In C++, the error "cout is ambiguous" occurs when the compiler cannot determine which overloaded version of `cout` to use due to conflicting namespaces or declarations.
Here’s a simple code snippet that demonstrates this issue:
#include <iostream>
using namespace std; // Ensure we are using the correct namespace
int main() {
// Uncommenting one of the two following lines will cause ambiguity
// int cout = 5; // This creates ambiguity for the std::cout
cout << "Hello, World!" << endl; // This line works fine when ambiguity is resolved
return 0;
}
What is cout in C++?
`cout`, short for "character output," is a fundamental part of the C++ Standard Library. It is used primarily for outputting data to the standard console (usually the screen) and is integral to almost every C++ program.
The syntax for using `cout` is straightforward and mirrors the typical output operations found in other programming languages. Here's a simple example:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this snippet, we include the `<iostream>` header, which gives us access to `cout`, and we use the `<<` operator to send a string to the console.
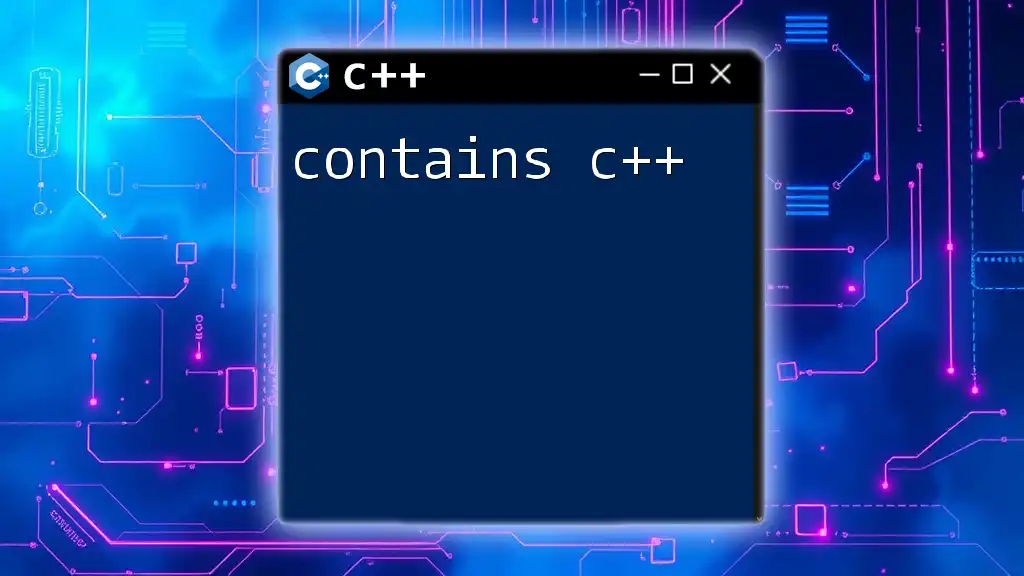
The Ambiguity of cout
Understanding Ambiguity in Programming
In programming, ambiguity refers to scenarios where a language construct can be interpreted in more than one way. When it comes to `cout`, ambiguity can arise due to various factors, such as the overloading of operators and multiple data types interacting with `cout`.
Reasons for cout Ambiguity in C++
Overloaded Operators
C++ supports operator overloading, allowing developers to define custom behaviors for operators like `<<`. This feature is powerful but can lead to ambiguity. For instance, if multiple classes overload the `<<` operator differently, the compiler may struggle to determine which version to use when outputting a specific object type.
Different Data Types
C++ is a statically typed language, which means that the type of each variable must be known at compile time. When using `cout`, attempting to output a variable that might belong to more than one type can confuse the compiler.
Common Sources of Ambiguity
Using User-Defined Types with cout
When you define your own types (e.g., classes), you may overload the `<<` operator to customize how objects are printed to `cout`. However, if multiple classes overload this operator without clear differentiation, you risk creating ambiguities.
For example:
class MyClass {
public:
friend ostream& operator<<(ostream& os, const MyClass& obj) {
os << "Custom Output";
return os;
}
};
If you attempt to print an object of `MyClass` without specifying how `cout` should handle it, ambiguous situations may arise, especially if similar classes exist with their own overloads.
Ambiguous Output with Multiple Libraries
Another source of ambiguity can occur when different libraries define their own versions of `cout`, especially if they exist in different namespaces. If you try to use `cout` without specifying a namespace, the compiler might not know which version you are referring to, leading to confusion.
Real-World Examples of C++ cout is Ambiguous
Example 1: Operator Overloading Conflicts
Consider two classes that both overload the `<<` operator:
class ClassA {
public:
friend ostream& operator<<(ostream& os, const ClassA& obj) {
os << "ClassA Output";
return os;
}
};
class ClassB {
public:
friend ostream& operator<<(ostream& os, const ClassB& obj) {
os << "ClassB Output";
return os;
}
};
If you try to use `cout` on an object that could belong to either class, the ambiguity will prevent the code from compiling.
Example 2: Name Clashes with Different Namespaces
Imagine you have two different libraries that both contain definitions for `cout`. If one is included via `using namespace std;` and the other via `using namespace myLib;`, you may face ambiguity unless you specify which `cout` to use in your code, leading to a compilation error.
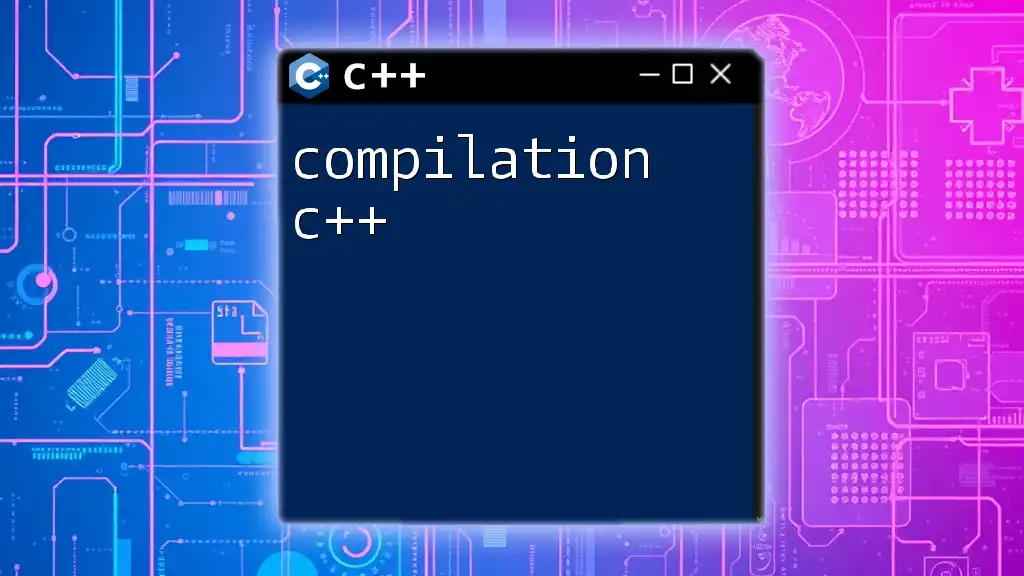
How to Resolve cout Ambiguity
Best Practices for Using cout
Always Specify Namespace
To avoid complications with ambiguity, it's a good practice to use the fully qualified name when working with `cout`:
std::cout << "This is a standard output." << std::endl;
By using `std::cout`, you ensure that your code specifically calls the standard library's version of `cout`, preventing potential conflicts with other libraries that might define their own output streams.
Explicit Casting
When dealing with mixed data types or user-defined types, explicit casting can clarify your intentions and resolve ambiguities:
double num = 5.5;
std::cout << static_cast<int>(num) << std::endl; // Outputs: 5
Using `static_cast` allows the compiler to deduce the exact type you wish to output, thus eliminating ambiguity.
Troubleshooting Ambiguity with cout
Using Compiler Errors as Guide
Compilers are typically quite effective at pointing out where ambiguity arises when using `cout`. Take note of compiler error messages; they often provide insights into which types or operators are causing the issue.
Debugging Techniques
If you encounter ambiguous situations, follow these steps to isolate the problem:
- Read the error message carefully. Understanding what the compiler is indicating can help you pinpoint ambiguities in your code.
- Identify conflicting types. Check all variables involved in the `cout` statement and their types.
- Simplify the statement. Reduce the complexity of your `cout` statement to determine which part is ambiguous. Once identified, use explicit casting or specify namespaces to clarify the intention.
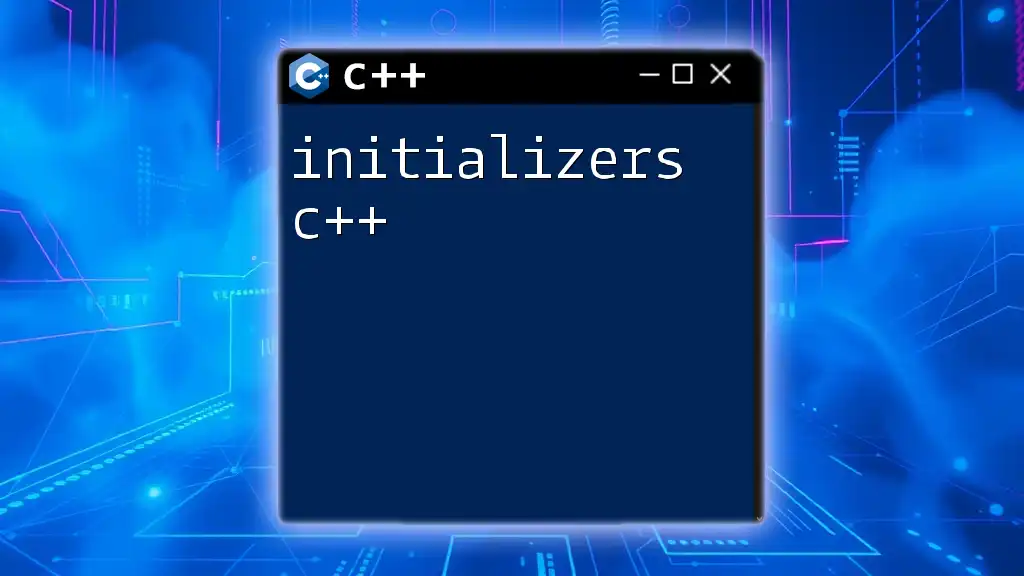
Conclusion
In conclusion, the "cout is ambiguous c++" issue is a common challenge faced by C++ programmers. Understanding why `cout` can be ambiguous, particularly when using overloaded operators or dealing with multiple libraries, is critical for writing reliable code. By adhering to best practices—such as always specifying namespaces and using explicit casting—you can mitigate ambiguity and enhance the clarity of your output statements. Remember, the key to resolving ambiguity lies in clear definitions and understanding the types you work with in C++. Through careful attention to detail, you can navigate the complexities of C++ output effectively.
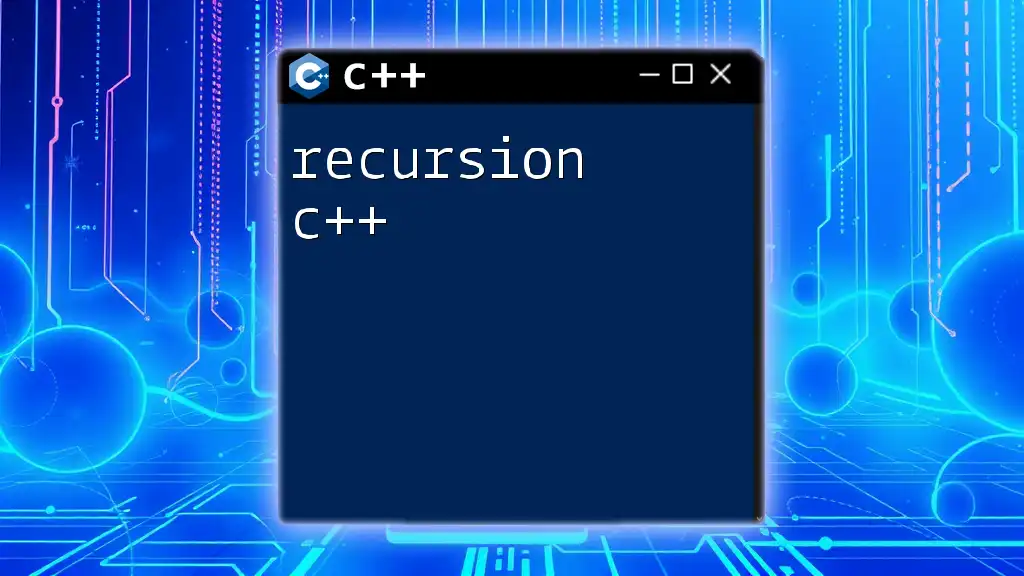
Resources and Further Reading
To deepen your understanding of C++ and the nuances of `cout`, explore the following resources:
- C++ Standard Library documentation (https://en.cppreference.com/w/cpp/io/cout)
- "The C++ Programming Language" by Bjarne Stroustrup for an authoritative overview of C++
- Online coding platforms such as LeetCode or Codecademy to practice and apply your knowledge in real-world programming challenges.