Here's a simple C++ program that demonstrates these components:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example:
- `#include <iostream>` includes the standard input-output stream library.
- `int main()` is the main function where execution starts.
- `std::cout` is used to output data to the console, while `std::endl` ends the line and flushes the output buffer.
- `return 0;` indicates that the program finished successfully.
Data Types and Variables
C++ supports various fundamental data types. Understanding these is pivotal as they form the backbone of variable declarations, which are essential for storing and manipulating data.
- Integer: Used for whole numbers.
- Float: Used for single-precision floating-point numbers.
- Double: Used for double-precision floating-point numbers.
- Char: Used for single characters.
- Boolean: Represents true or false values.
Declaring variables involves specifying the data type followed by the variable name. Initialization can be done at the time of declaration or later in the program.
Here's an example of variable declaration and initialization:
int age = 25;
float salary = 65000.50;
char grade = 'A';
bool isEmployed = true;
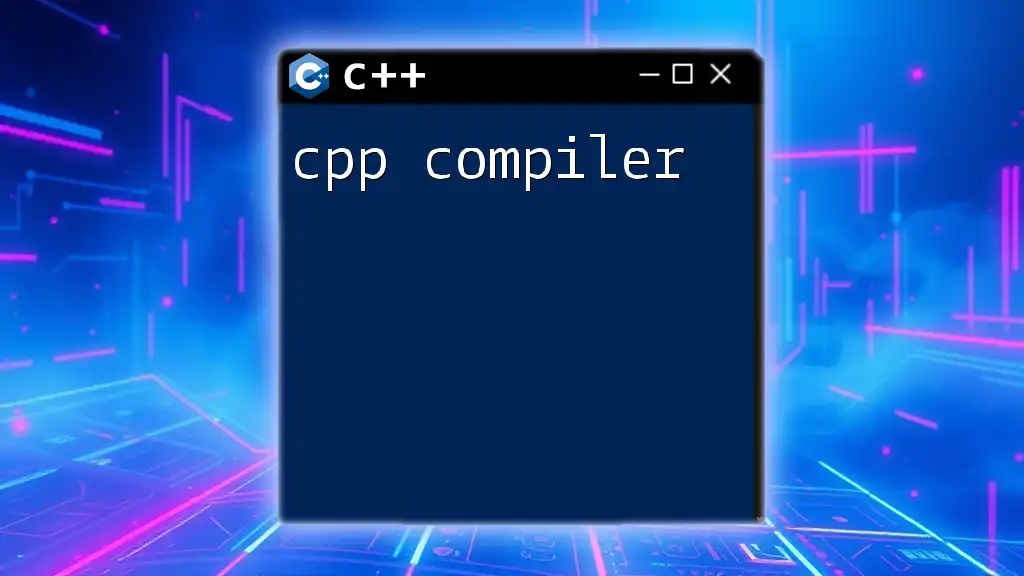
Control Flow Statements
Conditional Statements
Conditional statements allow the program to execute different code blocks based on certain conditions. The if-else construct is one of the most commonly used conditional statements in C++.
An example of an if-else statement is as follows:
if (age >= 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
This code checks if `age` is greater than or equal to 18 and prints "Adult"; otherwise, it prints "Minor".
Another useful conditional structure is the switch statement, which allows multiple possible execution paths based on the value of a single expression.
Looping Constructs
Looping constructs in C++ enable repeated execution of code based on certain conditions. The most commonly used loops are the for loop and while loop.
A simple example of a for loop is:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
This loop will print numbers from 0 to 4. The initialization occurs with `int i = 0`, the condition checked is `i < 5`, and `i++` is executed at the end of each iteration.
Similarly, a while loop can be used as follows:
int i = 0;
while (i < 5) {
std::cout << i << std::endl;
i++;
}
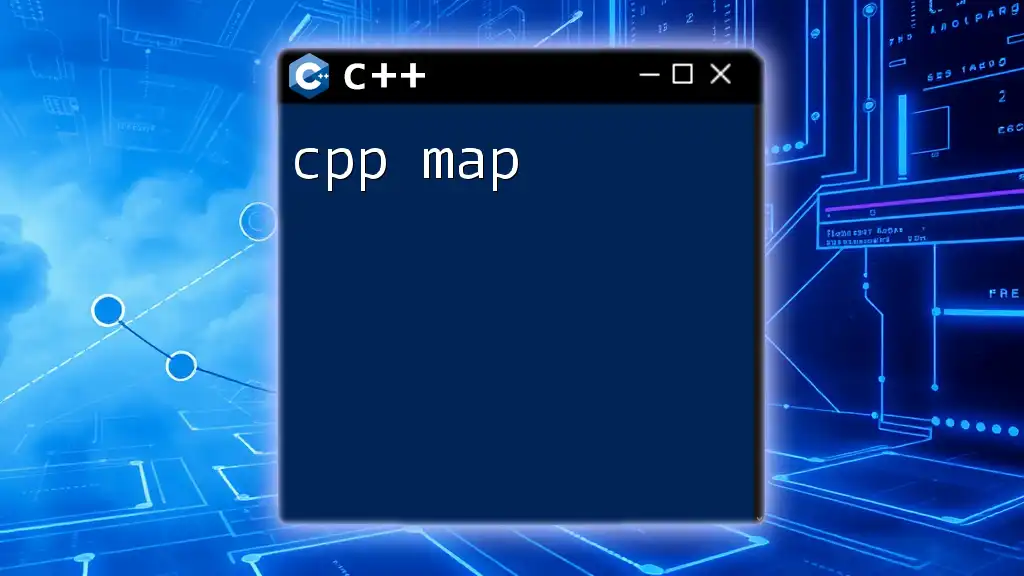
Functions in C++
Defining Functions
Functions allow developers to encapsulate reusable code into manageable blocks. A function typically has a return type, a name, and a list of parameters.
Here’s how you can define a simple addition function:
int add(int a, int b) {
return a + b;
}
To call this function, you simply use its name and supply the necessary parameters:
int result = add(5, 10); // result holds the value 15
Function Overloading
C++ supports function overloading, allowing multiple functions with the same name but different parameters. This feature enhances the flexibility of your programs.
Here’s an example of function overloading for the `add` function:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
With this, you can call `add(5, 10)` for integers or `add(5.2, 3.8)` for doubles, and the appropriate function will be executed based on the types of the arguments.
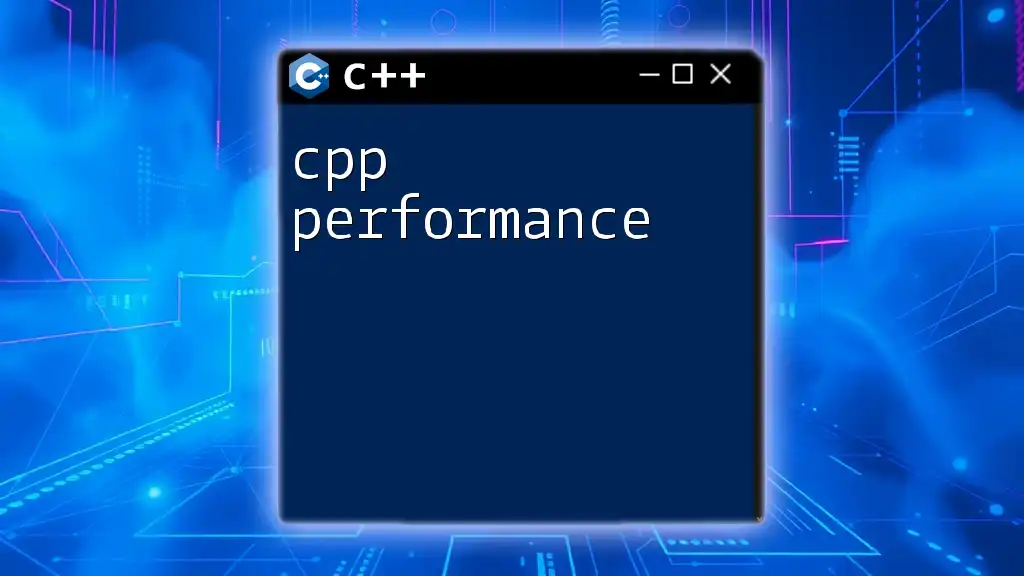
Object-Oriented Programming in C++
Introduction to OOP
C++ is an object-oriented programming language that facilitates a methodology of programming characterized by the concepts of classes and objects.
Key concepts include:
- Classes: Blueprints for creating objects containing data and functions.
- Objects: Instances of classes.
- Encapsulation: Bundling data and methods that operate on the data.
- Inheritance: Mechanism for creating new classes from existing ones.
- Polymorphism: Ability to treat objects of different classes through a common interface.
Creating Classes and Objects
To create a class in C++, you can use the following syntax:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
To instantiate an object of this class, you can do so as follows:
Dog myDog;
myDog.bark(); // Outputs: Woof!
Constructors and Destructors
Constructors are special class functions that are called when an object of that class is created. They often initialize members of the class. Conversely, destructors are called when an object is destroyed and are used to free resources.
Here’s an example:
class Car {
public:
Car() {
std::cout << "Car created." << std::endl;
}
~Car() {
std::cout << "Car destroyed." << std::endl;
}
};
In this example, when an object of `Car` is created, "Car created." is printed. When the object goes out of scope, "Car destroyed." is displayed.
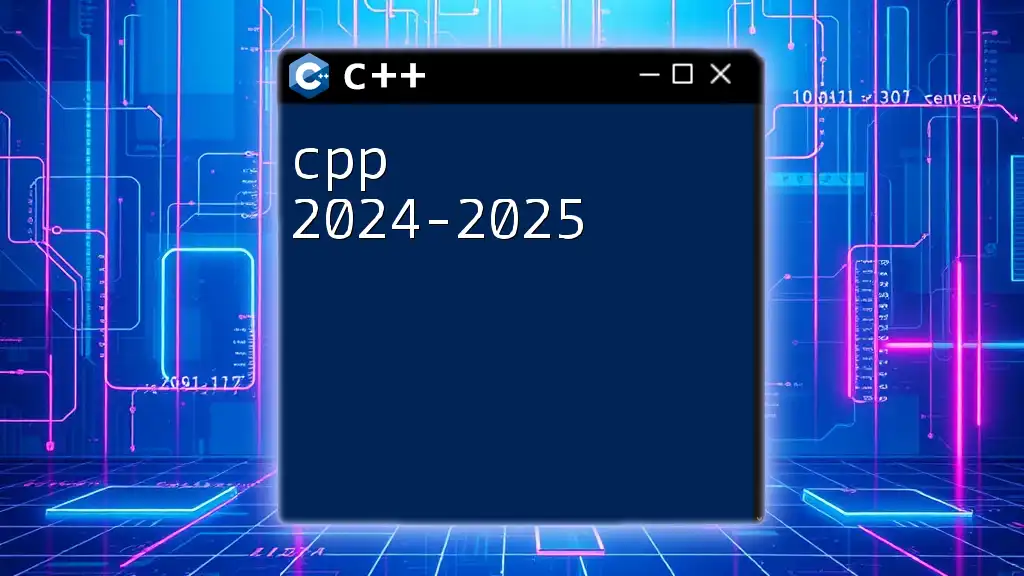
Conclusion
This guide has provided an overview of C++ 1, highlighting the foundational concepts such as basic syntax, data types, control statements, functions, and object-oriented programming. Each section included examples designed to reinforce your learning and encourage hands-on practice.
As you progress, the next steps for mastering C++ involve exploring more advanced topics, such as templates, the Standard Template Library (STL), and multi-threading. Ensure to engage with coding exercises and personal projects to solidify your understanding. Exploring these concepts will develop your proficiency and confidence in C++.